在Python中,可以通过使用库如matplotlib
和numpy
,将一个图像绕某一点进行旋转。 具体的方法包括计算旋转矩阵、应用矩阵变换、以及调整图像的显示位置。旋转矩阵的核心是三角函数,可以通过数学公式来计算图像中每个点的新位置。
一、引入必要的库
在开始处理图像之前,我们需要引入一些必要的Python库。matplotlib
用于图像处理和显示,numpy
用于数学计算和数组操作。
import matplotlib.pyplot as plt
import numpy as np
from skimage import io
二、读取和显示图像
首先,我们需要读取并显示原始图像。你可以使用io.imread
从文件中读取图像,并使用plt.imshow
来显示它。
image = io.imread('path_to_image.jpg')
plt.imshow(image)
plt.title('Original Image')
plt.show()
三、定义旋转矩阵
旋转矩阵是图像变换的核心。它依赖于旋转角度,并通过三角函数cos
和sin
计算。
def get_rotation_matrix(angle):
angle_rad = np.deg2rad(angle)
return np.array([
[np.cos(angle_rad), -np.sin(angle_rad)],
[np.sin(angle_rad), np.cos(angle_rad)]
])
四、计算新坐标
为了将图像绕某一点旋转,我们需要计算每个像素的新坐标。这里,我们定义一个函数来计算给定点的新位置。
def rotate_point(x, y, cx, cy, angle):
rotation_matrix = get_rotation_matrix(angle)
# 移动点,使其相对于中心点
translated_point = np.array([x - cx, y - cy])
# 旋转
rotated_point = rotation_matrix.dot(translated_point)
# 移回原来的位置
return rotated_point + np.array([cx, cy])
五、应用旋转
接下来,我们需要将旋转应用到整个图像上。我们将新坐标映射到图像的像素上。
def rotate_image(image, angle, center):
h, w = image.shape[:2]
rotated_image = np.zeros_like(image)
for i in range(h):
for j in range(w):
new_coords = rotate_point(j, i, center[0], center[1], angle)
new_x, new_y = int(new_coords[0]), int(new_coords[1])
if 0 <= new_x < w and 0 <= new_y < h:
rotated_image[new_y, new_x] = image[i, j]
return rotated_image
六、显示旋转后的图像
最后,我们将旋转后的图像显示出来,以检查旋转效果。
center = (image.shape[1] // 2, image.shape[0] // 2) # 图像中心
angle = 45 # 旋转角度
rotated_image = rotate_image(image, angle, center)
plt.imshow(rotated_image)
plt.title('Rotated Image')
plt.show()
七、优化和扩展
上述方法存在一些限制,比如旋转后图像的边缘可能会有空白区域,或者图像的某些部分可能会被剪裁。为了解决这些问题,可以考虑以下几种优化和扩展方法:
1. 图像填充: 通过在图像边缘添加填充来避免旋转后图像部分被剪裁。
def pad_image(image, padding):
return np.pad(image, ((padding, padding), (padding, padding), (0, 0)), mode='constant')
2. 插值: 通过插值方法来平滑旋转后的图像,使得旋转后的像素位置不必严格对齐原图像的整数坐标。
from scipy.ndimage import affine_transform
def rotate_image_interpolation(image, angle, center):
transform_matrix = np.linalg.inv(get_rotation_matrix(angle))
offset = np.dot(transform_matrix, np.array(center)) - np.array(center)
return affine_transform(image, transform_matrix, offset=offset, mode='constant')
八、实例应用
为了更好地理解和应用上述方法,我们提供一个具体的例子,展示如何将一个图像绕其中心点旋转45度,并通过插值方法来平滑旋转后的图像。
image = io.imread('path_to_image.jpg')
center = (image.shape[1] // 2, image.shape[0] // 2)
angle = 45
rotated_image = rotate_image_interpolation(image, angle, center)
plt.imshow(rotated_image)
plt.title('Interpolated Rotated Image')
plt.show()
九、结论
在Python中,使用matplotlib
和numpy
可以方便地实现图像的旋转。关键在于理解旋转矩阵的原理,以及如何将其应用到图像的每个像素点上。通过添加图像填充和插值方法,可以进一步优化旋转效果,避免图像边缘的空白区域和剪裁问题。希望通过这篇详尽的教程,能够帮助你更好地理解和实现图像旋转。
相关问答FAQs:
如何在Python中实现图像的旋转效果?
在Python中,使用图像处理库如PIL(Pillow)可以轻松实现图像的旋转。您可以通过Image.rotate()
方法来旋转图像,指定旋转角度和旋转中心。如果希望围绕特定点旋转图像,则需结合图像的裁剪和变换操作。
使用OpenCV库旋转图像有什么技巧?
OpenCV提供了强大的图像处理功能。使用cv2.getRotationMatrix2D()
可以获取旋转矩阵,接着使用cv2.warpAffine()
将图像应用于该矩阵,完成旋转。确保在传递旋转中心时,您已正确计算图像的宽高,以便定位旋转的准确位置。
在图形界面中如何实现图像旋转功能?
可以使用Tkinter或Pygame等库来构建图形用户界面(GUI),实现图像旋转功能。在界面中,您可以添加按钮或滑块,让用户选择旋转角度,并通过相应的事件处理程序调用图像旋转的函数,实时更新显示的图像。
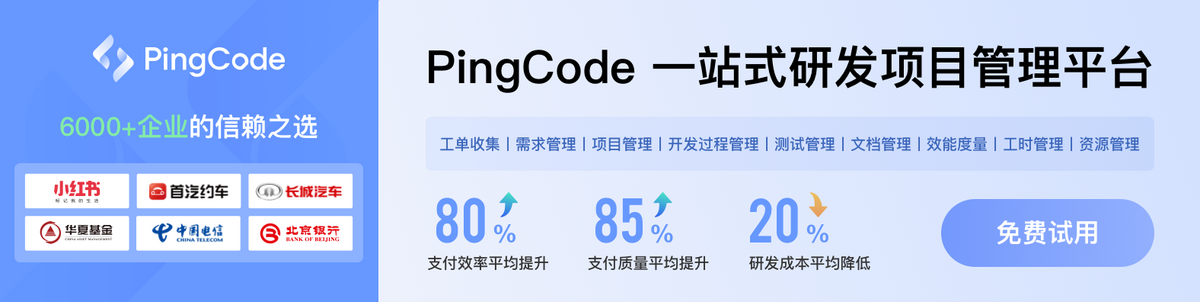