使用Python获取终端窗口的方法包括:使用subprocess模块、使用os模块、使用pty模块。其中,subprocess模块是最常用且功能强大的方法。下面将详细描述如何使用subprocess模块来获取终端窗口。
一、SUBPROCESS模块
subprocess模块是在Python中运行子进程的首选模块,它允许你启动新的应用程序或命令,并可以连接它们的输入/输出/错误管道。通过subprocess模块,你可以在Python中调用系统命令并在终端窗口中运行。
1、基本用法
subprocess模块提供了多种方法来运行系统命令,其中最常用的是subprocess.run
。下面是一个简单的例子:
import subprocess
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
print(result.stdout)
在这个例子中,我们使用subprocess.run
来运行ls -l
命令,并捕获其输出。通过设置capture_output=True
和text=True
,我们可以将输出直接作为字符串返回。
2、运行交互式命令
对于需要用户交互的命令,我们可以使用subprocess.Popen
。例如:
import subprocess
proc = subprocess.Popen(['python3'], stdin=subprocess.PIPE, stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
向子进程发送输入
proc.stdin.write('print("Hello from subprocess")\n')
proc.stdin.flush()
读取子进程的输出
output = proc.stdout.readline()
print(output)
在这个例子中,我们启动了一个Python解释器作为子进程,并通过其标准输入发送命令,然后读取标准输出的结果。
二、OS模块
os模块也可以用于运行系统命令,虽然它的功能不如subprocess模块强大,但在某些简单的场景下仍然非常有用。
1、使用os.system
os.system
可以直接运行系统命令,并将结果输出到终端窗口。例如:
import os
os.system('ls -l')
这个命令会在终端窗口中显示ls -l
的输出。
2、使用os.popen
os.popen
可以运行系统命令,并返回一个文件对象,用于读取命令的输出。例如:
import os
with os.popen('ls -l') as stream:
output = stream.read()
print(output)
这个方法相比os.system
更灵活,因为你可以直接处理命令的输出。
三、PTY模块
pty模块用于伪终端操作,适合需要精细控制终端交互的场景。这个模块通常用于创建和操作伪终端设备。
1、启动伪终端
你可以使用pty.spawn
来启动一个伪终端并运行命令。例如:
import pty
import os
def read(fd):
data = os.read(fd, 1024)
return data
pty.spawn('/bin/bash', read)
在这个例子中,我们使用pty.spawn
启动了一个bash终端,并通过自定义的read
函数来处理终端的输出。
2、使用pty.fork
pty.fork
可以创建一个新的伪终端会话,并返回主从终端的文件描述符。例如:
import os
import pty
pid, fd = pty.fork()
if pid == 0:
# 子进程
os.execlp('bash', 'bash')
else:
# 父进程
while True:
data = os.read(fd, 1024)
if not data:
break
print(data.decode(), end='')
在这个例子中,父进程创建了一个新的伪终端会话,并读取子进程的输出。
四、总结
获取终端窗口在Python中有多种方法,其中最常用的是subprocess模块。subprocess模块功能强大,适用于各种系统命令和交互式命令的运行。os模块虽然功能较为简单,但在某些场景下也非常实用。pty模块适合需要精细控制终端交互的场景,提供了对伪终端的操作接口。
通过这些方法,你可以在Python中轻松地运行系统命令、启动新应用程序,并与它们进行交互。根据具体需求选择合适的方法,可以提高代码的灵活性和可维护性。
五、实际应用案例
1、自动化脚本
在实际应用中,Python脚本常常用于自动化任务。比如,你可以编写一个脚本来备份文件:
import subprocess
def backup_files(source, destination):
command = ['cp', '-r', source, destination]
result = subprocess.run(command, capture_output=True, text=True)
if result.returncode == 0:
print("Backup successful")
else:
print(f"Error: {result.stderr}")
backup_files('/path/to/source', '/path/to/destination')
这个脚本使用subprocess.run
来执行cp
命令,将源目录备份到目标目录。
2、监控系统状态
你可以编写一个Python脚本来定期监控系统状态,并生成报告:
import subprocess
import time
def get_system_status():
