Python 验证导出成功的主要方法有:检查文件是否存在、检查文件内容、使用文件校验和、捕捉异常、检查文件大小。 检查文件是否存在是最基本的方法,它通过os.path.exists()函数来验证文件是否成功导出。接下来我们详细讲解一下如何使用这些方法来验证导出成功。
一、检查文件是否存在
验证导出成功的最基础方法是检查文件是否存在。使用Python标准库中的os模块,你可以轻松地检查某个文件是否存在。以下是一个示例代码:
import os
def check_file_exists(filepath):
if os.path.exists(filepath):
print(f"File {filepath} exists.")
else:
print(f"File {filepath} does not exist.")
在这个示例中,我们使用 os.path.exists()
方法来检查文件路径是否存在。如果文件存在,则返回 True
,否则返回 False
。这种方法简单且有效,但只能确认文件是否创建,而不能验证文件内容是否正确。
二、检查文件内容
为了确保文件内容的正确性,你可以读取文件并检查其内容。下面是一个示例代码,展示如何读取文本文件并检查其内容是否符合预期:
def check_file_content(filepath, expected_content):
with open(filepath, 'r') as file:
content = file.read()
if content == expected_content:
print(f"File content is as expected.")
else:
print(f"File content is not as expected.")
在这个示例中,我们打开文件并读取其内容,然后将读取的内容与预期内容进行比较。如果内容匹配,则表示文件导出成功且内容正确。
三、使用文件校验和
文件校验和(如MD5、SHA256等)是验证文件完整性的一种常用方法。通过计算文件的校验和并与预期校验和进行比较,可以确保文件没有被篡改。以下是一个示例代码,展示如何计算文件的SHA256校验和:
import hashlib
def calculate_file_checksum(filepath):
sha256 = hashlib.sha256()
with open(filepath, 'rb') as file:
for chunk in iter(lambda: file.read(4096), b""):
sha256.update(chunk)
return sha256.hexdigest()
def check_file_checksum(filepath, expected_checksum):
actual_checksum = calculate_file_checksum(filepath)
if actual_checksum == expected_checksum:
print(f"File checksum is as expected.")
else:
print(f"File checksum is not as expected.")
在这个示例中,我们使用 hashlib
模块计算文件的SHA256校验和,然后将计算得到的校验和与预期校验和进行比较。
四、捕捉异常
在导出文件的过程中,捕捉并处理可能出现的异常也是确保导出成功的一种方法。以下是一个示例代码,展示如何在导出文件时捕捉并处理异常:
def export_file(data, filepath):
try:
with open(filepath, 'w') as file:
file.write(data)
print(f"File exported successfully to {filepath}.")
except Exception as e:
print(f"Failed to export file: {e}")
data = "This is a test content."
filepath = "test_file.txt"
export_file(data, filepath)
在这个示例中,我们在导出文件的过程中使用 try
和 except
块来捕捉并处理可能出现的异常。如果导出成功,则输出成功信息;如果出现异常,则输出错误信息。
五、检查文件大小
文件大小是验证文件导出成功的另一种有效方法。你可以检查文件的大小是否符合预期,以确保文件内容的完整性。以下是一个示例代码:
def check_file_size(filepath, expected_size):
actual_size = os.path.getsize(filepath)
if actual_size == expected_size:
print(f"File size is as expected.")
else:
print(f"File size is not as expected.")
expected_size = len(data)
check_file_size(filepath, expected_size)
在这个示例中,我们使用 os.path.getsize()
方法获取文件的实际大小,并将其与预期大小进行比较。
结论
通过以上几种方法,你可以有效地验证文件是否成功导出。每种方法都有其独特的优点和适用场景,具体选择哪种方法取决于你的具体需求和应用场景。在实际应用中,你可以结合多种方法来确保文件导出的成功性和内容的完整性。
一、检查文件是否存在
检查文件是否存在是验证文件导出成功的最基本方法。它可以通过Python的os模块轻松实现。以下是一个更详细的示例代码,展示如何在实际项目中使用这一方法:
import os
def export_data_to_file(data, filepath):
with open(filepath, 'w') as file:
file.write(data)
def check_file_exists(filepath):
if os.path.exists(filepath):
print(f"File {filepath} exists.")
