在Python中,循环发送心跳通常用于维持长连接,确保两端的连接是活跃的。这在网络编程、分布式系统、和实时通信中非常重要。实现循环发送心跳的关键方法包括使用 while
循环、使用 time.sleep()
控制间隔时间、和通过 socket
或 requests
发送心跳信号。接下来我会详细介绍其中的一种实现方式。
例如,使用 socket
模块发送心跳信号。首先,创建一个 socket 连接到服务器,然后在一个循环中发送心跳信号。可以通过 time.sleep()
控制心跳信号的发送间隔,并在循环中监听服务器的响应,以确保连接的活跃状态。
import socket
import time
def send_heartbeat():
server_address = ('localhost', 10000) # 替换为实际的服务器地址和端口
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.connect(server_address)
try:
while True:
# 发送心跳信号
message = 'heartbeat'
sock.sendall(message.encode())
print(f'Sent: {message}')
# 等待一段时间
time.sleep(5)
except Exception as e:
print(f'Exception: {e}')
finally:
sock.close()
print('Connection closed')
send_heartbeat()
一、循环发送心跳的必要性
1、维持长连接
在许多实时通信应用中,长时间保持连接是至关重要的。例如,在线游戏、即时消息传递和视频流媒体等应用都需要维持长连接。心跳信号的发送可以确保连接的活跃状态,防止因为长时间没有数据传输而被网络设备误认为连接断开。
2、检测连接状态
心跳信号不仅可以维持连接,还可以用于检测连接状态。如果在规定的时间内没有收到心跳信号的响应,可以认为连接已经断开,从而触发重连机制。这对于提高系统的可靠性和稳定性非常重要。
二、实现心跳发送的基本方法
1、使用 while
循环
在 Python 中,使用 while
循环是实现循环发送心跳信号的常见方法。while
循环可以持续不断地执行心跳信号的发送操作,直到满足某个条件(例如,连接断开或程序终止)。
2、使用 time.sleep()
控制间隔时间
为了避免心跳信号发送得过于频繁,可以使用 time.sleep()
函数来控制发送的间隔时间。time.sleep()
函数可以让程序暂停指定的时间,然后再继续执行。这可以有效地降低系统的负载,并且防止网络拥塞。
三、详细实现步骤
1、创建 socket 连接
首先,需要创建一个 socket 并连接到服务器。可以使用 Python 的 socket
模块来实现这一点。以下是创建 socket 连接的示例代码:
import socket
server_address = ('localhost', 10000) # 替换为实际的服务器地址和端口
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.connect(server_address)
2、发送心跳信号
在创建好 socket 连接之后,可以在一个 while
循环中发送心跳信号。以下是发送心跳信号的示例代码:
while True:
# 发送心跳信号
message = 'heartbeat'
sock.sendall(message.encode())
print(f'Sent: {message}')
# 等待一段时间
time.sleep(5)
3、处理异常和关闭连接
在实际应用中,网络连接可能会出现各种异常情况。因此,需要在代码中添加异常处理逻辑,并在适当的时候关闭连接。以下是处理异常和关闭连接的示例代码:
try:
while True:
# 发送心跳信号
message = 'heartbeat'
sock.sendall(message.encode())
print(f'Sent: {message}')
# 等待一段时间
time.sleep(5)
except Exception as e:
print(f'Exception: {e}')
finally:
sock.close()
print('Connection closed')
四、使用 threading
模块实现并发心跳发送
1、引入 threading
模块
在某些应用场景中,可能需要在多个线程中同时发送心跳信号。可以使用 Python 的 threading
模块来实现这一点。threading
模块允许在一个程序中创建多个线程,每个线程可以独立地执行任务。
2、创建心跳发送线程
首先,需要定义一个函数来发送心跳信号,然后使用 threading.Thread
类来创建线程,并在后台运行该函数。以下是创建心跳发送线程的示例代码:
import threading
def send_heartbeat():
server_address = ('localhost', 10000) # 替换为实际的服务器地址和端口
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.connect(server_address)
try:
while True:
# 发送心跳信号
message = 'heartbeat'
sock.sendall(message.encode())
print(f'Sent: {message}')
# 等待一段时间
time.sleep(5)
except Exception as e:
print(f'Exception: {e}')
finally:
sock.close()
print('Connection closed')
heartbeat_thread = threading.Thread(target=send_heartbeat)
heartbeat_thread.start()
3、管理多个心跳发送线程
