要在Python中画出内切正三角形,有几种常见的方法:使用Matplotlib进行绘图、利用Turtle图形库、或使用Pygame进行更复杂的图形绘制。
其中,Matplotlib库非常适合简单的绘图任务、Turtle库更适合教育性和互动性绘图、Pygame则更适合游戏开发和复杂图形绘制。下面将详细描述如何使用这三种方法来绘制一个内切正三角形。
一、使用Matplotlib绘制内切正三角形
1.1 安装和导入所需库
首先,确保已安装Matplotlib库。如果未安装,可以使用以下命令进行安装:
pip install matplotlib
然后,在Python脚本中导入所需的库:
import matplotlib.pyplot as plt
import numpy as np
1.2 计算正三角形的顶点坐标
为了画一个内切正三角形,需要先计算正三角形的顶点坐标。假设三角形的中心在原点(0,0),边长为a
。
def calculate_vertices(a):
height = np.sqrt(3) / 2 * a
vertices = [
(0, height / 3), # top vertex
(-a / 2, -height / 3), # bottom-left vertex
(a / 2, -height / 3) # bottom-right vertex
]
return vertices
1.3 绘制正三角形
def plot_triangle(vertices):
fig, ax = plt.subplots()
triangle = plt.Polygon(vertices, closed=True, edgecolor='b', fill=None)
ax.add_patch(triangle)
ax.set_aspect('equal', 'box')
ax.plot(0, 0, 'ro') # mark the center
plt.xlim(-1, 1)
plt.ylim(-1, 1)
plt.grid(True)
plt.show()
1.4 完整代码
import matplotlib.pyplot as plt
import numpy as np
def calculate_vertices(a):
height = np.sqrt(3) / 2 * a
vertices = [
(0, height / 3), # top vertex
(-a / 2, -height / 3), # bottom-left vertex
(a / 2, -height / 3) # bottom-right vertex
]
return vertices
def plot_triangle(vertices):
fig, ax = plt.subplots()
triangle = plt.Polygon(vertices, closed=True, edgecolor='b', fill=None)
ax.add_patch(triangle)
ax.set_aspect('equal', 'box')
ax.plot(0, 0, 'ro') # mark the center
plt.xlim(-1, 1)
plt.ylim(-1, 1)
plt.grid(True)
plt.show()
side_length = 1 # Example side length
vertices = calculate_vertices(side_length)
plot_triangle(vertices)
通过以上代码,可以使用Matplotlib绘制一个内切正三角形。
二、使用Turtle绘制内切正三角形
2.1 安装和导入所需库
Turtle库通常是Python标准库的一部分,无需额外安装。直接导入即可:
import turtle
import math
2.2 计算正三角形的顶点坐标
假设三角形的中心在原点(0,0),边长为a
。
def calculate_vertices(a):
height = math.sqrt(3) / 2 * a
vertices = [
(0, height / 3), # top vertex
(-a / 2, -height / 3), # bottom-left vertex
(a / 2, -height / 3) # bottom-right vertex
]
return vertices
2.3 绘制正三角形
def draw_triangle(vertices):
turtle.penup()
turtle.goto(vertices[0])
turtle.pendown()
for vertex in vertices[1:]:
turtle.goto(vertex)
turtle.goto(vertices[0])
turtle.done()
2.4 完整代码
import turtle
import math
def calculate_vertices(a):
height = math.sqrt(3) / 2 * a
vertices = [
(0, height / 3), # top vertex
(-a / 2, -height / 3), # bottom-left vertex
