使用Python实现翻页的核心方法有:利用循环和分页算法、利用库如BeautifulSoup和Requests进行网页爬取、利用库如Selenium进行自动化操作。 其中,利用循环和分页算法是最基础的方法,它通过计算页码和偏移量来获取不同页的数据;利用BeautifulSoup和Requests进行网页爬取可以解析HTML并提取数据;利用Selenium进行自动化操作则可以模拟用户行为,适用于需要处理复杂的JavaScript渲染网页。下面将详细介绍如何使用这三种方法实现翻页。
一、利用循环和分页算法
这是最基础的方法,通过计算页码和偏移量来获取不同页的数据。假设一个API支持分页查询,返回结果中包含有下一页的链接或页码信息。
1、基本分页算法
分页算法通常包括两个变量:当前页码和每页记录数。通过循环控制页码来获取不同页的数据。
import requests
def fetch_page_data(url, page, page_size):
params = {
'page': page,
'page_size': page_size
}
response = requests.get(url, params=params)
return response.json()
base_url = 'https://example.com/api/data'
page_size = 20
current_page = 1
while True:
data = fetch_page_data(base_url, current_page, page_size)
if not data:
break
process_data(data)
current_page += 1
在这个例子中,fetch_page_data
函数用来获取指定页的数据,process_data
函数用来处理获取到的数据。循环通过控制current_page
和page_size
来获取不同页的数据,直到没有更多数据为止。
2、处理总页数
有些API会返回总记录数,通过计算总页数来控制循环。
import math
def fetch_page_data(url, page, page_size):
params = {
'page': page,
'page_size': page_size
}
response = requests.get(url, params=params)
return response.json()
def get_total_pages(total_records, page_size):
return math.ceil(total_records / page_size)
base_url = 'https://example.com/api/data'
page_size = 20
假设API返回总记录数
total_records = 1000
total_pages = get_total_pages(total_records, page_size)
for page in range(1, total_pages + 1):
data = fetch_page_data(base_url, page, page_size)
process_data(data)
在这个例子中,get_total_pages
函数用来计算总页数,循环通过控制page
来获取不同页的数据,直到达到总页数为止。
二、利用BeautifulSoup和Requests进行网页爬取
BeautifulSoup和Requests是常用的网页爬取工具,可以用来解析HTML并提取数据。
1、使用BeautifulSoup和Requests爬取单页数据
import requests
from bs4 import BeautifulSoup
def fetch_page_content(url):
response = requests.get(url)
return response.content
def parse_page(content):
soup = BeautifulSoup(content, 'html.parser')
items = soup.find_all('div', class_='item')
for item in items:
print(item.text)
url = 'https://example.com/page/1'
content = fetch_page_content(url)
parse_page(content)
在这个例子中,fetch_page_content
函数用来获取网页内容,parse_page
函数用来解析网页并提取数据。
2、处理分页
通过分析网页结构,找到分页链接或下一页按钮,循环获取不同页的数据。
import requests
from bs4 import BeautifulSoup
def fetch_page_content(url):
response = requests.get(url)
return response.content
def parse_page(content):
soup = BeautifulSoup(content, 'html.parser')
items = soup.find_all('div', class_='item')
for item in items:
print(item.text)
next_page = soup.find('a', class_='next-page')
return next_page['href'] if next_page else None
base_url = 'https://example.com'
current_page = '/page/1'
while current_page:
url = base_url + current_page
content = fetch_page_content(url)
current_page = parse_page(content)
在这个例子中,parse_page
函数除了解析数据外,还返回下一页链接,通过循环控制current_page
来获取不同页的数据,直到没有下一页为止。
三、利用Selenium进行自动化操作
Selenium是一个自动化测试工具,可以用来模拟用户行为,适用于需要处理复杂的JavaScript渲染网页。
1、基本使用
from selenium import webdriver
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get('https://example.com/page/1')
items = driver.find_elements(By.CLASS_NAME, 'item')
for item in items:
print(item.text)
driver.quit()
在这个例子中,Selenium用来打开网页并提取数据。
2、处理分页
通过模拟点击下一页按钮,循环获取不同页的数据。
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
driver = webdriver.Chrome()
driver.get('https://example.com/page/1')
while True:
items = driver.find_elements(By.CLASS_NAME, 'item')
for item in items:
print(item.text)
try:
