Python判断字符类型编码可以通过以下几种方法:使用chardet库、使用cchardet库、使用charset-normalizer库、手动检测BOM头。 其中,chardet库是最常用的方式,可以自动检测字符编码并返回结果。接下来我们详细讨论使用chardet库的方法。
使用chardet库
chardet 是一个通用字符编码检测器,能够检测出文本的字符编码。使用chardet库非常简单,只需要安装并调用相关函数即可。以下是使用chardet库的详细步骤:
-
安装chardet库:
首先需要安装chardet库,可以使用pip进行安装:
pip install chardet
-
使用chardet库检测字符编码:
使用chardet库检测字符编码只需要几行代码,以下是一个示例:
import chardet
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
result = chardet.detect(raw_data)
encoding = result['encoding']
confidence = result['confidence']
return encoding, confidence
file_path = 'example.txt'
encoding, confidence = detect_encoding(file_path)
print(f'Encoding: {encoding}, Confidence: {confidence}')
在这个示例中,我们读取了文件的原始二进制数据,然后使用chardet库的
detect
函数来检测其字符编码。detect
函数返回一个字典,包含检测到的编码和置信度。最后,我们打印出编码和置信度。
使用cchardet库
cchardet 是chardet库的一个快速版本,速度更快,但使用方法与chardet相同。以下是使用cchardet库的详细步骤:
-
安装cchardet库:
首先需要安装cchardet库,可以使用pip进行安装:
pip install cchardet
-
使用cchardet库检测字符编码:
使用cchardet库检测字符编码的方法与chardet库几乎相同,以下是一个示例:
import cchardet
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
result = cchardet.detect(raw_data)
encoding = result['encoding']
confidence = result['confidence']
return encoding, confidence
file_path = 'example.txt'
encoding, confidence = detect_encoding(file_path)
print(f'Encoding: {encoding}, Confidence: {confidence}')
在这个示例中,代码结构与chardet库完全相同,只是将导入的库从
chardet
换成了cchardet
。
使用charset-normalizer库
charset-normalizer 是另一个用于检测字符编码的库,特别适用于Python 3。以下是使用charset-normalizer库的详细步骤:
-
安装charset-normalizer库:
首先需要安装charset-normalizer库,可以使用pip进行安装:
pip install charset-normalizer
-
使用charset-normalizer库检测字符编码:
使用charset-normalizer库检测字符编码的方法如下:
from charset_normalizer import CharsetNormalizerMatches as CnM
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
matches = CnM.from_bytes(raw_data)
best_match = matches.best()
encoding = best_match.encoding
confidence = best_match.fingerprint().get('confidence')
return encoding, confidence
file_path = 'example.txt'
encoding, confidence = detect_encoding(file_path)
print(f'Encoding: {encoding}, Confidence: {confidence}')
在这个示例中,我们使用CharsetNormalizerMatches类从原始二进制数据中检测字符编码,并获取最佳匹配的编码和置信度。
手动检测BOM头
在某些情况下,可以通过手动检测文件的BOM(Byte Order Mark)头来确定字符编码。以下是几种常见的BOM头:
- UTF-8 BOM:
EF BB BF
- UTF-16 (LE) BOM:
FF FE
- UTF-16 (BE) BOM:
FE FF
- UTF-32 (LE) BOM:
FF FE 00 00
- UTF-32 (BE) BOM:
00 00 FE FF
以下是检测BOM头的示例代码:
def detect_bom(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read(4) # 读取前4个字节
if raw_data.startswith(b'\xef\xbb\xbf'):
return 'utf-8-sig'
elif raw_data.startswith(b'\xff\xfe\x00\x00'):
return 'utf-32le'
elif raw_data.startswith(b'\x00\x00\xfe\xff'):
return 'utf-32be'
elif raw_data.startswith(b'\xff\xfe'):
return 'utf-16le'
elif raw_data.startswith(b'\xfe\xff'):
return 'utf-16be'
else:
return 'unknown'
file_path = 'example.txt'
encoding = detect_bom(file_path)
print(f'Encoding: {encoding}')
在这个示例中,我们读取文件的前4个字节,并根据BOM头来判断字符编码。
一、使用chardet库
安装与基础使用
chardet库是一个非常流行的字符编码检测库,可以检测多种字符编码。安装chardet库非常简单,只需要使用pip命令即可:
pip install chardet
安装完成后,就可以在代码中导入chardet库并使用它来检测字符编码。以下是一个简单的示例代码:
import chardet
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
result = chardet.detect(raw_data)
encoding = result['encoding']
confidence = result['confidence']
return encoding, confidence
file_path = 'example.txt'
encoding, confidence = detect_encoding(file_path)
print(f'Encoding: {encoding}, Confidence: {confidence}')
在这个示例中,我们读取了文件的原始二进制数据,然后使用chardet库的detect
函数来检测其字符编码。detect
函数返回一个字典,包含检测到的编码和置信度。最后,我们打印出编码和置信度。
处理大文件
对于大文件,一次性读取全部内容可能会导致内存不足的问题。可以分块读取文件内容,并使用chardet的UniversalDetector
类逐块检测编码。以下是一个处理大文件的示例代码:
import chardet
def detect_encoding_large_file(file_path):
detector = chardet.UniversalDetector()
with open(file_path, 'rb') as file:
for line in file:
detector.feed(line)
if detector.done:
break
detector.close()
result = detector.result
encoding = result['encoding']
confidence = result['confidence']
return encoding, confidence
file_path = 'large_example.txt'
encoding, confidence = detect_encoding_large_file(file_path)
print(f'Encoding: {encoding}, Confidence: {confidence}')
在这个示例中,我们使用了chardet.UniversalDetector
类来逐块检测文件内容的编码。当检测器确认检测完成后,我们停止读取文件并获取检测结果。
二、使用cchardet库
安装与基础使用
cchardet库是chardet库的一个快速版本,可以提高检测速度。安装cchardet库也非常简单,只需要使用pip命令:
pip install cchardet
安装完成后,就可以在代码中导入cchardet库并使用它来检测字符编码。以下是一个简单的示例代码:
import cchardet
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
result = cchardet.detect(raw_data)
encoding = result['encoding']
confidence = result['confidence']
return encoding, confidence
file_path = 'example.txt'
encoding, confidence = detect_encoding(file_path)
print(f'Encoding: {encoding}, Confidence: {confidence}')
在这个示例中,代码结构与chardet库完全相同,只是将导入的库从chardet
换成了cchardet
。
处理大文件
对于大文件,同样可以分块读取文件内容,并使用cchardet库进行逐块检测。以下是一个处理大文件的示例代码:
import cchardet
def detect_encoding_large_file(file_path):
detector = cchardet.UniversalDetector()
with open(file_path, 'rb') as file:
for line in file:
detector.feed(line)
if detector.done:
break
detector.close()
result = detector.result
encoding = result['encoding']
confidence = result['confidence']
return encoding, confidence
file_path = 'large_example.txt'
encoding, confidence = detect_encoding_large_file(file_path)
print(f'Encoding: {encoding}, Confidence: {confidence}')
在这个示例中,我们使用了cchardet.UniversalDetector
类来逐块检测文件内容的编码。当检测器确认检测完成后,我们停止读取文件并获取检测结果。
三、使用charset-normalizer库
安装与基础使用
charset-normalizer库是另一个用于检测字符编码的库,特别适用于Python 3。安装charset-normalizer库可以使用pip命令:
pip install charset-normalizer
安装完成后,就可以在代码中导入charset-normalizer库并使用它来检测字符编码。以下是一个简单的示例代码:
from charset_normalizer import CharsetNormalizerMatches as CnM
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
matches = CnM.from_bytes(raw_data)
best_match = matches.best()
encoding = best_match.encoding
confidence = best_match.fingerprint().get('confidence')
return encoding, confidence
file_path = 'example.txt'
encoding, confidence = detect_encoding(file_path)
print(f'Encoding: {encoding}, Confidence: {confidence}')
