要通过Python访问Postman的接口,可以使用Python的请求库(如requests库)来发送HTTP请求。首先需要安装requests库、编写代码发送请求、处理响应数据。下面是详细的步骤和示例代码:
一、安装requests库
在开始编写代码之前,确保你已经安装了requests库。如果没有安装,可以通过以下命令安装:
pip install requests
二、编写代码发送请求
下面是一个简单的示例,展示了如何使用requests库发送GET请求和POST请求到Postman接口:
import requests
发送GET请求
def send_get_request(url, headers=None, params=None):
response = requests.get(url, headers=headers, params=params)
return response
发送POST请求
def send_post_request(url, headers=None, data=None, json=None):
response = requests.post(url, headers=headers, data=data, json=json)
return response
示例URL和headers
url = 'https://api.postman.com/collections'
headers = {
'X-Api-Key': 'YOUR_POSTMAN_API_KEY'
}
发送GET请求示例
response = send_get_request(url, headers=headers)
print('GET response status code:', response.status_code)
print('GET response body:', response.json())
发送POST请求示例
post_url = 'https://api.postman.com/collections'
post_data = {
'name': 'New Collection',
'description': 'Description of the new collection'
}
response = send_post_request(post_url, headers=headers, json=post_data)
print('POST response status code:', response.status_code)
print('POST response body:', response.json())
三、处理响应数据
在上述示例中,GET请求和POST请求的响应都保存在response对象中。可以通过response.status_code获取HTTP状态码,通过response.json()解析JSON响应体。以下是一些详细解释:
1. 解析响应数据
通过response.json()方法,可以将JSON响应体解析成Python字典或列表,方便进一步处理。
response_data = response.json()
print('Parsed JSON response:', response_data)
2. 处理错误情况
在实际应用中,可能会遇到各种错误情况,如网络问题、服务器错误等。可以通过检查HTTP状态码和捕获异常来处理这些情况。
try:
response = send_get_request(url, headers=headers)
response.raise_for_status() # 检查HTTP状态码是否为2xx
response_data = response.json()
print('Response data:', response_data)
except requests.exceptions.RequestException as e:
print('Request failed:', e)
四、使用POSTMAN生成请求代码
Postman工具非常方便,可以生成各种语言的请求代码,包括Python。可以按照以下步骤生成请求代码:
- 在Postman中配置好接口请求参数。
- 点击右上角的“Code”按钮。
- 在弹出的窗口中选择“Python – requests”。
- 将生成的代码复制到你的Python项目中。
五、应用场景示例
以下是一些应用场景示例,展示了如何使用Python与Postman接口进行交互:
1. 用户认证和授权
在调用需要认证的API接口时,可以先通过POST请求获取认证令牌,然后在后续请求中使用该令牌进行授权。
auth_url = 'https://api.postman.com/auth'
auth_data = {
'username': 'your_username',
'password': 'your_password'
}
auth_response = send_post_request(auth_url, json=auth_data)
auth_token = auth_response.json().get('token')
使用认证令牌发送GET请求
headers = {
'Authorization': f'Bearer {auth_token}'
}
response = send_get_request(url, headers=headers)
print('Authenticated GET response:', response.json())
2. 文件上传
通过POST请求将文件上传到服务器。在requests库中,可以使用files参数进行文件上传。
upload_url = 'https://api.postman.com/upload'
files = {'file': open('path/to/your/file', 'rb')}
response = requests.post(upload_url, files=files, headers=headers)
print('File upload response:', response.json())
3. 数据查询和过滤
通过GET请求向API接口发送查询参数,获取符合条件的数据。
query_params = {
'type': 'example',
'limit': 10
}
response = send_get_request(url, headers=headers, params=query_params)
print('Filtered data:', response.json())
六、深入理解HTTP请求和响应
为了更好地使用requests库与Postman接口进行交互,理解HTTP请求和响应的基本概念是非常重要的。
1. HTTP请求方法
常见的HTTP请求方法包括GET、POST、PUT、DELETE等。不同的方法用于不同的操作:
- GET:从服务器获取资源。
- POST:向服务器发送数据,通常用于创建资源。
- PUT:更新服务器上的资源。
- DELETE:删除服务器上的资源。
2. HTTP请求头
请求头包含了客户端发送给服务器的元数据,如认证信息、内容类型等。可以通过headers参数设置请求头。
headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer YOUR_TOKEN'
}
3. HTTP响应状态码
响应状态码是服务器返回的,用于表示请求的处理结果。常见的状态码包括:
- 200 OK:请求成功。
- 201 Created:成功创建资源。
- 400 Bad Request:请求无效。
- 401 Unauthorized:未授权。
