用Python实现温度转换的方法包括:使用简单的数学公式进行转换、创建函数来处理转换逻辑、利用类和对象进行温度转换。其中,使用简单的数学公式进行转换是最基础和直接的方法,可以帮助我们快速理解温度转换的基本逻辑。
例如,从摄氏度(Celsius)转换到华氏度(Fahrenheit)使用公式:F = C * 9/5 + 32。这个公式的作用是将摄氏度的温度值转换为相对应的华氏度温度值。通过这个公式,可以轻松地将输入的摄氏度值转换为华氏度值。以下是如何使用Python实现这个转换的详细示例:
def celsius_to_fahrenheit(celsius):
return celsius * 9/5 + 32
示例使用
celsius_temp = 25
fahrenheit_temp = celsius_to_fahrenheit(celsius_temp)
print(f"{celsius_temp} 摄氏度等于 {fahrenheit_temp} 华氏度")
这个简单的函数实现了从摄氏度到华氏度的转换。接下来将介绍更多的温度转换方法和技巧。
一、基础温度转换公式
1、摄氏度到华氏度
从摄氏度转换到华氏度的公式是:F = C * 9/5 + 32。这是一个线性转换公式,乘以9/5是将摄氏度转化为华氏度的比例,增加32是将比例值调整到华氏度的基准点。
def celsius_to_fahrenheit(celsius):
return celsius * 9/5 + 32
2、华氏度到摄氏度
从华氏度转换到摄氏度的公式是:C = (F – 32) * 5/9。这个公式通过先减去32,然后乘以5/9来转换华氏度到摄氏度。
def fahrenheit_to_celsius(fahrenheit):
return (fahrenheit - 32) * 5/9
3、摄氏度到开尔文
从摄氏度转换到开尔文的公式是:K = C + 273.15。开尔文是绝对温度单位,转换时需要在摄氏度基础上增加273.15。
def celsius_to_kelvin(celsius):
return celsius + 273.15
4、开尔文到摄氏度
从开尔文转换到摄氏度的公式是:C = K – 273.15。这个公式通过减去273.15来将开尔文转换为摄氏度。
def kelvin_to_celsius(kelvin):
return kelvin - 273.15
5、华氏度到开尔文
从华氏度转换到开尔文的公式是:K = (F – 32) * 5/9 + 273.15。这个公式结合了华氏度到摄氏度的转换以及摄氏度到开尔文的转换。
def fahrenheit_to_kelvin(fahrenheit):
return (fahrenheit - 32) * 5/9 + 273.15
6、开尔文到华氏度
从开尔文转换到华氏度的公式是:F = (K – 273.15) * 9/5 + 32。这个公式结合了开尔文到摄氏度的转换以及摄氏度到华氏度的转换。
def kelvin_to_fahrenheit(kelvin):
return (kelvin - 273.15) * 9/5 + 32
二、使用函数进行温度转换
使用函数进行温度转换不仅可以简化代码,还可以提高代码的可读性和可维护性。可以根据不同的转换需求创建不同的函数。
1、综合温度转换函数
为了方便,我们可以编写一个综合的温度转换函数,根据输入的温度和目标单位进行转换。
def convert_temperature(value, from_unit, to_unit):
if from_unit == 'C':
if to_unit == 'F':
return celsius_to_fahrenheit(value)
elif to_unit == 'K':
return celsius_to_kelvin(value)
elif from_unit == 'F':
if to_unit == 'C':
return fahrenheit_to_celsius(value)
elif to_unit == 'K':
return fahrenheit_to_kelvin(value)
elif from_unit == 'K':
if to_unit == 'C':
return kelvin_to_celsius(value)
elif to_unit == 'F':
return kelvin_to_fahrenheit(value)
return None
示例使用
print(convert_temperature(25, 'C', 'F')) # 输出: 77.0
print(convert_temperature(77, 'F', 'C')) # 输出: 25.0
print(convert_temperature(300, 'K', 'C')) # 输出: 26.85
2、带校验的温度转换函数
为了提高函数的健壮性,可以在函数中增加输入校验,确保输入的温度值和单位都是有效的。
def convert_temperature(value, from_unit, to_unit):
if not isinstance(value, (int, float)):
raise ValueError("温度值必须是数字")
valid_units = ['C', 'F', 'K']
if from_unit not in valid_units or to_unit not in valid_units:
raise ValueError("无效的温度单位")
if from_unit == 'C':
if to_unit == 'F':
return celsius_to_fahrenheit(value)
elif to_unit == 'K':
return celsius_to_kelvin(value)
elif from_unit == 'F':
if to_unit == 'C':
return fahrenheit_to_celsius(value)
elif to_unit == 'K':
return fahrenheit_to_kelvin(value)
elif from_unit == 'K':
if to_unit == 'C':
return kelvin_to_celsius(value)
elif to_unit == 'F':
return kelvin_to_fahrenheit(value)
return None
示例使用
try:
print(convert_temperature(25, 'C', 'F')) # 输出: 77.0
print(convert_temperature(77, 'F', 'C')) # 输出: 25.0
print(convert_temperature(300, 'K', 'C')) # 输出: 26.85
