在Python中实现天气信息有多种方法,主要依赖于调用天气API、使用Web爬虫等方式。使用天气API、解析JSON数据、处理数据并展示、错误处理是几种有效的方法。其中,使用天气API是最常见且便捷的方法。以下将详细介绍如何通过API获取天气信息并进行处理和展示。
一、API概述及获取API密钥
使用天气API:可以通过调用第三方天气服务提供的API来获取实时天气信息。知名的天气API提供商包括OpenWeatherMap、WeatherAPI、WeatherStack等。
1、获取API密钥
首先,需要在天气API提供商的网站上注册并获取API密钥。以OpenWeatherMap为例,可以按照以下步骤操作:
- 访问OpenWeatherMap官网,注册一个账号。
- 登录账号后,前往API页面,选择适合自己的API服务。
- 申请并获取API密钥。
二、使用OpenWeatherMap API获取天气信息
1、安装所需的库
使用requests库来发送HTTP请求,并解析API返回的JSON数据。
pip install requests
2、编写Python代码
下面是一个简单的示例代码,通过OpenWeatherMap API获取指定城市的天气信息:
import requests
def get_weather(api_key, city):
base_url = "http://api.openweathermap.org/data/2.5/weather"
params = {
'q': city,
'appid': api_key,
'units': 'metric' # 使用摄氏度
}
response = requests.get(base_url, params=params)
data = response.json()
if response.status_code == 200:
main = data['main']
weather = data['weather'][0]
temperature = main['temp']
humidity = main['humidity']
description = weather['description']
print(f"City: {city}")
print(f"Temperature: {temperature}°C")
print(f"Humidity: {humidity}%")
print(f"Weather Description: {description}")
else:
print(f"Error: {data['message']}")
if __name__ == "__main__":
api_key = 'YOUR_API_KEY'
city = 'London'
get_weather(api_key, city)
在上面的代码中,主要步骤包括:
- 构建API请求URL,并传递必要的参数(城市名称、API密钥、温度单位等)。
- 使用requests库发送HTTP GET请求。
- 解析返回的JSON数据,提取所需的天气信息(温度、湿度、天气描述等)。
- 打印天气信息。
三、解析JSON数据
在获取到API返回的JSON数据后,需要对其进行解析,提取出有用的信息。以下是一个简单的示例,展示如何提取JSON中的特定字段:
import json
json_data = '''
{
"coord": {"lon": -0.1257, "lat": 51.5085},
"weather": [{"id": 800, "main": "Clear", "description": "clear sky", "icon": "01d"}],
"main": {"temp": 15.0, "feels_like": 14.6, "temp_min": 13.0, "temp_max": 17.0, "pressure": 1020, "humidity": 77},
"name": "London"
}
'''
data = json.loads(json_data)
temperature = data['main']['temp']
humidity = data['main']['humidity']
description = data['weather'][0]['description']
print(f"Temperature: {temperature}°C")
print(f"Humidity: {humidity}%")
print(f"Weather Description: {description}")
四、处理数据并展示
获取和解析天气数据后,可以选择在控制台打印、在Web页面展示,或者绘制图表等方式展示数据。
1、在控制台打印
通过简单的print语句,将天气信息打印到控制台。
print(f"Temperature: {temperature}°C")
print(f"Humidity: {humidity}%")
print(f"Weather Description: {description}")
2、在Web页面展示
可以使用Flask等Web框架,将天气信息展示在Web页面上。
from flask import Flask, render_template
import requests
app = Flask(__name__)
@app.route('/weather/<city>')
def weather(city):
api_key = 'YOUR_API_KEY'
base_url = "http://api.openweathermap.org/data/2.5/weather"
params = {
'q': city,
'appid': api_key,
'units': 'metric'
}
response = requests.get(base_url, params=params)
data = response.json()
if response.status_code == 200:
main = data['main']
weather = data['weather'][0]
temperature = main['temp']
humidity = main['humidity']
description = weather['description']
return render_template('weather.html', city=city, temperature=temperature, humidity=humidity, description=description)
else:
return f"Error: {data['message']}"
if __name__ == "__main__":
app.run(debug=True)
3、绘制图表
使用matplotlib库绘制图表,展示一段时间内的天气变化情况。
import matplotlib.pyplot as plt
示例数据
