Python调用物联网接口的方法主要包括:使用HTTP请求、使用MQTT协议、使用WebSocket协议。使用HTTP请求是最常见且容易实现的方法。通过Python的requests库发送HTTP请求,可以方便地与物联网设备进行通信。下面详细介绍如何使用HTTP请求来调用物联网接口。
使用HTTP请求的步骤如下:
- 安装requests库。
- 构建HTTP请求。
- 发送HTTP请求并处理响应。
- 解析响应数据。
一、安装requests库
在使用requests库之前,需要确保已经安装该库。可以使用以下命令安装:
pip install requests
二、构建HTTP请求
构建HTTP请求时,需要确定请求的类型(GET、POST等)、目标URL、请求头和请求体。以下是一个简单的示例,演示如何构建和发送一个GET请求。
三、发送HTTP请求并处理响应
发送HTTP请求并处理响应是调用物联网接口的关键步骤。以下是一个示例代码,展示如何使用requests库发送GET请求并处理响应。
import requests
url = 'http://example.com/api/device'
headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer YOUR_ACCESS_TOKEN'
}
response = requests.get(url, headers=headers)
if response.status_code == 200:
print('Success:', response.json())
else:
print('Error:', response.status_code, response.text)
四、解析响应数据
解析响应数据是获取物联网设备信息的重要步骤。通常,响应数据是JSON格式。可以使用Python的内置库json来解析响应数据。
import json
data = response.json()
device_status = data['status']
print('Device Status:', device_status)
五、其他常用方法
使用MQTT协议
MQTT是一种轻量级的消息传输协议,非常适合物联网设备之间的通信。可以使用paho-mqtt库在Python中实现MQTT通信。
import paho.mqtt.client as mqtt
def on_connect(client, userdata, flags, rc):
print('Connected with result code ' + str(rc))
client.subscribe('topic/test')
def on_message(client, userdata, msg):
print(msg.topic + ' ' + str(msg.payload))
client = mqtt.Client()
client.on_connect = on_connect
client.on_message = on_message
client.connect('mqtt.example.com', 1883, 60)
client.loop_forever()
使用WebSocket协议
WebSocket是一种全双工通信协议,适用于实时通信。可以使用websocket-client库在Python中实现WebSocket通信。
import websocket
def on_message(ws, message):
print('Received:', message)
def on_error(ws, error):
print('Error:', error)
def on_close(ws):
print('Connection closed')
def on_open(ws):
ws.send('Hello, WebSocket!')
ws = websocket.WebSocketApp('ws://example.com/websocket',
on_message=on_message,
on_error=on_error,
on_close=on_close)
ws.on_open = on_open
ws.run_forever()
六、物联网接口调用实例
为了更好地理解如何调用物联网接口,下面是一个完整的示例,演示如何使用HTTP请求调用一个物联网设备接口并获取设备状态。
假设我们有一个温度传感器设备,提供一个HTTP接口用于获取设备状态。接口文档如下:
- URL:
http://example.com/api/temperature
- Method: GET
- Headers:
Content-Type: application/json
- Response:
{
"status": "ok",
"temperature": 22.5
}
示例代码如下:
import requests
url = 'http://example.com/api/temperature'
headers = {
'Content-Type': 'application/json'
}
response = requests.get(url, headers=headers)
if response.status_code == 200:
data = response.json()
status = data['status']
temperature = data['temperature']
print('Status:', status)
print('Temperature:', temperature)
else:
print('Error:', response.status_code, response.text)
七、处理错误和异常
在实际应用中,调用物联网接口时可能会遇到各种错误和异常。例如网络错误、服务器错误、数据解析错误等。需要在代码中加入相应的错误处理机制,以确保程序的稳定性。
import requests
url = 'http://example.com/api/temperature'
headers = {
'Content-Type': 'application/json'
}
try:
response = requests.get(url, headers=headers)
response.raise_for_status() # 检查HTTP请求是否成功
data = response.json()
status = data['status']
temperature = data['temperature']
print('Status:', status)
print('Temperature:', temperature)
except requests.exceptions.RequestException as e:
print('Request error:', e)
except ValueError as e:
print('JSON parse error:', e)
八、身份验证与安全
在调用物联网接口时,通常需要进行身份验证和安全防护。常见的身份验证方式包括API Key、OAuth等。需要根据接口文档的要求,添加相应的身份验证信息。
