Python发送测试结果邮件的方法包括:使用smtplib库、使用email库、集成第三方库如yagmail。 其中,smtplib库 是Python内置的模块,可以直接使用来发送邮件,较为基础和灵活。下面我将详细介绍如何使用smtplib库来发送测试结果邮件。
一、安装与配置邮件服务
在开始编写代码之前,首先需要确保Python环境已经安装,并且邮件服务已经配置好。我们这里以Gmail为例:
- 获取SMTP服务器和端口号: Gmail的SMTP服务器为
smtp.gmail.com
,端口号为587。 - 启用“低安全性应用访问”: 由于直接使用SMTP发送邮件会被认为是低安全性的行为,需要在Gmail中启用低安全性应用访问。
- 创建应用密码(推荐): 为了更安全的发送邮件,建议使用Gmail的应用密码功能。
二、使用smtplib发送邮件
1. 发送简单邮件
首先,编写一个简单的Python脚本,使用smtplib库发送一封简单的测试结果邮件:
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
邮件配置信息
smtp_server = 'smtp.gmail.com'
smtp_port = 587
username = 'your_email@gmail.com'
password = 'your_password' # 如果使用应用密码,这里填写应用密码
创建邮件对象
msg = MIMEMultipart()
msg['From'] = username
msg['To'] = 'recipient_email@example.com'
msg['Subject'] = 'Test Results'
邮件正文内容
body = 'Here are the test results.'
msg.attach(MIMEText(body, 'plain'))
发送邮件
try:
server = smtplib.SMTP(smtp_server, smtp_port)
server.starttls() # 启用TLS加密
server.login(username, password)
server.sendmail(username, 'recipient_email@example.com', msg.as_string())
server.quit()
print("Email sent successfully!")
except Exception as e:
print(f"Failed to send email: {e}")
2. 发送带附件的邮件
在实际应用中,可能需要将测试结果文件作为附件发送,例如一个测试报告的PDF文件。可以通过以下方式实现:
from email.mime.base import MIMEBase
from email import encoders
创建邮件对象
msg = MIMEMultipart()
msg['From'] = username
msg['To'] = 'recipient_email@example.com'
msg['Subject'] = 'Test Results with Attachment'
邮件正文内容
body = 'Here are the test results with the attached report.'
msg.attach(MIMEText(body, 'plain'))
添加附件
filename = 'test_report.pdf'
attachment = open(filename, 'rb')
part = MIMEBase('application', 'octet-stream')
part.set_payload(attachment.read())
encoders.encode_base64(part)
part.add_header('Content-Disposition', f'attachment; filename= {filename}')
msg.attach(part)
发送邮件
try:
server = smtplib.SMTP(smtp_server, smtp_port)
server.starttls() # 启用TLS加密
server.login(username, password)
server.sendmail(username, 'recipient_email@example.com', msg.as_string())
server.quit()
print("Email sent successfully!")
except Exception as e:
print(f"Failed to send email: {e}")
三、使用第三方库yagmail
yagmail 是一个更高级的邮件发送库,简化了smtplib的使用,特别适合发送带有附件和HTML内容的邮件。
1. 安装yagmail
首先,安装yagmail库:
pip install yagmail
2. 发送简单邮件
使用yagmail发送简单邮件的代码如下:
import yagmail
邮件配置信息
yag = yagmail.SMTP('your_email@gmail.com', 'your_password') # 如果使用应用密码,这里填写应用密码
发送邮件
try:
yag.send(
to='recipient_email@example.com',
subject='Test Results',
contents='Here are the test results.'
)
print("Email sent successfully!")
except Exception as e:
print(f"Failed to send email: {e}")
3. 发送带附件和HTML内容的邮件
使用yagmail发送带附件和HTML内容的邮件:
# 发送带附件和HTML内容的邮件
try:
yag.send(
to='recipient_email@example.com',
subject='Test Results with Attachment and HTML',
contents=[
'Here are the test results with the attached report.',
'<h1>Test Results</h1><p>This is an <b>HTML</b> test result.</p>',
'test_report.pdf'
]
)
print("Email sent successfully!")
except Exception as e:
print(f"Failed to send email: {e}")
四、处理测试结果数据并发送邮件
在实际应用中,测试结果数据可能来自于文件或数据库。以下是一个示例,展示如何读取测试结果数据并发送邮件:
1. 从文件读取测试结果数据
假设测试结果存储在一个CSV文件中,可以使用pandas库读取数据并发送邮件:
import pandas as pd
读取测试结果数据
test_results = pd.read_csv('test_results.csv')
将测试结果数据转换为HTML表格
html_table = test_results.to_html()
发送邮件
try:
yag.send(
to='recipient_email@example.com',
subject='Test Results with HTML Table',
contents=[
'Here are the test results in an HTML table format.',
html_table
]
)
print("Email sent successfully!")
