要在Python中求元素的个数,可以使用多种方法,包括count()
方法、Counter
类、遍历循环、字典计数等。最常用的方法是使用count()
方法、Counter
类,这些方法能够高效地统计元素的个数。在这篇文章中,我们将详细介绍这些方法,并提供代码示例和应用场景。
一、使用 count()
方法
count()
方法是 Python 中字符串和列表对象的一个内置方法,用于统计某个特定元素在字符串或列表中出现的次数。
1、列表中的 count()
方法
当我们需要统计列表中某个元素的出现次数时,可以使用列表对象的 count()
方法。语法格式如下:
list.count(element)
其中 list
是目标列表,element
是需要统计的元素。
示例代码:
my_list = [1, 2, 3, 2, 2, 4, 5]
count_of_2 = my_list.count(2)
print(f"The element 2 appears {count_of_2} times in the list.")
输出:
The element 2 appears 3 times in the list.
2、字符串中的 count()
方法
类似于列表,字符串对象也有 count()
方法用于统计子字符串在字符串中出现的次数。语法格式如下:
str.count(substring, start, end)
其中 str
是目标字符串,substring
是需要统计的子字符串,start
和 end
是可选参数,表示统计的起始和结束位置。
示例代码:
my_string = "hello world, hello python"
count_of_hello = my_string.count("hello")
print(f"The substring 'hello' appears {count_of_hello} times in the string.")
输出:
The substring 'hello' appears 2 times in the string.
二、使用 collections.Counter
类
collections
模块中的 Counter
类是一个非常有用的工具,它可以用来统计可迭代对象中各个元素的个数,并返回一个字典形式的对象。
1、统计列表元素个数
使用 Counter
类统计列表中各元素的出现次数非常方便,只需将列表传递给 Counter
构造函数即可。
示例代码:
from collections import Counter
my_list = [1, 2, 3, 2, 2, 4, 5]
counter = Counter(my_list)
print(counter)
输出:
Counter({2: 3, 1: 1, 3: 1, 4: 1, 5: 1})
2、统计字符串字符个数
同样的,Counter
类也可以用来统计字符串中各字符的出现次数。
示例代码:
from collections import Counter
my_string = "hello world"
counter = Counter(my_string)
print(counter)
输出:
Counter({'l': 3, 'o': 2, 'h': 1, 'e': 1, ' ': 1, 'w': 1, 'r': 1, 'd': 1})
3、统计单词出现次数
Counter
类还可以用来统计文本中各单词的出现次数,只需先将文本分割成单词列表,然后传递给 Counter
构造函数即可。
示例代码:
from collections import Counter
text = "hello world hello python"
words = text.split()
counter = Counter(words)
print(counter)
输出:
Counter({'hello': 2, 'world': 1, 'python': 1})
三、使用字典进行计数
除了使用内置方法和 Counter
类,我们还可以使用字典来手动统计元素的个数。这种方法虽然较为繁琐,但在某些特定场景下可能更具灵活性。
1、列表元素计数
通过遍历列表并使用字典记录每个元素的出现次数,我们可以实现列表元素的计数。
示例代码:
my_list = [1, 2, 3, 2, 2, 4, 5]
count_dict = {}
for element in my_list:
if element in count_dict:
count_dict[element] += 1
else:
count_dict[element] = 1
print(count_dict)
输出:
{1: 1, 2: 3, 3: 1, 4: 1, 5: 1}
2、字符串字符计数
类似地,我们也可以使用字典来统计字符串中各字符的出现次数。
示例代码:
my_string = "hello world"
count_dict = {}
for char in my_string:
if char in count_dict:
count_dict[char] += 1
else:
count_dict[char] = 1
print(count_dict)
输出:
{'h': 1, 'e': 1, 'l': 3, 'o': 2, ' ': 1, 'w': 1, 'r': 1, 'd': 1}
四、使用 pandas
统计元素个数
pandas
是一个强大的数据分析库,它提供了许多方便的数据处理方法。我们可以使用 pandas
来统计数据框列中元素的个数。
1、统计单列中元素个数
我们可以使用 value_counts()
方法来统计数据框某一列中各元素的出现次数。
示例代码:
import pandas as pd
data = {'fruits': ['apple', 'banana', 'apple', 'banana', 'cherry', 'banana']}
df = pd.DataFrame(data)
count_series = df['fruits'].value_counts()
print(count_series)
输出:
banana 3
apple 2
cherry 1
