在Python中,输出小数的方式有多种,可以使用内置的字符串格式化函数、f字符串、format()方法。其中,f字符串是一种较为简洁和直观的方法。下面将详细介绍f字符串的使用方法。
f字符串在Python 3.6及以上版本中引入,它允许在字符串中嵌入表达式,并以花括号{}表示嵌入的表达式。例如:
value = 3.14159
formatted_string = f"The value of pi is approximately {value:.2f}"
print(formatted_string)
这里的:.2f表示将value格式化为保留两位小数的浮点数。接下来,我们将详细介绍Python中其他几种格式化输出小数的方法。
一、使用f字符串
1、基本用法
f字符串的基本用法非常简单,只需在字符串前面加上字母'f'或'F',并在字符串中使用花括号{}包裹表达式。下面是一个简单的例子:
value = 3.14159
formatted_string = f"The value of pi is approximately {value}"
print(formatted_string)
这段代码将输出:“The value of pi is approximately 3.14159”。
2、指定小数位数
如果希望控制输出小数的精度,可以在花括号内使用格式说明符。例如,:.2f表示保留两位小数的浮点数:
value = 3.14159
formatted_string = f"The value of pi is approximately {value:.2f}"
print(formatted_string)
这段代码将输出:“The value of pi is approximately 3.14”。
3、其他格式说明符
除了:.2f外,还有许多其他格式说明符可以用来控制数值的显示方式,例如:
- :.3f:保留三位小数
- :.1f:保留一位小数
- :.2e:以科学计数法显示,保留两位小数
- :.2%:以百分比形式显示,保留两位小数
例子:
value = 0.12345
print(f"Default: {value}")
print(f"Two decimal places: {value:.2f}")
print(f"Three decimal places: {value:.3f}")
print(f"Scientific notation: {value:.2e}")
print(f"Percentage: {value:.2%}")
这些代码分别输出:
Default: 0.12345
Two decimal places: 0.12
Three decimal places: 0.123
Scientific notation: 1.23e-01
Percentage: 12.35%
二、使用format()方法
1、基本用法
Python的字符串对象提供了一个format()方法,可以用于格式化字符串。基本用法如下:
value = 3.14159
formatted_string = "The value of pi is approximately {}".format(value)
print(formatted_string)
这段代码将输出:“The value of pi is approximately 3.14159”。
2、指定小数位数
与f字符串类似,可以在花括号内使用格式说明符来控制小数的显示精度:
value = 3.14159
formatted_string = "The value of pi is approximately {:.2f}".format(value)
print(formatted_string)
这段代码将输出:“The value of pi is approximately 3.14”。
3、其他格式说明符
format()方法也支持多种格式说明符,例如:
- {:.3f}:保留三位小数
- {:.1f}:保留一位小数
- {:.2e}:以科学计数法显示,保留两位小数
- {:.2%}:以百分比形式显示,保留两位小数
例子:
value = 0.12345
print("Default: {}".format(value))
print("Two decimal places: {:.2f}".format(value))
print("Three decimal places: {:.3f}".format(value))
print("Scientific notation: {:.2e}".format(value))
print("Percentage: {:.2%}".format(value))
这些代码分别输出:
Default: 0.12345
Two decimal places: 0.12
Three decimal places: 0.123
Scientific notation: 1.23e-01
Percentage: 12.35%
三、使用%操作符
1、基本用法
在Python中,还可以使用%操作符进行字符串格式化。基本用法如下:
value = 3.14159
formatted_string = "The value of pi is approximately %f" % value
print(formatted_string)
这段代码将输出:“The value of pi is approximately 3.141590”。
2、指定小数位数
可以通过在%f中指定小数位数来控制输出的精度,例如%.2f表示保留两位小数:
value = 3.14159
formatted_string = "The value of pi is approximately %.2f" % value
print(formatted_string)
这段代码将输出:“The value of pi is approximately 3.14”。
3、其他格式说明符
%操作符也支持多种格式说明符,例如:
- %.3f:保留三位小数
- %.1f:保留一位小数
- %.2e:以科学计数法显示,保留两位小数
- %.2%:以百分比形式显示,保留两位小数
