使用print()函数、f-string、%格式化输出、str.format()方法等都可以在Python 3中输出变量。使用print()函数是最常见的方法,它可以直接输出变量的值。比如,print(variable)
。下面将详细介绍每种方法,并给出相关例子。
一、使用print()函数
print()
函数是Python中最基本的输出方式。它可以将变量的值输出到控制台,并自动在输出的末尾添加一个换行符。
name = "Alice"
age = 30
print(name)
print(age)
在这个例子中,print(name)
和print(age)
分别输出变量name
和age
的值。
二、使用f-string
f-string是从Python 3.6开始引入的一种新的字符串格式化方法,使用起来更简洁和直观。它使用大括号{}
来包含变量,并在字符串前加上字母f
。
name = "Alice"
age = 30
print(f"My name is {name} and I am {age} years old.")
在这个例子中,f"My name is {name} and I am {age} years old."
将变量name
和age
的值嵌入到字符串中,并输出到控制台。
三、使用%格式化输出
%格式化输出是Python中一种较早的字符串格式化方法,它类似于C语言中的printf函数。使用%
操作符可以将变量的值嵌入到字符串中。
name = "Alice"
age = 30
print("My name is %s and I am %d years old." % (name, age))
在这个例子中,%s
表示字符串占位符,%d
表示整数占位符,它们分别被name
和age
的值替换。
四、使用str.format()方法
str.format()
方法是Python中另一种常见的字符串格式化方法,它使用大括号{}
作为占位符,并在字符串后调用format()
方法来替换占位符。
name = "Alice"
age = 30
print("My name is {} and I am {} years old.".format(name, age))
在这个例子中,{}
占位符被format(name, age)
方法中的name
和age
的值替换。
五、使用repr()和str()函数
有时候,我们需要将对象转换为字符串形式进行输出。repr()
和str()
函数可以将对象转换为字符串,repr()
返回对象的官方字符串表示,str()
返回对象的可读字符串表示。
x = 42
print("repr:", repr(x))
print("str:", str(x))
在这个例子中,repr(x)
返回'42'
,str(x)
也返回'42'
,但对于某些对象,它们的输出可能会有所不同。
六、使用logging模块
在实际应用中,尤其是开发大型项目时,通常使用logging
模块来记录程序的运行状态。logging
模块提供了多种记录日志的方法,可以根据需要输出不同级别的日志信息。
import logging
logging.basicConfig(level=logging.INFO)
name = "Alice"
age = 30
logging.info("My name is %s and I am %d years old.", name, age)
在这个例子中,logging.info
方法将日志信息输出到控制台,并在信息前添加时间戳和日志级别。
七、使用sys.stdout
有时我们需要将输出重定向到其他地方,比如文件或网络连接。sys.stdout
是一个文件对象,可以使用write
方法将数据输出到指定的地方。
import sys
name = "Alice"
age = 30
sys.stdout.write(f"My name is {name} and I am {age} years old.\n")
在这个例子中,sys.stdout.write
方法将字符串输出到标准输出(通常是控制台)。
八、使用pprint模块
对于复杂的嵌套数据结构,比如列表和字典,pprint
模块可以提供更美观和可读的输出格式。pprint
模块提供了pprint
函数,可以对数据结构进行“美化”输出。
import pprint
data = {"name": "Alice", "age": 30, "hobbies": ["reading", "cycling", "swimming"]}
pprint.pprint(data)
在这个例子中,pprint.pprint
方法将字典data
的内容以更美观的格式输出。
九、使用json模块
当需要输出JSON格式的数据时,可以使用json
模块。json.dumps
方法可以将Python对象转换为JSON字符串,并提供缩进选项,使输出更具可读性。
import json
data = {"name": "Alice", "age": 30, "hobbies": ["reading", "cycling", "swimming"]}
print(json.dumps(data, indent=4))
在这个例子中,json.dumps
方法将字典data
转换为JSON字符串,并使用缩进选项使输出更具可读性。
十、使用IPython.display模块
在Jupyter Notebook或IPython环境中,IPython.display
模块提供了更多的显示选项。可以使用display
函数输出更丰富的内容,比如HTML、图片和音频。
from IPython.display import display, HTML
html_content = "<h1>Hello, World!</h1>"
display(HTML(html_content))
在这个例子中,display
函数将HTML内容输出到Jupyter Notebook的输出单元格中。
十一、使用tqdm模块
在处理长时间运行的循环或任务时,tqdm
模块可以提供进度条,帮助我们实时监控任务的进展。使用tqdm
模块,可以轻松地将进度条添加到循环中。
from tqdm import tqdm
import time
for i in tqdm(range(100)):
time.sleep(0.1)
在这个例子中,tqdm
函数将进度条添加到循环中,并实时更新进度。
十二、使用rich模块
rich
模块是一个用于在终端中显示富文本和美观日志的库。它可以输出彩色文本、表格、进度条等,提升输出的视觉效果。
from rich import print
name = "Alice"
age = 30
print(f"My name is [bold red]{name}[/bold red] and I am [bold blue]{age}[/bold blue] years old.")
