在Python中格式化带有括号的字符串,可以使用以下方法:f字符串、format方法、正则表达式。在这三种方法中,f字符串是最简洁和直观的,因为它直接在字符串中嵌入变量。
一、使用f字符串
f字符串,也称为格式化字符串字面量,是Python 3.6引入的一种新的字符串格式化方法。它允许你在字符串中嵌入表达式,并在运行时进行计算。
二、使用format方法
format方法是Python 2.7及以上版本提供的一种格式化字符串的方法。它通过在字符串中使用花括号{}来指定需要插入的变量位置,然后在调用format方法时传入这些变量。
三、使用正则表达式
正则表达式是一种用于匹配字符串的模式。它们可以用来查找、替换或提取字符串中的特定部分。在格式化带有括号的字符串时,正则表达式可以帮助我们找到括号及其内容,然后进行相应的替换或插入操作。
下面,我们将详细介绍这三种方法及其具体实现。
一、使用f字符串
f字符串是一种非常直观和简洁的字符串格式化方法。你只需要在字符串前加上字母f,然后在字符串中使用大括号{}包裹需要嵌入的变量或表达式即可。下面是一个简单的例子:
name = "Alice"
age = 30
formatted_string = f"Name: {name}, Age: {age}"
print(formatted_string)
上面的代码会输出:
Name: Alice, Age: 30
嵌入带有括号的字符串
如果你需要格式化带有括号的字符串,可以直接在f字符串中嵌入括号。下面是一个例子:
number = 42
formatted_string = f"The answer to life, the universe, and everything is ({number})"
print(formatted_string)
上面的代码会输出:
The answer to life, the universe, and everything is (42)
二、使用format方法
format方法是另一种常用的字符串格式化方法。它通过在字符串中使用花括号{}来指定变量的位置,然后在调用format方法时传入这些变量。下面是一个简单的例子:
name = "Alice"
age = 30
formatted_string = "Name: {}, Age: {}".format(name, age)
print(formatted_string)
上面的代码会输出:
Name: Alice, Age: 30
嵌入带有括号的字符串
如果你需要格式化带有括号的字符串,可以在花括号中直接嵌入括号。下面是一个例子:
number = 42
formatted_string = "The answer to life, the universe, and everything is ({})".format(number)
print(formatted_string)
上面的代码会输出:
The answer to life, the universe, and everything is (42)
三、使用正则表达式
正则表达式是一种功能强大的字符串处理工具。在格式化带有括号的字符串时,正则表达式可以帮助我们找到括号及其内容,然后进行相应的替换或插入操作。下面是一个简单的例子:
import re
pattern = r"\((.*?)\)"
replacement = r"[\1]"
string = "This is a test (string) with (brackets)."
formatted_string = re.sub(pattern, replacement, string)
print(formatted_string)
上面的代码会输出:
This is a test [string] with [brackets].
嵌入带有括号的字符串
如果你需要格式化带有括号的字符串,可以使用正则表达式找到括号及其内容,然后进行相应的替换。下面是一个例子:
import re
pattern = r"\((.*?)\)"
replacement = r"{\1}"
string = "This is a test (string) with (brackets)."
formatted_string = re.sub(pattern, replacement, string)
print(formatted_string)
上面的代码会输出:
This is a test {string} with {brackets}.
结合使用多种方法
在实际应用中,你可以结合使用多种方法来格式化带有括号的字符串。例如,你可以先使用正则表达式找到括号及其内容,然后使用f字符串或format方法进行进一步的格式化。下面是一个例子:
import re
pattern = r"\((.*?)\)"
string = "This is a test (string) with (brackets)."
使用正则表达式找到括号及其内容
matches = re.findall(pattern, string)
使用f字符串进行进一步的格式化
formatted_string = f"Found {len(matches)} matches: {', '.join(matches)}"
print(formatted_string)
上面的代码会输出:
Found 2 matches: string, brackets
总结
在Python中格式化带有括号的字符串,可以使用f字符串、format方法和正则表达式。f字符串是最简洁和直观的方法,适用于大多数场景。format方法提供了更灵活的格式化选项,适用于需要更复杂格式化需求的场景。正则表达式是一种功能强大的字符串处理工具,适用于需要查找、替换或提取字符串中特定部分的场景。在实际应用中,可以根据具体需求选择合适的方法,或者结合使用多种方法来实现更复杂的格式化操作。
相关问答FAQs:
如何在Python中格式化带有括号的字符串以便输出更美观?
在Python中,可以使用f-string或str.format()
方法来格式化带有括号的字符串。f-string允许你在字符串中直接插入变量,而str.format()
则使用占位符进行替换。示例代码如下:
name = "Alice"
age = 30
formatted_string = f"{name} (年龄: {age})"
print(formatted_string)
这将输出 Alice (年龄: 30)
,使得字符串看起来更加整齐。
在Python中处理包含括号的字符串时,如何避免语法错误?
当字符串中包含括号时,如果使用f-string或str.format()
,务必使用转义字符(\)来避免语法错误。例如,如果字符串本身包含大括号,可以使用双大括号来转义:
text = "这是一个包含{{括号}}的字符串"
print(text.format())
这样可以确保Python正确解析字符串而不引发错误。
是否有推荐的库用于格式化复杂的字符串,尤其是带有括号的情况?
可以考虑使用string.Template
模块来处理复杂字符串的格式化。它允许使用简单的占位符,并且能有效处理带有括号的字符串。示例代码如下:
from string import Template
template = Template("$name (年龄: $age)")
formatted_string = template.substitute(name="Bob", age=25)
print(formatted_string)
这种方法使得字符串的构建更加灵活,尤其是在需要多次替换时。
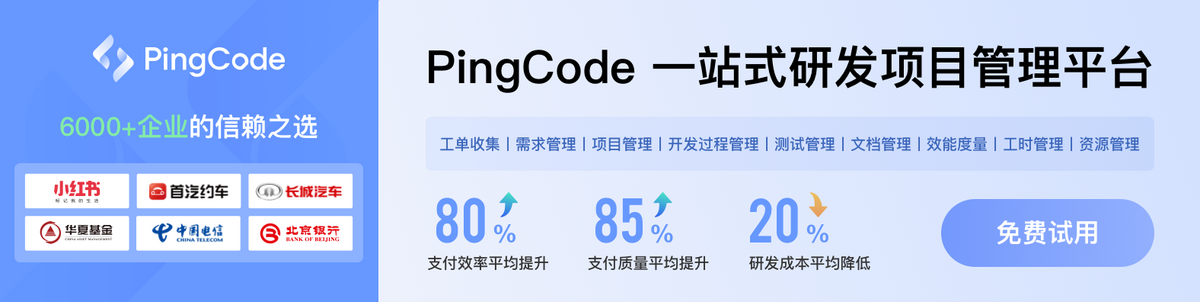