要使用Python来绘制星空,可以利用一些强大的绘图库,比如Matplotlib、Turtle和Pygame。首先,安装必需的库、理解基础图形绘制、选择合适的坐标系、使用随机生成星星的位置和大小、添加细节和效果,这些都是创建逼真星空图像的关键步骤。让我们详细展开这几个步骤中的一些:
安装必需的库:
要使用这些库,首先需要在你的Python环境中安装它们。可以使用pip工具来安装,比如:
pip install matplotlib
pip install pygame
一、理解基础图形绘制
基础绘图:
Python中有多个绘图库,但最常用的包括Matplotlib、Turtle和Pygame。这些库提供了丰富的API来绘制各种图形。理解这些API的基础用法是绘制任何图像的第一步。
1. Matplotlib
Matplotlib是一个非常强大的2D绘图库,特别适合用于创建图表和简单的图形。下面是一个使用Matplotlib绘制简单星空的例子:
import matplotlib.pyplot as plt
import numpy as np
生成随机星星的位置
num_stars = 100
x = np.random.rand(num_stars)
y = np.random.rand(num_stars)
绘制星空
plt.figure(figsize=(8, 8))
plt.scatter(x, y, s=np.random.randint(5, 20, num_stars), c='white')
plt.gca().set_facecolor('black')
plt.show()
2. Turtle
Turtle是一个非常直观的绘图库,特别适合初学者。它使用一个“乌龟”在屏幕上移动来绘制图形。下面是一个使用Turtle绘制简单星空的例子:
import turtle
import random
设置屏幕
screen = turtle.Screen()
screen.bgcolor("black")
创建乌龟
star_turtle = turtle.Turtle()
star_turtle.hideturtle()
star_turtle.speed(0)
star_turtle.color("white")
绘制星星
for _ in range(100):
x = random.randint(-300, 300)
y = random.randint(-300, 300)
star_turtle.penup()
star_turtle.goto(x, y)
star_turtle.pendown()
star_turtle.dot(random.randint(2, 6))
结束绘图
turtle.done()
3. Pygame
Pygame是一个非常强大的游戏开发库,也可以用于绘制复杂的图形。下面是一个使用Pygame绘制简单星空的例子:
import pygame
import random
初始化Pygame
pygame.init()
设置屏幕
screen = pygame.display.set_mode((800, 800))
pygame.display.set_caption("Starry Sky")
定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
绘制星空
screen.fill(BLACK)
for _ in range(100):
x = random.randint(0, 800)
y = random.randint(0, 800)
pygame.draw.circle(screen, WHITE, (x, y), random.randint(1, 3))
更新屏幕
pygame.display.flip()
等待退出
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
pygame.quit()
二、选择合适的坐标系
坐标系选择:
绘制星空时,需要选择一个适合的坐标系来生成和显示星星的位置。通常可以使用笛卡尔坐标系(x, y)来表示每颗星星的位置。可以通过随机生成坐标来模拟星空的随机性。
1. 笛卡尔坐标系
笛卡尔坐标系是最常用的二维坐标系,其中每个点有两个坐标值:x和y。这个坐标系非常适合用于绘制图形。使用Matplotlib、Turtle或Pygame时,默认都是使用笛卡尔坐标系。
2. 极坐标系
极坐标系使用一个角度和一个半径来表示点的位置。这个坐标系在某些情况下可能更直观,比如绘制同心圆或放射状图形。Matplotlib支持极坐标系,可以通过设置参数来切换:
import matplotlib.pyplot as plt
import numpy as np
生成随机星星的位置
num_stars = 100
r = np.random.rand(num_stars)
theta = 2 * np.pi * np.random.rand(num_stars)
绘制星空
plt.figure(figsize=(8, 8))
ax = plt.subplot(111, projection='polar')
ax.scatter(theta, r, s=np.random.randint(5, 20, num_stars), c='white')
ax.set_facecolor('black')
plt.show()
三、使用随机生成星星的位置和大小
随机生成:
为了使星空看起来更加自然,可以使用随机数生成器来生成星星的位置和大小。Python的random
模块提供了丰富的随机数生成函数,可以生成各种分布的随机数。
1. 随机位置
生成星星的随机位置可以使用random
模块中的randint
或random
函数。randint
生成一个指定范围内的整数,random
生成一个0到1之间的浮点数。
import random
num_stars = 100
for _ in range(num_stars):