在这个例子中,rich.print
函数将文本输出到终端,并使用颜色和样式进行装饰。
十三、输出到文件
有时我们需要将输出结果保存到文件中,而不是在控制台显示。可以使用open
函数打开一个文件,并使用write
方法将数据写入文件。
name = "Alice"
age = 30
with open("output.txt", "w") as file:
file.write(f"My name is {name} and I am {age} years old.\n")
在这个例子中,open
函数打开一个名为output.txt
的文件,并使用write
方法将字符串写入文件。
十四、使用csv模块
当需要输出CSV格式的数据时,可以使用csv
模块。csv
模块提供了writer
类,可以轻松地将数据写入CSV文件。
import csv
data = [("name", "age"), ("Alice", 30), ("Bob", 25)]
with open("output.csv", "w", newline="") as file:
writer = csv.writer(file)
writer.writerows(data)
在这个例子中,csv.writer
类将数据写入output.csv
文件。
十五、使用pandas模块
pandas
模块是一个强大的数据分析库,提供了丰富的数据结构和数据操作方法。可以使用pandas
模块将数据输出为CSV、Excel等格式。
import pandas as pd
data = {"name": ["Alice", "Bob"], "age": [30, 25]}
df = pd.DataFrame(data)
df.to_csv("output.csv", index=False)
在这个例子中,pandas.DataFrame
将数据转换为数据框,并使用to_csv
方法将数据框保存为CSV文件。
十六、使用matplotlib模块
在进行数据可视化时,matplotlib
模块是一个非常有用的工具。可以使用matplotlib
模块生成图表,并将其保存为图像文件。
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [1, 4, 9, 16, 25]
plt.plot(x, y)
plt.savefig("output.png")
plt.show()
在这个例子中,matplotlib.pyplot.plot
函数生成折线图,并使用savefig
方法将图表保存为PNG文件。
十七、使用openpyxl模块
当需要输出Excel格式的数据时,可以使用openpyxl
模块。openpyxl
模块提供了丰富的方法,可以轻松地将数据写入Excel文件。
from openpyxl import Workbook
data = [("name", "age"), ("Alice", 30), ("Bob", 25)]
wb = Workbook()
ws = wb.active
for row in data:
ws.append(row)
wb.save("output.xlsx")
在这个例子中,openpyxl.Workbook
类创建一个新的Excel工作簿,并使用append
方法将数据写入工作表。
十八、使用SQLite数据库
在处理大量数据时,使用数据库是一种常见的选择。SQLite是一种轻量级的嵌入式数据库,可以使用sqlite3
模块将数据保存到SQLite数据库中。
import sqlite3
data = [("Alice", 30), ("Bob", 25)]
conn = sqlite3.connect("output.db")
cursor = conn.cursor()
cursor.execute("CREATE TABLE IF NOT EXISTS users (name TEXT, age INTEGER)")
cursor.executemany("INSERT INTO users (name, age) VALUES (?, ?)", data)
conn.commit()
conn.close()
在这个例子中,sqlite3.connect
函数连接到SQLite数据库,并使用executemany
方法将数据插入到数据库表中。
十九、使用MongoDB数据库
在处理非结构化数据时,MongoDB是一种非常流行的选择。可以使用pymongo
模块将数据保存到MongoDB数据库中。
from pymongo import MongoClient
data = {"name": "Alice", "age": 30}
client = MongoClient("mongodb://localhost:27017/")
db = client["testdb"]
collection = db["users"]
collection.insert_one(data)
在这个例子中,MongoClient
类连接到MongoDB数据库,并使用insert_one
方法将数据插入到集合中。