uptime = subprocess.run(['uptime'], capture_output=True, text=True).stdout
free_memory = subprocess.run(['free', '-h'], capture_output=True, text=True).stdout
disk_usage = subprocess.run(['df', '-h'], capture_output=True, text=True).stdout
return uptime, free_memory, disk_usage
def monitor_system(interval):
while True:
uptime, free_memory, disk_usage = get_system_status()
print(f"Uptime: {uptime}")
print(f"Memory Usage: {free_memory}")
print(f"Disk Usage: {disk_usage}")
time.sleep(interval)
monitor_system(60) # 每隔60秒监控一次
这个脚本使用subprocess.run
来获取系统的运行时间、内存使用情况和磁盘使用情况,并定期打印这些信息。
3、交互式会话
在某些情况下,你可能需要与命令行工具进行交互。比如,你可以使用subprocess.Popen
来自动化输入和输出:
import subprocess
def interactive_session():
proc = subprocess.Popen(['python3'], stdin=subprocess.PIPE, stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
proc.stdin.write('print("Hello from Python subprocess")\n')
proc.stdin.flush()
output = proc.stdout.readline()
print(output)
interactive_session()
这个脚本启动了一个Python解释器,并自动向其发送命令,然后读取并打印输出。
六、错误处理和调试
在实际应用中,错误处理和调试是非常重要的环节。以下是一些常见的错误处理和调试技巧:
1、检查返回码
当使用subprocess.run
时,可以通过检查返回码来判断命令是否执行成功:
import subprocess
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
if result.returncode == 0:
print("Command executed successfully")
else:
print(f"Error: {result.stderr}")
返回码为0表示命令执行成功,非0表示执行失败。
2、捕获异常
在使用subprocess.run
时,可以捕获异常来处理运行时错误:
import subprocess
try:
result = subprocess.run(['nonexistent_command'], capture_output=True, text=True, check=True)
except subprocess.CalledProcessError as e:
print(f"Command failed with error: {e}")
通过设置check=True
,如果命令执行失败,将抛出subprocess.CalledProcessError
异常。
3、调试输出
在调试过程中,可以打印调试信息来帮助定位问题:
import subprocess
command = ['ls', '-l']
print(f"Running command: {command}")
result = subprocess.run(command, capture_output=True, text=True)
print(f"Command output: {result.stdout}")
print(f"Command error: {result.stderr}")
通过打印命令和输出信息,可以更容易地发现问题所在。
七、跨平台兼容性
在编写Python脚本时,跨平台兼容性是一个需要考虑的重要问题。以下是一些提高跨平台兼容性的方法:
1、使用Python内置方法
尽量使用Python内置方法和标准库函数,而不是直接调用系统命令。例如,使用os
模块来操作文件和目录,而不是使用ls
或cp
等命令。
import os
files = os.listdir('.')
print(files)
2、检测操作系统
在运行特定于操作系统的命令时,可以通过检测操作系统来选择合适的命令:
import os
import platform
if platform.system() == 'Windows':
os.system('dir')
else:
os.system('ls -l')
通过检测操作系统,可以编写跨平台兼容的脚本。
3、使用第三方库
在某些情况下,第三方库可以提供更好的跨平台支持。例如,shutil
库提供了跨平台的文件操作方法:
import shutil
shutil.copy('source_file', 'destination_file')
使用shutil
库的copy
方法,可以在不同操作系统上进行文件复制操作。
八、安全性考虑
在运行系统命令时,安全性是一个非常重要的考虑因素。以下是一些提高安全性的方法:
1、避免使用shell=True