如果需要管理多个心跳发送线程,可以创建一个线程池,并在其中启动多个线程。以下是管理多个心跳发送线程的示例代码:
import threading
def send_heartbeat():
server_address = ('localhost', 10000) # 替换为实际的服务器地址和端口
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.connect(server_address)
try:
while True:
# 发送心跳信号
message = 'heartbeat'
sock.sendall(message.encode())
print(f'Sent: {message}')
# 等待一段时间
time.sleep(5)
except Exception as e:
print(f'Exception: {e}')
finally:
sock.close()
print('Connection closed')
创建线程池
num_threads = 5
threads = []
for i in range(num_threads):
heartbeat_thread = threading.Thread(target=send_heartbeat)
threads.append(heartbeat_thread)
heartbeat_thread.start()
等待所有线程完成
for thread in threads:
thread.join()
五、使用 asyncio
实现异步心跳发送
1、引入 asyncio
模块
在某些高并发应用中,异步编程可以提供更高的性能和更好的资源利用率。Python 的 asyncio
模块允许编写异步代码,并且支持异步 I/O 操作。在这里,可以使用 asyncio
模块来实现异步心跳发送。
2、定义异步心跳发送函数
首先,需要定义一个异步函数来发送心跳信号。在这个函数中,可以使用 await
关键字来等待 I/O 操作完成。以下是定义异步心跳发送函数的示例代码:
import asyncio
async def send_heartbeat():
server_address = ('localhost', 10000) # 替换为实际的服务器地址和端口
reader, writer = await asyncio.open_connection(*server_address)
try:
while True:
# 发送心跳信号
message = 'heartbeat'
writer.write(message.encode())
await writer.drain()
print(f'Sent: {message}')
# 等待一段时间
await asyncio.sleep(5)
except Exception as e:
print(f'Exception: {e}')
finally:
writer.close()
await writer.wait_closed()
print('Connection closed')
3、运行异步心跳发送函数
在定义好异步心跳发送函数之后,可以使用 asyncio.run()
函数来运行它。以下是运行异步心跳发送函数的示例代码:
asyncio.run(send_heartbeat())
4、管理多个异步心跳发送任务
如果需要管理多个异步心跳发送任务,可以使用 asyncio.gather()
函数来并发地运行多个任务。以下是管理多个异步心跳发送任务的示例代码:
async def main():
tasks = [send_heartbeat() for _ in range(5)]
await asyncio.gather(*tasks)
asyncio.run(main())
六、使用 requests
模块发送心跳
1、引入 requests
模块
在某些应用场景中,可能需要通过 HTTP 协议发送心跳信号。可以使用 Python 的 requests
模块来实现这一点。requests
模块是一个简单易用的 HTTP 库,可以方便地发送 HTTP 请求。
2、发送 HTTP 心跳请求
首先,需要定义一个函数来发送 HTTP 心跳请求。以下是发送 HTTP 心跳请求的示例代码:
import requests
import time
def send_heartbeat():
url = 'http://localhost:8000/heartbeat' # 替换为实际的 URL
while True:
response = requests.post(url, data={'message': 'heartbeat'})
print(f'Sent: heartbeat, Response: {response.status_code}')
# 等待一段时间
time.sleep(5)
send_heartbeat()
3、处理异常情况
在实际应用中,网络请求可能会出现各种异常情况。因此,需要在代码中添加异常处理逻辑。以下是处理异常情况的示例代码:
import requests
import time
def send_heartbeat():
url = 'http://localhost:8000/heartbeat' # 替换为实际的 URL
while True:
try:
response = requests.post(url, data={'message': 'heartbeat'})
print(f'Sent: heartbeat, Response: {response.status_code}')
except requests.RequestException as e:
print(f'Exception: {e}')
# 等待一段时间
time.sleep(5)
send_heartbeat()
4、使用 threading
模块实现并发 HTTP 心跳请求
如果需要在多个线程中同时发送 HTTP 心跳请求,可以使用 threading
模块来实现。以下是使用 threading
