在Python中判断一个字符串的方法有很多,例如判断字符串是否为空、判断字符串是否包含特定的子字符串、判断字符串是否以某个特定的子字符串开头或结尾等。常用的方法包括:使用内置函数len()、in运算符、字符串方法startswith()和endswith()。
其中一个常用的方式是使用in
运算符来判断一个字符串是否包含特定的子字符串。这种方法简单直观,且代码可读性强。例如,if 'substring' in main_string:
可以直接判断main_string
中是否包含substring
。接下来,我们会详细介绍这些方法,并探讨其应用场景和最佳实践。
一、判断字符串是否为空
判断字符串是否为空是进行字符串处理的第一步。Python提供了一些简单的方法来实现这一点。
1、使用len()函数
使用len()
函数可以直接获取字符串的长度,如果长度为0,则该字符串为空。
def is_empty(string):
return len(string) == 0
示例
print(is_empty("")) # 输出: True
print(is_empty("abc")) # 输出: False
2、使用字符串的布尔值
在Python中,空字符串的布尔值为False
,非空字符串的布尔值为True
。
def is_empty(string):
return not string
示例
print(is_empty("")) # 输出: True
print(is_empty("abc")) # 输出: False
二、判断字符串是否包含特定子字符串
1、使用in运算符
in
运算符是最常见和直观的方法,用来检查一个子字符串是否存在于另一个字符串中。
def contains_substring(main_string, substring):
return substring in main_string
示例
print(contains_substring("hello world", "world")) # 输出: True
print(contains_substring("hello world", "python")) # 输出: False
2、使用find()方法
find()
方法返回子字符串在字符串中第一次出现的位置,如果找不到,返回-1。
def contains_substring(main_string, substring):
return main_string.find(substring) != -1
示例
print(contains_substring("hello world", "world")) # 输出: True
print(contains_substring("hello world", "python")) # 输出: False
三、判断字符串是否以特定子字符串开头或结尾
1、使用startswith()方法
startswith()
方法用于检查字符串是否以指定的子字符串开头。
def starts_with(main_string, prefix):
return main_string.startswith(prefix)
示例
print(starts_with("hello world", "hello")) # 输出: True
print(starts_with("hello world", "world")) # 输出: False
2、使用endswith()方法
endswith()
方法用于检查字符串是否以指定的子字符串结尾。
def ends_with(main_string, suffix):
return main_string.endswith(suffix)
示例
print(ends_with("hello world", "world")) # 输出: True
print(ends_with("hello world", "hello")) # 输出: False
四、判断字符串是否只包含特定字符类型
1、使用isalpha()方法
isalpha()
方法用于判断字符串是否只包含字母。
def is_alpha(string):
return string.isalpha()
示例
print(is_alpha("hello")) # 输出: True
print(is_alpha("hello123")) # 输出: False
2、使用isdigit()方法
isdigit()
方法用于判断字符串是否只包含数字。
def is_digit(string):
return string.isdigit()
示例
print(is_digit("12345")) # 输出: True
print(is_digit("12345abc")) # 输出: False
3、使用isalnum()方法
isalnum()
方法用于判断字符串是否只包含字母和数字。
def is_alnum(string):
return string.isalnum()
