提高爬虫速度的主要方法有:使用多线程、多进程、异步编程、使用更高效的HTTP库、优化爬虫逻辑、使用代理IP等。其中,使用异步编程是一个非常有效的方法,可以显著提高爬虫的速度。异步编程可以使程序在等待网络请求时不阻塞,从而可以同时处理多个请求,大大提高了程序的效率。使用Python的异步库如aiohttp和asyncio,可以实现高效的异步网络请求。
一、多线程和多进程
1、多线程
多线程是指在一个进程中同时运行多个线程,每个线程执行不同的任务。Python的threading
模块可以很方便地实现多线程。多线程的优势在于可以同时处理多个请求,从而提高爬虫的速度。
import threading
import requests
def fetch_url(url):
response = requests.get(url)
print(response.status_code)
urls = ['http://example.com', 'http://example.org', 'http://example.net']
threads = []
for url in urls:
t = threading.Thread(target=fetch_url, args=(url,))
t.start()
threads.append(t)
for t in threads:
t.join()
2、多进程
多进程是指在操作系统中同时运行多个进程,每个进程独立执行不同的任务。Python的multiprocessing
模块可以很方便地实现多进程。多进程的优势在于可以利用多核CPU的优势,提高程序的并行处理能力。
import multiprocessing
import requests
def fetch_url(url):
response = requests.get(url)
print(response.status_code)
urls = ['http://example.com', 'http://example.org', 'http://example.net']
processes = []
for url in urls:
p = multiprocessing.Process(target=fetch_url, args=(url,))
p.start()
processes.append(p)
for p in processes:
p.join()
二、异步编程
异步编程是指在等待网络请求时不阻塞程序的执行,从而可以同时处理多个请求。Python的aiohttp
和asyncio
库可以实现高效的异步网络请求。
1、使用aiohttp和asyncio
aiohttp
是一个异步HTTP客户端库,可以与asyncio
一起使用,实现异步网络请求。
import aiohttp
import asyncio
async def fetch_url(session, url):
async with session.get(url) as response:
print(response.status)
async def main():
urls = ['http://example.com', 'http://example.org', 'http://example.net']
async with aiohttp.ClientSession() as session:
tasks = [fetch_url(session, url) for url in urls]
await asyncio.gather(*tasks)
asyncio.run(main())
三、使用更高效的HTTP库
一些HTTP库如httpx
,requests
等提供了更高效的网络请求功能,可以显著提高爬虫的速度。
1、使用httpx
httpx
是一个高效的HTTP客户端库,支持同步和异步请求。
import httpx
import asyncio
async def fetch_url(client, url):
response = await client.get(url)
print(response.status_code)
async def main():
urls = ['http://example.com', 'http://example.org', 'http://example.net']
async with httpx.AsyncClient() as client:
tasks = [fetch_url(client, url) for url in urls]
await asyncio.gather(*tasks)
asyncio.run(main())
四、优化爬虫逻辑
优化爬虫逻辑可以显著提高爬虫的效率。以下是一些优化爬虫逻辑的方法:
1、减少不必要的请求
在编写爬虫时,应尽量减少不必要的请求。例如,可以通过缓存已经爬取的数据,避免重复请求。
import requests
import hashlib
cache = {}
def fetch_url(url):
url_hash = hashlib.md5(url.encode()).hexdigest()
if url_hash in cache:
print("Using cached response")
return cache[url_hash]
response = requests.get(url)
cache[url_hash] = response
return response
urls = ['http://example.com', 'http://example.org', 'http://example.net']
for url in urls:
fetch_url(url)
2、使用更高效的数据解析方法
在解析爬取的数据时,应选择更高效的数据解析方法。例如,使用lxml
库解析HTML数据,比使用BeautifulSoup
库更高效。
from lxml import html
import requests
def fetch_and_parse(url):
response = requests.get(url)
tree = html.fromstring(response.content)
return tree
urls = ['http://example.com', 'http://example.org', 'http://example.net']
for url in urls:
tree = fetch_and_parse(url)