二十、使用Redis数据库
Redis是一种高性能的键值存储数据库,可以使用redis
模块将数据保存到Redis数据库中。
import redis
data = {"name": "Alice", "age": "30"}
r = redis.Redis(host="localhost", port=6379, db=0)
r.mset(data)
在这个例子中,redis.Redis
类连接到Redis数据库,并使用mset
方法将数据保存到数据库中。
二十一、使用HTTP请求
在进行网络编程时,通常需要通过HTTP请求发送和接收数据。可以使用requests
模块发送HTTP请求,并输出响应结果。
import requests
response = requests.get("https://api.github.com")
print(response.json())
在这个例子中,requests.get
方法发送GET请求,并使用response.json
方法输出响应的JSON数据。
二十二、使用WebSocket
WebSocket是一种全双工通信协议,可以在客户端和服务器之间建立实时通信。可以使用websockets
模块发送和接收WebSocket消息。
import asyncio
import websockets
async def hello():
uri = "ws://localhost:8765"
async with websockets.connect(uri) as websocket:
await websocket.send("Hello, World!")
response = await websocket.recv()
print(response)
asyncio.get_event_loop().run_until_complete(hello())
在这个例子中,websockets.connect
方法连接到WebSocket服务器,并使用send
和recv
方法发送和接收消息。
二十三、使用Django框架
Django是一个高级的Python Web框架,可以快速构建Web应用程序。可以使用Django框架将数据输出到Web页面。
from django.shortcuts import render
def index(request):
context = {"name": "Alice", "age": 30}
return render(request, "index.html", context)
在这个例子中,render
函数将数据传递到HTML模板,并在Web页面上输出。
二十四、使用Flask框架
Flask是一个轻量级的Python Web框架,适合构建小型Web应用程序。可以使用Flask框架将数据输出到Web页面。
from flask import Flask, render_template
app = Flask(__name__)
@app.route("/")
def index():
context = {"name": "Alice", "age": 30}
return render_template("index.html", context)
if __name__ == "__main__":
app.run(debug=True)
在这个例子中,render_template
函数将数据传递到HTML模板,并在Web页面上输出。
二十五、使用Jinja2模板引擎
Jinja2是一个现代的Python模板引擎,可以在HTML模板中嵌入Python代码。可以使用Jinja2模板引擎将数据输出到HTML文件。
from jinja2 import Template
template = Template("My name is {{ name }} and I am {{ age }} years old.")
output = template.render(name="Alice", age=30)
print(output)
在这个例子中,Template
类创建一个模板,并使用render
方法将数据渲染到模板中。
二十六、使用Tkinter模块
在构建桌面应用程序时,Tkinter是Python的标准GUI库。可以使用Tkinter模块将数据输出到GUI窗口。
import tkinter as tk
root = tk.Tk()
label = tk.Label(root, text="Hello, World!")
label.pack()
root.mainloop()
在这个例子中,tk.Label
类创建一个标签,并使用pack
方法将标签添加到窗口中。
二十七、使用PyQt模块
PyQt是另一个流行的Python GUI库,可以构建跨平台的桌面应用程序。可以使用PyQt模块将数据输出到GUI窗口。
import sys
from PyQt5.QtWidgets import QApplication, QLabel
app = QApplication(sys.argv)
label = QLabel("Hello, World!")
