在Python中实现词频统计的方法有很多种,常用的方法包括使用Counter类、利用字典、正则表达式处理文本等。使用Counter类是最简单且高效的方法之一。下面详细介绍如何使用Counter类来实现词频统计。
使用Counter类:
Counter是collections模块中的一个类,专门用于统计元素的频率。使用Counter类进行词频统计非常简单,只需要几行代码即可完成。
from collections import Counter
def word_count(text):
words = text.split()
word_counts = Counter(words)
return word_counts
text = "This is a test. This test is only a test."
word_counts = word_count(text)
print(word_counts)
在上述代码中,首先使用split()
方法将文本按空白字符分割成单词列表,然后利用Counter
类对单词列表进行统计,最后返回一个Counter
对象,其中包含每个单词的频率。Counter类不仅简洁,而且性能较高,适合处理大规模文本。
接下来,我们将详细探讨如何使用不同的方法实现词频统计,并在不同场景中进行优化。
一、使用字典进行词频统计
使用字典进行词频统计是一种基本且直观的方法。我们可以通过遍历文本中的单词,并将每个单词作为字典的键,出现次数作为字典的值。以下是具体实现方法:
def word_count_dict(text):
words = text.split()
word_counts = {}
for word in words:
if word in word_counts:
word_counts[word] += 1
else:
word_counts[word] = 1
return word_counts
text = "This is a test. This test is only a test."
word_counts = word_count_dict(text)
print(word_counts)
在这个实现方法中,我们首先将文本按空白字符分割成单词列表,然后遍历单词列表,对于每个单词,如果它已经在字典中,则将其对应的值加1;如果不在字典中,则将其添加到字典中,并将值设为1。最终返回包含每个单词频率的字典。
虽然这种方法比使用Counter类稍微复杂一些,但它更灵活,可以方便地进行各种自定义处理。
二、利用正则表达式处理文本
在实际应用中,文本往往包含标点符号、大小写混合等情况,直接使用split()方法可能无法得到理想的结果。我们可以使用正则表达式对文本进行预处理,以提高词频统计的准确性。
import re
from collections import Counter
def word_count_regex(text):
words = re.findall(r'\b\w+\b', text.lower())
word_counts = Counter(words)
return word_counts
text = "This is a test. This test is only a test."
word_counts = word_count_regex(text)
print(word_counts)
在上述代码中,我们首先使用正则表达式r'\b\w+\b'
匹配所有单词,并将文本转换为小写,以确保统计结果不区分大小写。然后利用Counter类对单词列表进行统计。这样可以处理包含标点符号和大小写混合的文本,提高统计准确性。
三、处理大规模文本
当处理大规模文本时,内存和计算效率是需要考虑的重要因素。我们可以使用生成器和流式处理技术来优化词频统计的性能。
import re
from collections import Counter
def word_count_large_text(file_path):
def words_generator(file_path):
with open(file_path, 'r') as file:
for line in file:
for word in re.findall(r'\b\w+\b', line.lower()):
yield word
word_counts = Counter(words_generator(file_path))
return word_counts
file_path = 'large_text_file.txt'
word_counts = word_count_large_text(file_path)
print(word_counts)
在这个实现方法中,我们定义了一个生成器words_generator
,逐行读取文件,并使用正则表达式匹配单词。生成器可以在需要时生成单词,而不需要一次性将整个文本加载到内存中,从而节省内存空间。最后,我们使用Counter类对生成的单词进行统计。
四、多线程与多进程优化
对于超大规模文本,可以利用多线程和多进程技术进行并行处理,以进一步提高词频统计的效率。
使用多线程:
import re
from collections import Counter
from concurrent.futures import ThreadPoolExecutor
def word_count_thread(file_path, num_threads=4):
def process_chunk(chunk):
words = re.findall(r'\b\w+\b', chunk.lower())
return Counter(words)
with open(file_path, 'r') as file:
lines = file.readlines()
chunk_size = len(lines) // num_threads
chunks = [lines[i:i + chunk_size] for i in range(0, len(lines), chunk_size)]
with ThreadPoolExecutor(max_workers=num_threads) as executor:
results = executor.map(lambda chunk: process_chunk(' '.join(chunk)), chunks)
word_counts = Counter()
for result in results:
word_counts.update(result)
return word_counts
file_path = 'large_text_file.txt'
word_counts = word_count_thread(file_path)
print(word_counts)
在这个实现方法中,我们首先将文件按行读取并分割成多个块,每个块由一个线程处理。使用ThreadPoolExecutor创建线程池,并行处理每个块,最后合并各线程的统计结果。
使用多进程:
import re
from collections import Counter
from multiprocessing import Pool
def word_count_process(file_path, num_processes=4):
def process_chunk(chunk):
words = re.findall(r'\b\w+\b', chunk.lower())
return Counter(words)
with open(file_path, 'r') as file:
lines = file.readlines()
chunk_size = len(lines) // num_processes
chunks = [lines[i:i + chunk_size] for i in range(0, len(lines), chunk_size)]
with Pool(processes=num_processes) as pool:
results = pool.map(lambda chunk: process_chunk(' '.join(chunk)), chunks)
