利用Python获取文件路径的方法有多种,包括os模块、pathlib模块、tkinter模块等。其中,os模块和pathlib模块是最常用的方法。
一、os模块
os模块提供了多种操作系统相关功能,包括处理文件和目录路径。
获取当前工作目录
import os
current_directory = os.getcwd()
print("当前工作目录为:", current_directory)
os.getcwd()
函数用于获取当前工作目录的路径。
获取文件的绝对路径
import os
file_name = 'example.txt'
absolute_path = os.path.abspath(file_name)
print("文件的绝对路径为:", absolute_path)
os.path.abspath()
函数用于获取文件的绝对路径。
获取文件的目录名
import os
file_path = '/path/to/example.txt'
directory_name = os.path.dirname(file_path)
print("文件的目录名为:", directory_name)
os.path.dirname()
函数用于获取文件路径的目录部分。
组合路径
import os
directory = '/path/to'
file_name = 'example.txt'
full_path = os.path.join(directory, file_name)
print("组合路径为:", full_path)
os.path.join()
函数用于将目录和文件名组合成一个路径。
二、pathlib模块
pathlib模块是Python 3.4引入的,用于处理文件系统路径的面向对象模块。
获取当前工作目录
from pathlib import Path
current_directory = Path.cwd()
print("当前工作目录为:", current_directory)
Path.cwd()
方法用于获取当前工作目录的路径。
获取文件的绝对路径
from pathlib import Path
file_name = 'example.txt'
absolute_path = Path(file_name).resolve()
print("文件的绝对路径为:", absolute_path)
Path(file_name).resolve()
方法用于获取文件的绝对路径。
获取文件的目录名
from pathlib import Path
file_path = '/path/to/example.txt'
directory_name = Path(file_path).parent
print("文件的目录名为:", directory_name)
Path(file_path).parent
属性用于获取文件路径的目录部分。
组合路径
from pathlib import Path
directory = Path('/path/to')
file_name = 'example.txt'
full_path = directory / file_name
print("组合路径为:", full_path)
Path
对象可以通过/
运算符来组合路径。
三、tkinter模块
tkinter模块可以用来创建GUI应用程序,其中的文件对话框可以用来选择文件并获取路径。
打开文件选择对话框
import tkinter as tk
from tkinter import filedialog
root = tk.Tk()
root.withdraw() # 隐藏主窗口
file_path = filedialog.askopenfilename()
print("选择的文件路径为:", file_path)
filedialog.askopenfilename()
函数会打开一个文件选择对话框,并返回所选文件的路径。
打开目录选择对话框
import tkinter as tk
from tkinter import filedialog
root = tk.Tk()
root.withdraw() # 隐藏主窗口
directory_path = filedialog.askdirectory()
print("选择的目录路径为:", directory_path)
filedialog.askdirectory()
函数会打开一个目录选择对话框,并返回所选目录的路径。
四、其他方法
除了以上常用的模块,还可以使用其他方法获取文件路径。
sys模块
import sys
script_path = sys.argv[0]
print("脚本的路径为:", script_path)
sys.argv[0]
返回脚本自身的路径。
获取脚本所在目录
import os
script_directory = os.path.dirname(os.path.realpath(__file__))
print("脚本所在目录为:", script_directory)
os.path.realpath(__file__)
返回脚本的绝对路径,os.path.dirname()
返回其目录部分。
五、总结
利用Python获取文件路径的方法有很多,可以根据具体需求选择合适的方法。os模块提供了丰富的路径处理功能,pathlib模块提供了面向对象的路径处理方式,tkinter模块可以用来创建文件选择对话框。在实际开发中,熟练掌握这些方法可以大大提高代码的健壮性和可维护性。
相关问答FAQs:
如何在Python中获取当前工作目录的文件路径?
在Python中,可以使用os
模块来获取当前工作目录的文件路径。首先,导入os
模块,然后使用os.getcwd()
函数,这个函数会返回当前工作目录的路径。例如:
import os
current_directory = os.getcwd()
print(current_directory)
这样就可以获取到当前工作目录的完整路径。
在Python中如何获取特定文件的绝对路径?
要获取特定文件的绝对路径,可以使用os.path.abspath()
函数。只需将文件名作为参数传入,该函数将返回文件的绝对路径。例如:
import os
file_name = 'example.txt'
absolute_path = os.path.abspath(file_name)
print(absolute_path)
此代码将输出example.txt
文件的绝对路径,无论该文件位于哪个目录中。
如何在Python中获取文件的父目录路径?
可以使用os.path.dirname()
函数来获取文件的父目录路径。这个函数接受文件的路径作为参数,并返回该路径的父目录。例如:
import os
file_path = '/path/to/example.txt'
parent_directory = os.path.dirname(file_path)
print(parent_directory)
这个方法将输出example.txt
文件的父目录路径,帮助用户轻松查找文件所在的文件夹。
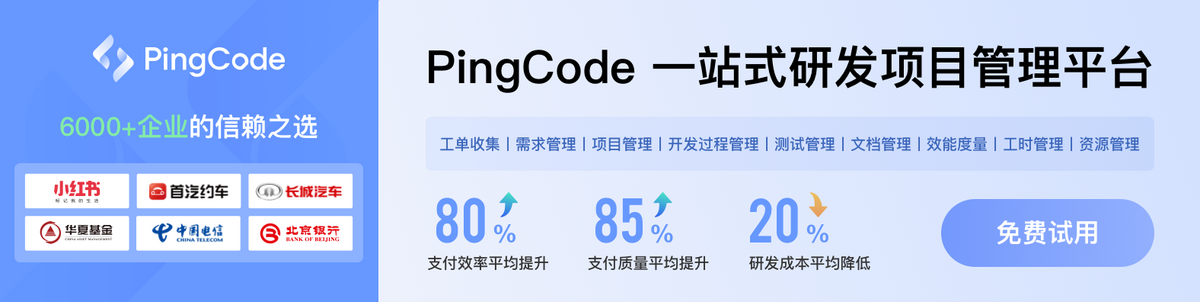