- 404 Not Found:资源未找到。
- 500 Internal Server Error:服务器内部错误。
七、异常处理和重试机制
在实际应用中,网络请求可能会因为各种原因失败,如网络不稳定、服务器故障等。为了提高代码的健壮性,可以加入异常处理和重试机制。
1. 捕获异常
可以使用try-except语句捕获requests库抛出的异常,并进行相应处理。
try:
response = send_get_request(url, headers=headers)
response.raise_for_status()
except requests.exceptions.RequestException as e:
print('Request failed:', e)
2. 重试机制
可以使用第三方库如tenacity
实现重试机制,当请求失败时自动重试。
from tenacity import retry, stop_after_attempt, wait_fixed
@retry(stop=stop_after_attempt(3), wait=wait_fixed(2))
def send_request_with_retry(url, headers=None, params=None):
response = requests.get(url, headers=headers, params=params)
response.raise_for_status()
return response
try:
response = send_request_with_retry(url, headers=headers)
print('Response:', response.json())
except requests.exceptions.RequestException as e:
print('Request failed after retries:', e)
八、使用环境变量管理敏感信息
在实际开发中,不建议将敏感信息如API密钥、认证令牌等硬编码在代码中。可以使用环境变量管理这些敏感信息,确保代码的安全性和可维护性。
1. 设置环境变量
可以在系统环境中设置环境变量,或者使用.env
文件存储环境变量。
2. 读取环境变量
可以使用os
库读取环境变量,并在代码中使用。
import os
api_key = os.getenv('POSTMAN_API_KEY')
headers = {
'X-Api-Key': api_key
}
response = send_get_request(url, headers=headers)
print('Response:', response.json())
九、使用请求库进行性能优化
在高并发场景下,使用requests库进行HTTP请求可能会遇到性能瓶颈。可以通过以下方法进行性能优化:
1. 使用会话对象
使用requests.Session对象可以复用TCP连接,减少连接建立的开销。
session = requests.Session()
response = session.get(url, headers=headers)
print('Session response:', response.json())
2. 并发请求
可以使用多线程或异步编程实现并发请求,提高请求效率。
使用多线程
import threading
def fetch_data(url, headers):
response = requests.get(url, headers=headers)
print('Thread response:', response.json())
threads = []
for i in range(5):
thread = threading.Thread(target=fetch_data, args=(url, headers))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
使用异步编程
import aiohttp
import asyncio
async def fetch_data_async(session, url, headers):
async with session.get(url, headers=headers) as response:
data = await response.json()
print('Async response:', data)
async def main():
async with aiohttp.ClientSession() as session:
tasks = [fetch_data_async(session, url, headers) for _ in range(5)]
await asyncio.gather(*tasks)
asyncio.run(main())
十、总结
通过本文的介绍,我们详细讨论了如何使用Python访问Postman的接口。主要包括以下几个方面:
- 安装requests库:确保安装requests库。
- 编写代码发送请求:使用requests库发送GET和POST请求。
- 处理响应数据:解析JSON响应体,处理错误情况。
- 使用POSTMAN生成请求代码:通过Postman生成Python代码。
- 应用场景示例:用户认证、文件上传、数据查询。
- 深入理解HTTP请求和响应:理解请求方法、请求头、响应状态码。
- 异常处理和重试机制:捕获异常,实现重试机制。
- 使用环境变量管理敏感信息:确保代码的安全性和可维护性。
- 使用请求库进行性能优化:使用会话对象、并发请求。
通过以上步骤和示例代码,相信你已经掌握了如何使用Python访问Postman的接口,并能够在实际项目中灵活应用。如果有任何问题或需要进一步了解的内容,可以参考requests库的官方文档和Postman的API文档。
相关问答FAQs:
如何使用Python发送POST请求到Postman接口?
要使用Python发送POST请求到Postman接口,可以使用requests
库。首先,确保安装了该库。可以使用以下命令安装:pip install requests
。接下来,您可以使用以下代码示例:
import requests
url = '您的Postman接口URL'
data = {'key1': 'value1', 'key2': 'value2'} # 根据接口需要的参数构造数据
response = requests.post(url, json=data)
print(response.status_code)
print(response.json()) # 如果返回的是JSON格式
在Python中如何处理Postman接口的响应?
处理Postman接口的响应可以通过requests
库的响应对象轻松实现。您可以访问状态码、响应体和响应头等信息。使用response.text
获取响应内容,response.json()
可以直接将JSON格式的响应内容转换为Python字典,便于进一步处理。
我能在Python中使用哪些库来访问Postman接口?
除了requests
库,Python中还有多个库可以用于访问HTTP接口,例如http.client
、urllib
和httpx
等。http.client
是Python内置库,用于底层HTTP请求;urllib
适用于简单的URL请求;而httpx
则是一个现代化的HTTP客户端,支持异步请求,适合需要并发处理的场景。选择适合您需求的库将帮助您更高效地与Postman接口进行交互。
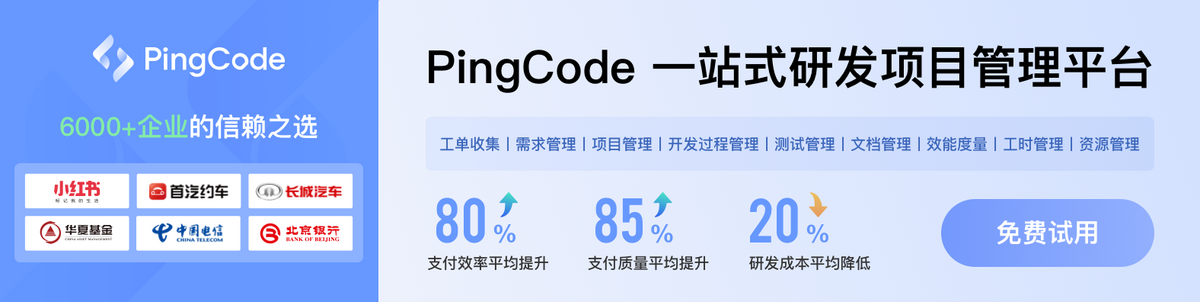