Python编写淘宝详情的步骤一般包括使用爬虫技术获取详情数据、解析数据、数据清洗与处理、将数据存储到合适的数据结构中、使用模板生成淘宝详情页面等。我们可以通过示例代码来更详细地展示其中的一个步骤,即使用爬虫技术获取淘宝详情数据,并解析这些数据。
一、使用爬虫技术获取详情数据
爬虫技术的核心在于模拟浏览器请求网页,并获取网页中的数据。常用的库有 requests
和 BeautifulSoup
。首先我们需要发送请求获取网页内容,然后解析网页内容。
import requests
from bs4 import BeautifulSoup
设置请求头,模拟浏览器请求
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
商品详情页URL
url = 'https://detail.tmall.com/item.htm?id=XXXXX'
发送请求获取网页内容
response = requests.get(url, headers=headers)
html_content = response.text
解析网页内容
soup = BeautifulSoup(html_content, 'html.parser')
获取商品标题
title = soup.find('div', {'class': 'tb-detail-hd'}).find('h1').text.strip()
print(f'商品标题: {title}')
获取商品价格
price = soup.find('span', {'class': 'tm-price'}).text.strip()
print(f'商品价格: {price}')
获取商品图片
image_urls = [img['src'] for img in soup.find_all('img', {'class': 'J_ItemPic'})]
print(f'商品图片: {image_urls}')
二、解析数据
在获取到网页的原始HTML内容后,需要提取有用的信息。使用 BeautifulSoup
可以方便地解析HTML结构,提取特定的元素。例如,上面的代码展示了如何提取商品的标题、价格和图片链接。
三、数据清洗与处理
在解析到数据后,可能需要对数据进行清洗和处理,以确保数据的准确性和格式的规范性。例如,需要去除多余的空格,转换数据格式等。
import re
清洗价格数据,去除非数字字符
cleaned_price = re.sub(r'[^\d.]+', '', price)
print(f'清洗后的价格: {cleaned_price}')
清洗图片URL,补全缺失的域名部分
cleaned_image_urls = [f'https:{url}' if url.startswith('//') else url for url in image_urls]
print(f'清洗后的图片URL: {cleaned_image_urls}')
四、将数据存储到合适的数据结构中
将清洗后的数据存储到合适的数据结构中,以便后续使用。例如,可以使用字典来存储商品详情。
item_details = {
'title': title,
'price': cleaned_price,
'images': cleaned_image_urls
}
print(item_details)
五、使用模板生成淘宝详情页面
最后一步是将提取到的商品详情数据,使用模板生成淘宝详情页面。可以使用 Jinja2
模板引擎来生成HTML页面。
from jinja2 import Template
定义HTML模板
html_template = """
<!DOCTYPE html>
<html>
<head>
<title>{{ title }}</title>
</head>
<body>
<h1>{{ title }}</h1>
<p>价格: {{ price }}</p>
<div>
{% for image in images %}
<img src="{{ image }}" alt="商品图片">
{% endfor %}
</div>
</body>
</html>
"""
使用模板生成HTML页面
template = Template(html_template)
html_content = template.render(title=item_details['title'], price=item_details['price'], images=item_details['images'])
保存HTML内容到文件
with open('item_details.html', 'w', encoding='utf-8') as file:
file.write(html_content)
