Python通过接口访问数据的主要步骤包括:发送HTTP请求、解析响应数据、处理数据、错误处理和使用第三方库。 在这些步骤中,发送HTTP请求是最关键的一步,它决定了接口访问的成功与否。下面将详细介绍这一点。
发送HTTP请求:通过Python访问接口数据的第一步是发送HTTP请求。这通常通过requests
库来完成。你需要知道接口的URL和请求方法(GET、POST等)。例如,使用GET方法获取数据时,可以这样做:
import requests
url = 'https://api.example.com/data'
response = requests.get(url)
if response.status_code == 200:
data = response.json()
print(data)
else:
print('Failed to retrieve data:', response.status_code)
上述代码中,requests.get(url)
发送了一个GET请求,如果请求成功(状态码200),则使用response.json()
方法将响应转换为Python字典或列表。
一、发送HTTP请求
1、GET请求
GET请求是最常见的HTTP请求方法之一,主要用于从服务器获取数据。通过requests
库发送GET请求非常简单,只需要知道接口的URL。
import requests
url = 'https://api.example.com/data'
response = requests.get(url)
if response.status_code == 200:
data = response.json()
print(data)
else:
print('Failed to retrieve data:', response.status_code)
在这个示例中,我们首先导入了requests
库,然后定义了接口的URL。通过requests.get(url)
发送GET请求,并检查响应的状态码。如果状态码是200,表示请求成功,我们将响应数据转换为JSON格式并输出。
2、POST请求
POST请求用于向服务器发送数据,通常用于提交表单、上传文件等操作。与GET请求不同,POST请求需要在请求体中包含数据。
import requests
url = 'https://api.example.com/data'
payload = {'key1': 'value1', 'key2': 'value2'}
response = requests.post(url, data=payload)
if response.status_code == 200:
data = response.json()
print(data)
else:
print('Failed to retrieve data:', response.status_code)
在这个示例中,我们使用requests.post(url, data=payload)
发送POST请求,并在请求体中包含了数据payload
。同样地,我们检查响应的状态码并处理响应数据。
二、解析响应数据
1、JSON格式
大多数API返回的数据都是JSON格式。使用requests
库的response.json()
方法可以轻松地将响应数据转换为Python字典或列表。
import requests
url = 'https://api.example.com/data'
response = requests.get(url)
if response.status_code == 200:
data = response.json()
print(data)
else:
print('Failed to retrieve data:', response.status_code)
在这个示例中,response.json()
方法将响应数据转换为Python字典或列表,并存储在变量data
中。
2、XML格式
尽管JSON是最常见的数据格式,但有些API也返回XML格式的数据。可以使用xml.etree.ElementTree
库来解析XML数据。
import requests
import xml.etree.ElementTree as ET
url = 'https://api.example.com/data'
response = requests.get(url)
if response.status_code == 200:
root = ET.fromstring(response.content)
for child in root:
print(child.tag, child.text)
else:
print('Failed to retrieve data:', response.status_code)
在这个示例中,我们首先导入了xml.etree.ElementTree
库,然后使用ET.fromstring(response.content)
方法将响应数据转换为XML树。接下来,我们遍历XML树并输出每个子元素的标签和文本内容。
三、处理数据
1、数据存储
从API获取数据后,通常需要将其存储在本地文件或数据库中。可以使用Python标准库或第三方库来实现数据存储。
import json
data = {'key1': 'value1', 'key2': 'value2'}
with open('data.json', 'w') as f:
json.dump(data, f)
在这个示例中,我们使用json
库将数据存储在本地JSON文件中。首先定义了数据,然后使用json.dump(data, f)
方法将数据写入文件data.json
。
2、数据处理
