Python代码删除空格的方法有多种,包括使用内置函数、正则表达式、列表解析等。主要方法包括:strip()方法、replace()方法、正则表达式、列表解析等。下面将详细描述使用replace()方法删除空格的过程。
使用replace()方法可以轻松地删除字符串中的所有空格。replace()方法的语法是str.replace(old, new[, count]),其中old是要替换的子字符串,new是替换后的子字符串,count是可选参数,表示替换的次数。通过将old设置为空格字符(' ')并将new设置为空字符串(''),我们可以删除所有空格。
# 示例代码
text = "Hello World! This is a test."
text_no_spaces = text.replace(" ", "")
print(text_no_spaces)
上述代码将输出“HelloWorld!Thisisatest.”,其中所有空格都被删除。
接下来,我们将深入探讨Python中删除空格的其他方法及其应用场景。
一、STRIP()方法
strip()方法用于删除字符串开头和结尾的空格。它有两个变体:lstrip()和rstrip(),分别用于删除左侧和右侧的空格。
1、strip()方法
# 示例代码
text = " Hello World! "
text_strip = text.strip()
print(text_strip) # 输出: "Hello World!"
2、lstrip()方法
# 示例代码
text = " Hello World! "
text_lstrip = text.lstrip()
print(text_lstrip) # 输出: "Hello World! "
3、rstrip()方法
# 示例代码
text = " Hello World! "
text_rstrip = text.rstrip()
print(text_rstrip) # 输出: " Hello World!"
二、REPLACE()方法
replace()方法不仅可以删除空格,还可以替换字符串中的任意字符。它在处理字符串时非常灵活。
1、删除所有空格
# 示例代码
text = "Hello World! This is a test."
text_no_spaces = text.replace(" ", "")
print(text_no_spaces) # 输出: "HelloWorld!Thisisatest."
2、替换部分空格
# 示例代码
text = "Hello World! This is a test."
text_partial_replace = text.replace(" ", "_", 2)
print(text_partial_replace) # 输出: "Hello_World!_This is a test."
三、正则表达式
正则表达式(regular expressions)是一种强大的字符串处理工具。在Python中,可以使用re模块来处理正则表达式。
1、删除所有空格
import re
示例代码
text = "Hello World! This is a test."
text_no_spaces = re.sub(r"\s+", "", text)
print(text_no_spaces) # 输出: "HelloWorld!Thisisatest."
2、删除开头和结尾的空格
import re
示例代码
text = " Hello World! "
text_strip = re.sub(r"^\s+|\s+$", "", text)
print(text_strip) # 输出: "Hello World!"
四、列表解析
列表解析(list comprehension)是一种简洁高效的生成列表的方法。通过结合join()方法,可以删除字符串中的空格。
1、删除所有空格
# 示例代码
text = "Hello World! This is a test."
text_no_spaces = "".join([c for c in text if c != " "])
print(text_no_spaces) # 输出: "HelloWorld!Thisisatest."
2、删除特定位置的空格
# 示例代码
text = "Hello World! This is a test."
text_partial_remove = "".join([c if i % 2 == 0 else "" for i, c in enumerate(text)])
print(text_partial_remove) # 输出: "Hlo ol!Ti s at."
五、其他方法
除了上述方法外,还有一些其他方法可以用来删除空格,例如使用translate()方法。
1、translate()方法
translate()方法通过字符映射表来替换字符串中的字符。可以使用str.maketrans()方法生成映射表。
# 示例代码
text = "Hello World! This is a test."
translation_table = str.maketrans("", "", " ")
text_no_spaces = text.translate(translation_table)
print(text_no_spaces) # 输出: "HelloWorld!Thisisatest."
