Aruco markers are widely used in computer vision applications to detect and estimate the pose of objects. In Python, you can use the OpenCV library to work with Aruco markers. To use Aruco markers in Python, you need to install OpenCV, create or detect Aruco markers, and then estimate their poses. Here, I will elaborate on how to perform these tasks step-by-step.
一、安装OpenCV
要在Python中使用Aruco标记,首先需要安装OpenCV库。你可以使用pip命令进行安装:
pip install opencv-python
pip install opencv-contrib-python
二、生成Aruco标记
Aruco标记是一种用于计算机视觉的二进制方块标记,通常用于相机标定、机器人定位等。首先,生成一些Aruco标记,以便在你的应用程序中使用。
import cv2
import numpy as np
Load the dictionary that was used to generate the markers.
aruco_dict = cv2.aruco.Dictionary_get(cv2.aruco.DICT_6X6_250)
Generate a marker with ID 0, and size 700x700 pixels.
marker_image = np.zeros((700, 700), dtype=np.uint8)
marker_image = cv2.aruco.drawMarker(aruco_dict, 0, 700, marker_image, 1)
Save the marker image to a file.
cv2.imwrite("aruco_marker.png", marker_image)
三、检测Aruco标记
生成了标记之后,你可以使用OpenCV来检测这些标记。在检测过程中,你需要提供一张包含Aruco标记的图像,OpenCV会返回检测到的标记及其位置信息。
import cv2
Load the dictionary that was used to generate the markers.
aruco_dict = cv2.aruco.Dictionary_get(cv2.aruco.DICT_6X6_250)
Initialize the detector parameters using default values.
parameters = cv2.aruco.DetectorParameters_create()
Load an image that contains the Aruco markers.
image = cv2.imread("image_with_markers.jpg")
Detect the markers in the image.
corners, ids, rejected_img_points = cv2.aruco.detectMarkers(image, aruco_dict, parameters=parameters)
Draw the detected markers on the image.
if ids is not None:
cv2.aruco.drawDetectedMarkers(image, corners, ids)
Display the image with the detected markers.
cv2.imshow("Aruco Markers", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
四、估计姿态
一旦检测到Aruco标记,你可以估计它们的姿态(位置和方向)。你需要相机的内参(相机矩阵和畸变系数)来进行姿态估计。
import cv2
import numpy as np
Load the dictionary that was used to generate the markers.
aruco_dict = cv2.aruco.Dictionary_get(cv2.aruco.DICT_6X6_250)
Initialize the detector parameters using default values.
parameters = cv2.aruco.DetectorParameters_create()
Load an image that contains the Aruco markers.
image = cv2.imread("image_with_markers.jpg")
Detect the markers in the image.
corners, ids, rejected_img_points = cv2.aruco.detectMarkers(image, aruco_dict, parameters=parameters)
If markers are detected
if ids is not None:
# Camera parameters (usually obtained from camera calibration)
camera_matrix = np.array([[1000, 0, 320],
[0, 1000, 240],
[0, 0, 1]], dtype=np.float64)
dist_coeffs = np.zeros((5, 1)) # Assuming no lens distortion
# Estimate pose of each marker and return the rotation and translation vectors
rvecs, tvecs, _ = cv2.aruco.estimatePoseSingleMarkers(corners, 0.05, camera_matrix, dist_coeffs)
for rvec, tvec in zip(rvecs, tvecs):
# Draw axis for each marker
cv2.aruco.drawAxis(image, camera_matrix, dist_coeffs, rvec, tvec, 0.1)
# Display the image with the detected markers and their axes
cv2.imshow("Aruco Markers with Axes", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
五、相机标定
为了提高姿态估计的准确性,你需要对相机进行标定。标定过程通常需要一组标定板图像(如棋盘格图像),通过这些图像计算相机的内参和畸变系数。
import cv2
import numpy as np
import glob
Termination criteria for corner sub-pix refinement
criteria = (cv2.TERM_CRITERIA_EPS + cv2.TERM_CRITERIA_MAX_ITER, 30, 0.001)
Prepare object points (0,0,0), (1,0,0), (2,0,0), ..., (7,5,0)
objp = np.zeros((6*7, 3), np.float32)
objp[:, :2] = np.mgrid[0:7, 0:6].T.reshape(-1, 2)
Arrays to store object points and image points from all the images
objpoints = [] # 3d point in real world space
imgpoints = [] # 2d points in image plane
Load calibration images
images = glob.glob('calibration_images/*.jpg')
for image in images:
img = cv2.imread(image)
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# Find the chessboard corners
ret, corners = cv2.findChessboardCorners(gray, (7, 6), None)
# If found, add object points, image points (after refining them)
if ret:
objpoints.append(objp)
corners2 = cv2.cornerSubPix(gray, corners, (11, 11), (-1, -1), criteria)
imgpoints.append(corners2)
# Draw and display the corners
cv2.drawChessboardCorners(img, (7, 6), corners2, ret)
cv2.imshow('img', img)
cv2.waitKey(500)
cv2.destroyAllWindows()
Camera calibration
ret, camera_matrix, dist_coeffs, rvecs, tvecs = cv2.calibrateCamera(objpoints, imgpoints, gray.shape[::-1], None, None)
Save the camera parameters for later use
np.savez('camera_params.npz', camera_matrix=camera_matrix, dist_coeffs=dist_coeffs)
六、加载相机参数
加载保存的相机参数,以便在姿态估计中使用。
import numpy as np
Load camera parameters
with np.load('camera_params.npz') as data:
camera_matrix = data['camera_matrix']
dist_coeffs = data['dist_coeffs']
七、实时检测与跟踪
使用相机实时检测和跟踪Aruco标记。
import cv2
import numpy as np
Load the dictionary that was used to generate the markers.