next_page_button = driver.find_element(By.CLASS_NAME, 'next-page')
next_page_button.click()
except:
break
driver.quit()
在这个例子中,通过find_element
找到下一页按钮并模拟点击,循环获取不同页的数据,直到没有下一页为止。
四、使用Scrapy框架实现翻页
Scrapy是一个强大的爬取框架,适用于大规模爬取任务。
1、基本配置
安装Scrapy:
pip install scrapy
创建项目:
scrapy startproject myproject
2、编写Spider
在myproject/spiders
目录下创建一个Spider文件,例如example_spider.py
。
import scrapy
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['https://example.com/page/1']
def parse(self, response):
items = response.css('.item')
for item in items:
yield {
'text': item.css('::text').get()
}
next_page = response.css('.next-page::attr(href)').get()
if next_page:
yield response.follow(next_page, self.parse)
在这个例子中,通过response.css
选择器提取数据,并通过response.follow
处理分页。
3、运行爬虫
在项目根目录下运行爬虫:
scrapy crawl example
五、处理动态网页
对于一些需要处理动态内容的网页,可以结合Selenium和Scrapy。
1、安装Scrapy-Selenium
pip install scrapy-selenium
2、配置Scrapy-Selenium
在settings.py
中添加配置:
from shutil import which
SELENIUM_DRIVER_NAME = 'chrome'
SELENIUM_DRIVER_EXECUTABLE_PATH = which('chromedriver')
SELENIUM_DRIVER_ARGUMENTS = ['--headless']
DOWNLOADER_MIDDLEWARES = {
'scrapy_selenium.SeleniumMiddleware': 800
}
3、编写Spider
在myproject/spiders
目录下创建一个Spider文件,例如example_spider.py
。
import scrapy
from scrapy_selenium import SeleniumRequest
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['https://example.com/page/1']
def start_requests(self):
for url in self.start_urls:
yield SeleniumRequest(url=url, callback=self.parse)
def parse(self, response):
items = response.css('.item')
for item in items:
yield {
'text': item.css('::text').get()
}
next_page = response.css('.next-page::attr(href)').get()
if next_page:
yield SeleniumRequest(url=response.urljoin(next_page), callback=self.parse)
在这个例子中,通过SeleniumRequest
处理动态网页,并通过response.follow
处理分页。
六、处理反爬虫机制
在实际应用中,常常需要处理反爬虫机制。常见的反爬虫机制包括IP封禁、验证码、人机验证等。
1、更换IP
可以使用代理池更换IP地址。
import requests
proxies = {
'http': 'http://your_proxy_ip:port',
'https': 'https://your_proxy_ip:port'
}
response = requests.get('https://example.com', proxies=proxies)
print(response.content)
在这个例子中,通过设置proxies
参数更换IP地址。
2、处理验证码
可以使用打码平台或OCR技术识别验证码。
from PIL import Image
import pytesseract
image = Image.open('captcha.png')
text = pytesseract.image_to_string(image)
print(text)
在这个例子中,通过pytesseract
识别验证码。
3、模拟人机验证
可以使用Selenium模拟人机验证。
from selenium import webdriver
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get('https://example.com')
checkbox = driver.find_element(By.ID, 'recaptcha-checkbox')
checkbox.click()
driver.quit()
在这个例子中,通过find_element
找到人机验证的复选框并模拟点击。
七、总结
通过上述方法,可以使用Python实现翻页功能。利用循环和分页算法可以处理基础的分页需求;利用BeautifulSoup和Requests可以解析HTML并提取数据;利用Selenium可以模拟用户行为,处理复杂的JavaScript渲染网页;利用Scrapy可以进行大规模爬取任务;结合Selenium和Scrapy可以处理动态网页;处理反爬虫机制可以更稳定地获取数据。
在实际应用中,可以根据具体需求选择合适的方法,并结合多种技术手段应对复杂的网页结构和反爬虫机制。
相关问答FAQs:
使用Python实现翻页的基本方法有哪些?
在Python中,可以通过多种方式实现翻页功能,比如使用图形用户界面(GUI)库如Tkinter、PyQt,或者在Web应用中使用Flask和Django等框架。具体实现通常涉及到对数据的分页处理,以及更新显示内容的方法。对于命令行应用,可以通过输入特定命令来实现翻页。
在Web应用中,如何使用Python进行翻页设计?
在Web应用中,翻页设计通常涉及到后端数据的分段处理和前端展示。可以使用Flask或Django框架,结合数据库查询来实现。通过设置每页显示的记录数,后端可以根据请求参数来返回不同页的数据。前端则可以通过按钮或链接来切换不同的页码。
如何处理翻页时的数据加载和性能问题?
在进行翻页时,性能是一个重要考量。可以通过懒加载(lazy loading)或无限滚动(infinite scrolling)来优化用户体验。对于数据库查询,可以使用索引来加速数据检索。此外,考虑到数据量大时,可以选择预加载热门数据或使用缓存技术,减少每次翻页时的延迟。
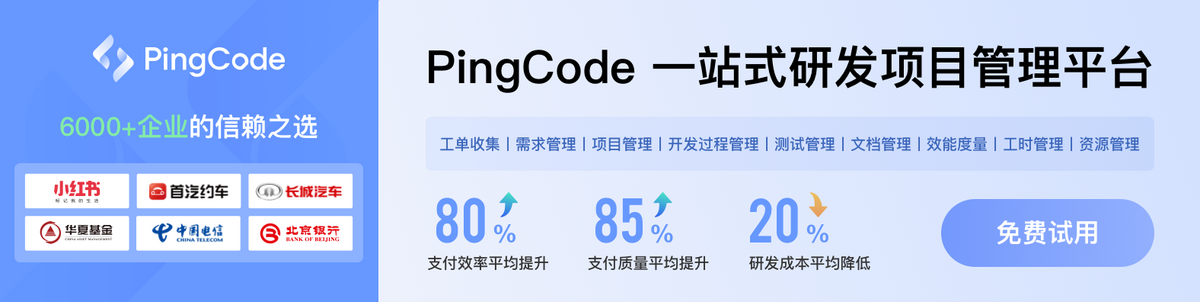