在这个示例中,我们使用CharsetNormalizerMatches类从原始二进制数据中检测字符编码,并获取最佳匹配的编码和置信度。
处理大文件
对于大文件,同样可以分块读取文件内容,并使用charset-normalizer库进行逐块检测。以下是一个处理大文件的示例代码:
from charset_normalizer import CharsetNormalizerMatches as CnM
def detect_encoding_large_file(file_path):
matches = CnM()
with open(file_path, 'rb') as file:
for line in file:
matches.feed(line)
if matches.done:
break
matches.close()
best_match = matches.best()
encoding = best_match.encoding
confidence = best_match.fingerprint().get('confidence')
return encoding, confidence
file_path = 'large_example.txt'
encoding, confidence = detect_encoding_large_file(file_path)
print(f'Encoding: {encoding}, Confidence: {confidence}')
在这个示例中,我们使用了CharsetNormalizerMatches类来逐块检测文件内容的编码。当检测器确认检测完成后,我们停止读取文件并获取检测结果。
四、手动检测BOM头
常见的BOM头
在某些情况下,可以通过手动检测文件的BOM(Byte Order Mark)头来确定字符编码。以下是几种常见的BOM头:
- UTF-8 BOM:
EF BB BF
- UTF-16 (LE) BOM:
FF FE
- UTF-16 (BE) BOM:
FE FF
- UTF-32 (LE) BOM:
FF FE 00 00
- UTF-32 (BE) BOM:
00 00 FE FF
检测BOM头的示例代码
以下是检测BOM头的示例代码:
def detect_bom(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read(4) # 读取前4个字节
if raw_data.startswith(b'\xef\xbb\xbf'):
return 'utf-8-sig'
elif raw_data.startswith(b'\xff\xfe\x00\x00'):
return 'utf-32le'
elif raw_data.startswith(b'\x00\x00\xfe\xff'):
return 'utf-32be'
elif raw_data.startswith(b'\xff\xfe'):
return 'utf-16le'
elif raw_data.startswith(b'\xfe\xff'):
return 'utf-16be'
else:
return 'unknown'
file_path = 'example.txt'
encoding = detect_bom(file_path)
print(f'Encoding: {encoding}')
在这个示例中,我们读取文件的前4个字节,并根据BOM头来判断字符编码。
五、总结
chardet库
chardet库是一个通用字符编码检测器,能够检测出文本的字符编码。使用chardet库非常简单,只需要安装并调用相关函数即可。chardet库支持多种字符编码,适用于大多数情况下的编码检测。
cchardet库
cchardet库是chardet库的一个快速版本,可以提高检测速度。cchardet库的使用方法与chardet库完全相同,适用于需要快速检测字符编码的场景。
charset-normalizer库
charset-normalizer库是另一个用于检测字符编码的库,特别适用于Python 3。charset-normalizer库能够提供较高的检测准确率,并支持逐块检测大文件内容的编码。
手动检测BOM头
在某些情况下,可以通过手动检测文件的BOM(Byte Order Mark)头来确定字符编码。手动检测BOM头的方法简单直观,但只能检测有限的几种字符编码。
选择合适的方法
在实际应用中,可以根据具体需求选择合适的方法进行字符编码检测。如果需要高效、准确地检测字符编码,可以优先选择chardet库或cchardet库。如果需要处理大文件,可以使用逐块读取的方法。如果文件包含BOM头,可以通过手动检测BOM头来确定字符编码。
通过对本文的学习,相信读者已经掌握了多种检测字符编码的方法,并能够在实际应用中灵活运用这些方法来解决字符编码检测的问题。
相关问答FAQs:
如何判断一个字符串的编码类型?
要判断一个字符串的编码类型,可以使用chardet
库,这是一个流行的Python库,能够自动检测字符编码。通过安装此库并调用其函数,可以获得字符串的编码信息。代码示例如下:
import chardet
rawdata = b'\xe4\xbd\xa0\xe5\xa5\xbd'
result = chardet.detect(rawdata)
print(result['encoding'])
这段代码将输出字符串的编码类型。
在Python中如何处理不同编码的字符串?
处理不同编码的字符串时,可以使用encode()
和decode()
方法。这些方法允许将字符串从一种编码转换为另一种编码。例如,可以将UTF-8编码的字符串转换为GBK编码,反之亦然。示例代码如下:
# UTF-8编码转换为GBK
utf8_string = "你好"
gbk_string = utf8_string.encode('gbk')
print(gbk_string)
# GBK编码转换回UTF-8
decoded_string = gbk_string.decode('gbk')
print(decoded_string)
如何解决编码错误的问题?
在处理字符编码时,常常会遇到编码错误。为了避免这些错误,可以在decode()
和encode()
方法中使用errors
参数,设置为'ignore'
或'replace'
,可以有效处理无法解码的字符。例如:
# 忽略错误
decoded_string = gbk_string.decode('gbk', errors='ignore')
# 替换无法解码的字符
decoded_string_with_replace = gbk_string.decode('gbk', errors='replace')
这将确保即使遇到错误,程序也能正常运行。
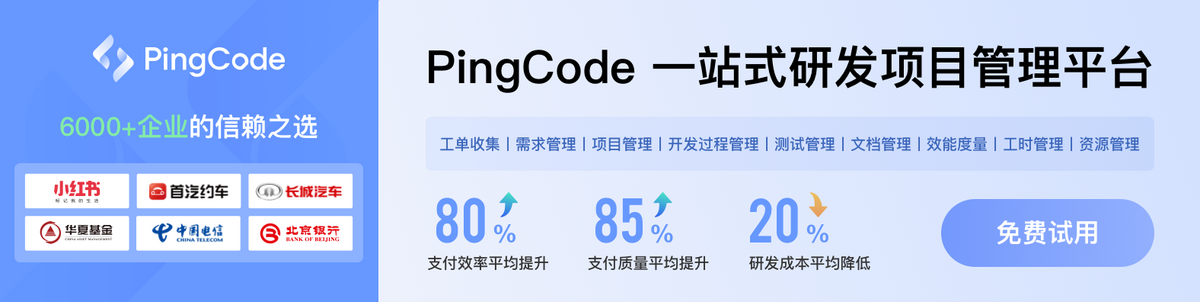