在Python中,实现选择函数有多种方法,常用的包括定义函数、使用lambda表达式、使用选择结构(如if-elif-else)、利用字典映射等。下面将详细介绍其中的一点——使用选择结构(if-elif-else)来实现选择函数,并且会逐步讲解其他方法。
一、定义函数
定义函数是Python中实现选择函数的基本方法之一。通过定义一个函数,可以将选择逻辑封装在函数内部,根据传入的参数执行不同的操作。
def choose_function(option):
if option == 1:
return function1()
elif option == 2:
return function2()
elif option == 3:
return function3()
else:
return "Invalid option"
def function1():
return "Function 1 executed"
def function2():
return "Function 2 executed"
def function3():
return "Function 3 executed"
Example usage
result = choose_function(2)
print(result) # Output: Function 2 executed
在上面的代码中,choose_function
函数根据传入的 option
参数选择执行不同的函数 function1
、function2
或 function3
,并返回相应的结果。
二、使用lambda表达式
Lambda表达式是一种简洁的方式来定义匿名函数。通过结合lambda表达式和选择结构,可以实现选择函数。
def choose_function(option):
functions = {
1: lambda: "Function 1 executed",
2: lambda: "Function 2 executed",
3: lambda: "Function 3 executed"
}
return functions.get(option, lambda: "Invalid option")()
Example usage
result = choose_function(2)
print(result) # Output: Function 2 executed
在这个例子中,使用字典将选项映射到相应的lambda表达式,并通过调用字典的 get
方法来实现选择函数。
三、使用选择结构(if-elif-else)
使用选择结构(if-elif-else)是实现选择函数的常用方法之一。通过在函数内部使用if-elif-else语句,可以根据传入的参数选择执行不同的操作。
def choose_function(option):
if option == 1:
return "Function 1 executed"
elif option == 2:
return "Function 2 executed"
elif option == 3:
return "Function 3 executed"
else:
return "Invalid option"
Example usage
result = choose_function(2)
print(result) # Output: Function 2 executed
在这个例子中,choose_function
函数使用if-elif-else语句来选择执行不同的操作,并返回相应的结果。
详细描述:使用选择结构(if-elif-else)
使用选择结构(if-elif-else)是实现选择函数最直观和易于理解的方法之一。它的优点在于代码清晰明了,易于阅读和维护。通过在函数内部使用if-elif-else语句,可以根据传入的参数选择执行不同的操作。
优点:
- 清晰易读:if-elif-else语句结构简单,逻辑清晰,易于阅读和理解。
- 易于维护:代码结构直观,修改和扩展方便,可以根据需要添加新的条件分支。
- 通用性强:适用于各种条件选择场景,不受具体实现方式的限制。
缺点:
- 代码冗长:当选择条件较多时,if-elif-else语句会显得比较冗长,影响代码简洁性。
- 性能问题:在选择条件较多的情况下,if-elif-else语句的执行效率可能会受到影响,因为每个条件都需要逐一判断。
使用场景:
if-elif-else语句适用于选择条件较少,逻辑简单的场景。例如,根据用户输入选择执行不同的操作,或者根据状态值选择不同的处理逻辑。
def choose_function(option):
if option == 1:
return "Function 1 executed"
elif option == 2:
return "Function 2 executed"
elif option == 3:
return "Function 3 executed"
else:
return "Invalid option"
Example usage
result = choose_function(2)
print(result) # Output: Function 2 executed
在这个例子中,choose_function
函数使用if-elif-else语句来选择执行不同的操作,并返回相应的结果。通过传入不同的参数,可以选择执行不同的函数逻辑。
四、利用字典映射
利用字典映射是一种高效且简洁的方法来实现选择函数。通过将选项映射到相应的函数,可以避免冗长的if-elif-else语句,提高代码的可维护性和执行效率。