x = random.randint(0, 800)
y = random.randint(0, 800)
print(f"Star at ({x}, {y})")
2. 随机大小
生成星星的随机大小可以使用randint
或uniform
函数。randint
生成一个指定范围内的整数,uniform
生成一个指定范围内的浮点数。
import random
num_stars = 100
for _ in range(num_stars):
size = random.randint(1, 5)
print(f"Star size: {size}")
四、添加细节和效果
细节和效果:
为了使星空更加逼真,可以添加一些细节和效果,比如星星的闪烁、颜色变化、星云和银河等。这些效果可以通过调整绘图参数和添加额外的图形元素来实现。
1. 星星闪烁
可以通过改变星星的亮度或颜色来模拟星星的闪烁效果。可以使用一个循环来不断更新星星的亮度或颜色,并重绘图形。
import matplotlib.pyplot as plt
import numpy as np
import time
生成随机星星的位置
num_stars = 100
x = np.random.rand(num_stars)
y = np.random.rand(num_stars)
绘制星空
fig, ax = plt.subplots(figsize=(8, 8))
ax.set_facecolor('black')
sc = ax.scatter(x, y, s=np.random.randint(5, 20, num_stars), c='white')
模拟星星闪烁
for _ in range(100):
sizes = np.random.randint(5, 20, num_stars)
sc.set_sizes(sizes)
plt.draw()
plt.pause(0.1)
2. 星云和银河
星云和银河可以通过绘制大量的点或使用图像来模拟。可以使用Matplotlib中的imshow
函数来显示星云或银河的图像。
import matplotlib.pyplot as plt
import numpy as np
生成随机星星的位置
num_stars = 100
x = np.random.rand(num_stars)
y = np.random.rand(num_stars)
绘制星空
plt.figure(figsize=(8, 8))
plt.scatter(x, y, s=np.random.randint(5, 20, num_stars), c='white')
添加星云
x_cloud = np.random.rand(1000)
y_cloud = np.random.rand(1000)
plt.scatter(x_cloud, y_cloud, s=1, c='gray', alpha=0.1)
plt.gca().set_facecolor('black')
plt.show()
3. 动态效果
动态效果可以通过不断更新图像来实现。可以使用Pygame的游戏循环或Matplotlib的动画功能来创建动态效果。
import pygame
import random
初始化Pygame
pygame.init()
设置屏幕
screen = pygame.display.set_mode((800, 800))
pygame.display.set_caption("Starry Sky")
定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
绘制星空
stars = []
for _ in range(100):
x = random.randint(0, 800)
y = random.randint(0, 800)
size = random.randint(1, 3)
stars.append((x, y, size))
动态效果
running = True
while running:
screen.fill(BLACK)
for star in stars:
pygame.draw.circle(screen, WHITE, (star[0], star[1]), star[2])
pygame.display.flip()
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
pygame.quit()
通过上述步骤,可以使用Python创建一个逼真的星空图像。根据具体需求和细节,可以进一步调整和优化代码。希望这个指南对你有所帮助!
相关问答FAQs:
在用Python绘制星空时,我需要哪些库?
要绘制星空,通常需要安装matplotlib
和numpy
这两个库。matplotlib
用于绘制图形,而numpy
则帮助生成随机星星的坐标。可以通过命令pip install matplotlib numpy
来安装这两个库。
在绘制星空时,如何生成星星的随机位置和数量?
可以使用numpy.random
模块生成随机数来创建星星的位置。通过numpy.random.rand()
方法,可以生成指定数量的随机坐标点,以模拟星星在天空中的分布。例如,x = np.random.rand(num_stars)
和y = np.random.rand(num_stars)
可以分别生成星星在x轴和y轴上的随机位置。
绘制星空时,如何调整星星的亮度和大小?
星星的亮度和大小可以通过scatter
函数中的参数进行调整。使用s
参数可以设置星星的大小,c
参数可以设置星星的颜色。通过给定一个数组来调整不同星星的亮度,例如可以使用np.random.rand(num_stars)
生成一个随机亮度数组,设置scatter
函数的c
参数为这个数组,就能实现星星的亮度变化。
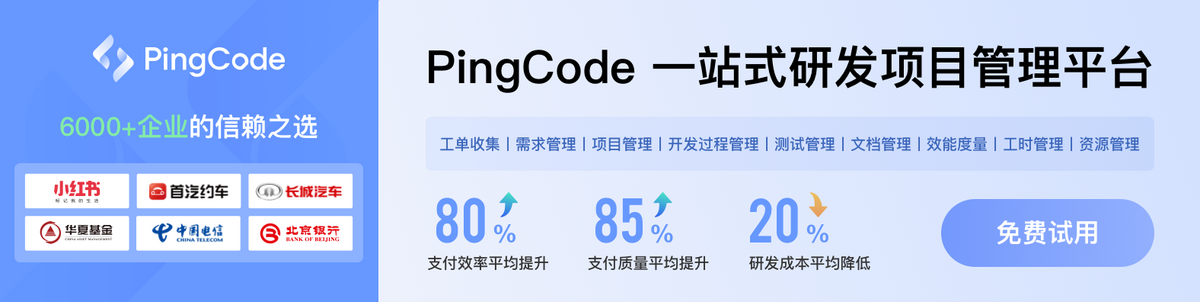