Python将多行数据以一行输出的几种方式有:使用字符串连接、列表解析、join
方法、正则表达式。 其中,最常用且简洁的方法是使用字符串的join
方法。join
方法通过指定的分隔符将一个可迭代对象中的元素连接成一个字符串。下面详细描述如何使用join
方法将多行数据合并为一行。
一、字符串连接
字符串连接是最简单直接的方法。可以使用加号(+
)将多行字符串连接成一行。虽然这种方法容易理解,但在处理大量数据时效率较低。
line1 = "This is the first line."
line2 = "This is the second line."
line3 = "This is the third line."
single_line = line1 + " " + line2 + " " + line3
print(single_line)
二、列表解析
列表解析是一种高效且易读的方法来处理多行数据。通过将每一行数据存储在列表中,并使用join
方法将其连接成一个字符串。
lines = [
"This is the first line.",
"This is the second line.",
"This is the third line."
]
single_line = " ".join(lines)
print(single_line)
三、join
方法
join
方法是处理多行数据的推荐方式,因为它不仅简洁,而且性能优越。下面是一个示例,展示了如何使用join
方法将多行数据合并为一行。
lines = [
"This is the first line.",
"This is the second line.",
"This is the third line."
]
single_line = " ".join(lines)
print(single_line)
四、正则表达式
正则表达式也是一种强大的工具,可以用来处理复杂的字符串操作。通过使用re
模块,可以轻松地将多行数据合并为一行。
import re
multiline_string = """
This is the first line.
This is the second line.
This is the third line.
"""
single_line = re.sub(r'\s+', ' ', multiline_string).strip()
print(single_line)
五、实际应用场景
1. 处理文本文件
在处理文本文件时,通常需要将文件内容读取并合并成一行,以便进行进一步处理。
with open('multiline_file.txt', 'r') as file:
lines = file.readlines()
single_line = " ".join(line.strip() for line in lines)
print(single_line)
2. 数据清洗
在数据科学和机器学习中,数据清洗是一个重要的步骤。将多行数据合并为一行可以简化数据处理过程。
data = """
Line 1: Data point one.
Line 2: Data point two.
Line 3: Data point three.
"""
cleaned_data = re.sub(r'\s+', ' ', data).strip()
print(cleaned_data)
3. 日志处理
在日志处理和分析时,将多行日志记录合并为一行,有助于提高可读性和简化分析过程。
log_entries = [
"2023-10-01 10:00:00 - INFO - Start process.",
"2023-10-01 10:01:00 - DEBUG - Process running.",
"2023-10-01 10:02:00 - INFO - Process completed."
]
single_log_entry = " ".join(log_entries)
print(single_log_entry)
六、性能比较
不同方法的性能可能有所差异。在处理大量数据时,选择高效的方法尤为重要。下面是对几种方法的性能比较。
import time
Generate a large list of lines
lines = ["Line " + str(i) for i in range(100000)]
Method 1: String concatenation
start_time = time.time()
single_line = ""
for line in lines:
single_line += line + " "
end_time = time.time()
print(f"String concatenation took {end_time - start_time:.2f} seconds")
Method 2: List comprehension + join
start_time = time.time()
single_line = " ".join([line for line in lines])
end_time = time.time()
print(f"List comprehension + join took {end_time - start_time:.2f} seconds")
Method 3: Direct join
start_time = time.time()
single_line = " ".join(lines)
end_time = time.time()
print(f"Direct join took {end_time - start_time:.2f} seconds")
七、注意事项
在处理多行数据时,应注意数据的格式和内容。例如,处理包含换行符、空格和特殊字符的数据时,可能需要额外的预处理步骤。
1. 处理空行
在处理包含空行的数据时,可以使用条件过滤掉空行。
lines = [
"This is the first line.",
"",
"This is the third line."
]
single_line = " ".join(line for line in lines if line.strip())
print(single_line)
2. 处理特殊字符
在处理包含特殊字符的数据时,可以使用正则表达式进行预处理,以确保输出结果符合预期。
import re
data = """
Line 1: Data point one.
Line 2: Data point two with special character: @#$%.
Line 3: Data point three.
"""
cleaned_data = re.sub(r'[^a-zA-Z0-9\s]', '', data).strip()
single_line = re.sub(r'\s+', ' ', cleaned_data)
print(single_line)
八、总结
将多行数据合并为一行是一个常见的需求,特别是在数据处理和文本分析领域。使用join
方法是最简洁和高效的方式,但在特定场景下,其他方法如字符串连接、列表解析和正则表达式也可以派上用场。通过选择适合的方法,可以显著提高代码的可读性和执行效率。希望本文能为你提供有价值的参考,使你在实际应用中能更好地处理多行数据。
相关问答FAQs:
如何在Python中将多行数据合并为一行输出?
在Python中,可以使用字符串的join()
方法来将多行数据合并为一行输出。例如,如果有一个包含多行字符串的列表,可以通过'\n'.join(list_of_strings)
将其转换为一行字符串。这样做能够确保每行数据之间用换行符分隔,形成一个整齐的输出。
在Python中如何处理换行符以确保输出格式正确?
处理换行符时,使用strip()
方法可以去除多余的空白字符和换行符,确保输出格式整洁。此外,使用replace()
方法可以将换行符替换为其他字符,比如空格或逗号,以适应不同的输出需求。
如何将多行文本文件的内容读取并输出为一行?
要将多行文本文件的内容读取并输出为一行,可以使用以下步骤:首先,打开文件并读取所有行,然后使用join()
方法将其合并。在代码中,with open('filename.txt') as file:
可以确保文件在读取后被正确关闭。这样,能够有效地将文件内容转换为单行输出,便于后续处理或显示。
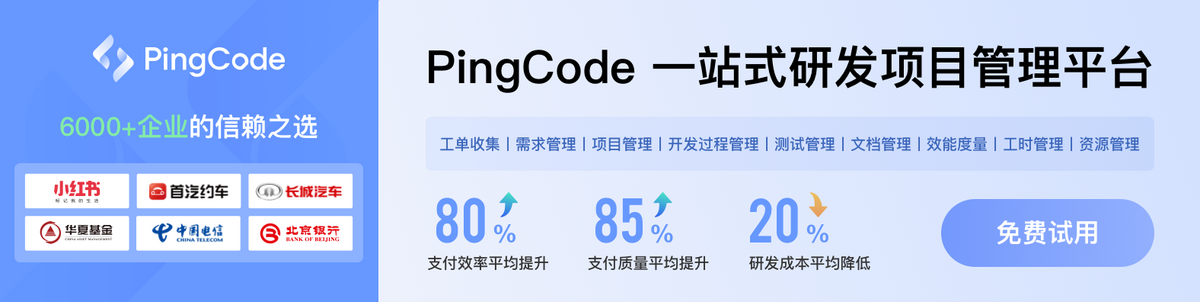