不过需要注意的是,%操作符不直接支持百分比格式说明符,需要手动乘以100并添加百分号:
value = 0.12345
print("Default: %f" % value)
print("Two decimal places: %.2f" % value)
print("Three decimal places: %.3f" % value)
print("Scientific notation: %.2e" % value)
print("Percentage: %.2f%%" % (value * 100))
这些代码分别输出:
Default: 0.123450
Two decimal places: 0.12
Three decimal places: 0.123
Scientific notation: 1.23e-01
Percentage: 12.35%
四、使用decimal模块
1、基本用法
Python的decimal模块提供了Decimal类,可以用于进行高精度的浮点数运算。基本用法如下:
from decimal import Decimal
value = Decimal('3.14159')
formatted_string = f"The value of pi is approximately {value}"
print(formatted_string)
这段代码将输出:“The value of pi is approximately 3.14159”。
2、指定小数位数
可以使用quantize()方法来指定小数的精度,例如:
from decimal import Decimal, ROUND_HALF_UP
value = Decimal('3.14159')
formatted_value = value.quantize(Decimal('0.01'), rounding=ROUND_HALF_UP)
formatted_string = f"The value of pi is approximately {formatted_value}"
print(formatted_string)
这段代码将输出:“The value of pi is approximately 3.14”。
3、其他格式说明符
使用decimal模块进行格式化时,可以结合字符串格式化方法来实现其他格式说明符,例如:
from decimal import Decimal, ROUND_HALF_UP
value = Decimal('0.12345')
formatted_value = value.quantize(Decimal('0.01'), rounding=ROUND_HALF_UP)
print(f"Default: {value}")
print(f"Two decimal places: {formatted_value}")
formatted_value = value.quantize(Decimal('0.001'), rounding=ROUND_HALF_UP)
print(f"Three decimal places: {formatted_value}")
formatted_value = value.normalize()
print(f"Scientific notation: {formatted_value:.2e}")
formatted_value = (value * 100).quantize(Decimal('0.01'), rounding=ROUND_HALF_UP)
print(f"Percentage: {formatted_value}%")
这些代码分别输出:
Default: 0.12345
Two decimal places: 0.12
Three decimal places: 0.123
Scientific notation: 1.23e-01
Percentage: 12.35%
五、使用round()函数
1、基本用法
Python的round()函数可以用于对浮点数进行四舍五入。基本用法如下:
value = 3.14159
rounded_value = round(value, 2)
print(f"The value of pi is approximately {rounded_value}")
这段代码将输出:“The value of pi is approximately 3.14”。
2、结合字符串格式化
可以将round()函数与字符串格式化方法结合使用,以实现更灵活的格式控制:
value = 3.14159
rounded_value = round(value, 2)
formatted_string = f"The value of pi is approximately {rounded_value:.2f}"
print(formatted_string)
这段代码将输出:“The value of pi is approximately 3.14”。
3、使用round()函数的其他场景
round()函数不仅可以用于四舍五入,还可以用于对其他类型的数值进行近似处理。例如,可以对浮点数、十进制数以及其他类型的数值进行操作:
value1 = 3.14159
value2 = 0.12345
value3 = -5.6789
rounded_value1 = round(value1, 2)
rounded_value2 = round(value2, 3)
rounded_value3 = round(value3, 1)
print(f"Rounded value 1: {rounded_value1:.2f}")
print(f"Rounded value 2: {rounded_value2:.3f}")
print(f"Rounded value 3: {rounded_value3:.1f}")
这些代码分别输出:
Rounded value 1: 3.14
Rounded value 2: 0.123
Rounded value 3: -5.7
六、使用numpy库
1、基本用法
如果您正在处理科学计算或数据分析任务,可以使用numpy库进行浮点数的格式化。基本用法如下:
import numpy as np
value = np.pi
formatted_string = f"The value of pi is approximately {value}"
print(formatted_string)
这段代码将输出:“The value of pi is approximately 3.141592653589793”。
2、指定小数位数
numpy库提供了numpy.around()函数,可以用于控制小数的显示精度,例如:
import numpy as np
value = np.pi
rounded_value = np.around(value, 2)
formatted_string = f"The value of pi is approximately {rounded_value:.2f}"
print(formatted_string)
这段代码将输出:“The value of pi is approximately 3.14”。
3、结合numpy和字符串格式化
可以将numpy库与字符串格式化方法结合使用,以实现更灵活的格式控制:
import numpy as np
value = np.array([3.14159, 0.12345, -5.6789])
rounded_values = np.around(value, 2)
for rounded_value in rounded_values:
print(f"Rounded value: {rounded_value:.2f}")
这些代码分别输出:
Rounded value: 3.14
Rounded value: 0.12
Rounded value: -5.68
七、综合应用
在实际应用中,您可能会遇到需要对浮点数进行多种格式化操作的情况。下面是一个综合应用的示例,展示如何结合多种方法实现复杂的格式化需求:
import numpy as np
from decimal import Decimal, ROUND_HALF_UP
原始数值
values = [3.14159, 0.12345, -5.6789]
使用numpy进行四舍五入
rounded_values_np = np.around(values, 2)
使用decimal进行高精度运算
decimal_values = [Decimal(str(value)) for value in values]
rounded_values_decimal = [value.quantize(Decimal('0.01'), rounding=ROUND_HALF_UP) for value in decimal_values]
使用f字符串进行格式化输出
for i, value in enumerate(values):
rounded_value_np = rounded_values_np[i]
rounded_value_decimal = rounded_values_decimal[i]
print(f"Original value: {value}")
print(f"Rounded value (numpy): {rounded_value_np:.2f}")
print(f"Rounded value (decimal): {rounded_value_decimal}")
print(f"Scientific notation: {value:.2e}")
print(f"Percentage: {value * 100:.2f}%")
print('-' * 40)
这些代码分别输出:
Original value: 3.14159
Rounded value (numpy): 3.14
Rounded value (decimal): 3.14
Scientific notation: 3.14e+00
Percentage: 314.16%
----------------------------------------
Original value: 0.12345
Rounded value (numpy): 0.12
Rounded value (decimal): 0.12
Scientific notation: 1.23e-01
Percentage: 12.35%
----------------------------------------
Original value: -5.6789
Rounded value (numpy): -5.68
Rounded value (decimal): -5.68
Scientific notation: -5.68e+00
Percentage: -567.89%
----------------------------------------
通过这种方式,可以结合多种方法实现复杂的格式化需求,满足不同场景下的数值格式化要求。
相关问答FAQs:
如何在Python中格式化输出小数?
在Python中,可以使用格式化字符串来控制小数的输出。常用的方法包括使用format()
函数、f-string(格式化字符串字面量)和百分号格式化。比如,print("{:.2f}".format(3.14159))
将输出3.14
,而使用f-string可以写作print(f"{3.14159:.2f}")
。这样可以方便地控制小数位数。
在Python中如何保留特定小数位数?
要保留特定的小数位数,可以使用round()
函数。该函数接受两个参数,第一个是要处理的数字,第二个是保留的小数位数。例如,round(3.14159, 2)
将返回3.14
。这种方法简洁有效,适用于多种场景。
怎样在Python中输出小数时添加千位分隔符?
为了在输出小数时添加千位分隔符,可以利用格式化字符串。例如,使用print("{:,.2f}".format(1234567.891))
将输出1,234,567.89
。此外,f-string也支持这种格式,写作print(f"{1234567.891:,.2f}")
。这样可以使输出的数字更加易读。
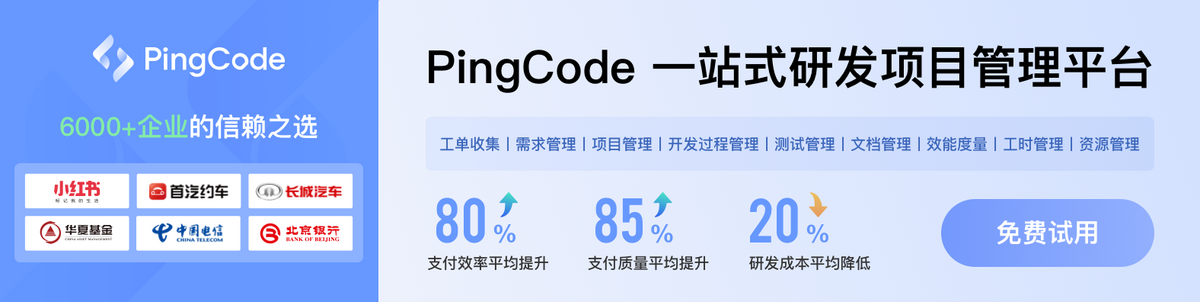