aruco_dict = cv2.aruco.Dictionary_get(cv2.aruco.DICT_6X6_250)
Initialize the detector parameters using default values.
parameters = cv2.aruco.DetectorParameters_create()
Load camera parameters
with np.load('camera_params.npz') as data:
camera_matrix = data['camera_matrix']
dist_coeffs = data['dist_coeffs']
Open a video capture
cap = cv2.VideoCapture(0)
while cap.isOpened():
ret, frame = cap.read()
if not ret:
break
# Detect markers in the frame
corners, ids, rejected_img_points = cv2.aruco.detectMarkers(frame, aruco_dict, parameters=parameters)
if ids is not None:
# Estimate pose of each marker and return the rotation and translation vectors
rvecs, tvecs, _ = cv2.aruco.estimatePoseSingleMarkers(corners, 0.05, camera_matrix, dist_coeffs)
for rvec, tvec in zip(rvecs, tvecs):
# Draw axis for each marker
cv2.aruco.drawAxis(frame, camera_matrix, dist_coeffs, rvec, tvec, 0.1)
# Draw detected markers
cv2.aruco.drawDetectedMarkers(frame, corners, ids)
# Display the frame
cv2.imshow('Aruco Markers', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
八、总结
使用Aruco标记进行姿态估计是计算机视觉中的一个重要任务。通过上述步骤,你可以生成、检测Aruco标记,并估计其姿态。以下几点是使用Aruco标记的核心步骤:
- 安装OpenCV库:通过pip命令安装所需的OpenCV库。
- 生成Aruco标记:使用OpenCV生成Aruco标记图像。
- 检测Aruco标记:在图像中检测Aruco标记,并绘制其边界。
- 估计姿态:通过相机内参估计标记的旋转和位移向量。
- 相机标定:使用标定板图像计算相机的内参和畸变系数。
- 实时检测与跟踪:使用相机实时检测和跟踪Aruco标记。
通过这些步骤,你可以在Python中有效地使用Aruco标记进行各种计算机视觉任务。
相关问答FAQs:
什么是Aruco标记,为什么我需要使用它?
Aruco标记是一种用于计算机视觉的图案,可以被摄像头识别并用于定位和跟踪对象。它们在机器人技术、增强现实和视觉测量等领域中应用广泛。使用Aruco标记可以帮助开发者实现精确的空间定位和对象识别,提升项目的交互性和智能化水平。
我该如何在Python中安装和配置Aruco库?
要在Python中使用Aruco,通常需要安装OpenCV库,因为Aruco是OpenCV的一部分。您可以通过使用pip命令安装OpenCV:pip install opencv-python
。安装完成后,您还需要确保安装了OpenCV的额外模块,使用命令pip install opencv-contrib-python
。完成这些步骤后,您就可以在Python代码中导入Aruco模块并开始使用。
如何在Python中生成和识别Aruco标记?
生成Aruco标记可以通过OpenCV的cv2.aruco
模块实现。您可以使用cv2.aruco.drawMarker()
函数来创建自定义大小的标记。识别Aruco标记需要使用cv2.aruco.detectMarkers()
函数来捕捉摄像头图像中的标记,并返回其ID和位置。确保在使用时正确设置相机参数,以提高识别的准确性和效率。
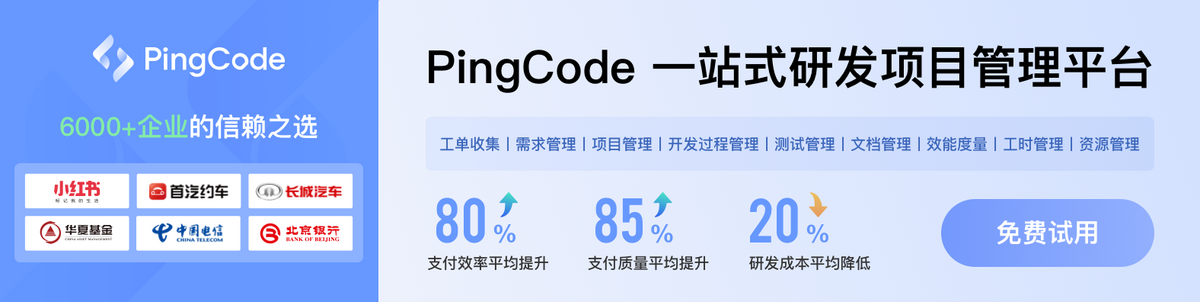