print(tree)
五、使用代理IP
使用代理IP可以避免被目标网站封禁,同时可以提高爬虫的速度。可以使用一些免费的代理IP服务,也可以购买付费的代理IP服务。
1、使用requests库和代理IP
可以使用requests
库的proxies
参数来设置代理IP。
import requests
proxies = {
'http': 'http://10.10.1.10:3128',
'https': 'http://10.10.1.10:1080',
}
response = requests.get('http://example.com', proxies=proxies)
print(response.status_code)
2、使用aiohttp库和代理IP
可以使用aiohttp
库的proxy
参数来设置代理IP。
import aiohttp
import asyncio
async def fetch_url(session, url):
async with session.get(url, proxy='http://10.10.1.10:3128') as response:
print(response.status)
async def main():
urls = ['http://example.com', 'http://example.org', 'http://example.net']
async with aiohttp.ClientSession() as session:
tasks = [fetch_url(session, url) for url in urls]
await asyncio.gather(*tasks)
asyncio.run(main())
六、使用分布式爬虫框架
使用分布式爬虫框架可以显著提高爬虫的速度。分布式爬虫框架可以将爬虫任务分发到多个节点上进行并行处理,从而提高爬虫的效率。常用的分布式爬虫框架有Scrapy、PySpider等。
1、使用Scrapy
Scrapy是一个强大的爬虫框架,支持分布式爬虫。可以通过配置Scrapy的DOWNLOAD_DELAY
和CONCURRENT_REQUESTS
参数来提高爬虫的速度。
# settings.py
DOWNLOAD_DELAY = 0.25
CONCURRENT_REQUESTS = 32
import scrapy
class MySpider(scrapy.Spider):
name = 'myspider'
start_urls = ['http://example.com', 'http://example.org', 'http://example.net']
def parse(self, response):
self.log(response.status)
2、使用PySpider
PySpider是一个功能强大的分布式爬虫框架,支持多种调度器和存储后端。
from pyspider.libs.base_handler import *
class Handler(BaseHandler):
crawl_config = {
}
@every(minutes=24 * 60)
def on_start(self):
self.crawl('http://example.com', callback=self.index_page)
@config(age=10 * 24 * 60 * 60)
def index_page(self, response):
for each in response.doc('a[href^="http"]').items():
self.crawl(each.attr.href, callback=self.detail_page)
@config(priority=2)
def detail_page(self, response):
return {
"url": response.url,
"title": response.doc('title').text(),
}
七、使用缓存
使用缓存可以避免重复请求,提高爬虫的速度。可以使用一些缓存库如requests-cache
来缓存请求结果。
1、使用requests-cache
requests-cache
是一个用于requests
库的缓存扩展,可以缓存HTTP请求的结果。
import requests_cache
requests_cache.install_cache('demo_cache')
import requests
response = requests.get('http://example.com')
print(response.status_code)
八、使用消息队列
使用消息队列可以实现任务的异步处理,提高爬虫的速度。可以使用一些消息队列如RabbitMQ、Kafka等来实现任务的异步处理。
1、使用RabbitMQ
RabbitMQ是一个开源的消息队列系统,可以实现任务的异步处理。
import pika
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
channel.queue_declare(queue='hello')
channel.basic_publish(exchange='', routing_key='hello', body='Hello World!')
print(" [x] Sent 'Hello World!'")
connection.close()
2、使用Kafka
Kafka是一个高吞吐量的分布式消息队列系统,可以实现任务的异步处理。
from kafka import KafkaProducer
producer = KafkaProducer(bootstrap_servers='localhost:9092')
producer.send('my-topic', b'Hello, World!')
producer.close()
九、使用分布式存储
使用分布式存储可以提高爬虫的数据存储效率。可以使用一些分布式存储系统如HDFS、Cassandra等来存储爬取的数据。
1、使用HDFS
HDFS是一个分布式文件系统,可以存储大规模的数据。
from hdfs import InsecureClient
client = InsecureClient('http://localhost:50070', user='user')
client.write('/user/data.txt', b'Hello, World!')