def function1():
return "Function 1 executed"
def function2():
return "Function 2 executed"
def function3():
return "Function 3 executed"
def choose_function(option):
functions = {
1: function1,
2: function2,
3: function3
}
return functions.get(option, lambda: "Invalid option")()
Example usage
result = choose_function(2)
print(result) # Output: Function 2 executed
在这个例子中,使用字典将选项映射到相应的函数,并通过调用字典的 get
方法来实现选择函数。如果传入的参数不在字典中,则返回默认的lambda表达式。
详细描述:利用字典映射
利用字典映射是实现选择函数的一种高效且简洁的方法。通过将选项映射到相应的函数,可以避免冗长的if-elif-else语句,提高代码的可维护性和执行效率。
优点:
- 代码简洁:利用字典映射可以避免冗长的if-elif-else语句,使代码更加简洁易读。
- 高效执行:通过字典查找可以快速定位到相应的函数,提高执行效率。
- 易于扩展:添加新的选项和对应的函数只需在字典中添加新的键值对,方便扩展和维护。
缺点:
- 适用范围有限:字典映射适用于选项和函数之间存在直接对应关系的场景,不适用于复杂逻辑选择。
使用场景:
字典映射适用于选项和函数之间存在直接对应关系,且选择条件较多的场景。例如,根据用户输入选择执行不同的操作函数,或者根据状态值选择不同的处理函数。
def function1():
return "Function 1 executed"
def function2():
return "Function 2 executed"
def function3():
return "Function 3 executed"
def choose_function(option):
functions = {
1: function1,
2: function2,
3: function3
}
return functions.get(option, lambda: "Invalid option")()
Example usage
result = choose_function(2)
print(result) # Output: Function 2 executed
在这个例子中,使用字典将选项映射到相应的函数,并通过调用字典的 get
方法来实现选择函数。如果传入的参数不在字典中,则返回默认的lambda表达式。
五、使用类和方法
在面向对象编程(OOP)中,可以通过使用类和方法来实现选择函数。将不同的功能实现为类的方法,并通过实例化对象调用相应的方法来实现选择函数。
class FunctionChooser:
def function1(self):
return "Function 1 executed"
def function2(self):
return "Function 2 executed"
def function3(self):
return "Function 3 executed"
def choose_function(self, option):
if option == 1:
return self.function1()
elif option == 2:
return self.function2()
elif option == 3:
return self.function3()
else:
return "Invalid option"
Example usage
chooser = FunctionChooser()
result = chooser.choose_function(2)
print(result) # Output: Function 2 executed
在这个例子中,FunctionChooser
类包含了三个方法 function1
、function2
和 function3
,以及一个选择函数方法 choose_function
。通过实例化 FunctionChooser
类的对象,可以选择调用不同的方法。
详细描述:使用类和方法
使用类和方法是实现选择函数的一种面向对象的方式。通过将不同的功能实现为类的方法,并通过实例化对象调用相应的方法来实现选择函数。
优点:
- 结构清晰:将功能封装在类中,结构清晰,代码组织更加合理。
- 可扩展性强:可以通过继承和多态实现功能扩展和重用,提高代码的可维护性。
- 面向对象:符合面向对象编程的思想,通过类和对象实现选择函数。
缺点:
- 复杂性增加:相对于简单的函数实现,使用类和方法会增加代码的复杂性。
- 性能开销:创建类的实例会带来一定的性能开销,适用于需要面向对象设计的场景。
使用场景:
使用类和方法适用于需要面向对象设计的复杂应用场景。例如,需要封装多个功能,并通过对象调用相应的方法来实现选择函数。
class FunctionChooser:
def function1(self):
return "Function 1 executed"
def function2(self):
return "Function 2 executed"
def function3(self):
