使用Python绘制半圆可以通过多个方法实现,主要方法包括使用matplotlib库、使用turtle库、以及使用pygame库。其中最常用和方便的方法是使用matplotlib库,它提供了强大的绘图库,能够轻松实现包括半圆在内的各种图形绘制。下面我们详细介绍如何使用matplotlib库绘制半圆。
一、安装必要的库
首先,在开始编写代码之前,需要确保安装了必要的库。可以使用以下命令安装matplotlib库:
pip install matplotlib
二、使用matplotlib绘制半圆
1. 绘制基本半圆
要绘制一个基本的半圆,可以使用matplotlib的Arc
类。下面是一个示例代码:
import matplotlib.pyplot as plt
from matplotlib.patches import Arc
def draw_half_circle():
fig, ax = plt.subplots()
# 创建一个半圆弧,center是圆心坐标,width和height是椭圆的宽度和高度,theta1和theta2分别是起始角度和终止角度
half_circle = Arc((0, 0), width=2, height=2, theta1=0, theta2=180, edgecolor='blue')
ax.add_patch(half_circle)
ax.set_xlim(-2, 2)
ax.set_ylim(-2, 2)
ax.set_aspect('equal', 'box')
plt.grid(True)
plt.show()
draw_half_circle()
详细解释:
- Arc类:
Arc
类用于创建弧形。参数包括圆心坐标center
、宽度width
、高度height
、起始角度theta1
、终止角度theta2
。 - add_patch:
ax.add_patch
方法将创建的弧形添加到子图中。 - set_xlim、set_ylim:用于设置x轴和y轴的显示范围。
- set_aspect:确保图形的横纵比相等。
2. 半圆的填充
如果需要填充半圆,可以使用Patch
类中的Wedge
类。以下是一个示例代码:
import matplotlib.pyplot as plt
from matplotlib.patches import Wedge
def draw_filled_half_circle():
fig, ax = plt.subplots()
# 创建一个填充半圆,center是圆心坐标,r是半径,theta1和theta2分别是起始角度和终止角度
filled_half_circle = Wedge((0, 0), r=1, theta1=0, theta2=180, facecolor='blue')
ax.add_patch(filled_half_circle)
ax.set_xlim(-2, 2)
ax.set_ylim(-2, 2)
ax.set_aspect('equal', 'box')
plt.grid(True)
plt.show()
draw_filled_half_circle()
三、使用turtle库绘制半圆
turtle库是Python的标准库之一,适用于绘制基本图形。以下是使用turtle库绘制半圆的示例代码:
import turtle
def draw_half_circle():
turtle.speed(1)
turtle.penup()
turtle.goto(0, 0)
turtle.pendown()
turtle.circle(100, 180) # 半径为100,绘制180度的弧线
turtle.done()
draw_half_circle()
详细解释:
- turtle.circle(radius, extent):
circle
方法用于绘制圆或弧线。radius
是半径,extent
是绘制的角度。 - penup、pendown:用于抬起和放下画笔。
四、使用pygame库绘制半圆
pygame是一个用于制作2D游戏的第三方库,也可以用来绘制基本图形。以下是使用pygame库绘制半圆的示例代码:
import pygame
import sys
def draw_half_circle():
pygame.init()
screen = pygame.display.set_mode((400, 300))
pygame.display.set_caption('Draw Half Circle')
# 设置颜色
blue = (0, 0, 255)
# 圆心坐标和半径
center = (200, 150)
radius = 100
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.fill((255, 255, 255))
# 绘制半圆
pygame.draw.arc(screen, blue, [center[0]-radius, center[1]-radius, 2*radius, 2*radius], 0, 3.14159, 2)
pygame.display.flip()
draw_half_circle()
详细解释:
- pygame.draw.arc:用于绘制弧线。参数包括屏幕对象
screen
、颜色color
、矩形区域rect
、起始角度start_angle
、终止角度stop_angle
、线宽width
。 - pygame.display.flip():更新屏幕显示。