2、使用Cassandra
Cassandra是一个分布式数据库系统,可以存储大规模的数据。
from cassandra.cluster import Cluster
cluster = Cluster(['127.0.0.1'])
session = cluster.connect()
session.execute("""
CREATE KEYSPACE IF NOT EXISTS mykeyspace
WITH replication = { 'class': 'SimpleStrategy', 'replication_factor': '1' }
""")
session.execute("""
CREATE TABLE IF NOT EXISTS mykeyspace.mytable (
id int PRIMARY KEY,
value text
)
""")
session.execute("""
INSERT INTO mykeyspace.mytable (id, value)
VALUES (1, 'Hello, World!')
""")
十、使用数据压缩
使用数据压缩可以减少数据传输的时间,提高爬虫的速度。可以使用一些压缩库如gzip、brotli等来压缩数据。
1、使用gzip
gzip是一个常用的数据压缩格式,可以减少数据传输的时间。
import requests
response = requests.get('http://example.com', headers={'Accept-Encoding': 'gzip'})
print(response.content)
2、使用brotli
brotli是一个高效的数据压缩格式,可以减少数据传输的时间。
import requests
response = requests.get('http://example.com', headers={'Accept-Encoding': 'br'})
print(response.content)
十一、使用数据分片
使用数据分片可以将大规模的数据分成多个小块,提高爬虫的速度。可以使用一些数据分片库如sharding、partition等来实现数据分片。
1、使用sharding
sharding是一个将大规模数据分成多个小块的技术,可以提高数据处理的效率。
# 使用sharding库实现数据分片
from sharding import Sharding
shard = Sharding()
shard.add_data('http://example.com')
shard.add_data('http://example.org')
shard.add_data('http://example.net')
for data in shard.get_shards():
print(data)
2、使用partition
partition是一个将大规模数据分成多个小块的技术,可以提高数据处理的效率。
# 使用partition库实现数据分片
from partition import Partition
part = Partition()
part.add_data('http://example.com')
part.add_data('http://example.org')
part.add_data('http://example.net')
for data in part.get_partitions():
print(data)
十二、使用CDN加速
使用CDN加速可以减少数据传输的时间,提高爬虫的速度。可以使用一些CDN服务如Cloudflare、Akamai等来加速数据传输。
1、使用Cloudflare
Cloudflare是一个常用的CDN服务,可以加速数据传输。
# 使用Cloudflare加速
import requests
response = requests.get('http://example.com', headers={'CF-Connecting-IP': '1.2.3.4'})
print(response.status_code)
2、使用Akamai
Akamai是一个高效的CDN服务,可以加速数据传输。
# 使用Akamai加速
import requests
response = requests.get('http://example.com', headers={'X-Akamai-Edge-IP': '1.2.3.4'})
print(response.status_code)
十三、优化网络设置
优化网络设置可以减少数据传输的时间,提高爬虫的速度。可以通过调整网络设置如MTU、TCP窗口大小等来优化网络性能。
1、调整MTU
MTU(Maximum Transmission Unit)是指网络传输的最大数据包大小,调整MTU可以提高网络传输效率。
# 调整MTU
import socket
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.setsockopt(socket.IPPROTO_TCP, socket.TCP_MAXSEG, 1500)