else:
print(f"File {filepath} does not exist.")
raise FileNotFoundError(f"File {filepath} was not found.")
data = "Sample data for export."
filepath = "output.txt"
Export data to file
export_data_to_file(data, filepath)
Check if file exists
check_file_exists(filepath)
在这个示例中,我们首先定义了一个 export_data_to_file
函数,用于将数据导出到指定文件路径。然后,我们调用 check_file_exists
函数来验证文件是否存在。如果文件不存在,则抛出 FileNotFoundError
异常。这种方法简单且高效,适用于大多数基本的文件导出验证场景。
二、检查文件内容
为了确保文件内容的正确性,除了检查文件是否存在外,还需要验证文件内容是否符合预期。以下是一个更详细的示例代码,展示如何在实际项目中使用这一方法:
def export_data_to_file(data, filepath):
with open(filepath, 'w') as file:
file.write(data)
def check_file_content(filepath, expected_content):
with open(filepath, 'r') as file:
content = file.read()
if content == expected_content:
print(f"File content is as expected.")
else:
print(f"File content is not as expected.")
raise ValueError("File content does not match expected content.")
data = "Sample data for export."
filepath = "output.txt"
expected_content = data
Export data to file
export_data_to_file(data, filepath)
Check file content
check_file_content(filepath, expected_content)
在这个示例中,我们首先定义了一个 export_data_to_file
函数,用于将数据导出到指定文件路径。然后,我们调用 check_file_content
函数来验证文件内容是否符合预期。如果文件内容不符合预期,则抛出 ValueError
异常。这种方法更为细致,适用于需要验证文件内容的场景。
三、使用文件校验和
文件校验和是一种确保文件内容完整性和正确性的重要方法,特别适用于需要防止文件被篡改的场景。以下是一个更详细的示例代码,展示如何在实际项目中使用这一方法:
import hashlib
def export_data_to_file(data, filepath):
with open(filepath, 'w') as file:
file.write(data)
def calculate_file_checksum(filepath):
sha256 = hashlib.sha256()
with open(filepath, 'rb') as file:
for chunk in iter(lambda: file.read(4096), b""):
sha256.update(chunk)
return sha256.hexdigest()
def check_file_checksum(filepath, expected_checksum):
actual_checksum = calculate_file_checksum(filepath)
if actual_checksum == expected_checksum:
print(f"File checksum is as expected.")
else:
print(f"File checksum is not as expected.")
raise ValueError("File checksum does not match expected checksum.")
data = "Sample data for export."
filepath = "output.txt"
Export data to file
export_data_to_file(data, filepath)
Calculate expected checksum
expected_checksum = hashlib.sha256(data.encode()).hexdigest()
Check file checksum
check_file_checksum(filepath, expected_checksum)
在这个示例中,我们首先定义了一个 export_data_to_file
函数,用于将数据导出到指定文件路径。然后,我们计算数据的预期SHA256校验和,并调用 check_file_checksum
函数来验证文件的实际校验和是否符合预期。如果校验和不匹配,则抛出 ValueError
异常。这种方法适用于需要确保文件内容完整性和防篡改的场景。
四、捕捉异常
捕捉并处理导出文件过程中可能出现的异常,是确保导出成功的一种有效方法。以下是一个更详细的示例代码,展示如何在实际项目中使用这一方法:
def export_data_to_file(data, filepath):
try:
with open(filepath, 'w') as file:
file.write(data)
print(f"File exported successfully to {filepath}.")
except IOError as e:
print(f"Failed to export file: {e}")
raise
except Exception as e:
print(f"An unexpected error occurred: {e}")
raise
data = "Sample data for export."