word_counts = Counter()
for result in results:
word_counts.update(result)
return word_counts
file_path = 'large_text_file.txt'
word_counts = word_count_process(file_path)
print(word_counts)
在这个实现方法中,我们使用了多进程技术,采用Pool创建进程池,并行处理每个块,最后合并各进程的统计结果。多进程技术可以更好地利用多核CPU的计算能力,适合处理计算密集型任务。
五、使用NLP工具包
在进行文本处理时,利用自然语言处理(NLP)工具包可以简化很多工作。常用的NLP工具包包括NLTK、spaCy等。
使用NLTK:
import nltk
from collections import Counter
def word_count_nltk(text):
words = nltk.word_tokenize(text.lower())
word_counts = Counter(words)
return word_counts
text = "This is a test. This test is only a test."
word_counts = word_count_nltk(text)
print(word_counts)
在这个实现方法中,我们使用NLTK的word_tokenize
函数对文本进行分词,并将其转换为小写,然后利用Counter类进行统计。NLTK提供了丰富的文本处理功能,可以方便地进行更多高级处理。
使用spaCy:
import spacy
from collections import Counter
def word_count_spacy(text):
nlp = spacy.load('en_core_web_sm')
doc = nlp(text.lower())
words = [token.text for token in doc if token.is_alpha]
word_counts = Counter(words)
return word_counts
text = "This is a test. This test is only a test."
word_counts = word_count_spacy(text)
print(word_counts)
在这个实现方法中,我们使用spaCy的分词功能,并过滤掉非字母的token,然后利用Counter类进行统计。spaCy在处理大规模文本时表现出色,适合进行高效的文本分析。
六、可视化词频统计结果
为了更直观地展示词频统计结果,我们可以使用matplotlib或其他可视化工具对结果进行可视化。
使用matplotlib:
import matplotlib.pyplot as plt
def plot_word_frequency(word_counts, top_n=10):
common_words = word_counts.most_common(top_n)
words, counts = zip(*common_words)
plt.bar(words, counts)
plt.xlabel('Words')
plt.ylabel('Frequency')
plt.title('Top {} Words by Frequency'.format(top_n))
plt.show()
text = "This is a test. This test is only a test."
word_counts = word_count_spacy(text)
plot_word_frequency(word_counts)
在这个实现方法中,我们首先获取词频最高的前N个单词及其频率,然后使用matplotlib绘制柱状图,展示这些单词的频率分布。可视化结果可以帮助我们更直观地理解文本中的词频分布情况。
七、处理不同语言的文本
在进行词频统计时,不同语言的文本可能需要不同的处理方式。例如,中文文本的分词需要使用专门的工具包,如jieba。
使用jieba处理中文文本:
import jieba
from collections import Counter
def word_count_chinese(text):
words = jieba.lcut(text)
word_counts = Counter(words)
return word_counts
text = "这是一个测试。这只是一个测试。"
word_counts = word_count_chinese(text)
print(word_counts)
在这个实现方法中,我们使用jieba的lcut
函数对中文文本进行分词,然后利用Counter类进行统计。jieba是一个常用的中文分词工具包,支持多种分词模式,适合处理中文文本。
八、处理特殊文本格式
在实际应用中,文本数据可能存储在各种不同的格式中,如CSV、JSON等。我们需要根据具体格式进行预处理,以进行词频统计。
处理CSV文件:
import csv
from collections import Counter
def word_count_csv(file_path, column_name):
with open(file_path, 'r') as file:
reader = csv.DictReader(file)
text = ' '.join([row[column_name] for row in reader])
words = text.split()
word_counts = Counter(words)
return word_counts
file_path = 'data.csv'
column_name = 'text'
word_counts = word_count_csv(file_path, column_name)
print(word_counts)
在这个实现方法中,我们使用csv模块读取CSV文件,并提取指定列的文本进行词频统计。通过这种方式,可以方便地处理存储在CSV文件中的文本数据。
处理JSON文件:
import json
from collections import Counter
def word_count_json(file_path, key):
with open(file_path, 'r') as file:
data = json.load(file)
text = ' '.join([item[key] for item in data])
words = text.split()
word_counts = Counter(words)
return word_counts
file_path = 'data.json'
key = 'text'
word_counts = word_count_json(file_path, key)
print(word_counts)
在这个实现方法中,我们使用json模块读取JSON文件,并提取指定键的文本进行词频统计。通过这种方式,可以方便地处理存储在JSON文件中的文本数据。
九、处理文本预处理
在进行词频统计之前,对文本进行适当的预处理,可以提高统计结果的准确性和有效性。常见的预处理步骤包括去除停用词、词形还原(词干提取和词形归一化)等。
去除停用词:
from collections import Counter
from nltk.corpus import stopwords
def word_count_stopwords(text):
stop_words = set(stopwords.words('english'))
words = [word for word in text.split() if word.lower() not in stop_words]
word_counts = Counter(words)
return word_counts
text = "This is a test. This test is only a test."
