在Python中计算前10个词频的方法有很多种,你可以使用collections模块中的Counter类、使用nltk库、或者使用pandas库。其中,使用collections.Counter类是最常见和简便的方法。下面,我将详细介绍如何使用collections.Counter类来计算前10个词频,并给出具体代码示例。
使用collections模块中的Counter类
Python的collections模块提供了一个Counter类,可以用来轻松计算可哈希对象(如字符串、列表等)中元素的频率。
步骤一:读取文本数据
首先,我们需要读取文本数据。文本数据可以来自文件、网页或直接的字符串。在这个例子中,我们将从一个文本文件读取数据。
import collections
def read_text_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
return text
file_path = 'your_text_file.txt'
text = read_text_file(file_path)
步骤二:清理和分割文本
接下来,我们需要对文本进行一些基本的清理工作,比如去除标点符号和转换为小写字母,然后将文本分割成单词列表。
import re
def clean_and_split_text(text):
# 去除标点符号
text = re.sub(r'[^\w\s]', '', text)
# 转换为小写
text = text.lower()
# 分割成单词列表
words = text.split()
return words
words = clean_and_split_text(text)
步骤三:计算词频
使用collections.Counter类计算词频。
def get_word_frequencies(words):
word_counts = collections.Counter(words)
return word_counts
word_counts = get_word_frequencies(words)
步骤四:获取前10个高频词
最后,我们可以使用Counter类的most_common方法获取前10个高频词。
def get_top_n_words(word_counts, n):
top_n_words = word_counts.most_common(n)
return top_n_words
top_10_words = get_top_n_words(word_counts, 10)
print(top_10_words)
以上代码实现了从读取文本文件到计算前10个高频词的全过程。通过这些步骤,你可以轻松地计算文本数据中的高频词。下面我们将深入探讨其他计算词频的方法,以及如何进一步优化和扩展这些方法。
二、使用nltk库
NLTK(Natural Language Toolkit)是一个强大的Python库,专门用于处理和分析自然语言数据。它提供了丰富的工具和资源,可以大大简化文本处理工作。
步骤一:安装和导入nltk库
首先,你需要安装nltk库。如果你还没有安装,可以使用pip进行安装:
pip install nltk
然后在Python代码中导入nltk库。
import nltk
nltk.download('punkt')
步骤二:读取和分词
读取文本文件并使用nltk的word_tokenize函数进行分词。
def read_text_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
return text
file_path = 'your_text_file.txt'
text = read_text_file(file_path)
from nltk.tokenize import word_tokenize
words = word_tokenize(text)
步骤三:清理文本
你可以使用nltk的其他工具来进一步清理文本,比如去除停用词和标点符号。
from nltk.corpus import stopwords
nltk.download('stopwords')
stop_words = set(stopwords.words('english'))
def clean_text(words):
cleaned_words = [word.lower() for word in words if word.isalpha() and word.lower() not in stop_words]
return cleaned_words
cleaned_words = clean_text(words)
步骤四:计算词频
使用nltk的FreqDist类来计算词频。
from nltk.probability import FreqDist
def get_word_frequencies(words):
word_frequencies = FreqDist(words)
return word_frequencies
word_frequencies = get_word_frequencies(cleaned_words)
步骤五:获取前10个高频词
使用FreqDist类的most_common方法获取前10个高频词。
def get_top_n_words(word_frequencies, n):
top_n_words = word_frequencies.most_common(n)
return top_n_words
top_10_words = get_top_n_words(word_frequencies, 10)
print(top_10_words)
三、使用pandas库
Pandas是一个强大的数据分析和处理库,它提供了许多方便的数据操作方法,可以用来处理文本数据并计算词频。
步骤一:安装和导入pandas库
首先,你需要安装pandas库。如果你还没有安装,可以使用pip进行安装:
pip install pandas
然后在Python代码中导入pandas库。
import pandas as pd
步骤二:读取文本数据
读取文本文件并将其存储在一个pandas DataFrame中。
def read_text_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
return text
file_path = 'your_text_file.txt'
text = read_text_file(file_path)
data = {'text': [text]}
df = pd.DataFrame(data)
步骤三:清理和分割文本
使用pandas的str方法对文本进行清理和分割。
def clean_and_split_text(df):
# 去除标点符号
df['text'] = df['text'].str.replace(r'[^\w\s]', '', regex=True)
# 转换为小写
df['text'] = df['text'].str.lower()
# 分割成单词列表
words = df['text'].str.split().explode()
return words
