Python逐个读取HTML网址
Python逐个读取HTML网址的方法包括使用requests库、BeautifulSoup进行解析、处理HTTP请求异常。我们可以通过requests库来发送HTTP请求,获取网页的HTML内容,然后使用BeautifulSoup来解析和提取我们需要的数据。requests库处理HTTP请求异常非常重要,因为它可以帮助我们处理网络问题或者目标网址不存在的情况。下面我将详细描述如何使用requests库和BeautifulSoup逐个读取HTML网址。
一、使用requests库发送HTTP请求
requests库是Python中用于发送HTTP请求的流行库。它简单易用,可以发送GET、POST等各种HTTP请求,并能处理Cookies、会话等功能。以下是一个基本的使用requests库发送GET请求的示例:
import requests
url = 'http://example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
print(html_content)
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
在这个示例中,我们首先导入requests库,然后使用requests.get()
方法发送GET请求。如果请求成功(状态码为200),则获取网页的HTML内容并打印出来。如果请求失败,则打印失败的状态码。
二、使用BeautifulSoup解析HTML内容
BeautifulSoup是一个用于解析HTML和XML文档的Python库。它可以方便地从HTML文档中提取数据。以下是一个使用BeautifulSoup解析HTML内容的示例:
from bs4 import BeautifulSoup
html_content = '''<html>
<head><title>Example Page</title></head>
<body><p>This is an example page.</p></body>
</html>'''
soup = BeautifulSoup(html_content, 'html.parser')
title = soup.title.text
paragraph = soup.p.text
print(f"Title: {title}")
print(f"Paragraph: {paragraph}")
在这个示例中,我们首先导入BeautifulSoup库,然后创建一个包含HTML内容的字符串。使用BeautifulSoup()
方法解析HTML内容,接着通过访问soup.title.text
和soup.p.text
获取网页的标题和段落内容。
三、处理HTTP请求异常
在实际应用中,处理HTTP请求异常是非常重要的。requests库提供了一些异常处理机制,可以帮助我们捕获和处理各种HTTP请求异常。以下是一个处理HTTP请求异常的示例:
import requests
url = 'http://example.com'
try:
response = requests.get(url)
response.raise_for_status()
except requests.exceptions.HTTPError as http_err:
print(f"HTTP error occurred: {http_err}")
except Exception as err:
print(f"Other error occurred: {err}")
else:
html_content = response.text
print(html_content)
在这个示例中,我们使用try-except
结构来捕获HTTP请求异常。如果发生HTTP错误,则打印错误信息。如果发生其他错误,也会打印相应的错误信息。如果请求成功,则打印网页的HTML内容。
四、逐个读取多个HTML网址
要逐个读取多个HTML网址,我们可以将这些网址存储在一个列表中,并使用循环来逐个读取。以下是一个逐个读取多个HTML网址的示例:
import requests
from bs4 import BeautifulSoup
urls = [
'http://example.com',
'http://example.org',
'http://example.net'
]
for url in urls:
try:
response = requests.get(url)
response.raise_for_status()
except requests.exceptions.HTTPError as http_err:
print(f"HTTP error occurred while fetching {url}: {http_err}")
except Exception as err:
print(f"Other error occurred while fetching {url}: {err}")
else:
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
title = soup.title.text if soup.title else 'No title'
print(f"URL: {url}")
print(f"Title: {title}")
print("\n")
在这个示例中,我们首先定义一个包含多个网址的列表,然后使用for
循环逐个读取这些网址。在循环中,我们使用requests库发送HTTP请求,并处理可能发生的异常。如果请求成功,则使用BeautifulSoup解析HTML内容,并打印网页的标题。
五、保存解析结果
在实际应用中,我们可能需要将解析结果保存到文件中,以便后续处理。以下是一个将解析结果保存到文件的示例:
import requests
from bs4 import BeautifulSoup
urls = [
'http://example.com',
'http://example.org',
'http://example.net'
]
with open('output.txt', 'w') as file:
for url in urls:
try:
response = requests.get(url)
response.raise_for_status()
except requests.exceptions.HTTPError as http_err:
file.write(f"HTTP error occurred while fetching {url}: {http_err}\n")
except Exception as err:
file.write(f"Other error occurred while fetching {url}: {err}\n")