模块实现并发 HTTP 心跳请求的示例代码:
import requests
import time
import threading
def send_heartbeat():
url = 'http://localhost:8000/heartbeat' # 替换为实际的 URL
while True:
try:
response = requests.post(url, data={'message': 'heartbeat'})
print(f'Sent: heartbeat, Response: {response.status_code}')
except requests.RequestException as e:
print(f'Exception: {e}')
# 等待一段时间
time.sleep(5)
创建线程池
num_threads = 5
threads = []
for i in range(num_threads):
heartbeat_thread = threading.Thread(target=send_heartbeat)
threads.append(heartbeat_thread)
heartbeat_thread.start()
等待所有线程完成
for thread in threads:
thread.join()
七、使用 aiohttp
实现异步 HTTP 心跳请求
1、引入 aiohttp
模块
在某些高并发应用中,异步 HTTP 请求可以提供更高的性能和更好的资源利用率。Python 的 aiohttp
模块允许编写异步 HTTP 客户端和服务器。在这里,可以使用 aiohttp
模块来实现异步 HTTP 心跳请求。
2、定义异步 HTTP 心跳请求函数
首先,需要定义一个异步函数来发送 HTTP 心跳请求。在这个函数中,可以使用 await
关键字来等待 I/O 操作完成。以下是定义异步 HTTP 心跳请求函数的示例代码:
import aiohttp
import asyncio
async def send_heartbeat():
url = 'http://localhost:8000/heartbeat' # 替换为实际的 URL
async with aiohttp.ClientSession() as session:
while True:
try:
async with session.post(url, data={'message': 'heartbeat'}) as response:
print(f'Sent: heartbeat, Response: {response.status}')
except aiohttp.ClientError as e:
print(f'Exception: {e}')
# 等待一段时间
await asyncio.sleep(5)
3、运行异步 HTTP 心跳请求函数
在定义好异步 HTTP 心跳请求函数之后,可以使用 asyncio.run()
函数来运行它。以下是运行异步 HTTP 心跳请求函数的示例代码:
asyncio.run(send_heartbeat())
4、管理多个异步 HTTP 心跳请求任务
如果需要管理多个异步 HTTP 心跳请求任务,可以使用 asyncio.gather()
函数来并发地运行多个任务。以下是管理多个异步 HTTP 心跳请求任务的示例代码:
async def main():
tasks = [send_heartbeat() for _ in range(5)]
await asyncio.gather(*tasks)
asyncio.run(main())
通过以上几种方法,可以有效地实现 Python 中的循环发送心跳信号。不同的方法适用于不同的应用场景,可以根据实际需求选择合适的实现方式。无论是哪种方法,关键都是通过循环、控制间隔时间、并发送心跳信号来确保连接的活跃状态。希望本文对你理解和实现循环发送心跳信号有所帮助。
相关问答FAQs:
如何在Python中实现心跳机制?
在Python中,可以使用定时器或者循环来实现心跳机制。通常,您可以使用time.sleep()
函数来控制发送心跳的间隔时间。可以使用while
循环来持续发送心跳信号,比如通过HTTP请求或Socket连接。示例代码如下:
import time
import requests
while True:
response = requests.get("http://your-server/heartbeat")
print("Heartbeat sent, response:", response.status_code)
time.sleep(5) # 每5秒发送一次心跳
在发送心跳时如何处理异常?
在进行网络请求时,处理异常是非常重要的。可以使用try-except
语句来捕获可能发生的异常,例如连接错误或超时。这样可以确保程序在遇到问题时不会崩溃,您可以选择重新尝试或记录错误信息。示例代码如下:
import time
import requests
while True:
try:
response = requests.get("http://your-server/heartbeat")
print("Heartbeat sent, response:", response.status_code)
except requests.exceptions.RequestException as e:
print("Error sending heartbeat:", e)
time.sleep(5) # 每5秒发送一次心跳
如何调整心跳间隔以适应不同场景?
心跳间隔的设置通常取决于应用的需求。例如,对于实时通信应用,您可能希望设置较短的间隔(如每1-2秒),而对于普通监控用途,较长的间隔(如每10-30秒)可能更合适。根据网络条件和系统负载,您可以通过调整time.sleep()
中的参数来实现这一点。确保在设置间隔时考虑到网络带宽和服务器的处理能力。
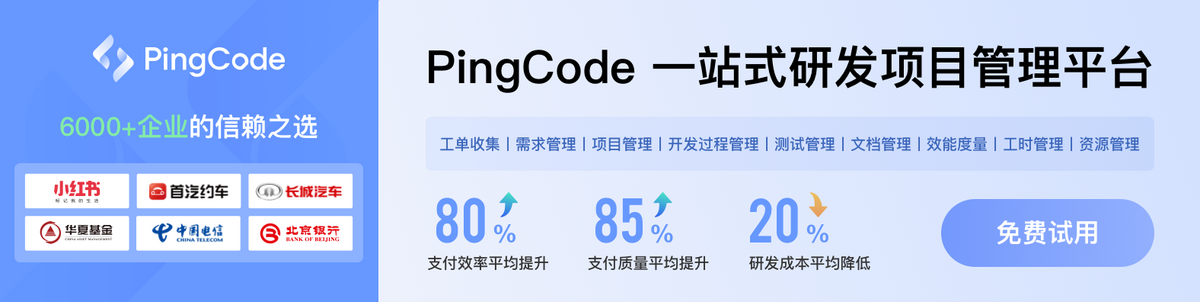