filepath = "output.txt"
Export data to file and handle exceptions
export_data_to_file(data, filepath)
在这个示例中,我们在导出文件的过程中使用 try
和 except
块来捕捉并处理可能出现的异常。如果导出成功,则输出成功信息;如果出现 IOError
异常,则输出失败信息并重新抛出异常;如果出现其他异常,则输出错误信息并重新抛出异常。这种方法适用于需要处理各种异常情况的场景。
五、检查文件大小
检查文件大小是验证文件导出成功的另一种有效方法。你可以检查文件的大小是否符合预期,以确保文件内容的完整性。以下是一个更详细的示例代码,展示如何在实际项目中使用这一方法:
import os
def export_data_to_file(data, filepath):
with open(filepath, 'w') as file:
file.write(data)
def check_file_size(filepath, expected_size):
actual_size = os.path.getsize(filepath)
if actual_size == expected_size:
print(f"File size is as expected.")
else:
print(f"File size is not as expected.")
raise ValueError("File size does not match expected size.")
data = "Sample data for export."
filepath = "output.txt"
expected_size = len(data)
Export data to file
export_data_to_file(data, filepath)
Check file size
check_file_size(filepath, expected_size)
在这个示例中,我们首先定义了一个 export_data_to_file
函数,用于将数据导出到指定文件路径。然后,我们调用 check_file_size
函数来验证文件的实际大小是否符合预期。如果文件大小不符合预期,则抛出 ValueError
异常。这种方法适用于需要确保文件大小正确的场景。
结论
通过以上几种方法,你可以有效地验证文件是否成功导出。每种方法都有其独特的优点和适用场景,具体选择哪种方法取决于你的具体需求和应用场景。在实际应用中,你可以结合多种方法来确保文件导出的成功性和内容的完整性。无论是检查文件是否存在、验证文件内容、使用文件校验和、捕捉异常,还是检查文件大小,这些方法都可以帮助你确保文件导出的正确性和完整性。
相关问答FAQs:
如何确认Python导出的文件格式正确?
在导出文件后,您可以通过检查文件扩展名和打开文件来验证其格式。确保导出的文件扩展名与您预期的一致,例如.csv、.json或.xlsx等。打开文件并检查内容,以确认数据的结构和格式是否符合预期。
在Python中如何捕捉导出过程中的错误?
使用异常处理机制(try-except语句)可以帮助您捕捉在导出过程中可能出现的错误。例如,如果您在写入文件时遇到IOError或其他异常,可以通过打印错误信息来了解问题所在,并进行相应的处理。
如何使用自动化测试验证导出结果的准确性?
可以编写自动化测试脚本,通过读取导出的文件并与原始数据进行对比,来验证导出结果的准确性。使用Python的unittest或pytest框架,可以轻松实现数据的比对,确保导出的数据与预期一致。
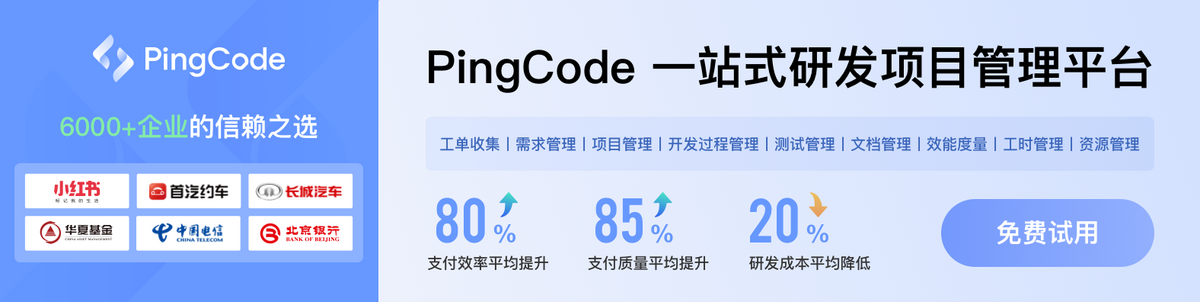