通过以上几个步骤,我们可以使用Python编写淘宝详情页面。每一步都需要注意数据的准确性和完整性,确保最终生成的详情页面能够正确展示商品信息。
六、完整代码示例
下面是一个完整的代码示例,将上述步骤整合起来,实现从获取淘宝详情数据到生成详情页面的全过程。
import requests
from bs4 import BeautifulSoup
import re
from jinja2 import Template
设置请求头,模拟浏览器请求
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
商品详情页URL
url = 'https://detail.tmall.com/item.htm?id=XXXXX'
发送请求获取网页内容
response = requests.get(url, headers=headers)
html_content = response.text
解析网页内容
soup = BeautifulSoup(html_content, 'html.parser')
获取商品标题
title = soup.find('div', {'class': 'tb-detail-hd'}).find('h1').text.strip()
获取商品价格
price = soup.find('span', {'class': 'tm-price'}).text.strip()
获取商品图片
image_urls = [img['src'] for img in soup.find_all('img', {'class': 'J_ItemPic'})]
清洗价格数据,去除非数字字符
cleaned_price = re.sub(r'[^\d.]+', '', price)
清洗图片URL,补全缺失的域名部分
cleaned_image_urls = [f'https:{url}' if url.startswith('//') else url for url in image_urls]
将数据存储到字典中
item_details = {
'title': title,
'price': cleaned_price,
'images': cleaned_image_urls
}
定义HTML模板
html_template = """
<!DOCTYPE html>
<html>
<head>
<title>{{ title }}</title>
</head>
<body>
<h1>{{ title }}</h1>
<p>价格: {{ price }}</p>
<div>
{% for image in images %}
<img src="{{ image }}" alt="商品图片">
{% endfor %}
</div>
</body>
</html>
"""
使用模板生成HTML页面
template = Template(html_template)
html_content = template.render(title=item_details['title'], price=item_details['price'], images=item_details['images'])
保存HTML内容到文件
with open('item_details.html', 'w', encoding='utf-8') as file:
file.write(html_content)
通过这个完整的代码示例,我们可以看到如何使用Python从获取淘宝详情数据到生成详情页面的全过程。这些步骤包括发送请求获取网页内容、解析网页内容、清洗和处理数据、将数据存储到字典中、使用模板生成HTML页面等。每一步都非常重要,只有确保每一步都正确执行,才能生成一个完整且准确的淘宝详情页面。
相关问答FAQs:
如何使用Python自动生成淘宝商品详情?
利用Python的爬虫技术,可以从已有的商品详情中提取信息并自动生成新的商品详情。通过使用BeautifulSoup或Scrapy等库,可以抓取网页内容,解析HTML结构,从而提取出商品的图片、标题、描述等信息,进而组合成符合淘宝格式的详情页。
使用Python编写淘宝商品详情时,有哪些需要注意的事项?
在编写商品详情时,应确保内容的原创性,避免直接复制他人内容。还需注意淘宝的内容规范,包括禁止使用的关键词、图片格式及大小要求等。此外,优化商品详情的SEO也是重要的一环,使用合适的关键词能够提升商品在搜索中的排名。
Python生成的淘宝详情如何进行美化和优化?
可以利用Python中的图形处理库如Pillow,来处理商品图片,实现缩放、剪裁等功能。同时,使用Markdown或HTML格式来组织文本内容,使其更具可读性和吸引力。通过合理的排版和配色,能够提升顾客的购物体验,从而增加转化率。
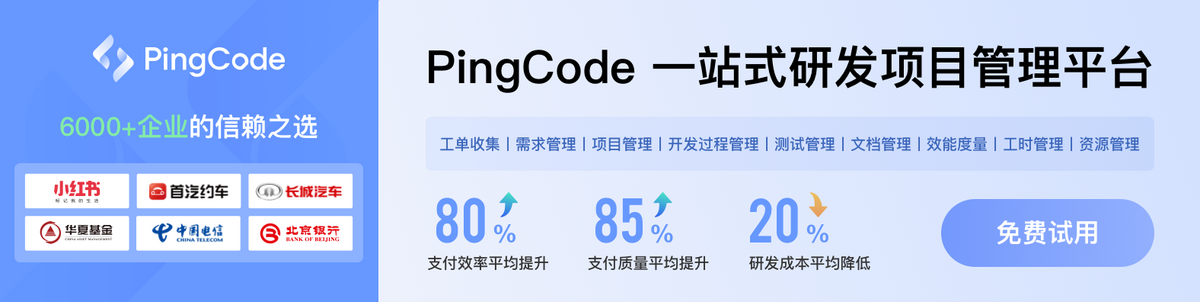