print(convert_temperature(25, 'C', 'X')) # 引发错误: 无效的温度单位
except ValueError as e:
print(e)
三、使用类和对象进行温度转换
面向对象编程(OOP)是另一种实现温度转换的有效方法。通过定义类和方法,可以更好地组织和管理代码。
1、定义温度转换类
可以定义一个 TemperatureConverter
类,包含各种温度转换方法。
class TemperatureConverter:
@staticmethod
def celsius_to_fahrenheit(celsius):
return celsius * 9/5 + 32
@staticmethod
def fahrenheit_to_celsius(fahrenheit):
return (fahrenheit - 32) * 5/9
@staticmethod
def celsius_to_kelvin(celsius):
return celsius + 273.15
@staticmethod
def kelvin_to_celsius(kelvin):
return kelvin - 273.15
@staticmethod
def fahrenheit_to_kelvin(fahrenheit):
return (fahrenheit - 32) * 5/9 + 273.15
@staticmethod
def kelvin_to_fahrenheit(kelvin):
return (kelvin - 273.15) * 9/5 + 32
2、使用温度转换类进行转换
通过实例化或直接使用类方法,可以进行温度转换。
# 示例使用
print(TemperatureConverter.celsius_to_fahrenheit(25)) # 输出: 77.0
print(TemperatureConverter.fahrenheit_to_celsius(77)) # 输出: 25.0
print(TemperatureConverter.celsius_to_kelvin(25)) # 输出: 298.15
3、改进温度转换类
可以进一步改进 TemperatureConverter
类,添加更通用的转换方法和输入校验。
class TemperatureConverter:
@staticmethod
def convert_temperature(value, from_unit, to_unit):
if not isinstance(value, (int, float)):
raise ValueError("温度值必须是数字")
valid_units = ['C', 'F', 'K']
if from_unit not in valid_units or to_unit not in valid_units:
raise ValueError("无效的温度单位")
if from_unit == 'C':
if to_unit == 'F':
return TemperatureConverter.celsius_to_fahrenheit(value)
elif to_unit == 'K':
return TemperatureConverter.celsius_to_kelvin(value)
elif from_unit == 'F':
if to_unit == 'C':
return TemperatureConverter.fahrenheit_to_celsius(value)
elif to_unit == 'K':
return TemperatureConverter.fahrenheit_to_kelvin(value)
elif from_unit == 'K':
if to_unit == 'C':
return TemperatureConverter.kelvin_to_celsius(value)
elif to_unit == 'F':
return TemperatureConverter.kelvin_to_fahrenheit(value)
return None
@staticmethod
def celsius_to_fahrenheit(celsius):
return celsius * 9/5 + 32
@staticmethod
def fahrenheit_to_celsius(fahrenheit):
return (fahrenheit - 32) * 5/9
@staticmethod
def celsius_to_kelvin(celsius):
return celsius + 273.15
@staticmethod
def kelvin_to_celsius(kelvin):
return kelvin - 273.15
@staticmethod
def fahrenheit_to_kelvin(fahrenheit):
return (fahrenheit - 32) * 5/9 + 273.15
@staticmethod
def kelvin_to_fahrenheit(kelvin):
return (kelvin - 273.15) * 9/5 + 32
示例使用
try:
print(TemperatureConverter.convert_temperature(25, 'C', 'F')) # 输出: 77.0
print(TemperatureConverter.convert_temperature(77, 'F', 'C')) # 输出: 25.0
print(TemperatureConverter.convert_temperature(300, 'K', 'C')) # 输出: 26.85
print(TemperatureConverter.convert_temperature(25, 'C', 'X')) # 引发错误: 无效的温度单位
except ValueError as e:
print(e)
四、图形用户界面(GUI)实现温度转换
除了在命令行界面(CLI)进行温度转换,还可以使用图形用户界面(GUI)来实现更直观的温度转换。Python的 tkinter
库可以帮助我们实现这一点。
1、创建基本的GUI窗口
首先,创建一个基本的GUI窗口,并添加输入框和按钮。
import tkinter as tk
from tkinter import messagebox
class TemperatureConverterGUI:
def __init__(self, root):
self.root = root
self.root.title("温度转换器")