尽量避免在subprocess.run
或subprocess.Popen
中使用shell=True
,因为这可能导致命令注入攻击:
import subprocess
不推荐的做法
subprocess.run('ls -l', shell=True)
推荐的做法
subprocess.run(['ls', '-l'])
通过传递命令列表而不是字符串,可以避免shell注入攻击。
2、验证输入
在接受用户输入并传递给系统命令时,一定要进行验证和过滤:
import subprocess
def list_files(directory):
if not os.path.isdir(directory):
raise ValueError("Invalid directory")
subprocess.run(['ls', '-l', directory])
user_input = input("Enter directory: ")
list_files(user_input)
通过验证输入,可以防止恶意用户传递危险命令。
3、限制权限
在运行系统命令时,尽量以最低权限运行,以减少潜在的安全风险。例如,可以在受限的用户环境中运行命令,避免对系统造成影响。
九、性能优化
在某些情况下,运行系统命令可能会消耗大量资源,因此需要进行性能优化。以下是一些常见的优化方法:
1、异步运行
对于耗时较长的命令,可以使用异步运行来提高性能。例如,使用subprocess.Popen
来异步运行命令:
import subprocess
proc = subprocess.Popen(['long_running_command'])
主进程继续执行其他任务
proc.wait() # 等待子进程完成
通过异步运行,可以避免阻塞主进程,提高程序的响应速度。
2、并行执行
对于多个独立的命令,可以使用并行执行来提高性能。例如,使用多线程或多进程来并行运行命令:
import subprocess
import threading
def run_command(command):
subprocess.run(command)
commands = [['command1'], ['command2'], ['command3']]
threads = [threading.Thread(target=run_command, args=(cmd,)) for cmd in commands]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
通过并行执行,可以充分利用多核处理器的性能优势。
3、缓存结果
对于频繁执行的命令,可以缓存结果以避免重复计算。例如,可以使用字典来缓存命令的输出:
import subprocess
cache = {}
def run_command(command):
if command in cache:
return cache[command]
result = subprocess.run(command, capture_output=True, text=True).stdout
cache[command] = result
return result
output = run_command(['ls', '-l'])
print(output)
通过缓存结果,可以显著减少重复计算的开销。
十、总结与展望
通过本文的介绍,我们详细探讨了在Python中获取终端窗口的多种方法,包括subprocess模块、os模块和pty模块。我们不仅介绍了这些方法的基本用法,还深入探讨了实际应用案例、错误处理与调试、跨平台兼容性、安全性考虑以及性能优化等方面的内容。
在未来,随着Python生态系统的不断发展,我们可能会看到更多功能强大且易用的库和工具,进一步简化与系统命令和终端窗口交互的过程。希望本文能为你在Python中进行终端操作提供有益的参考和指导。
相关问答FAQs:
如何在Python中打开一个新的终端窗口?
可以使用os
或subprocess
模块来打开新的终端窗口。具体实现方法取决于操作系统,例如在Windows中,可以使用os.system('start cmd')
,而在Linux或MacOS中,可以使用os.system('gnome-terminal')
或os.system('open -a Terminal')
。确保在运行这些命令时,Python脚本具有足够的权限。
在Python中如何获取终端窗口的输出?
如果想要在Python中捕获终端窗口的输出,可以使用subprocess
模块的Popen
函数。通过设置stdout
参数为subprocess.PIPE
,可以读取终端命令的输出。例如:
import subprocess
process = subprocess.Popen(['ls', '-l'], stdout=subprocess.PIPE)
output, error = process.communicate()
print(output.decode())
这样可以在Python脚本中直接获取终端命令的结果。
可以在Python中模拟终端输入吗?
是的,可以使用pexpect
库来模拟终端输入。这个库允许您与子进程进行交互,适用于需要输入密码或其他命令的场景。示例代码如下:
import pexpect
child = pexpect.spawn('ssh user@hostname')
child.expect('password:')
child.sendline('your_password')
这种方式可以帮助您在Python中实现更复杂的终端操作。
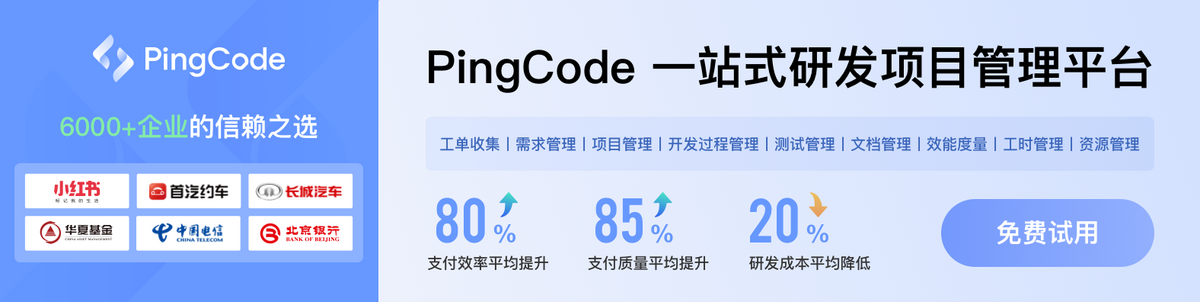