Python的PIL如何安装:使用pip安装、使用conda安装、从源码安装、在虚拟环境中安装、在不同操作系统中安装。
使用pip安装
Pillow(PIL的维护版本)是最常用的方式来安装PIL库。使用pip命令非常简单,只需运行以下命令:
pip install Pillow
详细描述: 这条命令会从Python Package Index (PyPI) 下载并安装Pillow库及其所有依赖项。Pillow是PIL库的一个分支和升级版,保持了PIL的API,同时解决了许多bug并添加了新的特性。安装完成后,你可以通过导入Pillow库来使用它。
使用conda安装
如果你使用的是Anaconda或Miniconda,则可以通过以下命令安装Pillow:
conda install Pillow
Anaconda会从其官方的包管理器中下载并安装Pillow库,这对某些系统来说可能更方便,尤其是当你管理多个数据科学或机器学习环境时。
从源码安装
有时候,你可能需要从源码安装Pillow,这种方法特别适合需要自定义编译选项的用户。你可以从Pillow的GitHub仓库克隆源码,并手动编译和安装:
git clone https://github.com/python-pillow/Pillow.git
cd Pillow
python setup.py install
在虚拟环境中安装
为了避免包冲突或其他环境问题,强烈建议在虚拟环境中安装Pillow。你可以使用virtualenv或venv来创建虚拟环境:
python -m venv myenv
source myenv/bin/activate # 在Windows上使用 myenv\Scripts\activate
pip install Pillow
这种方法确保Pillow及其依赖项只会影响当前的虚拟环境,不会干扰系统的全局设置。
在不同操作系统中安装
安装Pillow在不同操作系统中可能有些许差异。
在Windows上:
Windows用户可以直接使用pip命令来安装Pillow,没有特别的要求。然而,确保你已经安装了Python和pip。
在Linux上:
对于Linux用户,可能需要安装一些开发工具和库。在Ubuntu上,运行以下命令以确保所有依赖项都已安装:
sudo apt-get install python3-dev python3-setuptools
sudo apt-get install libjpeg8-dev zlib1g-dev
pip install Pillow
在macOS上:
macOS用户可能需要安装一些库,例如libjpeg和zlib。你可以使用Homebrew来安装这些库:
brew install libjpeg
brew install zlib
pip install Pillow
一、安装后验证
安装完成后,可以通过以下方式验证Pillow是否安装成功:
from PIL import Image
print(Image.__version__)
如果没有错误消息,并且输出了版本号,则表示安装成功。
二、Pillow的基本使用
安装成功后,你就可以开始使用Pillow来处理图像了。以下是一些基本的图像处理操作:
打开和显示图像
from PIL import Image
打开图像文件
img = Image.open('example.jpg')
显示图像
img.show()
图像转换
你可以将图像转换为其他格式,例如将JPEG图像转换为PNG:
img = Image.open('example.jpg')
img.save('example.png')
图像裁剪
你可以裁剪图像的一部分:
box = (100, 100, 400, 400)
cropped_img = img.crop(box)
cropped_img.show()
调整图像大小
调整图像的尺寸:
resized_img = img.resize((800, 600))
resized_img.show()
旋转图像
你可以旋转图像,例如旋转45度:
rotated_img = img.rotate(45)
rotated_img.show()
三、处理多个图像
Pillow也非常适合处理多个图像,例如批量处理图像文件夹中的所有图像。以下是一个示例,展示如何将文件夹中的所有JPEG图像转换为PNG:
import os
from PIL import Image
input_folder = 'input_images'
output_folder = 'output_images'
if not os.path.exists(output_folder):
os.makedirs(output_folder)
for filename in os.listdir(input_folder):
if filename.endswith('.jpg'):
img = Image.open(os.path.join(input_folder, filename))
output_file = os.path.splitext(filename)[0] + '.png'
img.save(os.path.join(output_folder, output_file))
四、图像增强和滤镜
Pillow还提供了一些图像增强和滤镜功能,例如调整对比度、亮度和颜色平衡:
