Python下载网站文件的方法有很多,包括使用标准库中的urllib
、第三方库requests
以及结合BeautifulSoup
进行网页解析等。常见的方法有:使用requests
库、使用urllib
库、结合BeautifulSoup
进行网页解析。
使用requests
库
requests
库是Python中一个非常流行的HTTP库,它使HTTP请求变得非常简单。我们可以使用requests
库来发送HTTP请求,获取网站的响应内容,并将其保存为文件。以下是一个详细的示例:
import requests
def download_file(url, file_path):
response = requests.get(url)
response.raise_for_status() # 如果请求不成功,抛出异常
with open(file_path, 'wb') as file:
file.write(response.content)
url = 'https://example.com/somefile.zip'
file_path = 'somefile.zip'
download_file(url, file_path)
在这个示例中,我们定义了一个函数download_file
,它接受文件的URL和保存路径作为参数。然后使用requests.get
方法发送GET请求,获取响应内容,并将其写入到指定的文件中。
使用urllib
库
urllib
是Python标准库中的模块,它提供了一些用于处理URL和HTTP请求的方法。以下是一个使用urllib
库下载文件的示例:
import urllib.request
def download_file(url, file_path):
urllib.request.urlretrieve(url, file_path)
url = 'https://example.com/somefile.zip'
file_path = 'somefile.zip'
download_file(url, file_path)
在这个示例中,我们使用urllib.request.urlretrieve
方法直接下载文件并将其保存到指定路径。这个方法非常简单,但在处理复杂的HTTP请求时可能不如requests
库灵活。
结合BeautifulSoup
进行网页解析
如果你需要从网页中提取文件链接并下载文件,可以使用BeautifulSoup
库进行网页解析。以下是一个示例:
import requests
from bs4 import BeautifulSoup
def download_files_from_webpage(url, file_extension, download_folder):
response = requests.get(url)
response.raise_for_status()
soup = BeautifulSoup(response.text, 'html.parser')
for link in soup.find_all('a', href=True):
if link['href'].endswith(file_extension):
file_url = link['href']
if not file_url.startswith('http'):
file_url = url + file_url
file_name = file_url.split('/')[-1]
file_path = f'{download_folder}/{file_name}'
download_file(file_url, file_path)
def download_file(url, file_path):
response = requests.get(url)
response.raise_for_status()
with open(file_path, 'wb') as file:
file.write(response.content)
url = 'https://example.com/page_with_files'
file_extension = '.zip'
download_folder = 'downloads'
download_files_from_webpage(url, file_extension, download_folder)
在这个示例中,我们定义了一个函数download_files_from_webpage
,它接受网页URL、文件扩展名和下载文件夹作为参数。首先,我们使用requests
库获取网页内容,然后使用BeautifulSoup
解析网页,并找到所有的文件链接。最后,使用之前定义的download_file
函数下载文件。
处理大文件下载
在处理大文件下载时,我们需要逐块读取文件内容并写入到本地文件中,以避免内存溢出。以下是一个处理大文件下载的示例:
import requests
def download_large_file(url, file_path, chunk_size=1024):
response = requests.get(url, stream=True)
response.raise_for_status()
with open(file_path, 'wb') as file:
for chunk in response.iter_content(chunk_size=chunk_size):
if chunk:
file.write(chunk)
url = 'https://example.com/largefile.zip'
file_path = 'largefile.zip'
download_large_file(url, file_path)
在这个示例中,我们使用requests.get
方法时传递参数stream=True
,使得响应内容逐块读取。然后使用iter_content
方法逐块读取文件内容,并将其写入到本地文件中。
处理身份验证和会话
有些网站需要用户身份验证才能下载文件。在这种情况下,我们可以使用requests
库的会话对象来处理身份验证。以下是一个处理身份验证的示例:
import requests
def download_file_with_auth(url, file_path, username, password):
session = requests.Session()
session.auth = (username, password)
response = session.get(url)
response.raise_for_status()
with open(file_path, 'wb') as file:
file.write(response.content)
url = 'https://example.com/protectedfile.zip'
file_path = 'protectedfile.zip'
username = 'your_username'
password = 'your_password'
download_file_with_auth(url, file_path, username, password)
在这个示例中,我们创建了一个会话对象,并设置身份验证信息。然后使用会话对象发送GET请求,获取文件内容并将其保存到本地文件中。
处理文件重定向
有些文件下载链接会进行重定向。在这种情况下,我们需要处理重定向并获取最终的文件URL。以下是一个处理文件重定向的示例:
import requests
def download_file_with_redirect(url, file_path):
session = requests.Session()
response = session.head(url, allow_redirects=True)
final_url = response.url
response = session.get(final_url)
