在python中操作mysql的步骤包括:安装MySQL连接器、连接MySQL数据库、执行SQL查询、处理查询结果、关闭连接。这些步骤可以帮助开发者实现Python与MySQL的交互,从而进行数据存储、查询、更新和删除等操作。
其中,安装MySQL连接器是最基础也是最重要的一步。Python与MySQL的连接需要借助MySQL的连接器库,比如mysql-connector-python
或PyMySQL
。通过pip命令可以轻松安装这些库,如pip install mysql-connector-python
。安装完成后,便可以在Python代码中导入并使用这些库来进行数据库操作。
以下是详细介绍每个步骤的内容:
一、安装MySQL连接器
要在Python中与MySQL交互,首先需要安装一个MySQL的连接器库。常用的连接器有mysql-connector-python
和PyMySQL
。可以通过以下命令进行安装:
pip install mysql-connector-python
或者:
pip install pymysql
二、连接MySQL数据库
安装连接器库后,可以通过Python脚本连接到MySQL数据库。以下是使用mysql-connector-python
连接MySQL数据库的示例:
import mysql.connector
创建数据库连接
conn = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
创建一个游标对象
cursor = conn.cursor()
三、执行SQL查询
连接成功后,可以执行各种SQL查询,包括SELECT、INSERT、UPDATE、DELETE等。以下是一些示例:
执行SELECT查询:
# 执行SQL查询
cursor.execute("SELECT * FROM yourtable")
获取所有结果
results = cursor.fetchall()
遍历结果
for row in results:
print(row)
执行INSERT查询:
# 执行SQL查询
sql = "INSERT INTO yourtable (column1, column2) VALUES (%s, %s)"
val = ("value1", "value2")
cursor.execute(sql, val)
提交到数据库执行
conn.commit()
print(cursor.rowcount, "record inserted.")
执行UPDATE查询:
# 执行SQL查询
sql = "UPDATE yourtable SET column1 = %s WHERE column2 = %s"
val = ("new_value", "value2")
cursor.execute(sql, val)
提交到数据库执行
conn.commit()
print(cursor.rowcount, "record(s) affected")
执行DELETE查询:
# 执行SQL查询
sql = "DELETE FROM yourtable WHERE column1 = %s"
val = ("value1",)
cursor.execute(sql, val)
提交到数据库执行
conn.commit()
print(cursor.rowcount, "record(s) deleted")
四、处理查询结果
查询结果可以通过游标对象的fetchall()
方法获取,返回值是一个包含所有结果的列表。每个结果也是一个元组,表示数据库表中的一行记录。
# 获取所有结果
results = cursor.fetchall()
遍历结果
for row in results:
print(row)
五、关闭连接
操作完成后,记得关闭游标和数据库连接,以释放资源。
# 关闭游标
cursor.close()
关闭数据库连接
conn.close()
六、错误处理
在进行数据库操作时,可能会遇到各种错误,如连接失败、SQL语法错误等。可以使用try-except语句进行错误处理,以提高程序的健壮性。
import mysql.connector
from mysql.connector import Error
try:
conn = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
if conn.is_connected():
print("Successfully connected to the database")
cursor = conn.cursor()
cursor.execute("SELECT * FROM yourtable")
results = cursor.fetchall()
for row in results:
print(row)
except Error as e:
print("Error while connecting to MySQL", e)
finally:
if conn.is_connected():
cursor.close()
conn.close()
print("MySQL connection is closed")
七、使用ORM工具
除了直接使用MySQL连接器库操作数据库,还可以使用ORM(对象关系映射)工具,如SQLAlchemy,它提供了更高级的接口,使数据库操作更加简便。
安装SQLAlchemy:
pip install sqlalchemy
使用SQLAlchemy连接MySQL:
from sqlalchemy import create_engine
from sqlalchemy.orm import sessionmaker
创建数据库引擎
engine = create_engine('mysql+mysqlconnector://yourusername:yourpassword@localhost/yourdatabase')
创建会话
Session = sessionmaker(bind=engine)
session = Session()
定义映射类
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy import Column, Integer, String
Base = declarative_base()
class YourTable(Base):
__tablename__ = 'yourtable'
id = Column(Integer, primary_key=True)
column1 = Column(String(50))
column2 = Column(String(50))
查询数据
results = session.query(YourTable).all()
for row in results:
print(row.column1, row.column2)
关闭会话
session.close()
八、事务管理
在进行多条SQL操作时,可以使用事务管理,以确保操作的原子性。可以使用conn.start_transaction()
、conn.commit()
和conn.rollback()
方法进行事务管理。
try:
conn.start_transaction()
# 执行多条SQL操作
cursor.execute("INSERT INTO yourtable (column1, column2) VALUES (%s, %s)", ("value1", "value2"))
cursor.execute("UPDATE yourtable SET column1 = %s WHERE column2 = %s", ("new_value", "value2"))
# 提交事务
conn.commit()
except Error as e:
print("Transaction failed, rolling back", e)
conn.rollback()
finally:
cursor.close()
conn.close()
通过以上步骤和示例,可以在Python中实现对MySQL数据库的操作,包括连接、查询、插入、更新、删除、事务管理和错误处理等。希望这些内容对您有所帮助。
相关问答FAQs:
在Python中如何连接MySQL数据库?
要连接MySQL数据库,您需要安装MySQL驱动程序,常用的有mysql-connector-python
和PyMySQL
。安装后,可以使用以下代码进行连接:
import mysql.connector
connection = mysql.connector.connect(
host='localhost',
user='your_username',
password='your_password',
database='your_database'
)
if connection.is_connected():
print("成功连接到数据库")
确保替换your_username
、your_password
和your_database
为实际的数据库凭据和数据库名称。
在Python中如何执行SQL查询?
执行SQL查询需要通过连接对象创建一个游标,然后利用游标执行SQL语句。例如,下面的代码演示了如何执行一个简单的SELECT查询:
cursor = connection.cursor()
cursor.execute("SELECT * FROM your_table")
for row in cursor.fetchall():
print(row)
cursor.close()
在执行其他类型的SQL操作(如INSERT、UPDATE和DELETE)时,可以使用类似的方式,只需将SQL语句更改为相应的操作即可。
如何处理Python中MySQL数据库的异常?
在进行数据库操作时,处理异常是非常重要的。可以使用try...except
结构来捕获异常并进行相应处理。以下是一个示例:
try:
connection = mysql.connector.connect(...)
cursor = connection.cursor()
cursor.execute("INSERT INTO your_table (column1) VALUES ('value')")
connection.commit()
except mysql.connector.Error as err:
print(f"数据库错误: {err}")
finally:
if connection.is_connected():
cursor.close()
connection.close()
这种方式能确保在出现错误时,能够及时捕获并处理,从而避免程序崩溃。
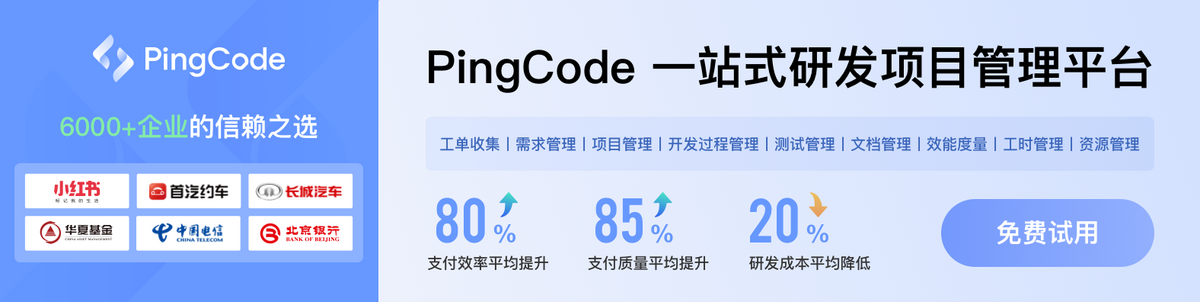