调整亮度
from PIL import ImageEnhance
enhancer = ImageEnhance.Brightness(img)
bright_img = enhancer.enhance(1.5) # 增加亮度
bright_img.show()
调整对比度
enhancer = ImageEnhance.Contrast(img)
contrast_img = enhancer.enhance(2.0) # 增加对比度
contrast_img.show()
应用滤镜
from PIL import ImageFilter
blurred_img = img.filter(ImageFilter.BLUR)
blurred_img.show()
五、高级功能
Pillow除了基本的图像处理功能外,还提供了一些高级功能,例如绘图和文本处理。
绘制图形
from PIL import ImageDraw
draw = ImageDraw.Draw(img)
draw.rectangle([50, 50, 150, 150], outline="red", width=5)
img.show()
添加文本
from PIL import ImageFont
font = ImageFont.truetype("arial.ttf", 40)
draw.text((50, 50), "Hello, PIL!", font=font, fill="blue")
img.show()
六、图像格式支持
Pillow支持多种图像格式,包括但不限于:
- JPEG
- PNG
- BMP
- GIF
- TIFF
你可以使用Pillow来打开、保存和转换这些格式的图像。
七、性能优化
在处理大批量图像时,性能可能成为一个问题。以下是一些优化建议:
使用多线程
你可以使用Python的多线程库来并行处理图像,提高处理速度:
import threading
def process_image(filename):
img = Image.open(filename)
img = img.resize((800, 600))
img.save('resized_' + filename)
threads = []
for filename in os.listdir(input_folder):
thread = threading.Thread(target=process_image, args=(filename,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
使用缓存
如果你需要多次访问相同的图像,可以使用缓存来减少重复的I/O操作:
from functools import lru_cache
@lru_cache(maxsize=32)
def load_image(filename):
return Image.open(filename)
img = load_image('example.jpg')
img.show()
八、错误处理
在使用Pillow时,可能会遇到一些错误和异常。以下是一些常见的错误处理方法:
文件不存在
try:
img = Image.open('nonexistent.jpg')
except FileNotFoundError:
print("文件不存在")
无法识别的图像文件
try:
img = Image.open('corrupted.jpg')
except IOError:
print("无法识别的图像文件")
九、文档和社区资源
Pillow有一个活跃的开发者社区和丰富的文档资源。你可以访问以下资源来获取更多信息:
通过这些资源,你可以深入了解Pillow的所有功能,解决遇到的问题,并找到最佳实践。
十、总结
Pillow是一个强大的图像处理库,提供了丰富的功能和灵活的API。无论你是需要进行简单的图像处理,还是复杂的图像增强和过滤,Pillow都能满足你的需求。通过学习和掌握Pillow,你可以大大提高图像处理的效率和效果。
从安装到高级使用,本指南涵盖了Pillow的各个方面,希望能够帮助你更好地使用这个强大的工具进行图像处理。
相关问答FAQs:
如何在Python中安装Pillow库?
要安装Pillow库(PIL的一个分支),可以使用Python的包管理工具pip。打开命令行界面,输入以下命令进行安装:
pip install Pillow
确保你的Python环境已经正确配置,并且pip已成功安装。
Pillow库的安装过程中遇到错误该如何解决?
在安装Pillow时,可能会遇到一些常见问题,例如网络问题或权限不足。可以尝试使用管理员权限运行命令提示符,或检查网络连接。如果错误提示与库的依赖项有关,确保已经安装相关的开发工具和库,例如libjpeg和zlib等。
Pillow库安装后,如何验证安装是否成功?
安装完成后,可以通过在Python环境中导入Pillow来验证安装是否成功。在Python交互式命令行或脚本中,输入以下命令:
from PIL import Image
print("Pillow安装成功!")
如果没有出现错误,说明安装已经成功,可以开始使用Pillow库进行图像处理。
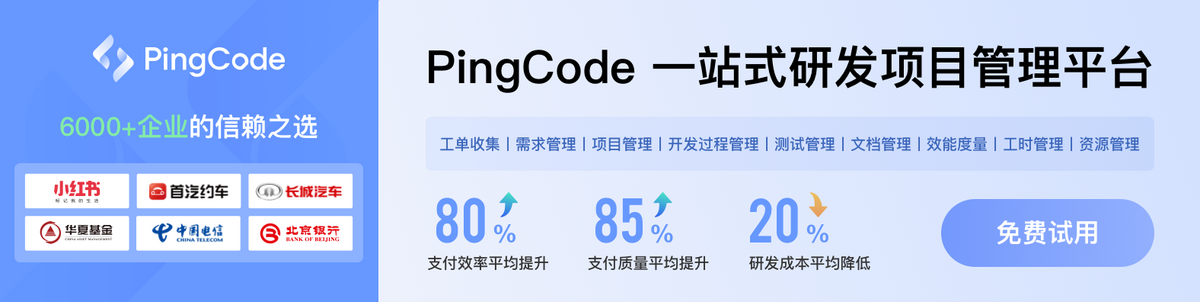