除了存储数据,有时还需要对数据进行处理。例如,可以使用Pandas库来分析和处理从API获取的数据。
import requests
import pandas as pd
url = 'https://api.example.com/data'
response = requests.get(url)
if response.status_code == 200:
data = response.json()
df = pd.DataFrame(data)
print(df.head())
else:
print('Failed to retrieve data:', response.status_code)
在这个示例中,我们使用pandas
库将JSON数据转换为DataFrame,并输出前五行数据。Pandas库提供了丰富的数据处理和分析功能,使得处理API数据更加方便。
四、错误处理
1、HTTP错误
在访问API时,可能会遇到各种HTTP错误。需要检查响应的状态码,并根据不同的状态码进行相应的处理。
import requests
url = 'https://api.example.com/data'
response = requests.get(url)
if response.status_code == 200:
data = response.json()
print(data)
elif response.status_code == 404:
print('Data not found')
else:
print('Failed to retrieve data:', response.status_code)
在这个示例中,我们检查了响应的状态码,并根据不同的状态码输出相应的错误信息。如果状态码是200,表示请求成功;如果状态码是404,表示数据未找到;否则,输出通用错误信息。
2、异常处理
除了HTTP错误,还可能会遇到网络连接错误、超时错误等异常。可以使用try-except
块来捕获和处理这些异常。
import requests
url = 'https://api.example.com/data'
try:
response = requests.get(url)
response.raise_for_status()
data = response.json()
print(data)
except requests.exceptions.RequestException as e:
print('An error occurred:', e)
在这个示例中,我们使用try-except
块捕获所有requests
库可能抛出的异常。如果请求成功,输出数据;如果发生异常,输出错误信息。
五、使用第三方库
1、requests
库
requests
库是Python中最流行的HTTP库之一,提供了简单而强大的API来发送HTTP请求和处理响应数据。前面已经介绍了如何使用requests
库发送GET和POST请求。
2、httpx
库
httpx
库是另一个流行的HTTP库,提供了同步和异步请求的支持。与requests
库类似,httpx
库也提供了简单的API来发送HTTP请求和处理响应数据。
import httpx
url = 'https://api.example.com/data'
with httpx.Client() as client:
response = client.get(url)
if response.status_code == 200:
data = response.json()
print(data)
else:
print('Failed to retrieve data:', response.status_code)
在这个示例中,我们使用httpx
库的同步API发送GET请求。首先创建一个httpx.Client
实例,然后使用client.get(url)
发送请求,并检查响应的状态码。
3、aiohttp
库
aiohttp
库是一个异步HTTP库,适用于需要处理大量并发请求的场景。使用aiohttp
库可以显著提高请求的性能和效率。
import aiohttp
import asyncio
async def fetch_data(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
if response.status == 200:
data = await response.json()
print(data)
else:
print('Failed to retrieve data:', response.status)
url = 'https://api.example.com/data'
loop = asyncio.get_event_loop()
loop.run_until_complete(fetch_data(url))
在这个示例中,我们使用aiohttp
库的异步API发送GET请求。首先定义了一个异步函数fetch_data(url)
,在函数内部使用aiohttp.ClientSession
创建一个会话,并发送异步请求。最后,使用事件循环loop
运行异步函数。
4、urllib
库
urllib
库是Python标准库中的一个HTTP库,提供了基本的HTTP请求和响应处理功能。尽管urllib
库不如requests
库和httpx
库功能强大,但在某些情况下仍然可以使用。
import urllib.request
import json
url = 'https://api.example.com/data'
with urllib.request.urlopen(url) as response:
if response.status == 200:
data = json.load(response)
print(data)
else:
print('Failed to retrieve data:', response.status)
在这个示例中,我们使用urllib.request.urlopen(url)
发送GET请求,并检查响应的状态码。如果请求成功,使用json.load(response)
将响应数据转换为Python字典或列表。
六、认证和授权
1、API密钥
许多API需要认证和授权,通常通过API密钥来实现。API密钥是一个唯一的标识符,允许你访问API并执行特定操作。
import requests
url = 'https://api.example.com/data'
headers = {'Authorization': 'Bearer YOUR_API_KEY'}
response = requests.get(url, headers=headers)
if response.status_code == 200:
data = response.json()
print(data)
else:
print('Failed to retrieve data:', response.status_code)
在这个示例中,我们在请求头中包含了API密钥headers = {'Authorization': 'Bearer YOUR_API_KEY'}
,并使用requests.get(url, headers=headers)
发送GET请求。
2、OAuth认证
OAuth是一种常用的认证和授权协议,允许第三方应用访问用户资源而无需暴露用户凭据。可以使用requests-oauthlib
库来处理OAuth认证。
from requests_oauthlib import OAuth1Session
url = 'https://api.example.com/data'
client_key = 'YOUR_CLIENT_KEY'
client_secret = 'YOUR_CLIENT_SECRET'
resource_owner_key = 'YOUR_RESOURCE_OWNER_KEY'
resource_owner_secret = 'YOUR_RESOURCE_OWNER_SECRET'
oauth = OAuth1Session(client_key, client_secret=client_secret,
resource_owner_key=resource_owner_key,
resource_owner_secret=resource_owner_secret)
response = oauth.get(url)
if response.status_code == 200:
data = response.json()
print(data)
else:
print('Failed to retrieve data:', response.status_code)
在这个示例中,我们使用OAuth1Session
创建了一个OAuth会话,并使用oauth.get(url)
发送GET请求。需要提供客户端密钥、客户端密钥、资源所有者密钥和资源所有者密钥等认证信息。
七、API速率限制
许多API对请求速率有限制,以防止滥用和过载。在访问这些API时,需要遵守速率限制,并在必要时实现重试机制。
1、处理速率限制
可以使用time.sleep
函数在发送请求之间添加延迟,以确保遵守API的速率限制。
import requests
import time
url = 'https://api.example.com/data'
headers = {'Authorization': 'Bearer YOUR_API_KEY'}
for i in range(10):
response = requests.get(url, headers=headers)
if response.status_code == 200:
data = response.json()
print(data)
else:
print('Failed to retrieve data:', response.status_code)
time.sleep(1) # 延迟1秒
在这个示例中,我们在发送每个请求之间添加了1秒的延迟,以确保遵守API的速率限制。
2、实现重试机制
可以使用retrying
库实现重试机制,以处理临时错误和速率限制。
import requests
from retrying import retry
@retry(stop_max_attempt_number=3, wait_fixed=2000)
def fetch_data(url, headers):
response = requests.get(url, headers=headers)
if response.status_code == 200:
return response.json()
else:
response.raise_for_status()
url = 'https://api.example.com/data'
headers = {'Authorization': 'Bearer YOUR_API_KEY'}
try:
data = fetch_data(url, headers)
print(data)
except requests.exceptions.RequestException as e:
print('An error occurred:', e)
在这个示例中,我们使用retry
装饰器为fetch_data
函数添加了重试机制。如果请求失败,函数将最多重试3次,每次重试之间等待2秒。
