Python 删除数据中的非文本文件的方法包括:使用 os
模块遍历文件目录、检查文件扩展名、删除非文本文件。
在数据处理中,有时会遇到需要清理数据目录的情况,特别是删除非文本文件。以下是一种详细的方法,使用 Python 的 os
模块和文件扩展名检查来实现这一目标。
一、遍历文件目录
首先,我们需要遍历目标目录中的所有文件。Python 提供了 os
模块中的 os.listdir()
方法来获取目录中的文件和子目录列表。我们还可以使用 os.path.join()
来构建文件的完整路径。
import os
def list_files(directory):
files = os.listdir(directory)
for file in files:
full_path = os.path.join(directory, file)
print(full_path)
二、检查文件扩展名
接下来,我们需要检查每个文件的扩展名。文本文件通常具有特定的扩展名,如 .txt
、.md
、.csv
等。我们可以使用 os.path.splitext()
方法来分离文件名和扩展名。
def is_text_file(filename):
text_extensions = ['.txt', '.md', '.csv']
_, ext = os.path.splitext(filename)
return ext.lower() in text_extensions
三、删除非文本文件
最后,结合前面的两个步骤,我们可以删除所有非文本文件。我们将遍历目录中的所有文件,检查它们的扩展名,如果不是文本文件,就删除它们。
def delete_non_text_files(directory):
files = os.listdir(directory)
for file in files:
full_path = os.path.join(directory, file)
if not is_text_file(full_path):
os.remove(full_path)
print(f"Deleted: {full_path}")
示例调用
directory_path = '/path/to/your/directory'
delete_non_text_files(directory_path)
四、处理子目录
在实际应用中,文件目录可能包含子目录。为了确保删除所有非文本文件,我们需要递归地遍历所有子目录。我们可以使用 os.walk()
方法,它会生成目录树中的文件名。
def delete_non_text_files_recursive(directory):
for root, dirs, files in os.walk(directory):
for file in files:
full_path = os.path.join(root, file)
if not is_text_file(full_path):
os.remove(full_path)
print(f"Deleted: {full_path}")
示例调用
directory_path = '/path/to/your/directory'
delete_non_text_files_recursive(directory_path)
五、错误处理
在实际操作中,可能会遇到文件无法删除的情况,例如文件被占用或没有权限。因此,我们需要添加错误处理机制。
def delete_non_text_files_safe(directory):
for root, dirs, files in os.walk(directory):
for file in files:
full_path = os.path.join(root, file)
if not is_text_file(full_path):
try:
os.remove(full_path)
print(f"Deleted: {full_path}")
except Exception as e:
print(f"Error deleting {full_path}: {e}")
示例调用
directory_path = '/path/to/your/directory'
delete_non_text_files_safe(directory_path)
六、日志记录
为了更好地监控和调试,我们可以添加日志记录功能,将删除操作的结果记录到日志文件中。
import logging
logging.basicConfig(filename='file_cleanup.log', level=logging.INFO)
def delete_non_text_files_with_logging(directory):
for root, dirs, files in os.walk(directory):
for file in files:
full_path = os.path.join(root, file)
if not is_text_file(full_path):
try:
os.remove(full_path)
logging.info(f"Deleted: {full_path}")
except Exception as e:
logging.error(f"Error deleting {full_path}: {e}")
示例调用
directory_path = '/path/to/your/directory'
delete_non_text_files_with_logging(directory_path)
七、总结
通过上述步骤,我们可以使用 Python 轻松地删除数据目录中的非文本文件。遍历文件目录、检查文件扩展名、删除非文本文件 是实现这一目标的核心步骤。在实际应用中,我们还需要考虑子目录的处理、错误处理和日志记录等方面,以确保操作的可靠性和可维护性。
这种方法可以广泛应用于数据清理、文件管理和数据预处理等场景,为数据处理工作提供了便利和效率。
相关问答FAQs:
如何识别数据中的非文本文件?
识别非文本文件通常可以通过检查文件的扩展名或内容类型来实现。常见的文本文件扩展名包括 .txt
, .csv
, .json
等,而非文本文件可能是 .jpg
, .png
, .exe
等。可以使用 Python 的 os
模块结合文件扩展名检查,或者读取文件的二进制内容来判断其是否为文本。
在删除非文本文件之前,我应该备份我的数据吗?
是的,备份数据是一个非常重要的步骤。在进行删除操作之前,确保你拥有文件的备份,这样即使误删了重要文件,也可以轻松恢复。同时,备份也能帮助你避免数据丢失带来的风险,特别是在处理大量文件时。
使用 Python 删除非文本文件有什么安全措施?
在使用 Python 删除文件时,可以采取一些安全措施。首先,使用 os.path.isfile()
确保要删除的确实是文件,而不是目录。其次,可以在删除之前打印出将要删除的文件列表,以便确认。同时,实施一个交互式确认步骤,让用户在删除文件之前进行确认,进一步减少错误删除的风险。
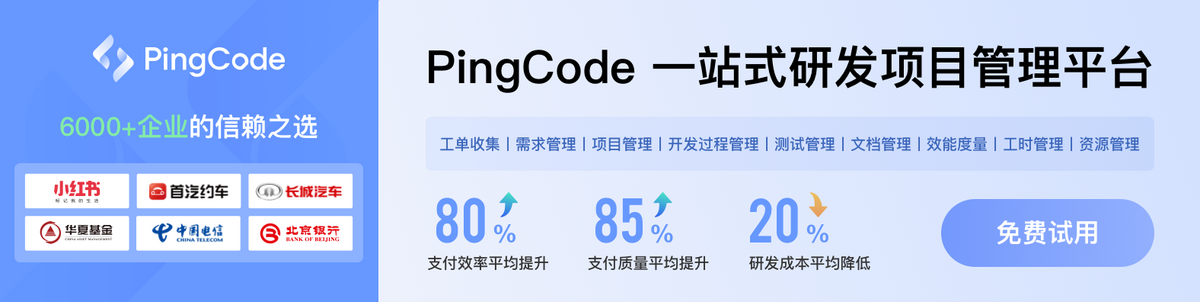