Python中可以使用type()函数、isinstance()函数、以及__class__属性来查询某个变量的类型。其中,type()函数是最常用的方法,它直接返回变量的类型,可以用于简单的类型检查。isinstance()函数不仅能够检查变量类型,还可以检查变量是否是某个类的实例。__class__属性是每个对象的内置属性,它指向该对象的类。
下面我将详细介绍这三种方法,帮助您更好地理解和使用它们。
一、type()函数
type()函数是Python内置的一个函数,它可以返回对象的类型。使用方法非常简单,直接将变量作为参数传递给type()函数即可。例如:
a = 10
print(type(a)) # <class 'int'>
b = 3.14
print(type(b)) # <class 'float'>
c = "Hello, World!"
print(type(c)) # <class 'str'>
从上面的例子中可以看出,type()函数直接返回变量的类型,比如整数类型、浮点数类型、字符串类型等。这种方法简单直观,适合用于快速的类型检查。
二、isinstance()函数
isinstance()函数不仅可以检查变量的类型,还可以检查变量是否是某个类的实例。它的使用方法是将变量和类型作为参数传递给isinstance()函数,返回值是一个布尔值,表示变量是否是该类型或该类型的子类。例如:
a = 10
print(isinstance(a, int)) # True
b = 3.14
print(isinstance(b, float)) # True
c = "Hello, World!"
print(isinstance(c, str)) # True
class MyClass:
pass
d = MyClass()
print(isinstance(d, MyClass)) # True
从上面的例子中可以看出,isinstance()函数不仅可以检查基本数据类型,还可以检查自定义类的实例。这种方法更加灵活,适用于多态检查和复杂类型判断。
三、__class__属性
__class__属性是每个对象的内置属性,它指向该对象的类。通过访问__class__属性,可以获取对象的类型。例如:
a = 10
print(a.__class__) # <class 'int'>
b = 3.14
print(b.__class__) # <class 'float'>
c = "Hello, World!"
print(c.__class__) # <class 'str'>
从上面的例子中可以看出,__class__属性与type()函数的结果类似,都是返回对象的类型。这种方法适用于需要访问对象的类信息的场景。
四、比较三种方法的适用场景
虽然type()函数、isinstance()函数和__class__属性都可以用来查询变量的类型,但它们在实际应用中各有适用场景。
- type()函数适用于简单直观的类型检查,适合用于对变量类型的快速判断。
- isinstance()函数适用于多态检查和复杂类型判断,特别是在面向对象编程中,可以检查变量是否是某个类的实例或其子类的实例。
- __class__属性适用于需要访问对象的类信息的场景,特别是在反射和动态类型检查中。
五、总结
总的来说,Python提供了多种方法来查询变量的类型,每种方法都有其适用的场景。type()函数简单直观,适合快速判断类型;isinstance()函数灵活多样,适合复杂类型判断;__class__属性适用于需要访问对象类信息的场景。根据实际需求选择合适的方法,能够提高代码的可读性和可维护性。希望本文对您理解Python中如何查询变量类型有所帮助。
六、示例代码
为了更好地理解上述内容,这里提供一些示例代码,展示如何在不同场景下使用type()函数、isinstance()函数和__class__属性。
示例一:使用type()函数
def check_type(variable):
var_type = type(variable)
print(f"The type of the variable is {var_type}")
Test cases
check_type(100) # The type of the variable is <class 'int'>
check_type(3.14) # The type of the variable is <class 'float'>
check_type("Hello") # The type of the variable is <class 'str'>
check_type([1, 2, 3]) # The type of the variable is <class 'list'>
check_type({"a": 1}) # The type of the variable is <class 'dict'>
示例二:使用isinstance()函数
def is_instance_of(variable, var_type):
result = isinstance(variable, var_type)
print(f"Is the variable an instance of {var_type}? {result}")
Test cases
is_instance_of(100, int) # Is the variable an instance of <class 'int'>? True
is_instance_of(3.14, float) # Is the variable an instance of <class 'float'>? True
is_instance_of("Hello", str) # Is the variable an instance of <class 'str'>? True
is_instance_of([1, 2, 3], list) # Is the variable an instance of <class 'list'>? True
is_instance_of({"a": 1}, dict) # Is the variable an instance of <class 'dict'>? True
is_instance_of(100, float) # Is the variable an instance of <class 'float'>? False
示例三:使用__class__属性
def get_class(variable):
var_class = variable.__class__