八、API文档和测试
1、阅读API文档
在使用API之前,阅读API文档非常重要。API文档通常包括接口的URL、请求方法、请求参数、响应格式、错误代码等信息。通过阅读API文档,可以了解如何正确地使用API,并避免常见的错误。
2、使用API测试工具
可以使用Postman、Insomnia等API测试工具来测试和调试API请求。这些工具提供了图形界面,使得发送请求和查看响应更加方便。
# 使用Postman或Insomnia测试API请求
在使用API测试工具时,可以输入接口的URL、请求方法、请求参数和请求头,并发送请求查看响应数据。这有助于在编写代码之前验证API的正确性和可用性。
九、示例应用
1、获取天气数据
下面是一个使用OpenWeatherMap API获取天气数据的示例应用。
import requests
def get_weather(city):
api_key = 'YOUR_API_KEY'
url = f'http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}'
response = requests.get(url)
if response.status_code == 200:
data = response.json()
weather = data['weather'][0]['description']
temperature = data['main']['temp']
print(f'Weather: {weather}')
print(f'Temperature: {temperature}K')
else:
print('Failed to retrieve data:', response.status_code)
city = 'London'
get_weather(city)
在这个示例中,我们定义了一个get_weather
函数,使用OpenWeatherMap API获取指定城市的天气数据。首先构建API请求的URL,并发送GET请求。然后解析响应数据,输出天气描述和温度。
2、获取股票价格
下面是一个使用Alpha Vantage API获取股票价格的示例应用。
import requests
def get_stock_price(symbol):
api_key = 'YOUR_API_KEY'
url = f'https://www.alphavantage.co/query?function=TIME_SERIES_INTRADAY&symbol={symbol}&interval=5min&apikey={api_key}'
response = requests.get(url)
if response.status_code == 200:
data = response.json()
time_series = data['Time Series (5min)']
latest_time = sorted(time_series.keys())[0]
latest_price = time_series[latest_time]['1. open']
print(f'Stock Price: {latest_price}')
else:
print('Failed to retrieve data:', response.status_code)
symbol = 'AAPL'
get_stock_price(symbol)
在这个示例中,我们定义了一个get_stock_price
函数,使用Alpha Vantage API获取指定股票的价格。首先构建API请求的URL,并发送GET请求。然后解析响应数据,输出最新的股票价格。
十、总结
通过本文的介绍,我们了解了Python通过接口访问数据的主要步骤和方法,包括发送HTTP请求、解析响应数据、处理数据、错误处理和使用第三方库。我们还介绍了如何处理认证和授权、API速率限制、阅读API文档和使用API测试工具。最后,通过两个示例应用,展示了如何实际使用API获取天气数据和股票价格。
总之,使用Python通过接口访问数据是一项非常实用的技能,可以帮助我们获取和处理各种在线数据。希望本文对你有所帮助,并能在实际项目中应用这些知识和技巧。
相关问答FAQs:
如何使用Python调用RESTful API获取数据?
使用Python调用RESTful API可以通过requests
库实现。首先,您需要安装该库,使用命令pip install requests
。接下来,您可以使用requests.get()
方法发送GET请求,并通过API的URL获取数据。获取的数据通常为JSON格式,您可以使用response.json()
将其转换为Python字典,以便于后续处理。
在Python中如何处理API返回的错误信息?
在访问API时,可能会遇到各种错误,例如404(未找到)、500(服务器错误)等。您可以通过检查响应状态码来处理这些错误。使用response.status_code
可以获取状态码,您可以根据不同的状态码进行相应的错误处理。此外,使用try...except
语句可以捕获异常,确保程序不会因未处理的错误而崩溃。
如何在Python中使用API进行身份验证?
许多API需要身份验证才能访问数据。在Python中,可以通过在请求中添加适当的身份验证头来实现。常见的身份验证方式包括基本认证和Bearer Token认证。使用requests
库时,您可以在请求中添加auth
参数或者使用headers
参数设置Authorization头,以提供必要的凭据或令牌。这将确保您能够顺利访问需要身份验证的API。
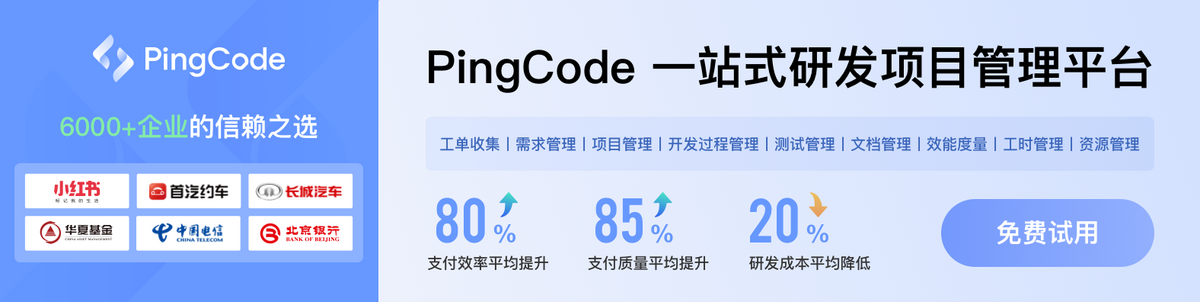