else:
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
title = soup.title.text if soup.title else 'No title'
file.write(f"URL: {url}\n")
file.write(f"Title: {title}\n")
file.write("\n")
在这个示例中,我们使用with open()
语句打开一个文件,并在循环中将解析结果写入文件。这样可以将所有解析结果保存到文件中,方便后续处理。
六、处理动态网页
有些网页的内容是通过JavaScript动态加载的,使用requests库无法直接获取这些内容。对于这种情况,我们可以使用Selenium库来模拟浏览器行为,从而获取动态加载的内容。以下是一个使用Selenium获取动态网页内容的示例:
from selenium import webdriver
from bs4 import BeautifulSoup
url = 'http://example.com'
driver = webdriver.Chrome()
driver.get(url)
html_content = driver.page_source
soup = BeautifulSoup(html_content, 'html.parser')
title = soup.title.text if soup.title else 'No title'
print(f"Title: {title}")
driver.quit()
在这个示例中,我们首先导入Selenium的webdriver模块,然后使用webdriver.Chrome()
创建一个Chrome浏览器实例。使用driver.get()
方法打开目标网址,并通过driver.page_source
获取网页的HTML内容。接下来,我们使用BeautifulSoup解析HTML内容,并打印网页的标题。最后,我们使用driver.quit()
关闭浏览器。
七、并发处理多个网址
如果需要处理大量网址,逐个读取可能会比较慢。我们可以使用并发编程来提高效率。以下是一个使用concurrent.futures库并发处理多个网址的示例:
import requests
from bs4 import BeautifulSoup
from concurrent.futures import ThreadPoolExecutor
urls = [
'http://example.com',
'http://example.org',
'http://example.net'
]
def fetch_url(url):
try:
response = requests.get(url)
response.raise_for_status()
except requests.exceptions.HTTPError as http_err:
return f"HTTP error occurred while fetching {url}: {http_err}"
except Exception as err:
return f"Other error occurred while fetching {url}: {err}"
else:
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
title = soup.title.text if soup.title else 'No title'
return f"URL: {url}\nTitle: {title}\n"
with ThreadPoolExecutor(max_workers=5) as executor:
results = executor.map(fetch_url, urls)
for result in results:
print(result)
在这个示例中,我们首先定义一个fetch_url
函数,用于发送HTTP请求并解析HTML内容。然后使用ThreadPoolExecutor
创建一个线程池,并发处理多个网址。使用executor.map()
方法将fetch_url
函数应用于所有网址,并获取结果。最后,我们打印所有结果。
八、总结
通过上述步骤,我们可以使用Python逐个读取HTML网址,解析网页内容,并处理可能发生的异常。requests库和BeautifulSoup是处理静态网页的常用工具,而Selenium可以处理动态网页。对于大量网址,可以使用并发编程提高效率。希望这篇文章对你有所帮助。
相关库的安装
在使用requests、BeautifulSoup和Selenium等库之前,需要先安装它们。以下是安装这些库的命令:
pip install requests
pip install beautifulsoup4
pip install selenium
进一步优化
在实际应用中,我们可以根据具体需求进一步优化上述代码。例如,可以将解析结果存储到数据库中,或者使用更高级的数据处理和分析工具。希望你能根据自己的需求,灵活运用这些技术。
相关问答FAQs:
如何使用Python读取HTML网址中的内容?
在Python中,可以使用requests
库来发送HTTP请求,获取HTML页面的内容。通过BeautifulSoup
库,可以方便地解析HTML代码,提取所需信息。以下是一个简单的示例:
import requests
from bs4 import BeautifulSoup
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
print(soup.prettify())
这个代码将获取指定网址的HTML内容,并以易于阅读的格式输出。
我需要安装哪些库才能读取HTML网址?
要逐个读取HTML网址,常用的库包括requests
和BeautifulSoup
。可以使用以下命令通过pip
进行安装:
pip install requests beautifulsoup4
确保在使用这些库之前,Python环境已经设置好并且网络连接正常。
如何处理读取HTML网址时可能出现的错误?
在读取HTML网址时,可能会遇到各种错误,比如网络连接问题或URL格式错误。可以使用try-except
语句来处理这些异常情况。示例如下:
try:
response = requests.get(url)
response.raise_for_status() # 检查请求是否成功
except requests.exceptions.RequestException as e:
print(f"请求错误:{e}")
这种方式能够帮助你捕获并处理错误,确保程序的稳定运行。
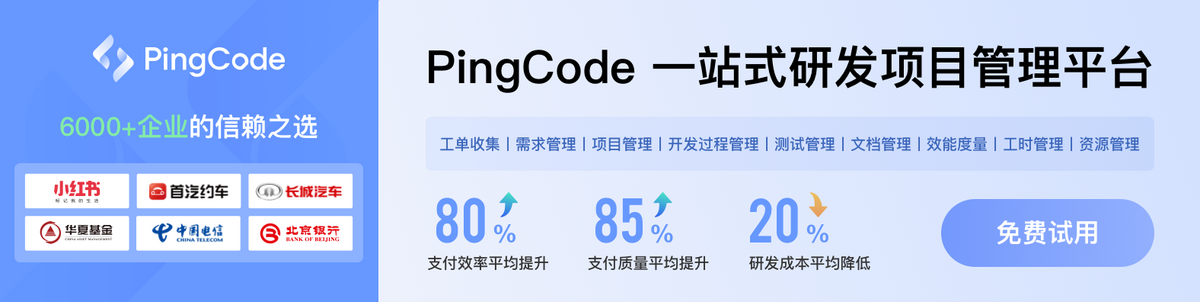