要用Python编写一个抽签程序,可以使用一些基本的Python库和逻辑来实现。基本步骤包括:导入所需库、定义参与者列表、随机选择、显示结果。 其中,随机选择是核心部分,Python的random
库提供了许多便捷的方法来实现这一点。
一、导入所需库
首先,我们需要导入Python的random
库,它包含了我们需要的随机函数。
import random
二、定义参与者列表
接下来,我们需要定义一个参与者列表,这个列表可以包含任意数量的名字。
participants = ['Alice', 'Bob', 'Charlie', 'David', 'Eve']
三、随机选择
使用random.choice()
函数来从列表中随机选择一个名字。
winner = random.choice(participants)
四、显示结果
最后,将结果打印出来。
print(f"The winner is {winner}!")
详细实现及扩展
为了让程序更加专业和灵活,我们可以添加一些功能,比如:允许用户输入参与者名单、进行多次抽签、处理异常情况等。下面是一个更完整的示例。
import random
def draw_lots(participants, num_winners=1):
if num_winners > len(participants):
raise ValueError("Number of winners cannot be greater than the number of participants")
winners = random.sample(participants, num_winners)
return winners
def main():
# 获取用户输入
participants = input("Enter the participants' names, separated by commas: ").split(',')
participants = [name.strip() for name in participants]
num_winners = int(input("Enter the number of winners: "))
try:
winners = draw_lots(participants, num_winners)
print(f"The winners are: {', '.join(winners)}")
except ValueError as e:
print(e)
if __name__ == "__main__":
main()
一、导入库与定义主要函数
我们在代码开头导入了random
库并定义了一个draw_lots
函数来进行抽签操作。这个函数使用random.sample()
来从参与者列表中随机选择指定数量的获胜者。
import random
def draw_lots(participants, num_winners=1):
if num_winners > len(participants):
raise ValueError("Number of winners cannot be greater than the number of participants")
winners = random.sample(participants, num_winners)
return winners
二、获取用户输入
在main
函数中,我们获取用户输入的参与者名单并将其转换为列表,同时获取用户输入的获胜者数量。
def main():
participants = input("Enter the participants' names, separated by commas: ").split(',')
participants = [name.strip() for name in participants]
num_winners = int(input("Enter the number of winners: "))
三、进行抽签并处理异常
我们调用draw_lots
函数进行抽签,并捕获可能的异常(例如用户输入的获胜者数量大于参与者数量)。
try:
winners = draw_lots(participants, num_winners)
print(f"The winners are: {', '.join(winners)}")
except ValueError as e:
print(e)
四、运行程序
最后,我们通过检查__name__
变量来运行main
函数。这是一个常见的Python惯例,用于确保代码在作为脚本运行时执行,而不是在被导入时执行。
if __name__ == "__main__":
main()
扩展与优化
- 增加抽签方式:可以增加不同的抽签方式,比如顺序抽签、单独抽签等。
- 存储结果:将抽签结果存储到文件或数据库中,以便后续查询和分析。
- 图形界面:使用Tkinter或其他GUI库创建图形界面,使用户操作更加简便。
- 网络应用:将程序部署为Web应用,使用Flask或Django框架,让更多人可以通过浏览器进行抽签。
示例:添加图形界面
下面是使用Tkinter创建的简单图形界面抽签程序示例:
import random
import tkinter as tk
from tkinter import messagebox
def draw_lots(participants, num_winners=1):
if num_winners > len(participants):
raise ValueError("Number of winners cannot be greater than the number of participants")
winners = random.sample(participants, num_winners)
return winners
def on_draw_click():
participants = entry_participants.get().split(',')
participants = [name.strip() for name in participants]
num_winners = int(entry_num_winners.get())
try:
winners = draw_lots(participants, num_winners)
messagebox.showinfo("Winners", f"The winners are: {', '.join(winners)}")
except ValueError as e:
messagebox.showerror("Error", str(e))
创建主窗口
root = tk.Tk()
root.title("抽签程序")
创建并放置组件
tk.Label(root, text="Participants (comma separated):").grid(row=0, column=0)
entry_participants = tk.Entry(root)
entry_participants.grid(row=0, column=1)
tk.Label(root, text="Number of winners:").grid(row=1, column=0)
entry_num_winners = tk.Entry(root)
entry_num_winners.grid(row=1, column=1)
btn_draw = tk.Button(root, text="Draw", command=on_draw_click)
btn_draw.grid(row=2, columnspan=2)
运行主循环
root.mainloop()
结论
通过上述步骤,我们可以使用Python轻松创建一个功能完备的抽签程序。从简单的命令行版本到带图形界面的应用,Python都能提供强大的支持。 根据实际需求,开发者可以进一步扩展和优化程序,使其更加符合用户的需求。
相关问答FAQs:
如何使用Python创建一个简单的抽签程序?
要创建一个简单的抽签程序,可以使用Python内置的random
模块。首先,您需要准备一个参与抽签的名单,然后使用random.choice()
函数从名单中随机选择一个参与者。以下是一个基本的示例代码:
import random
participants = ['Alice', 'Bob', 'Charlie', 'David']
winner = random.choice(participants)
print(f'The winner is: {winner}')
这个程序会随机从参与者中抽取一个赢家。
抽签程序可以支持哪些功能?
除了基本的随机选择功能,抽签程序还可以增加多种功能。例如:
- 多轮抽签:允许用户进行多轮抽签,直到确定最终赢家。
- 排除某些参与者:在某些情况下,您可能需要排除特定的参与者。
- 设置权重:对参与者设置不同的权重,以影响他们被抽中的概率。
- 保存历史记录:记录每次抽签的结果,便于后续查询。
如何确保抽签结果的随机性和公平性?
为了确保抽签的随机性和公平性,可以使用以下方法:
- 使用高质量的随机数生成器:Python的
random
模块基于梅森旋转算法,适合大多数用途,但对于更高的安全性需求,可以考虑使用secrets
模块。 - 避免人为干预:确保抽签过程不受外部因素影响,保持程序的独立性。
- 多次验证:可以进行多次抽签,确保每次结果的随机性相似。
通过这些方法,您可以增加抽签程序的公正性和可信度。
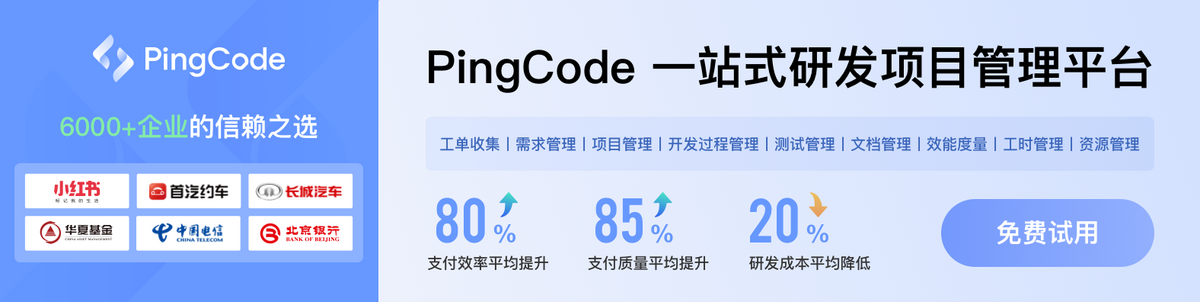