Python连接期货自动交易的方式有多种,包括使用API、通过第三方库、利用自动化平台等。本文将详细介绍其中一种方式,即如何使用API进行期货自动交易。
要使用API进行期货自动交易,首先需要选择一个支持API的交易平台。许多知名的期货交易平台(如Interactive Brokers、Tradovate、CQG等)都提供API接口。下面以Interactive Brokers为例,详细讲解如何使用Python连接期货交易API。
一、获取API密钥和认证信息
首先,你需要在交易平台上注册并获取API密钥和认证信息。通常,这包括API密钥、API密钥ID和密钥密码等。具体的获取方法可以参考交易平台的文档或客服支持。
二、安装必要的Python库
为了连接API并进行自动交易,你需要安装一些Python库。以下是常用的库及其安装方法:
pip install ibapi
pip install pandas
pip install numpy
pip install requests
三、建立与API的连接
使用Interactive Brokers的API,需要通过ibapi库进行连接。以下是一个简单的连接示例代码:
from ibapi.client import EClient
from ibapi.wrapper import EWrapper
from ibapi.contract import Contract
class IBApi(EWrapper, EClient):
def __init__(self):
EClient.__init__(self, self)
def main():
app = IBApi()
app.connect("127.0.0.1", 7497, clientId=1)
app.run()
if __name__ == "__main__":
main()
四、创建期货合约对象
为了进行期货交易,你需要创建一个期货合约对象。以下是创建期货合约对象的示例代码:
def create_contract(symbol, secType, exchange, currency, lastTradeDateOrContractMonth):
contract = Contract()
contract.symbol = symbol
contract.secType = secType
contract.exchange = exchange
contract.currency = currency
contract.lastTradeDateOrContractMonth = lastTradeDateOrContractMonth
return contract
contract = create_contract("ES", "FUT", "GLOBEX", "USD", "202112")
五、下单交易
一旦创建了期货合约对象,就可以下单进行交易。以下是下单交易的示例代码:
from ibapi.order import Order
def create_order(action, quantity, orderType, price=None):
order = Order()
order.action = action
order.totalQuantity = quantity
order.orderType = orderType
if price is not None:
order.lmtPrice = price
return order
order = create_order("BUY", 1, "LMT", 4500)
app.placeOrder(app.nextOrderId(), contract, order)
六、处理交易回报
为了处理交易回报和更新,你需要实现回调函数。以下是处理交易回报的示例代码:
class IBApi(EWrapper, EClient):
def __init__(self):
EClient.__init__(self, self)
self.nextOrderId = 0
def nextValidId(self, orderId):
self.nextOrderId = orderId
print(f"The next valid order id is: {orderId}")
def orderStatus(self, orderId, status, filled, remaining, avgFillPrice, permId, parentId, lastFillPrice, clientId, whyHeld, mktCapPrice):
print(f"Order Status - Id: {orderId}, Status: {status}, Filled: {filled}, Remaining: {remaining}, AvgFillPrice: {avgFillPrice}")
def openOrder(self, orderId, contract, order, orderState):
print(f"Open Order - Id: {orderId}, Contract: {contract.symbol}, OrderType: {order.orderType}, Status: {orderState.status}")
def execDetails(self, reqId, contract, execution):