五、综合比较与实际应用
1. 比较各方法的优缺点
- matplotlib:功能强大,适用于科学计算和数据可视化。绘制精度高,适合生成高质量的图形。
- turtle:简单易用,适合初学者和教育用途。图形精度较低,不适合复杂图形。
- pygame:适用于游戏开发,功能较为全面。适合实时交互和动态图形。
2. 实际应用场景
- 数据可视化:在科学研究和数据分析中,使用matplotlib绘制各种图形,包括半圆。
- 教育用途:使用turtle库教授编程和基本图形绘制。
- 游戏开发:在2D游戏中,使用pygame库绘制游戏元素和图形。
六、代码优化与扩展
1. 动态绘制半圆
可以将半圆的绘制过程动态展示出来,增强用户体验。以下是matplotlib动态绘制半圆的示例代码:
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
import numpy as np
def draw_dynamic_half_circle():
fig, ax = plt.subplots()
ax.set_xlim(-2, 2)
ax.set_ylim(-2, 2)
ax.set_aspect('equal', 'box')
plt.grid(True)
line, = ax.plot([], [], 'b-')
def init():
line.set_data([], [])
return line,
def update(frame):
theta = np.linspace(0, np.pi, frame)
x = np.cos(theta)
y = np.sin(theta)
line.set_data(x, y)
return line,
ani = FuncAnimation(fig, update, frames=100, init_func=init, blit=True)
plt.show()
draw_dynamic_half_circle()
2. 扩展到3D半圆
可以使用matplotlib的3D绘图库绘制三维半圆。以下是一个示例代码:
import matplotlib.pyplot as plt
import numpy as np
from mpl_toolkits.mplot3d import Axes3D
def draw_3d_half_circle():
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
theta = np.linspace(0, np.pi, 100)
z = np.linspace(0, 1, 100)
theta, z = np.meshgrid(theta, z)
x = np.cos(theta)
y = np.sin(theta)
ax.plot_surface(x, y, z, cmap='viridis')
plt.show()
draw_3d_half_circle()
七、总结
通过上述方法,我们可以使用Python中的不同库绘制半圆。其中,matplotlib库是最常用和方便的选择,它提供了强大的绘图库,能够轻松实现包括半圆在内的各种图形绘制。turtle库适合初学者,而pygame库适合游戏开发。根据实际需求选择合适的方法,可以高效地实现图形绘制。
相关问答FAQs:
如何使用Python绘制半圆的基本步骤是什么?
要使用Python绘制半圆,首先需要安装一个图形库,如Matplotlib。安装完成后,可以使用matplotlib.pyplot
模块中的plot
或fill
函数来绘制半圆。具体步骤包括定义半圆的参数(如半径和中心坐标),使用极坐标或参数方程生成半圆的点,然后将这些点绘制到图形中。
有没有示例代码可以参考?
当然可以。以下是一个简单的示例代码,使用Matplotlib绘制半圆:
import numpy as np
import matplotlib.pyplot as plt
# 设置半径和中心
radius = 1
theta = np.linspace(0, np.pi, 100) # 生成从0到π的100个点
# 计算半圆的x和y坐标
x = radius * np.cos(theta)
y = radius * np.sin(theta)
# 创建图形
plt.figure()
plt.fill(x, y, 'b') # 填充半圆为蓝色
plt.xlim(-1.5, 1.5)
plt.ylim(-0.5, 1.5)
plt.gca().set_aspect('equal') # 设置图形比例
plt.title('Half Circle')
plt.show()
这个代码片段将绘制一个半径为1的蓝色半圆。
在绘制半圆时可以添加哪些自定义选项?
在绘制半圆时,可以添加多种自定义选项,例如改变半圆的颜色、边框样式、透明度等。可以使用fill
函数中的参数来设置颜色,使用edgecolor
来指定边框颜色,alpha
参数可以设置填充的透明度。此外,还可以在图中添加标题、坐标轴标签、网格线等,以增强图形的可读性和美观度。
如何将绘制的半圆保存为图片文件?
在使用Matplotlib绘制半圆后,可以使用savefig
函数将图形保存为图片文件。只需在绘图代码的末尾添加以下行:
plt.savefig('half_circle.png') # 保存为PNG格式
这样可以将当前图形保存为指定名称的文件,支持多种格式,如PNG、JPEG、PDF等。
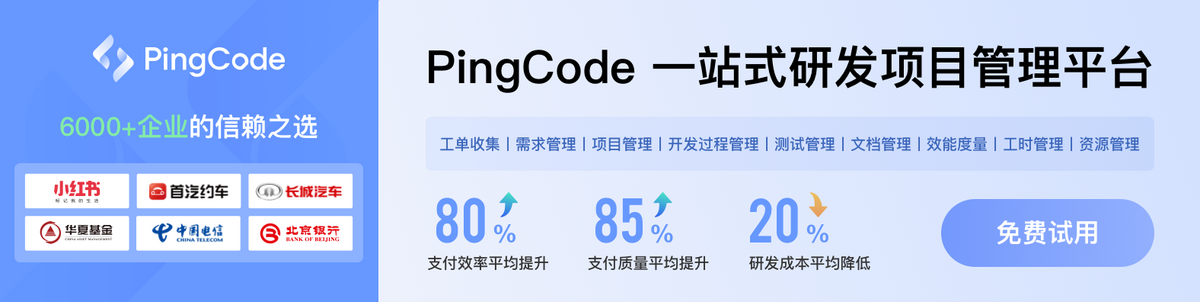