dates = ['2023-10-01', '2023-10-02', '2023-10-03', '2023-10-04', '2023-10-05']
temperatures = [15, 17, 16, 14, 18]
plt.plot(dates, temperatures, marker='o')
plt.xlabel('Date')
plt.ylabel('Temperature (°C)')
plt.title('Temperature over Time')
plt.show()
五、错误处理
在调用API和处理数据时,可能会遇到各种错误情况,如网络错误、API返回错误信息等。需要进行适当的错误处理,确保程序的健壮性。
1、网络错误
使用try-except块捕获网络错误,并进行相应的处理。
try:
response = requests.get(base_url, params=params)
response.raise_for_status()
except requests.exceptions.RequestException as e:
print(f"Network error: {e}")
return
2、API返回错误信息
检查API返回的状态码和错误信息,并进行相应的处理。
if response.status_code == 200:
# 处理数据
else:
print(f"Error: {data['message']}")
六、使用其他API
除了OpenWeatherMap,还有其他许多天气API可以使用,如WeatherAPI、WeatherStack等。不同的API可能有不同的调用方式和返回数据结构,需要根据具体API的文档进行适当的调整。
1、WeatherAPI
WeatherAPI提供了丰富的天气数据接口,使用方法类似于OpenWeatherMap。
import requests
def get_weather(api_key, city):
base_url = "http://api.weatherapi.com/v1/current.json"
params = {
'key': api_key,
'q': city,
'aqi': 'no'
}
response = requests.get(base_url, params=params)
data = response.json()
if response.status_code == 200:
current = data['current']
temperature = current['temp_c']
humidity = current['humidity']
description = current['condition']['text']
print(f"City: {city}")
print(f"Temperature: {temperature}°C")
print(f"Humidity: {humidity}%")
print(f"Weather Description: {description}")
else:
print(f"Error: {data['error']['message']}")
if __name__ == "__main__":
api_key = 'YOUR_API_KEY'
city = 'London'
get_weather(api_key, city)
2、WeatherStack
WeatherStack提供了简单易用的天气API,使用方法也类似。
import requests
def get_weather(api_key, city):
base_url = "http://api.weatherstack.com/current"
params = {
'access_key': api_key,
'query': city
}
response = requests.get(base_url, params=params)
data = response.json()
if 'current' in data:
current = data['current']
temperature = current['temperature']
humidity = current['humidity']
description = current['weather_descriptions'][0]
print(f"City: {city}")
print(f"Temperature: {temperature}°C")
print(f"Humidity: {humidity}%")
print(f"Weather Description: {description}")
else:
print(f"Error: {data['error']['info']}")
if __name__ == "__main__":
api_key = 'YOUR_API_KEY'
city = 'London'
get_weather(api_key, city)
七、将天气信息整合到项目中
天气信息通常是项目的一部分,可以将其整合到更大的项目中。例如,将天气信息集成到智能家居系统、旅游推荐系统等。
1、智能家居系统
在智能家居系统中,可以根据当前天气情况自动调整室内温度、湿度等环境参数,提升用户的舒适度。
2、旅游推荐系统
在旅游推荐系统中,可以根据目的地的天气情况,推荐适合的旅游时间和活动,并提供相应的出行建议。
八、总结
通过调用天气API获取天气信息、解析返回的JSON数据、处理并展示数据、进行错误处理,可以实现一个功能完备的天气信息系统。不同的API提供商可能有不同的调用方式和数据结构,需要根据具体情况进行调整。在实际项目中,可以将天气信息整合到更大的系统中,提供更丰富的功能和服务。
相关问答FAQs:
如何使用Python获取实时天气信息?
使用Python获取实时天气信息通常涉及调用天气API,例如OpenWeatherMap或WeatherAPI。您需要注册获取API密钥,然后通过HTTP请求获取天气数据。使用requests库可以方便地发送请求和解析返回的JSON数据,从中提取所需的天气信息。
在Python中如何处理天气数据的格式化?
处理天气数据时,通常需要对API返回的数据进行格式化,以便更易于理解和展示。您可以使用Python的pandas库来处理和分析数据,或者使用内置的json库来解析JSON数据。格式化后,您可以将数据转化为易读的字符串,或者将其输出为CSV文件,以便进行进一步分析。
Python中有哪些库可用于天气信息的可视化?
在Python中,有多个库可以帮助您可视化天气信息。Matplotlib和Seaborn是常用的绘图库,可以用于创建图表和图形,展示温度变化、降水量等信息。此外,Plotly和Bokeh提供了更为互动的可视化效果,可以使您的数据展示更生动。使用这些工具,您可以创建热图、折线图等多种形式的天气数据可视化。
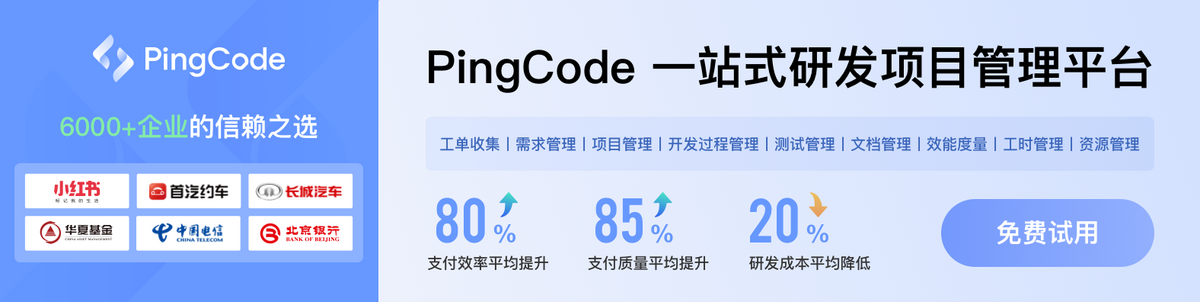