Python输出字典内容的方法有很多,主要包括使用print函数、使用for循环遍历字典、使用json模块进行美化输出、使用pprint模块进行美化输出、转换为字符串后输出。其中使用for循环遍历字典是最常用和灵活的一种方法。
一、使用print函数
使用print函数是最直接的方法,将字典变量传给print函数即可输出字典的内容。这种方法适用于字典内容不多且格式简单的情况。
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
print(my_dict)
二、使用for循环遍历字典
通过for循环遍历字典,可以分别输出字典的键和值,甚至可以按特定格式输出,适用于需要对字典内容进行更详细处理和格式化输出的情况。
1. 遍历字典的键和值
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
for key, value in my_dict.items():
print(f"{key}: {value}")
2. 遍历字典的键
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
for key in my_dict.keys():
print(f"Key: {key}")
3. 遍历字典的值
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
for value in my_dict.values():
print(f"Value: {value}")
三、使用json模块进行美化输出
json模块可以将字典转换为格式化的字符串输出,美化输出效果较好,适用于字典嵌套结构复杂的情况。
import json
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
print(json.dumps(my_dict, indent=4))
四、使用pprint模块进行美化输出
pprint模块是Python自带的一个专门用于美化输出的模块,适用于需要输出复杂字典结构的情况。
from pprint import pprint
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
pprint(my_dict)
五、转换为字符串后输出
将字典转换为字符串后进行输出,可以使用str()函数。这种方法适用于需要将字典内容输出到文件或其他数据流的情况。
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
print(str(my_dict))
六、综合实例
以下是一个综合实例,演示了如何使用以上方法输出字典内容,并展示了不同方法的效果。
import json
from pprint import pprint
my_dict = {
'name': 'Alice',
'age': 25,
'city': 'New York',
'hobbies': ['reading', 'travelling'],
'education': {
'degree': 'Bachelor',
'major': 'Computer Science'
}
}
使用print函数
print("Using print function:")
print(my_dict)
使用for循环遍历字典
print("\nUsing for loop:")
for key, value in my_dict.items():
print(f"{key}: {value}")
使用json模块进行美化输出
print("\nUsing json module:")
print(json.dumps(my_dict, indent=4))
使用pprint模块进行美化输出
print("\nUsing pprint module:")
pprint(my_dict)
转换为字符串后输出
print("\nUsing str() function:")
print(str(my_dict))
七、字典输出的应用场景
1. 日志记录
在开发过程中,常常需要将程序运行过程中的数据进行记录,字典输出是记录复杂数据结构的有效方法。
import logging
import json
logging.basicConfig(level=logging.DEBUG)
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
logging.debug(f"Dictionary content: {json.dumps(my_dict, indent=4)}")
2. 数据分析
在数据分析过程中,输出字典内容可以帮助分析数据结构和内容,便于后续处理和分析。
import pandas as pd
data = {
'name': ['Alice', 'Bob', 'Charlie'],
'age': [25, 30, 35],
'city': ['New York', 'Los Angeles', 'Chicago']
}
df = pd.DataFrame(data)
print("DataFrame content:")
print(df.to_dict())
3. 配置文件
在程序中经常需要读取和输出配置文件,配置文件通常使用字典结构存储,输出字典内容可以帮助调试和验证配置的正确性。
import json
config = {
'database': {
'host': 'localhost',