except Exception as e:
print(f"Failed to send email: {e}")
2. 从数据库读取测试结果数据
假设测试结果存储在SQLite数据库中,可以使用sqlite3库读取数据并发送邮件:
import sqlite3
连接数据库
conn = sqlite3.connect('test_results.db')
cursor = conn.cursor()
查询测试结果数据
cursor.execute("SELECT * FROM results")
rows = cursor.fetchall()
将测试结果数据转换为HTML表格
html_table = '<table border="1">'
html_table += '<tr><th>ID</th><th>Name</th><th>Result</th></tr>'
for row in rows:
html_table += f'<tr><td>{row[0]}</td><td>{row[1]}</td><td>{row[2]}</td></tr>'
html_table += '</table>'
发送邮件
try:
yag.send(
to='recipient_email@example.com',
subject='Test Results from Database',
contents=[
'Here are the test results from the database in an HTML table format.',
html_table
]
)
print("Email sent successfully!")
except Exception as e:
print(f"Failed to send email: {e}")
finally:
conn.close()
五、定时发送测试结果邮件
在实际应用中,可能需要定时发送测试结果邮件。可以使用schedule库实现定时任务。
1. 安装schedule
首先,安装schedule库:
pip install schedule
2. 定时发送邮件
使用schedule库定时发送邮件的代码如下:
import schedule
import time
def send_test_results_email():
# 读取测试结果数据
test_results = pd.read_csv('test_results.csv')
html_table = test_results.to_html()
# 发送邮件
try:
yag.send(
to='recipient_email@example.com',
subject='Scheduled Test Results',
contents=[
'Here are the scheduled test results in an HTML table format.',
html_table
]
)
print("Email sent successfully!")
except Exception as e:
print(f"Failed to send email: {e}")
定时任务,每天早上9点发送邮件
schedule.every().day.at("09:00").do(send_test_results_email)
while True:
schedule.run_pending()
time.sleep(1)
以上内容详细介绍了如何使用Python发送测试结果邮件,包括使用smtplib库、yagmail库、处理测试结果数据并发送邮件以及定时发送邮件的实现方法。希望这些内容对您有所帮助。
相关问答FAQs:
如何在Python中发送带有附件的测试结果邮件?
在Python中,您可以使用smtplib
库结合email
模块来发送带有附件的邮件。首先,您需要构建邮件内容并将测试结果文件作为附件添加。以下是一个简单的示例代码:
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
from email.mime.base import MIMEBase
from email import encoders
def send_email_with_attachment(subject, body, to_email, attachment_file):
from_email = "your_email@example.com"
password = "your_password"
msg = MIMEMultipart()
msg['From'] = from_email
msg['To'] = to_email
msg['Subject'] = subject
msg.attach(MIMEText(body, 'plain'))
attachment = open(attachment_file, "rb")
part = MIMEBase('application', 'octet-stream')
part.set_payload(attachment.read())
encoders.encode_base64(part)
part.add_header('Content-Disposition', f'attachment; filename={attachment_file}')
msg.attach(part)
with smtplib.SMTP('smtp.example.com', 587) as server:
server.starttls()
server.login(from_email, password)
server.send_message(msg)
# 使用示例
send_email_with_attachment("测试结果", "这是测试结果的邮件", "recipient@example.com", "test_results.txt")
发送测试结果邮件时需要注意哪些安全问题?
在发送测试结果邮件时,确保使用安全的SMTP服务器,并启用TLS或SSL加密连接,以保护您的邮箱信息。此外,避免在代码中硬编码密码,可以考虑使用环境变量或配置文件来存储敏感信息。
如何确保发送的测试结果邮件不被标记为垃圾邮件?
为了降低邮件被标记为垃圾邮件的风险,您可以采取以下措施:使用真实的发件人邮箱地址,确保邮件内容合法且相关,避免使用过多的链接或图片,定期清理收件人列表,以确保只发送给愿意接收的用户。
如何使用Python自动化发送测试结果邮件?
通过结合自动化测试框架(如pytest、unittest)和邮件发送功能,您可以在测试完成后自动发送结果。可以在测试脚本中添加发送邮件的逻辑,并在测试完成时调用该函数,确保测试结果能够及时反馈给相关人员。
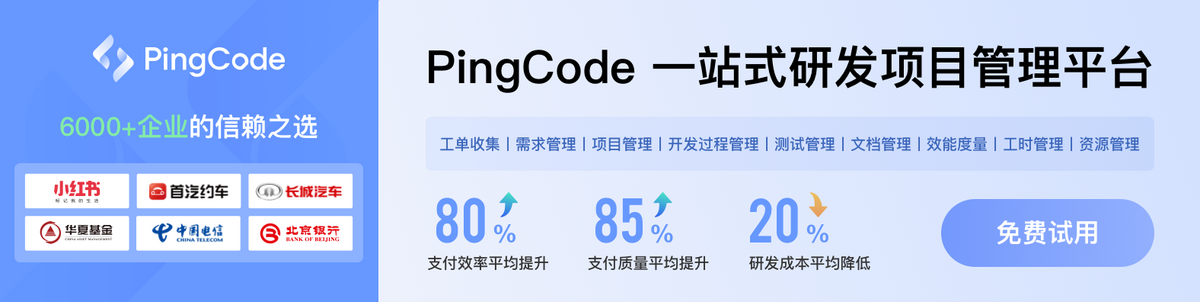