print(f"Exec Details - ReqId: {reqId}, Contract: {contract.symbol}, ExecutionId: {execution.execId}, Shares: {execution.shares}, Price: {execution.price}")
if __name__ == "__main__":
main()
七、数据处理和分析
在实际交易中,数据处理和分析是非常重要的。你可以使用pandas和numpy库来处理和分析交易数据。例如:
import pandas as pd
import numpy as np
创建示例数据
data = {
'Date': ['2021-01-01', '2021-01-02', '2021-01-03'],
'Open': [100, 102, 104],
'High': [105, 107, 109],
'Low': [99, 101, 103],
'Close': [104, 106, 108],
'Volume': [1000, 1500, 2000]
}
转换为DataFrame
df = pd.DataFrame(data)
计算简单移动平均线
df['SMA'] = df['Close'].rolling(window=2).mean()
打印结果
print(df)
八、风险管理和策略优化
在进行自动交易时,风险管理和策略优化是关键。你需要设定止损和止盈点,并不断优化交易策略。例如:
def risk_management(close_price, stop_loss, take_profit):
if close_price <= stop_loss:
return "SELL"
elif close_price >= take_profit:
return "SELL"
else:
return "HOLD"
示例数据
close_price = 105
stop_loss = 100
take_profit = 110
风险管理
action = risk_management(close_price, stop_loss, take_profit)
print(f"Action: {action}")
九、测试和回测
在正式运行自动交易程序之前,进行测试和回测是必要的。你可以使用历史数据进行回测,以验证交易策略的有效性。例如:
import backtrader as bt
class TestStrategy(bt.Strategy):
def __init__(self):
self.sma = bt.indicators.SimpleMovingAverage(self.data.close, period=15)
def next(self):
if self.sma > self.data.close:
self.buy()
elif self.sma < self.data.close:
self.sell()
创建数据源
data = bt.feeds.YahooFinanceData(dataname='AAPL', fromdate=datetime(2020, 1, 1), todate=datetime(2021, 1, 1))
创建回测系统
cerebro = bt.Cerebro()
cerebro.addstrategy(TestStrategy)
cerebro.adddata(data)
cerebro.run()
cerebro.plot()
十、实际运行和监控
在完成所有准备工作后,可以实际运行自动交易程序并进行监控。你可以使用日志和报警系统来监控交易状态。例如:
import logging
配置日志
logging.basicConfig(filename='trading.log', level=logging.INFO)
示例交易函数
def trade():
logging.info("Trade executed")
运行交易
trade()
总结
通过以上步骤,你可以使用Python连接期货交易API并进行自动交易。需要注意的是,自动交易涉及到大量的技术细节和风险管理,建议在实际运行前进行充分的测试和回测。此外,保持代码的简洁和可维护性也是非常重要的。希望本文对你有所帮助,祝你交易顺利!
相关问答FAQs:
如何使用Python实现期货自动交易?
要使用Python进行期货自动交易,您需要选择一个合适的交易平台,确保该平台提供API接口。通常情况下,您可以使用如Interactive Brokers、Binance Futures等支持Python的交易所。此外,安装相关的Python库(如ccxt、ib_insync等)可以简化API的调用过程。编写交易策略后,确保进行充分的回测和模拟交易,确保策略的有效性和稳定性。
在期货自动交易中,如何管理风险?
风险管理在期货自动交易中至关重要。您可以通过设置止损单、合理配置仓位和分散投资来有效控制风险。此外,利用技术指标进行风险评估和交易信号生成也是一个不错的选择。定期回顾和调整您的交易策略,以适应市场变化,能够进一步降低潜在的风险。
Python自动交易的性能如何优化?
为了优化Python自动交易的性能,您可以考虑以下几个方面:使用高效的数据结构和算法,减少不必要的计算和数据处理;通过多线程或异步编程提高程序的并发能力;定期更新和优化您的交易策略,确保其在不同市场条件下的适应性。同时,监控系统性能指标,及时发现和解决瓶颈问题。
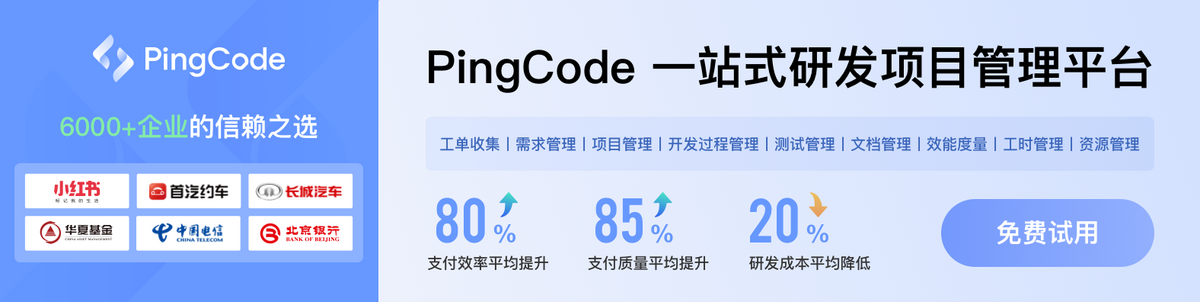