words = clean_and_split_text(df)
步骤四:计算词频
使用pandas的value_counts方法计算词频。
def get_word_frequencies(words):
word_counts = words.value_counts()
return word_counts
word_counts = get_word_frequencies(words)
步骤五:获取前10个高频词
获取前10个高频词。
def get_top_n_words(word_counts, n):
top_n_words = word_counts.head(n)
return top_n_words
top_10_words = get_top_n_words(word_counts, 10)
print(top_10_words)
四、优化和扩展
以上三种方法各有优劣,你可以根据实际需求选择合适的方法。此外,你还可以对这些方法进行优化和扩展,以提高性能和适应更多的应用场景。
优化文本清理
在清理文本时,你可以使用更复杂的正则表达式和文本处理方法,提高清理的准确性。例如,可以使用正则表达式去除HTML标签、处理缩写等。
def advanced_clean_text(text):
# 去除HTML标签
text = re.sub(r'<.*?>', '', text)
# 处理缩写
text = re.sub(r"can't", 'cannot', text)
text = re.sub(r"won't", 'will not', text)
# 其他清理操作
text = re.sub(r'[^\w\s]', '', text)
text = text.lower()
return text
多线程处理
对于大规模文本数据,可以考虑使用多线程或多进程来提高处理速度。Python的concurrent.futures模块提供了方便的多线程和多进程接口。
from concurrent.futures import ThreadPoolExecutor
def process_chunk(chunk):
words = clean_and_split_text(chunk)
word_counts = get_word_frequencies(words)
return word_counts
def parallel_word_count(text, chunk_size=1000):
chunks = [text[i:i+chunk_size] for i in range(0, len(text), chunk_size)]
with ThreadPoolExecutor() as executor:
results = executor.map(process_chunk, chunks)
total_counts = collections.Counter()
for result in results:
total_counts.update(result)
return total_counts
total_counts = parallel_word_count(text)
top_10_words = get_top_n_words(total_counts, 10)
print(top_10_words)
使用数据库
如果你需要处理和存储大量的文本数据,可以考虑使用数据库来管理数据。可以使用SQLite、MySQL、PostgreSQL等关系型数据库,或者使用MongoDB等NoSQL数据库。
import sqlite3
def create_database(db_name):
conn = sqlite3.connect(db_name)
cursor = conn.cursor()
cursor.execute('''
CREATE TABLE IF NOT EXISTS word_counts (
word TEXT PRIMARY KEY,
count INTEGER
)
''')
conn.commit()
return conn
def insert_word_counts(conn, word_counts):
cursor = conn.cursor()
for word, count in word_counts.items():
cursor.execute('''
INSERT INTO word_counts (word, count)
VALUES (?, ?)
ON CONFLICT(word) DO UPDATE SET count=count+excluded.count
''', (word, count))
conn.commit()
conn = create_database('word_counts.db')
insert_word_counts(conn, total_counts)
五、总结
通过本文的介绍,你已经了解了如何使用Python计算前10个词频的方法,包括使用collections模块中的Counter类、使用nltk库、以及使用pandas库。每种方法都有其优点和适用场景,你可以根据实际需求选择合适的方法。此外,我们还探讨了如何优化和扩展这些方法,以提高性能和适应更多的应用场景。
总而言之,计算词频是文本分析中的一个基本任务,通过合理的工具和方法,你可以轻松地实现这一功能,并从中获取有价值的信息。希望本文能够帮助你更好地理解和应用Python进行词频计算。
相关问答FAQs:
如何使用Python计算文本中前10个词的频率?
要计算文本中前10个词的频率,可以使用Python的collections.Counter
类。首先,读取文本数据,然后将其分割成单词,使用Counter
来统计每个单词的出现次数。接着,使用most_common
方法获取前10个频率最高的词。你可以参考以下示例代码:
from collections import Counter
text = "这里是你的文本数据,包含多个单词。"
words = text.split()
word_counts = Counter(words)
top_10_words = word_counts.most_common(10)
print(top_10_words)
在Python中有哪些库可以帮助我分析词频?
Python提供了多个强大的库来分析词频。nltk
是一个流行的自然语言处理库,可以用来进行词汇分割和频率分析。pandas
也可以用于处理数据框和分析频率。此外,sklearn
中的CountVectorizer
可以将文本转换为词频矩阵,方便进行更复杂的分析。
如何处理文本数据中的标点符号以提高词频统计的准确性?
在进行词频统计之前,清洗文本数据是非常重要的。可以使用正则表达式去除标点符号和特殊字符。这样可以确保统计的单词是准确的。例如,可以使用re
模块中的sub
函数来实现这一点。以下是一个简单的示例:
import re
text = "你好,世界!这是一段测试文本。"
cleaned_text = re.sub(r'[^\w\s]', '', text)
words = cleaned_text.split()
这样就可以更准确地计算每个单词的频率。
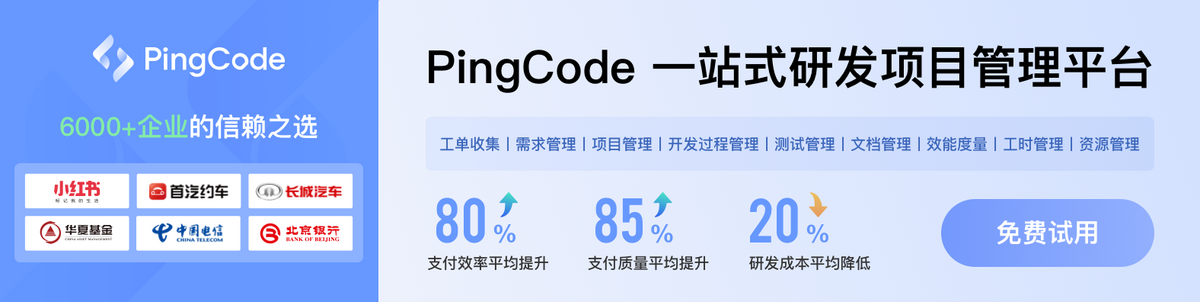