response.raise_for_status()
with open(file_path, 'wb') as file:
file.write(response.content)
url = 'https://example.com/redirectedfile.zip'
file_path = 'redirectedfile.zip'
download_file_with_redirect(url, file_path)
在这个示例中,我们首先使用session.head
方法发送HEAD请求,允许重定向以获取最终的文件URL。然后使用最终的URL发送GET请求,获取文件内容并将其保存到本地文件中。
处理不同类型的文件
不同类型的文件可能需要不同的处理方式。例如,下载和保存图像文件、PDF文件或CSV文件等。以下是一些处理不同类型文件的示例:
下载和保存图像文件
import requests
def download_image(url, file_path):
response = requests.get(url)
response.raise_for_status()
with open(file_path, 'wb') as file:
file.write(response.content)
url = 'https://example.com/image.jpg'
file_path = 'image.jpg'
download_image(url, file_path)
下载和保存PDF文件
import requests
def download_pdf(url, file_path):
response = requests.get(url)
response.raise_for_status()
with open(file_path, 'wb') as file:
file.write(response.content)
url = 'https://example.com/document.pdf'
file_path = 'document.pdf'
download_pdf(url, file_path)
下载和保存CSV文件
import requests
def download_csv(url, file_path):
response = requests.get(url)
response.raise_for_status()
with open(file_path, 'wb') as file:
file.write(response.content)
url = 'https://example.com/data.csv'
file_path = 'data.csv'
download_csv(url, file_path)
处理异常和错误
在下载文件时,可能会遇到各种异常和错误。例如,网络连接问题、文件不存在、权限不足等。我们需要处理这些异常,以确保程序的健壮性。以下是一些处理异常和错误的示例:
import requests
def download_file(url, file_path):
try:
response = requests.get(url)
response.raise_for_status()
except requests.exceptions.RequestException as e:
print(f"Error downloading file: {e}")
return
try:
with open(file_path, 'wb') as file:
file.write(response.content)
except IOError as e:
print(f"Error saving file: {e}")
url = 'https://example.com/somefile.zip'
file_path = 'somefile.zip'
download_file(url, file_path)
在这个示例中,我们使用try-except
块捕获并处理异常。如果下载文件或保存文件时出现异常,我们将打印错误信息并退出函数。
多线程下载文件
对于需要下载多个文件的情况,可以使用多线程来提高下载效率。以下是一个多线程下载文件的示例:
import requests
import threading
def download_file(url, file_path):
response = requests.get(url)
response.raise_for_status()
with open(file_path, 'wb') as file:
file.write(response.content)
def download_files(urls, download_folder):
threads = []
for url in urls:
file_name = url.split('/')[-1]
file_path = f'{download_folder}/{file_name}'
thread = threading.Thread(target=download_file, args=(url, file_path))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
urls = [
'https://example.com/file1.zip',
'https://example.com/file2.zip',
'https://example.com/file3.zip'
]
download_folder = 'downloads'
download_files(urls, download_folder)
在这个示例中,我们使用threading
模块创建多个线程,每个线程负责下载一个文件。我们将所有线程存储在一个列表中,并使用thread.join()
方法等待所有线程完成下载。
使用进度条显示下载进度
为了提高用户体验,可以在下载文件时显示下载进度。以下是一个使用tqdm
库显示下载进度的示例:
import requests
from tqdm import tqdm
def download_file_with_progress(url, file_path):
response = requests.get(url, stream=True)
response.raise_for_status()
total_size = int(response.headers.get('content-length', 0))
with open(file_path, 'wb') as file, tqdm(
desc=file_path,
total=total_size,
unit='iB',
unit_scale=True,
unit_divisor=1024,
) as bar:
for chunk in response.iter_content(chunk_size=1024):
size = file.write(chunk)
bar.update(size)
url = 'https://example.com/largefile.zip'
file_path = 'largefile.zip'
download_file_with_progress(url, file_path)
在这个示例中,我们使用tqdm
库显示下载进度条。我们逐块读取文件内容,并更新进度条的进度。
总结
Python提供了多种下载网站文件的方法,包括使用requests
库、urllib
库以及结合BeautifulSoup
进行网页解析等。我们可以根据具体需求选择合适的方法,并处理大文件下载、身份验证、文件重定向、不同类型文件、异常和错误、多线程下载以及显示下载进度等情况。
通过上述示例,我们可以掌握如何使用Python下载网站文件,并根据具体需求进行灵活处理。希望这些内容对你有所帮助,能够让你在实际项目中更加得心应手。
相关问答FAQs:
如何使用Python下载特定类型的文件?