import requests
url = 'http://example.com/api/temperature'
headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer YOUR_ACCESS_TOKEN'
}
try:
response = requests.get(url, headers=headers)
response.raise_for_status()
data = response.json()
status = data['status']
temperature = data['temperature']
print('Status:', status)
print('Temperature:', temperature)
except requests.exceptions.RequestException as e:
print('Request error:', e)
except ValueError as e:
print('JSON parse error:', e)
九、定时任务与数据采集
在物联网应用中,常常需要定时采集设备数据。可以使用Python的schedule库来实现定时任务。
import requests
import schedule
import time
def fetch_temperature():
url = 'http://example.com/api/temperature'
headers = {
'Content-Type': 'application/json'
}
try:
response = requests.get(url, headers=headers)
response.raise_for_status()
data = response.json()
status = data['status']
temperature = data['temperature']
print('Status:', status)
print('Temperature:', temperature)
except requests.exceptions.RequestException as e:
print('Request error:', e)
except ValueError as e:
print('JSON parse error:', e)
定时每分钟执行一次
schedule.every(1).minutes.do(fetch_temperature)
while True:
schedule.run_pending()
time.sleep(1)
十、数据存储与分析
采集到的物联网数据通常需要存储和分析。可以使用数据库(如MySQL、MongoDB)来存储数据,使用数据分析工具(如Pandas)来处理和分析数据。
import requests
import pymysql
import schedule
import time
import pandas as pd
def fetch_temperature():
url = 'http://example.com/api/temperature'
headers = {
'Content-Type': 'application/json'
}
try:
response = requests.get(url, headers=headers)
response.raise_for_status()
data = response.json()
status = data['status']
temperature = data['temperature']
print('Status:', status)
print('Temperature:', temperature)
save_to_database(temperature)
except requests.exceptions.RequestException as e:
print('Request error:', e)
except ValueError as e:
print('JSON parse error:', e)
def save_to_database(temperature):
connection = pymysql.connect(host='localhost',
user='user',
password='password',
database='iot_data')
cursor = connection.cursor()
sql = 'INSERT INTO temperature_data (temperature) VALUES (%s)'
cursor.execute(sql, (temperature,))
connection.commit()
cursor.close()
connection.close()
定时每分钟执行一次
schedule.every(1).minutes.do(fetch_temperature)
while True:
schedule.run_pending()
time.sleep(1)
数据分析示例
connection = pymysql.connect(host='localhost',
user='user',
password='password',
database='iot_data')
df = pd.read_sql('SELECT * FROM temperature_data', connection)
print(df.describe())
connection.close()
十一、总结
通过上述步骤,可以使用Python方便地调用物联网接口,与物联网设备进行通信。主要方法包括使用HTTP请求、MQTT协议和WebSocket协议。结合错误处理、身份验证、定时任务、数据存储与分析等技术,可以构建一个完整的物联网数据采集与处理系统。在实际应用中,需要根据具体需求选择合适的技术方案,并进行相应的优化和调整。
相关问答FAQs:
如何使用Python连接物联网设备?
要连接物联网设备,首先需要确保设备支持相应的通信协议(如MQTT、HTTP或CoAP)。使用Python的第三方库,如paho-mqtt(用于MQTT)或requests(用于HTTP),可以轻松地发送和接收数据。具体步骤包括安装必要的库、设置连接参数(如IP地址和端口),然后使用相应的函数进行连接和数据交互。
在Python中如何处理物联网接口返回的数据?
处理物联网接口返回的数据通常涉及解析JSON或XML格式。Python的内置json库和xml.etree.ElementTree库可以用来解析这些数据格式。解析后,可以提取所需的信息并进一步处理,例如存储到数据库或进行实时监控。
使用Python调用物联网接口时如何处理错误和异常?
在调用物联网接口时,网络问题、超时或数据格式不正确等错误可能会发生。使用Python的异常处理机制(try-except语句)可以捕捉和处理这些异常。建议在代码中添加适当的日志记录,以便追踪和分析问题的根源,并确保程序的稳定性。
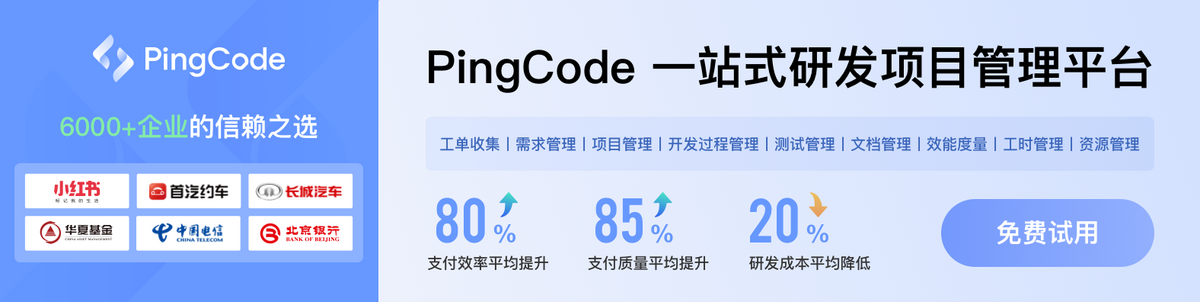