word_counts = word_count_stopwords(text)
print(word_counts)
在这个实现方法中,我们使用NLTK的停用词列表,去除文本中的停用词,然后利用Counter类进行统计。去除停用词可以避免常见词对统计结果的干扰,提高统计结果的有效性。
词形还原:
from collections import Counter
from nltk.stem import PorterStemmer
def word_count_stemming(text):
stemmer = PorterStemmer()
words = [stemmer.stem(word) for word in text.split()]
word_counts = Counter(words)
return word_counts
text = "This is a test. This test is only a test."
word_counts = word_count_stemming(text)
print(word_counts)
在这个实现方法中,我们使用NLTK的Porter词干提取器,将单词还原为词干形式,然后利用Counter类进行统计。词形还原可以将同一词汇的不同形式合并,提高统计结果的准确性。
十、总结
在Python中实现词频统计的方法有很多,从简单的使用Counter类到复杂的多线程、多进程优化,每种方法都有其适用的场景。通过对文本进行预处理、利用NLP工具包和可视化工具,我们可以更准确、高效地进行词频统计,并展示统计结果。
使用Counter类、利用字典、正则表达式处理文本是实现词频统计的基本方法,针对不同的文本和场景,可以选择合适的方法进行处理。希望通过本文的介绍,能够帮助你更好地理解和实现词频统计。
相关问答FAQs:
如何在Python中读取文本文件以进行词频统计?
在进行词频统计之前,需要将文本数据导入Python。可以使用内置的open()
函数来读取文本文件,结合read()
方法获取文件内容。接着,可以使用字符串的split()
方法将内容拆分为单词,从而为后续的词频统计做准备。
使用Python中的哪些库可以更方便地进行词频统计?
为了简化词频统计的过程,可以使用一些强大的库,例如collections
中的Counter
类。这一类提供了一种简单的方法来统计可哈希对象的频率。此外,nltk
和pandas
等库也提供了丰富的文本处理和数据分析工具,可以帮助更高效地进行词频统计。
如何处理文本中的标点符号和大小写,以提高词频统计的准确性?
在进行词频统计时,通常需要对文本进行预处理,以去除标点符号和统一大小写。可以使用str.lower()
方法将文本转换为小写,使用re
模块中的正则表达式来删除标点符号。这样可以确保相同的词汇在统计中只被计算一次,提高统计结果的准确性。
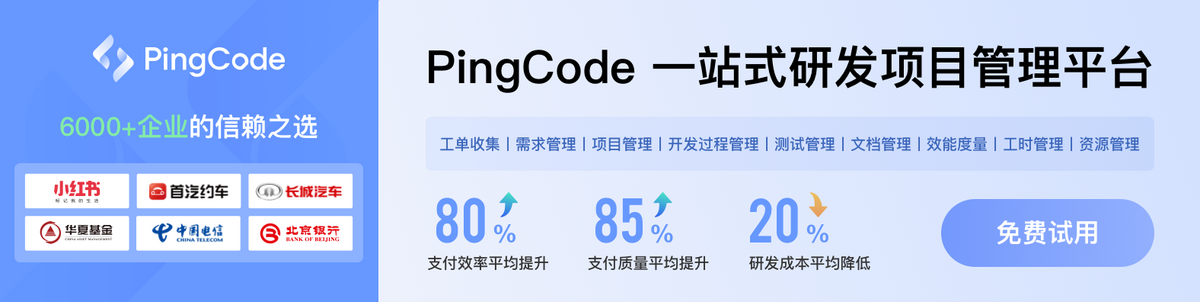