Python返回树的形状,可以通过递归遍历树、收集节点信息并构造形状描述、使用树的遍历方法、构建树的嵌套列表表示。 其中,使用递归遍历树是较为常见且有效的方法。通过递归遍历树的节点,可以收集每个节点的信息,并按照一定的规则构造树的形状描述。下面将详细描述递归遍历树的方法。
递归遍历树的方法可以分为几个步骤:
- 定义树的节点类和树的结构
- 编写递归函数来遍历树的节点
- 在递归过程中收集节点信息并构造树的形状表示
一、定义树的节点类和树的结构
首先,我们需要定义一个树的节点类,节点类中包含节点的数据以及子节点的信息。可以根据具体的需求选择不同的树结构,例如二叉树、多叉树等。
class TreeNode:
def __init__(self, value):
self.value = value
self.children = []
def add_child(self, child):
self.children.append(child)
二、编写递归函数来遍历树的节点
接下来,我们需要编写一个递归函数来遍历树的节点。在递归函数中,我们将访问每个节点并收集其子节点的信息。
def get_tree_shape(node):
if not node:
return None
shape = {"value": node.value, "children": []}
for child in node.children:
shape["children"].append(get_tree_shape(child))
return shape
三、在递归过程中收集节点信息并构造树的形状表示
在递归函数中,我们可以按照一定的规则构造树的形状表示。例如,可以使用嵌套的字典来表示树的结构,每个字典包含节点的值和子节点的信息。
def print_tree_shape(tree_shape, level=0):
if not tree_shape:
return
indent = " " * (level * 2)
print(f"{indent}{tree_shape['value']}")
for child_shape in tree_shape["children"]:
print_tree_shape(child_shape, level + 1)
创建树结构
root = TreeNode("A")
child1 = TreeNode("B")
child2 = TreeNode("C")
child3 = TreeNode("D")
root.add_child(child1)
root.add_child(child2)
child1.add_child(child3)
获取树的形状
tree_shape = get_tree_shape(root)
打印树的形状
print_tree_shape(tree_shape)
以上代码展示了如何定义树的节点类、编写递归函数来遍历树的节点,并在递归过程中收集节点信息并构造树的形状表示。通过运行代码,可以得到树的形状,并以层次结构的方式打印出来。
四、其他方法
除了递归遍历树的方法,还可以使用其他方法来返回树的形状,例如使用广度优先搜索(BFS)遍历树、构建树的嵌套列表表示等。
1. 使用广度优先搜索(BFS)遍历树
广度优先搜索是一种遍历树的算法,它按照层次从上到下依次遍历树的节点。可以使用队列来实现BFS遍历,并在遍历过程中收集节点信息。
from collections import deque
def get_tree_shape_bfs(root):
if not root:
return None
queue = deque([root])
shape = {"value": root.value, "children": []}
node_map = {root: shape}
while queue:
node = queue.popleft()
node_shape = node_map[node]
for child in node.children:
child_shape = {"value": child.value, "children": []}
node_shape["children"].append(child_shape)
node_map[child] = child_shape
queue.append(child)
return shape
使用BFS遍历树可以得到与递归遍历树相同的结果。
2. 构建树的嵌套列表表示
另一种表示树的方法是使用嵌套列表,每个列表的第一个元素是节点的值,后面的元素是子节点的嵌套列表。
def get_tree_shape_list(node):
if not node:
return None
shape = [node.value]
for child in node.children:
shape.append(get_tree_shape_list(child))
return shape
使用嵌套列表表示树的形状,可以得到类似以下的结果:
['A', ['B', ['D']], ['C']]
无论使用哪种方法,都可以有效地返回树的形状,并根据具体需求选择合适的方法。
五、总结
Python返回树的形状的方法有多种,包括递归遍历树、广度优先搜索(BFS)遍历树、构建树的嵌套列表表示等。递归遍历树的方法是较为常见且有效的方法,通过递归遍历树的节点,可以收集每个节点的信息,并按照一定的规则构造树的形状描述。在实际应用中,可以根据具体需求选择合适的方法来返回树的形状。
相关问答FAQs:
如何在Python中定义树的结构?
在Python中,树的结构通常可以通过定义一个节点类来实现。每个节点可以包含数据和指向其子节点的指针。以下是一个简单的示例:
class TreeNode:
def __init__(self, value):
self.value = value
self.children = []
def add_child(self, child_node):
self.children.append(child_node)
这种结构允许你灵活地创建和管理树的节点。
如何遍历一棵树并获取其形状?
遍历树的常见方式包括前序遍历、中序遍历和后序遍历。使用递归函数可以很方便地实现这些遍历方式。以下是一个前序遍历的示例:
def preorder_traversal(node):
if node:
print(node.value)
for child in node.children:
preorder_traversal(child)
这种方法能够帮助你按顺序访问树的每个节点,从而获取树的形状。
如何可视化树的结构?
可视化树结构可以使用图形库,如Matplotlib或Graphviz。通过绘制树的节点和连接线,可以直观展示树的形状。例如,使用Graphviz库:
from graphviz import Digraph
def visualize_tree(node, graph=None):
if graph is None:
graph = Digraph()
graph.node(str(node.value))
for child in node.children:
graph.edge(str(node.value), str(child.value))
visualize_tree(child, graph)
return graph
# 使用示例
root = TreeNode(1)
child1 = TreeNode(2)
child2 = TreeNode(3)
root.add_child(child1)
root.add_child(child2)
visualize_tree(root).render('tree', format='png')
这种方法能够将树的结构以图像的形式展示出来,方便理解和分析。
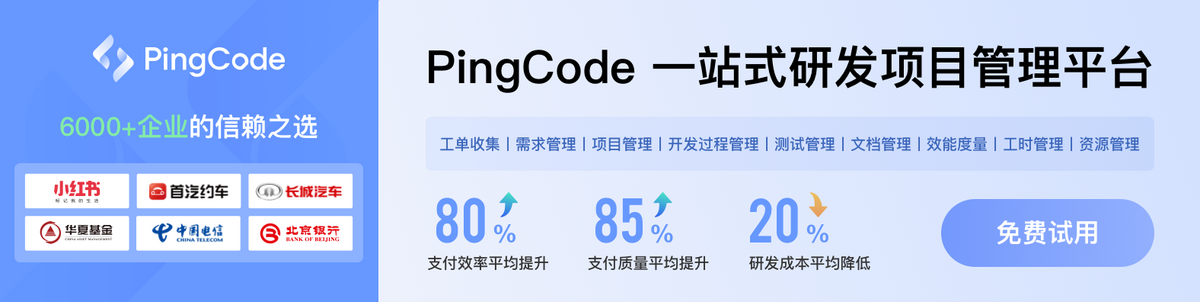