使用Python下载特定类型的文件可以通过requests
库实现。首先,确保安装了该库。然后,使用requests.get()
方法请求文件的URL,并将返回的内容写入本地文件。示例代码如下:
import requests
url = 'http://example.com/file.pdf' # 替换为目标文件的URL
response = requests.get(url)
with open('file.pdf', 'wb') as file:
file.write(response.content)
这样就可以下载PDF文件,其他类型的文件也可以用类似的方式处理。
在下载过程中如何处理错误?
在下载文件时,可能会遇到网络问题或文件不存在等错误。可以通过检查响应的状态码来处理这些情况。使用response.raise_for_status()
方法可以确保在请求失败时抛出异常。此外,可以使用try...except
结构捕获异常并进行相应处理。示例代码如下:
try:
response = requests.get(url)
response.raise_for_status() # 检查请求是否成功
except requests.exceptions.HTTPError as err:
print(f"HTTP错误: {err}")
except requests.exceptions.RequestException as e:
print(f"请求错误: {e}")
这样可以更稳健地处理下载过程中的问题。
如何下载整个网站的文件?
要下载整个网站的文件,可以使用wget
或HTTrack
等工具,Python也可以通过爬虫库如BeautifulSoup
和Scrapy
实现。使用这些工具可以遍历网页,提取文件链接并逐个下载。以下是一个简单的示例,展示如何使用BeautifulSoup
和requests
下载网页中的所有PDF文件:
from bs4 import BeautifulSoup
import requests
import os
url = 'http://example.com' # 替换为目标网站的URL
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
for link in soup.find_all('a'):
file_url = link.get('href')
if file_url.endswith('.pdf'):
file_response = requests.get(file_url)
file_name = os.path.join('downloads', file_url.split('/')[-1]) # 指定下载目录
with open(file_name, 'wb') as file:
file.write(file_response.content)
确保在运行代码前创建了下载目录。通过这些方法,可以有效下载整个网站上的文件。
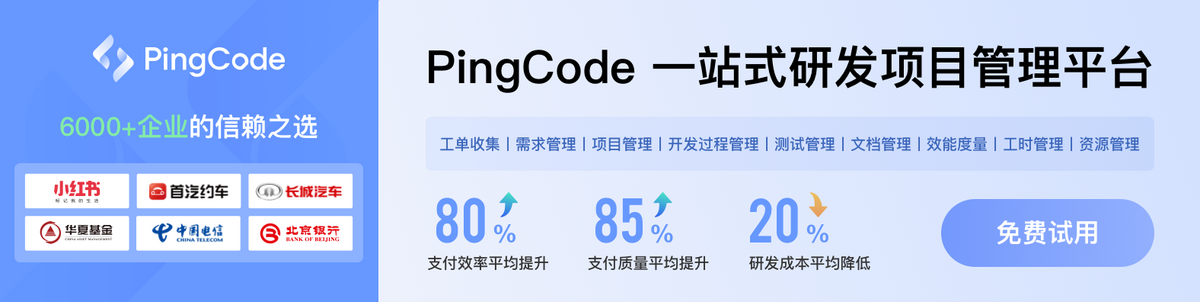