Name: fruits, dtype: int64
2、统计多列中元素个数
如果我们需要统计多个列中元素的出现次数,可以使用 apply()
方法和 value_counts()
方法的组合。
示例代码:
import pandas as pd
data = {'fruits': ['apple', 'banana', 'apple', 'banana', 'cherry', 'banana'],
'colors': ['red', 'yellow', 'red', 'yellow', 'red', 'yellow']}
df = pd.DataFrame(data)
count_df = df.apply(pd.Series.value_counts)
print(count_df)
输出:
fruits colors
apple 2.0 NaN
banana 3.0 NaN
cherry 1.0 NaN
red NaN 3.0
yellow NaN 3.0
五、使用 numpy
统计元素个数
numpy
是一个强大的科学计算库,提供了许多高效的数组操作方法。我们可以使用 numpy
来统计数组中元素的个数。
1、统计数组中元素个数
我们可以使用 numpy.unique()
函数来统计数组中各元素的出现次数。
示例代码:
import numpy as np
my_array = np.array([1, 2, 3, 2, 2, 4, 5])
unique_elements, counts = np.unique(my_array, return_counts=True)
element_counts = dict(zip(unique_elements, counts))
print(element_counts)
输出:
{1: 1, 2: 3, 3: 1, 4: 1, 5: 1}
2、统计矩阵中元素个数
我们也可以使用 numpy.unique()
函数来统计矩阵中各元素的出现次数。
示例代码:
import numpy as np
my_matrix = np.array([[1, 2, 2],
[3, 2, 4],
[5, 1, 2]])
unique_elements, counts = np.unique(my_matrix, return_counts=True)
element_counts = dict(zip(unique_elements, counts))
print(element_counts)
输出:
{1: 2, 2: 4, 3: 1, 4: 1, 5: 1}
六、使用 itertools
统计元素个数
itertools
模块提供了一些高效的迭代器函数,可以用于统计元素的个数。
1、使用 itertools.groupby
统计元素个数
itertools.groupby
函数可以用于分组统计元素的个数,但需要先对数据进行排序。
示例代码:
import itertools
my_list = [1, 2, 3, 2, 2, 4, 5]
sorted_list = sorted(my_list)
grouped = itertools.groupby(sorted_list)
element_counts = {key: len(list(group)) for key, group in grouped}
print(element_counts)
输出:
{1: 1, 2: 3, 3: 1, 4: 1, 5: 1}
2、使用 itertools.chain
统计多个列表元素个数
itertools.chain
函数可以将多个迭代器合并为一个,然后进行统计。
示例代码:
import itertools
from collections import Counter
list1 = [1, 2, 2]
list2 = [2, 3, 4]
merged_list = itertools.chain(list1, list2)
counter = Counter(merged_list)
print(counter)
输出:
Counter({2: 3, 1: 1, 3: 1, 4: 1})
七、总结
通过以上几种方法,我们可以在Python中高效地统计元素的个数。count()
方法适用于简单的列表和字符串统计,Counter
类和字典计数方法则适用于更复杂的统计需求。pandas
和 numpy
提供了强大的数据处理功能,适用于大规模数据的统计。根据具体的应用场景选择合适的方法,可以事半功倍地完成统计任务。希望这篇文章对你有所帮助!
相关问答FAQs:
如何在Python中统计列表中某个特定元素的出现次数?
在Python中,可以使用count()
方法来统计列表中某个特定元素的出现次数。该方法的语法为list.count(element)
,其中element
是你想要统计的元素。例如,假设有一个列表my_list = [1, 2, 2, 3, 4, 2]
,你可以通过my_list.count(2)
来获得数字2在列表中出现的次数,返回结果将是3。
有没有其他方法可以计算字典中元素的数量?
当然可以。对于字典而言,可以使用len()
函数来获取字典中键值对的数量。比如,如果你有一个字典my_dict = {'a': 1, 'b': 2, 'c': 3}
,使用len(my_dict)
将返回3,表示字典中有三个键值对。如果需要统计某个特定值的数量,可以通过遍历字典并使用条件判断来实现。
在Python中,如何使用collections模块来计数?
Python的collections
模块提供了一个名为Counter
的类,可以非常方便地统计可迭代对象中元素的数量。使用方法如下:首先导入Counter
,然后将要计数的列表或其他可迭代对象传递给Counter
。例如:
from collections import Counter
my_list = [1, 2, 2, 3, 4, 2]
counter = Counter(my_list)
print(counter[2]) # 输出将是3
这种方法不仅简洁,而且能够快速得到所有元素的计数结果,便于进一步分析。
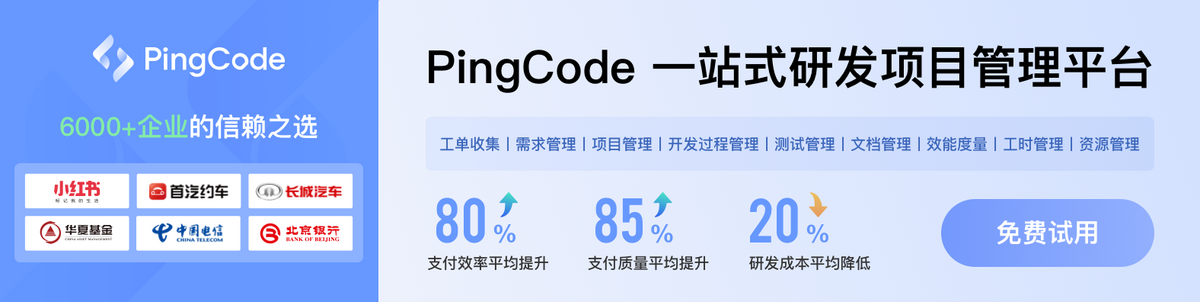