'port': 3306,
'user': 'root',
'password': 'password'
},
'logging': {
'level': 'DEBUG',
'file': 'app.log'
}
}
print("Configuration:")
print(json.dumps(config, indent=4))
八、字典输出的注意事项
1. 字典内容的隐私保护
在输出字典内容时,要注意保护敏感信息,如密码、密钥等,避免泄露隐私。
import json
config = {
'database': {
'host': 'localhost',
'port': 3306,
'user': 'root',
'password': 'password'
},
'logging': {
'level': 'DEBUG',
'file': 'app.log'
}
}
保护敏感信息
config['database']['password'] = '<strong></strong>'
print(json.dumps(config, indent=4))
2. 字典内容的格式化输出
在输出复杂嵌套结构的字典时,使用json或pprint模块进行格式化输出,可以提高可读性,便于分析和调试。
import json
from pprint import pprint
nested_dict = {
'level1': {
'level2': {
'level3': {
'key': 'value'
}
}
}
}
print("Using json module:")
print(json.dumps(nested_dict, indent=4))
print("\nUsing pprint module:")
pprint(nested_dict)
九、字典输出的高级应用
1. 自定义输出格式
在某些情况下,需要自定义字典内容的输出格式,可以通过自定义函数实现。
def custom_output(dictionary):
for key, value in dictionary.items():
if isinstance(value, dict):
print(f"{key}:")
custom_output(value)
else:
print(f" {key}: {value}")
nested_dict = {
'level1': {
'level2': {
'level3': {
'key': 'value'
}
}
}
}
custom_output(nested_dict)
2. 输出字典到文件
将字典内容输出到文件,可以用于保存数据或生成报告。
import json
my_dict = {
'name': 'Alice',
'age': 25,
'city': 'New York',
'hobbies': ['reading', 'travelling'],
'education': {
'degree': 'Bachelor',
'major': 'Computer Science'
}
}
with open('output.json', 'w') as file:
json.dump(my_dict, file, indent=4)
十、总结
Python输出字典内容的方法有很多,包括使用print函数、for循环遍历字典、json模块进行美化输出、pprint模块进行美化输出、转换为字符串后输出等。使用for循环遍历字典是最常用和灵活的一种方法,通过遍历字典的键和值,可以根据需要进行格式化输出。此外,json模块和pprint模块可以用于美化输出,提高可读性。输出字典内容的应用场景包括日志记录、数据分析、配置文件等。在输出字典内容时,要注意保护敏感信息,选择合适的输出格式,提高输出的可读性和实用性。
相关问答FAQs:
如何在Python中打印字典的所有键和值?
在Python中,可以使用for
循环遍历字典的键和值。通过items()
方法,您可以同时访问键和值。例如:
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
for key, value in my_dict.items():
print(f"{key}: {value}")
这种方式输出格式清晰,适合需要同时查看键和值的场景。
是否可以将字典内容以JSON格式输出?
是的,Python提供了json
模块,可以轻松将字典转换为JSON格式字符串。可以使用json.dumps()
方法实现:
import json
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
json_output = json.dumps(my_dict, indent=4) # indent用于格式化输出
print(json_output)
这种方法特别适合需要与其他系统或API交互时使用。
在Python中如何输出字典的特定键的值?
要输出字典中特定键的值,可以直接使用键作为索引访问相应的值。例如:
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
print(my_dict['name']) # 输出:Alice
在访问字典时,要确保所访问的键存在,避免引发KeyError
。可以使用get()
方法来安全地获取值:
age = my_dict.get('age', '未找到该键')
print(age)
这样可以提供一个默认值,避免程序崩溃。
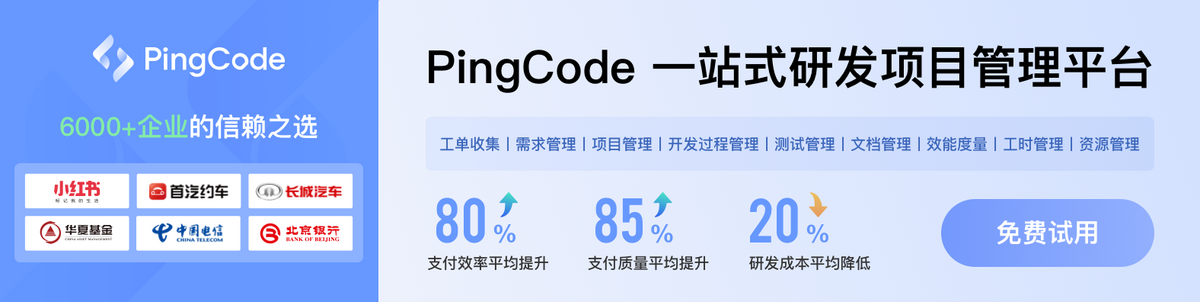