return "Function 3 executed"
def choose_function(self, option):
if option == 1:
return self.function1()
elif option == 2:
return self.function2()
elif option == 3:
return self.function3()
else:
return "Invalid option"
Example usage
chooser = FunctionChooser()
result = chooser.choose_function(2)
print(result) # Output: Function 2 executed
在这个例子中,FunctionChooser
类包含了三个方法 function1
、function2
和 function3
,以及一个选择函数方法 choose_function
。通过实例化 FunctionChooser
类的对象,可以选择调用不同的方法。
六、使用策略模式
策略模式是一种行为设计模式,通过将不同的算法封装在独立的类中,并通过上下文类来选择和执行相应的算法。可以使用策略模式来实现选择函数。
from abc import ABC, abstractmethod
class Strategy(ABC):
@abstractmethod
def execute(self):
pass
class Function1(Strategy):
def execute(self):
return "Function 1 executed"
class Function2(Strategy):
def execute(self):
return "Function 2 executed"
class Function3(Strategy):
def execute(self):
return "Function 3 executed"
class FunctionChooser:
def __init__(self):
self.strategies = {
1: Function1(),
2: Function2(),
3: Function3()
}
def choose_function(self, option):
strategy = self.strategies.get(option)
if strategy:
return strategy.execute()
else:
return "Invalid option"
Example usage
chooser = FunctionChooser()
result = chooser.choose_function(2)
print(result) # Output: Function 2 executed
在这个例子中,通过使用策略模式,将不同的功能封装在独立的策略类中,并通过上下文类 FunctionChooser
来选择和执行相应的策略。
详细描述:使用策略模式
策略模式是一种行为设计模式,通过将不同的算法封装在独立的类中,并通过上下文类来选择和执行相应的算法。可以使用策略模式来实现选择函数。
优点:
- 灵活扩展:可以方便地添加新的策略类,而不需要修改已有的代码,符合开闭原则。
- 代码复用:不同的策略类可以复用相同的接口,减少代码重复,提高代码复用性。
- 清晰结构:将不同的算法封装在独立的类中,结构清晰,易于理解和维护。
缺点:
- 类增多:策略模式会增加类的数量,可能会带来一定的代码复杂性。
- 接口依赖:需要定义和实现统一的策略接口,增加了一定的设计和实现成本。
使用场景:
策略模式适用于算法或行为需要在运行时动态选择的场景。例如,根据不同的条件选择不同的处理算法,或者在不同的上下文中执行不同的策略。
from abc import ABC, abstractmethod
class Strategy(ABC):
@abstractmethod
def execute(self):
pass
class Function1(Strategy):
def execute(self):
return "Function 1 executed"
class Function2(Strategy):
def execute(self):
return "Function 2 executed"
class Function3(Strategy):
def execute(self):
return "Function 3 executed"
class FunctionChooser:
def __init__(self):
self.strategies = {
1: Function1(),
2: Function2(),
3: Function3()
}
def choose_function(self, option):
strategy = self.strategies.get(option)
if strategy:
return strategy.execute()
else:
return "Invalid option"
Example usage
chooser = FunctionChooser()
result = chooser.choose_function(2)
print(result) # Output: Function 2 executed
在这个例子中,通过使用策略模式,将不同的功能封装在独立的策略类中,并通过上下文类 FunctionChooser
来选择和执行相应的策略。