2、调整TCP窗口大小
TCP窗口大小是指网络传输的缓冲区大小,调整TCP窗口大小可以提高网络传输效率。
# 调整TCP窗口大小
import socket
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.setsockopt(socket.IPPROTO_TCP, socket.TCP_WINDOW_CLAMP, 65536)
十四、使用高效的数据结构
使用高效的数据结构可以提高数据处理的效率,从而提高爬虫的速度。可以使用一些高效的数据结构如字典、集合等来处理数据。
1、使用字典
字典是一种高效的数据结构,可以快速查找和存储数据。
# 使用字典
data = {'url1': 'http://example.com', 'url2': 'http://example.org', 'url3': 'http://example.net'}
for key, value in data.items():
print(key, value)
2、使用集合
集合是一种高效的数据结构,可以快速查找和存储数据。
# 使用集合
data = {'http://example.com', 'http://example.org', 'http://example.net'}
for value in data:
print(value)
十五、使用高效的算法
使用高效的算法可以提高数据处理的效率,从而提高爬虫的速度。可以使用一些高效的算法如哈希算法、排序算法等来处理数据。
1、使用哈希算法
哈希算法是一种高效的数据查找算法,可以快速查找数据。
# 使用哈希算法
data = {'url1': 'http://example.com', 'url2': 'http://example.org', 'url3': 'http://example.net'}
def hash_func(key):
return hash(key) % len(data)
key = 'url1'
value = data.get(key)
print(value)
2、使用排序算法
排序算法是一种高效的数据处理算法,可以快速排序数据。
# 使用排序算法
data = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
sorted_data = sorted(data)
print(sorted_data)
十六、使用高效的数据传输协议
使用高效的数据传输协议可以提高数据传输的效率,从而提高爬虫的速度。可以使用一些高效的数据传输协议如HTTP/2、gRPC等来传输数据。
1、使用HTTP/2
HTTP/2是一种高效的数据传输协议,可以提高数据传输的效率。
import requests
response = requests.get('http://example.com', headers={'Upgrade': 'h2c'})
print(response.status_code)
2、使用gRPC
gRPC是一种高效的数据传输协议,可以提高数据传输的效率。
import grpc
channel = grpc.insecure_channel('localhost:50051')
stub = helloworld_pb2_grpc.GreeterStub(channel)
response = stub.SayHello(helloworld_pb2.HelloRequest(name='world'))
print(response.message)
十七、使用高效的数据存储格式
使用高效的数据存储格式可以提高数据存储和读取的效率,从而提高爬虫
相关问答FAQs:
如何优化Python爬虫的速度?
为了提升Python爬虫的速度,可以采用多线程或异步编程的方法。使用threading
或asyncio
库可以帮助您同时处理多个请求,从而减少总的爬取时间。此外,选择合适的请求库,如requests
和aiohttp
,也能提高请求的效率。避免过多的请求重定向和保持请求的简洁性也是关键。
在提高爬虫速度的同时,如何确保数据的准确性?
在追求速度的同时,确保数据的准确性同样重要。可以通过设置合适的请求间隔,避免因请求过快而导致目标网站的反爬虫机制触发。使用数据校验机制,如对比抓取的数据与源数据,能够有效提高数据的可靠性。同时,使用异常处理来捕获可能出现的错误,确保每次请求后都能正确处理和记录结果。
是否有推荐的库或工具来提升爬虫性能?
有许多库和工具可以帮助提升Python爬虫的性能。例如,Scrapy
是一个强大的爬虫框架,提供了内置的异步处理功能,适合大规模爬取任务。Beautiful Soup
和lxml
等库可以用来高效解析HTML,提高数据提取的速度。此外,使用Redis
等缓存工具,可以减少对同一数据的重复请求,提高整体效率。
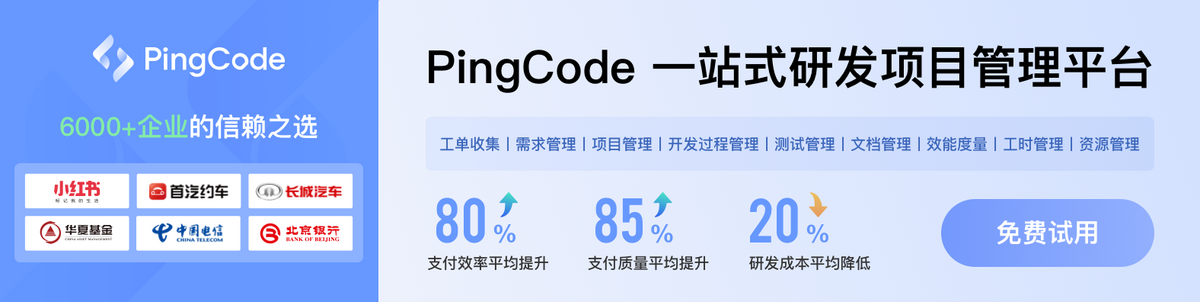