示例
print(is_alnum("hello123")) # 输出: True
print(is_alnum("hello 123")) # 输出: False
五、判断字符串是否符合特定格式
1、使用正则表达式
正则表达式是检查字符串是否符合特定格式的强大工具。Python的re
模块提供了相关支持。
import re
def matches_pattern(string, pattern):
return re.match(pattern, string) is not None
示例
pattern = r'^[a-zA-Z0-9_]+$' # 仅包含字母、数字和下划线
print(matches_pattern("hello123", pattern)) # 输出: True
print(matches_pattern("hello 123", pattern)) # 输出: False
六、判断字符串是否相等
1、使用==运算符
直接使用==
运算符可以判断两个字符串是否相等。
def is_equal(string1, string2):
return string1 == string2
示例
print(is_equal("hello", "hello")) # 输出: True
print(is_equal("hello", "world")) # 输出: False
2、使用casefold()方法进行忽略大小写比较
casefold()
方法可以将字符串转换为小写,从而实现忽略大小写的比较。
def is_equal_ignore_case(string1, string2):
return string1.casefold() == string2.casefold()
示例
print(is_equal_ignore_case("Hello", "hello")) # 输出: True
print(is_equal_ignore_case("Hello", "world")) # 输出: False
七、判断字符串长度是否在特定范围内
使用len()
函数可以获取字符串的长度,从而判断其是否在特定范围内。
def is_length_in_range(string, min_length, max_length):
return min_length <= len(string) <= max_length
示例
print(is_length_in_range("hello", 1, 10)) # 输出: True
print(is_length_in_range("hello", 6, 10)) # 输出: False
八、判断字符串是否包含特定字符集合
1、使用集合操作
将字符串和特定字符集合转换为集合,通过集合操作来判断是否包含特定字符集合。
def contains_char_set(string, char_set):
return set(char_set).issubset(set(string))
示例
print(contains_char_set("hello world", "he")) # 输出: True
print(contains_char_set("hello world", "xyz")) # 输出: False
结论
在Python中判断一个字符串的方法多种多样,可以根据具体需求选择合适的方法。常用的方法包括:使用内置函数len()
判断字符串是否为空、使用in
运算符判断字符串是否包含特定子字符串、使用字符串方法startswith()
和endswith()
判断字符串是否以特定子字符串开头或结尾等。选择合适的方法不仅能提高代码的可读性,还能提升代码的执行效率。
相关问答FAQs:
如何在Python中判断一个字符串是否为空?
在Python中,可以使用简单的条件判断来检查字符串是否为空。可以使用if not my_string:
来判断字符串my_string
是否为空。如果字符串为空,条件为真;如果字符串非空,条件为假。还可以使用len(my_string) == 0
来判断字符串长度是否为零,这也能有效判断字符串是否为空。
Python中有哪些方法可以检查字符串的内容?
Python提供了多种方法来检查字符串的内容。例如,可以使用str.isalpha()
方法判断字符串是否只包含字母,使用str.isdigit()
判断是否只包含数字,使用str.isspace()
判断是否只包含空白字符。通过这些方法,用户可以很方便地验证字符串的具体类型和内容。
如何在Python中判断一个字符串是否包含特定的子字符串?
要判断一个字符串是否包含特定的子字符串,可以使用in
运算符。例如,if "keyword" in my_string:
可以检查my_string
中是否包含"keyword"
。这种方法非常直观,易于理解,并且适合各种场景,比如搜索、过滤等操作。
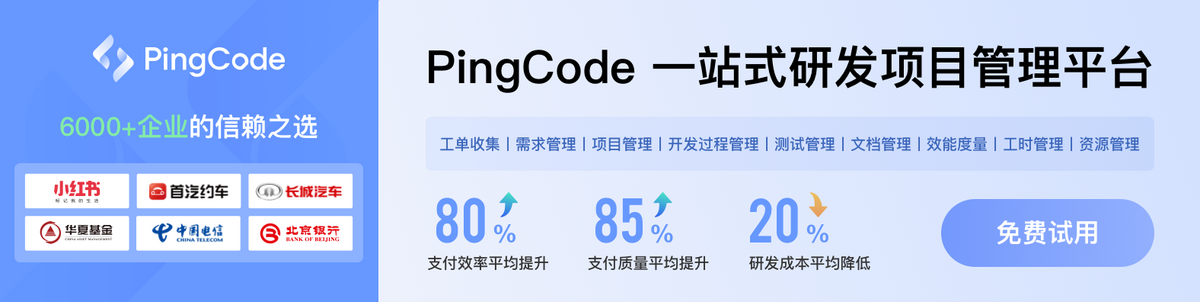