七、使用装饰器模式
装饰器模式是一种结构性设计模式,通过动态地向对象添加新的行为,可以在不改变对象结构的情况下增强对象的功能。可以使用装饰器模式来实现选择函数。
class Function:
def execute(self):
return "Base Function executed"
class FunctionDecorator(Function):
def __init__(self, function):
self._function = function
def execute(self):
return self._function.execute()
class Function1Decorator(FunctionDecorator):
def execute(self):
return "Function 1 executed"
class Function2Decorator(FunctionDecorator):
def execute(self):
return "Function 2 executed"
class Function3Decorator(FunctionDecorator):
def execute(self):
return "Function 3 executed"
class FunctionChooser:
def choose_function(self, option):
function = Function()
if option == 1:
function = Function1Decorator(function)
elif option == 2:
function = Function2Decorator(function)
elif option == 3:
function = Function3Decorator(function)
else:
return "Invalid option"
return function.execute()
Example usage
chooser = FunctionChooser()
result = chooser.choose_function(2)
print(result) # Output: Function 2 executed
在这个例子中,通过使用装饰器模式,动态地向 Function
对象添加新的行为,并通过 FunctionChooser
类选择相应的装饰器来实现选择函数。
详细描述:使用装饰器模式
装饰器模式是一种结构性设计模式,通过动态地向对象添加新的行为,可以在不改变对象结构的情况下增强对象的功能。可以使用装饰器模式来实现选择函数。
优点:
- 动态扩展:可以在运行时动态地向对象添加新的行为,而不需要修改对象的结构。
- 灵活组合:不同的装饰器可以灵活组合,增强对象的功能,提供更强的灵活性和可扩展性。
- 符合开闭原则:可以通过添加新的装饰器类来扩展功能,而不需要修改已有的代码,符合开闭原则。
缺点:
- 类增多:装饰器模式会增加类的数量,可能会带来一定的代码复杂性。
- 调试困难:由于装饰器是动态地添加行为,调试和排查问题可能会比较困难。
使用场景:
装饰器模式适用于需要动态地向对象添加新的行为,或者需要灵活组合对象功能的场景。例如,根据不同的条件动态地增强对象的功能,或者在不同的上下文中组合不同的行为。
class Function:
def execute(self):
return "Base Function executed"
class FunctionDecorator(Function):
def __init__(self, function):
self._function = function
def execute(self):
return self._function.execute()
class Function1Decorator(FunctionDecorator):
def execute(self):
return "Function 1 executed"
class Function2Decorator(FunctionDecorator):
def execute(self):
return "Function 2 executed"
class Function3Decorator(FunctionDecorator):
def execute(self):
相关问答FAQs:
如何在Python中定义一个选择函数?
在Python中,选择函数通常是指根据特定条件从一组选项中选择一个或多个元素。可以通过使用if
语句、lambda
函数或列表推导来定义选择函数。例如,使用if
语句可以根据条件判断返回不同的值,使用列表推导可以在满足条件时快速筛选出符合要求的元素。
Python中选择函数的应用场景有哪些?
选择函数在数据处理、决策分析和用户交互等场景中应用广泛。例如,在数据分析中,可以使用选择函数从数据集中选择特定的行或列;在游戏开发中,选择函数可以用于根据玩家的选择来决定剧情走向;在机器学习中,选择函数可以帮助模型在不同的特征中进行选择。
如何使用Python的内置函数来实现选择功能?
Python提供了一些内置函数,如filter()
、map()
和reduce()
,可以帮助实现选择功能。使用filter()
可以根据条件从列表中筛选出符合要求的元素,而map()
则可以对列表中的每个元素应用一个函数来生成新列表。这些函数结合选择逻辑,可以高效地处理数据。
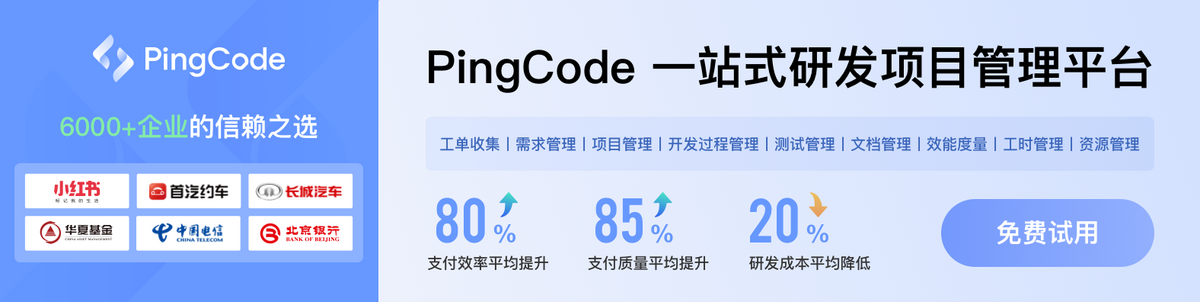