label.show()
sys.exit(app.exec_())
在这个例子中,QLabel
类创建一个标签,并使用show
方法将标签显示在窗口中。
二十八、使用Kivy模块
Kivy是一个开源的Python框架,可以构建跨平台的移动和桌面应用程序。可以使用Kivy模块将数据输出到GUI窗口。
from kivy.app import App
from kivy.uix.label import Label
class MyApp(App):
def build(self):
return Label(text="Hello, World!")
if __name__ == "__main__":
MyApp().run()
在这个例子中,Label
类创建一个标签,并将标签显示在窗口中。
二十九、使用Pygame模块
Pygame是一个用于构建游戏的Python库,可以使用Pygame模块将数据输出到游戏窗口。
import pygame
pygame.init()
screen = pygame.display.set_mode((640, 480))
pygame.display.set_caption("Hello, World!")
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((255, 255, 255))
font = pygame.font.Font(None, 36)
text = font.render("Hello, World!", True, (0, 0, 0))
screen.blit(text, (100, 100))
pygame.display.flip()
pygame.quit()
在这个例子中,pygame.font.Font
类创建一个字体对象,并使用render
方法将文本渲染到屏幕上。
三十、使用Turtle模块
Turtle是Python的标准库之一,主要用于绘制图形。可以使用Turtle模块将数据输出到图形窗口。
import turtle
t = turtle.Turtle()
t.write("Hello, World!", move=False, align="left", font=("Arial", 16, "normal"))
turtle.done()
相关问答FAQs:
如何在Python3中打印多个变量的值?
在Python3中,可以使用print()
函数同时输出多个变量的值。只需将变量以逗号分隔放在print()
函数内即可。例如:
a = 10
b = 20
print(a, b)
这将输出10 20
,并在变量之间添加一个空格。
Python3中的格式化输出有哪些方法?
在Python3中,格式化输出可以通过多种方式实现。常见的方法包括使用f-string(格式化字符串字面量)、str.format()
方法和百分号格式化。例如,使用f-string可以这样写:
name = "Alice"
age = 30
print(f"{name} is {age} years old.")
这种方式不仅简洁,而且易于阅读。
如何将变量的值写入文件而不是打印到控制台?
在Python3中,可以使用open()
函数创建或打开一个文件,并使用write()
方法将变量的值写入文件。例如:
with open('output.txt', 'w') as file:
variable = "Hello, World!"
file.write(variable)
这段代码将把"Hello, World!"
写入名为output.txt
的文件中。使用with
语句可以确保文件在操作完成后自动关闭。
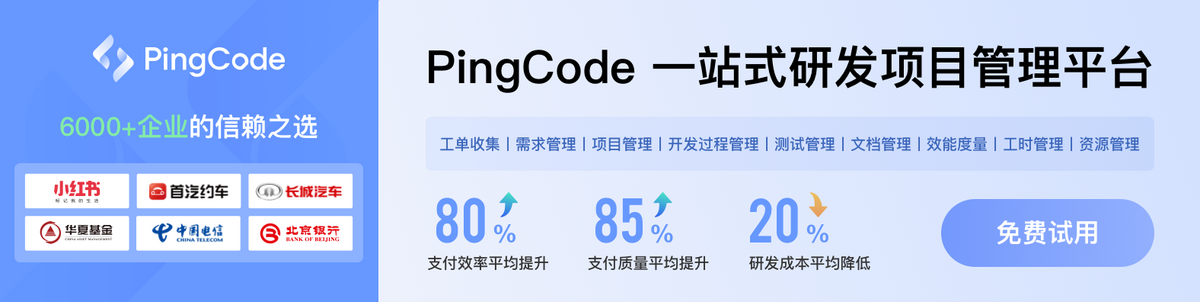