self.value_label = tk.Label(root, text="温度值:")
self.value_label.pack()
self.value_entry = tk.Entry(root)
self.value_entry.pack()
self.from_unit_label = tk.Label(root, text="从单位:")
self.from_unit_label.pack()
self.from_unit_entry = tk.Entry(root)
self.from_unit_entry.pack()
self.to_unit_label = tk.Label(root, text="到单位:")
self.to_unit_label.pack()
self.to_unit_entry = tk.Entry(root)
self.to_unit_entry.pack()
self.convert_button = tk.Button(root, text="转换", command=self.convert)
self.convert_button.pack()
self.result_label = tk.Label(root, text="")
self.result_label.pack()
def convert(self):
try:
value = float(self.value_entry.get())
from_unit = self.from_unit_entry.get().upper()
to_unit = self.to_unit_entry.get().upper()
result = TemperatureConverter.convert_temperature(value, from_unit, to_unit)
self.result_label.config(text=f"结果: {result} {to_unit}")
except ValueError as e:
messagebox.showerror("错误", str(e))
if __name__ == "__main__":
root = tk.Tk()
app = TemperatureConverterGUI(root)
root.mainloop()
2、改进GUI界面
可以进一步改进GUI界面,增加单位选择的下拉菜单和更友好的用户提示。
import tkinter as tk
from tkinter import ttk
from tkinter import messagebox
class TemperatureConverterGUI:
def __init__(self, root):
self.root = root
self.root.title("温度转换器")
self.value_label = tk.Label(root, text="温度值:")
self.value_label.pack()
self.value_entry = tk.Entry(root)
self.value_entry.pack()
self.from_unit_label = tk.Label(root, text="从单位:")
self.from_unit_label.pack()
self.from_unit_combobox = ttk.Combobox(root, values=["C", "F", "K"])
self.from_unit_combobox.pack()
self.to_unit_label = tk.Label(root, text="到单位:")
self.to_unit_label.pack()
self.to_unit_combobox = ttk.Combobox(root, values=["C", "F", "K"])
self.to_unit_combobox.pack()
self.convert_button = tk.Button(root, text="转换", command=self.convert)
self.convert_button.pack()
self.result_label = tk.Label(root, text="")
self.result_label.pack()
def convert(self):
try:
value = float(self.value_entry.get())
from_unit = self.from_unit_combobox.get()
to_unit = self.to_unit_combobox.get()
result = TemperatureConverter.convert_temperature(value, from_unit, to_unit)
self.result_label.config(text=f"结果: {result} {to_unit}")
except ValueError as e:
messagebox.showerror("错误", str(e))
if __name__ == "__main__":
root = tk.Tk()
app = TemperatureConverterGUI(root)
root.mainloop()
五、总结
通过本文的介绍,您已经了解了如何使用Python实现温度转换的多种方法,从最基础的数学公式,到使用函数、类和对象,再到图形用户界面的实现。使用简单的数学公式进行转换可以快速上手,而使用函数和类则能提高代码的组织性和可维护性。而通过图形用户界面,可以提供更友好的用户体验。希望本文能帮助您更好地理解和掌握Python温度转换的实现方法。
相关问答FAQs:
如何在Python中进行华氏度和摄氏度之间的转换?
在Python中,华氏度和摄氏度之间的转换公式分别为:摄氏度 = (华氏度 – 32) * 5/9,华氏度 = 摄氏度 * 9/5 + 32。可以通过定义函数来实现这些转换。例如,您可以创建一个函数接受温度值和单位作为参数,返回转换后的温度。
使用Python进行温度转换时,如何处理输入错误?
在进行温度转换时,输入数据的有效性很重要。可以使用异常处理来捕获输入错误,例如非数字值或不支持的温度单位。通过使用try
和except
语句,您可以确保程序在遇到错误时不会崩溃,并向用户提供友好的错误提示。
有没有现成的Python库可以帮助我实现温度转换?
是的,有一些现成的Python库可以帮助您进行温度转换。例如,pint
库提供了单位转换的功能,支持多种物理量的转换,包括温度。您只需安装该库并使用它提供的API来轻松进行温度单位的转换。
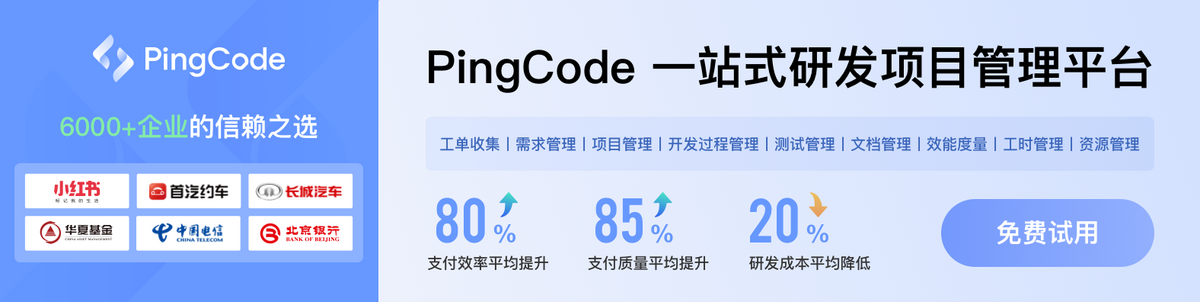