print(f"The class of the variable is {var_class}")
Test cases
get_class(100) # The class of the variable is <class 'int'>
get_class(3.14) # The class of the variable is <class 'float'>
get_class("Hello") # The class of the variable is <class 'str'>
get_class([1, 2, 3]) # The class of the variable is <class 'list'>
get_class({"a": 1}) # The class of the variable is <class 'dict'>
通过上述示例代码,您可以更直观地了解如何在不同场景下使用type()函数、isinstance()函数和__class__属性来查询变量的类型。
七、实践应用
在实际项目中,查询变量类型的需求非常常见,特别是在数据处理、参数校验和调试中。下面列举几个实际应用场景,展示如何在项目中使用这些方法。
应用场景一:数据处理
在数据处理过程中,往往需要根据数据类型进行不同的处理。例如,处理来自不同数据源的数据时,需要先判断数据类型,然后执行相应的处理逻辑:
def process_data(data):
if isinstance(data, list):
# 处理列表类型的数据
print("Processing list data")
elif isinstance(data, dict):
# 处理字典类型的数据
print("Processing dictionary data")
elif isinstance(data, str):
# 处理字符串类型的数据
print("Processing string data")
else:
# 处理其他类型的数据
print("Processing other types of data")
Test cases
process_data([1, 2, 3]) # Processing list data
process_data({"a": 1, "b": 2}) # Processing dictionary data
process_data("Hello, World!") # Processing string data
process_data(100) # Processing other types of data
应用场景二:参数校验
在函数调用时,通常需要对参数进行类型检查,以确保函数能够正确处理传入的参数。例如,实现一个计算两个数之和的函数,需要检查参数是否为数值类型:
def add_numbers(a, b):
if not isinstance(a, (int, float)) or not isinstance(b, (int, float)):
raise TypeError("Both arguments must be int or float")
return a + b
Test cases
print(add_numbers(10, 20)) # 30
print(add_numbers(3.14, 2.72)) # 5.86
print(add_numbers(10, "20")) # TypeError: Both arguments must be int or float
应用场景三:调试
在调试过程中,了解变量的类型有助于快速定位问题。例如,在调试复杂的数据结构时,可以通过打印变量类型来辅助分析问题:
def debug_variable(variable):
var_type = type(variable)
print(f"Variable type: {var_type}, Value: {variable}")
Test cases
debug_variable(100) # Variable type: <class 'int'>, Value: 100
debug_variable(3.14) # Variable type: <class 'float'>, Value: 3.14
debug_variable("Hello, World!") # Variable type: <class 'str'>, Value: Hello, World!
debug_variable([1, 2, 3]) # Variable type: <class 'list'>, Value: [1, 2, 3]
debug_variable({"a": 1, "b": 2}) # Variable type: <class 'dict'>, Value: {'a': 1, 'b': 2}
通过实际应用场景的示例,您可以更好地理解如何在项目中使用查询变量类型的方法,提高代码的健壮性和可维护性。
八、总结
本文详细介绍了Python中查询变量类型的三种方法:type()函数、isinstance()函数、__class__属性,并分析了它们的适用场景。通过示例代码和实际应用场景的讲解,希望能够帮助您更好地理解和使用这些方法。在实际项目中,根据需求选择合适的方法,可以提高代码的健壮性和可维护性。
查询变量类型是Python编程中的基础操作,掌握这些方法不仅能帮助您编写更健壮的代码,还能提升调试和维护代码的效率。希望本文对您有所帮助,祝您在Python编程中取得更大的进步。
相关问答FAQs:
如何在Python中查看一个变量的类型?
在Python中,可以使用内置的type()
函数来查询某个变量的类型。例如,使用type(variable_name)
就能返回该变量的类型信息。这对于调试和理解代码中的数据结构非常有帮助。
Python中是否有其他方式可以检查变量类型?
除了使用type()
函数,Python还提供了isinstance()
函数。该函数可以检查一个变量是否是特定类型的实例。例如,isinstance(variable_name, int)
可以用来判断variable_name
是否是整数类型。这种方式更为灵活,尤其在需要检查变量是否属于某个类型的子类时。
为什么了解变量类型在Python编程中很重要?
了解变量的类型在编程中至关重要,因为不同类型的数据有不同的操作和方法。通过确定变量的类型,可以避免类型错误,从而提高代码的稳定性和可读性。此外,变量类型还影响性能,合理选择数据类型能够优化程序的运行效率。
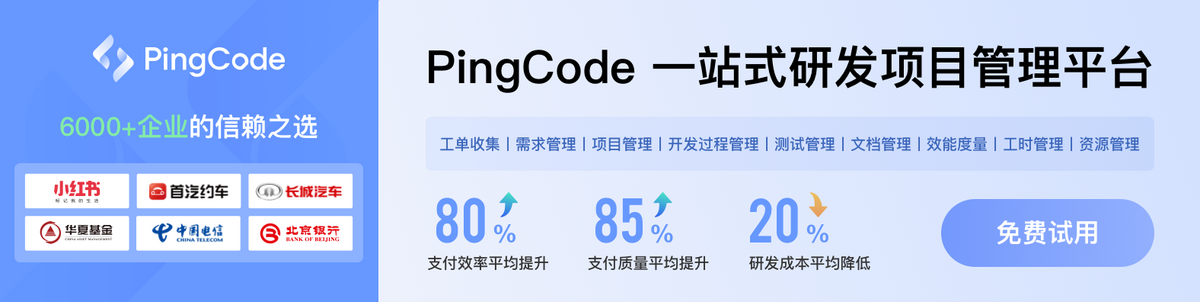