六、性能比较
不同方法在删除空格时的性能差异可以通过使用timeit模块进行比较。以下是一个简单的性能测试示例:
import timeit
测试文本
text = "Hello World! This is a test."
strip()方法
def strip_method():
return text.strip()
replace()方法
def replace_method():
return text.replace(" ", "")
正则表达式方法
def regex_method():
import re
return re.sub(r"\s+", "", text)
列表解析方法
def list_comprehension_method():
return "".join([c for c in text if c != " "])
translate()方法
def translate_method():
translation_table = str.maketrans("", "", " ")
return text.translate(translation_table)
性能测试
methods = [strip_method, replace_method, regex_method, list_comprehension_method, translate_method]
for method in methods:
time = timeit.timeit(method, number=100000)
print(f"{method.__name__}: {time:.5f} seconds")
通过上述代码,可以比较不同方法的性能表现,选择最适合的删除空格方法。
七、应用场景
不同方法适用于不同的应用场景。根据实际需求选择合适的方法,可以提高代码的可读性和性能。
1、处理用户输入
在处理用户输入时,通常需要删除开头和结尾的空格。strip()方法是一个常用的选择。
# 示例代码
user_input = " user input "
clean_input = user_input.strip()
print(clean_input) # 输出: "user input"
2、格式化字符串
在格式化字符串时,可能需要删除所有空格。replace()方法和正则表达式方法都可以完成此任务。
# 示例代码
text = "Hello World! This is a test."
formatted_text = text.replace(" ", "")
print(formatted_text) # 输出: "HelloWorld!Thisisatest."
3、处理大文本
在处理大文本时,性能是一个重要的考虑因素。translate()方法在删除空格时通常具有较高的性能。
# 示例代码
text = "Hello World! This is a test." * 1000
translation_table = str.maketrans("", "", " ")
text_no_spaces = text.translate(translation_table)
print(text_no_spaces) # 输出: "HelloWorld!Thisisatest." * 1000
八、总结
删除空格是Python字符串处理中的常见任务。通过使用不同的方法,如strip()、replace()、正则表达式、列表解析和translate(),可以满足不同的需求。在选择方法时,需要考虑代码的可读性、性能和具体应用场景。通过合理选择合适的方法,可以提高代码的效率和可维护性。
总之,掌握多种删除空格的方法,可以让你在处理字符串时更加游刃有余。
相关问答FAQs:
如何在Python中删除字符串开头和结尾的空格?
在Python中,可以使用strip()
方法来删除字符串两端的空格。例如,my_string.strip()
将返回一个新的字符串,其中开头和结尾的空格已被移除。这个方法不会改变原始字符串,因为字符串是不可变的。
有没有方法可以删除字符串中间的所有空格?
可以使用replace()
方法来移除字符串中间的所有空格。通过my_string.replace(" ", "")
,您可以获得一个没有空格的新字符串。如果您只想去掉多余的空格而保留单个空格,可以使用正则表达式模块re
中的sub()
函数,来将多个空格替换为一个空格。
如何在列表中删除每个字符串的空格?
如果您有一个包含字符串的列表,可以使用列表推导式来删除每个元素的空格。例如,cleaned_list = [s.strip() for s in my_list]
将创建一个新列表,其中每个字符串的开头和结尾的空格已被去除。如果需要同时处理中间的空格,可以结合使用replace()
或正则表达式。
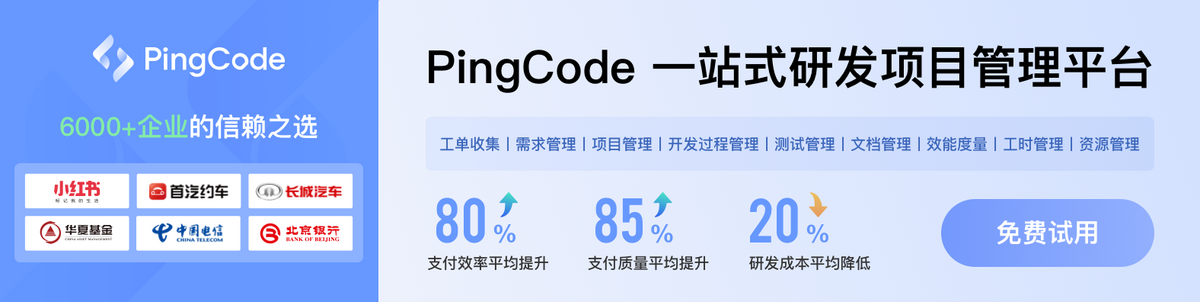