(a / 2, -height / 3) # bottom-right vertex
]
return vertices
def draw_triangle(vertices):
turtle.penup()
turtle.goto(vertices[0])
turtle.pendown()
for vertex in vertices[1:]:
turtle.goto(vertex)
turtle.goto(vertices[0])
turtle.done()
side_length = 100 # Example side length
vertices = calculate_vertices(side_length)
draw_triangle(vertices)
通过以上代码,可以使用Turtle绘制一个内切正三角形。
三、使用Pygame绘制内切正三角形
3.1 安装和导入所需库
首先,确保已安装Pygame库。如果未安装,可以使用以下命令进行安装:
pip install pygame
然后,在Python脚本中导入所需的库:
import pygame
import math
3.2 计算正三角形的顶点坐标
假设三角形的中心在原点(0,0),边长为a
。
def calculate_vertices(a):
height = math.sqrt(3) / 2 * a
vertices = [
(0, height / 3), # top vertex
(-a / 2, -height / 3), # bottom-left vertex
(a / 2, -height / 3) # bottom-right vertex
]
return vertices
3.3 绘制正三角形
def draw_triangle(screen, vertices):
pygame.draw.polygon(screen, (0, 0, 255), vertices)
3.4 完整代码
import pygame
import math
def calculate_vertices(a):
height = math.sqrt(3) / 2 * a
vertices = [
(0, height / 3), # top vertex
(-a / 2, -height / 3), # bottom-left vertex
(a / 2, -height / 3) # bottom-right vertex
]
return vertices
def draw_triangle(screen, vertices):
pygame.draw.polygon(screen, (0, 0, 255), vertices)
def main():
pygame.init()
screen = pygame.display.set_mode((400, 400))
pygame.display.set_caption('Inscribed Equilateral Triangle')
screen.fill((255, 255, 255))
side_length = 200 # Example side length
vertices = calculate_vertices(side_length)
# Shift vertices to the center of the screen
vertices = [(x + 200, y + 200) for x, y in vertices]
draw_triangle(screen, vertices)
pygame.display.flip()
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
pygame.quit()
if __name__ == '__main__':
main()
通过以上代码,可以使用Pygame绘制一个内切正三角形。
结论
在Python中,有多种方法可以绘制内切正三角形,包括Matplotlib、Turtle和Pygame。根据具体需求选择合适的工具,可以更高效地完成任务。无论是进行简单的绘图任务还是复杂的图形绘制,这些库都提供了丰富的功能和灵活性。希望这篇文章能帮助你更好地理解和应用这些工具来绘制内切正三角形。
相关问答FAQs:
如何使用Python绘制一个内切正三角形?
要绘制内切正三角形,首先需要了解其性质。内切正三角形的圆心是三角形的重心。可以使用matplotlib库,结合一些几何知识来完成绘制。首先计算出正三角形的三个顶点坐标,然后利用matplotlib绘图。
在绘制内切正三角形时需要考虑哪些参数?
在绘制内切正三角形时,主要需要考虑正三角形的边长和圆的半径。边长可以影响三角形的大小,而圆的半径则决定了内切圆的大小。确保在绘制时这些参数设置准确,以便得到理想的图形效果。
有哪些Python库可以帮助我绘制几何图形?
Python中有多个库可以帮助绘制几何图形,最常用的是matplotlib和turtle。matplotlib适合用于科学绘图和复杂的图形,而turtle则更适合初学者进行简单的图形绘制。选择合适的库可以提高绘图效率和效果。
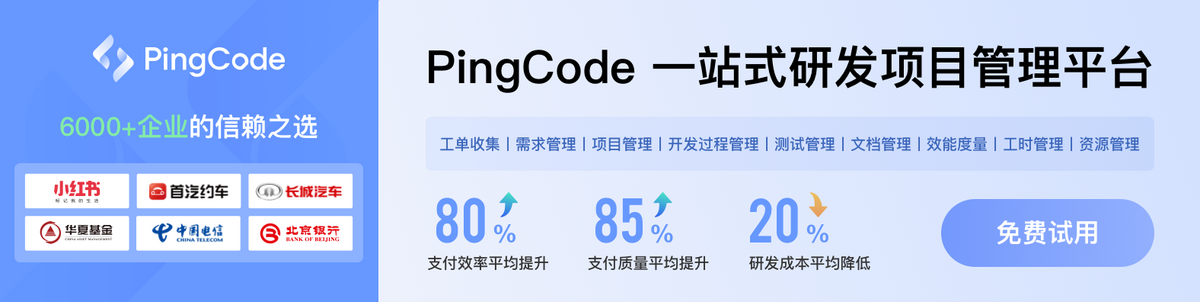