使用Python抓取微博的方法包括使用微博API、模拟登录并爬取、使用第三方库。其中,使用微博API 是最常见且规范的方式。接下来,我们将详细介绍如何使用微博API来抓取微博数据。
一、注册微博开发者账号并申请API
1、注册微博开发者账号
首先,你需要在微博开放平台(http://open.weibo.com/)注册一个开发者账号。注册完成后,登录你的账号。
2、创建应用
在微博开放平台中,选择“管理中心”,点击“创建应用”。在创建应用时,你需要填写一些必要的信息,如应用名称、应用描述、应用类别等。创建完成后,你将获得应用的App Key和App Secret,这两个信息在后续的API调用中非常重要。
3、获取Access Token
在进行API调用之前,需要获取Access Token。你可以在“管理中心”下的“我的应用”中找到你创建的应用,点击“应用详情”,然后点击“授权设置”来获取Access Token。你需要设置一个回调地址,用于授权成功后的跳转。
通过以下方式获取Access Token:
- 打开授权页面:https://api.weibo.com/oauth2/authorize?client_id=YOUR_APP_KEY&redirect_uri=YOUR_CALLBACK_URL&response_type=code
- 用户登录并授权后,会跳转到回调地址,并带有code参数。
- 使用code获取Access Token:
import requests
url = 'https://api.weibo.com/oauth2/access_token'
data = {
'client_id': 'YOUR_APP_KEY',
'client_secret': 'YOUR_APP_SECRET',
'grant_type': 'authorization_code',
'code': 'CODE_FROM_CALLBACK_URL',
'redirect_uri': 'YOUR_CALLBACK_URL'
}
response = requests.post(url, data=data)
access_token = response.json()['access_token']
二、使用API抓取微博数据
1、获取用户信息
获取用户信息是抓取微博数据的基础,首先我们可以通过用户ID或用户名来获取用户的基本信息:
import requests
def get_user_info(access_token, uid):
url = 'https://api.weibo.com/2/users/show.json'
params = {
'access_token': access_token,
'uid': uid
}
response = requests.get(url, params=params)
return response.json()
示例
user_info = get_user_info(access_token, 'USER_ID')
print(user_info)
2、获取用户微博
获取用户微博主要是通过调用statuses/user_timeline接口:
def get_user_timeline(access_token, uid, count=10):
url = 'https://api.weibo.com/2/statuses/user_timeline.json'
params = {
'access_token': access_token,
'uid': uid,
'count': count
}
response = requests.get(url, params=params)
return response.json()
示例
user_timeline = get_user_timeline(access_token, 'USER_ID')
for status in user_timeline['statuses']:
print(status['text'])
3、获取热门微博
获取热门微博可以使用statuses/public_timeline接口:
def get_public_timeline(access_token, count=10):
url = 'https://api.weibo.com/2/statuses/public_timeline.json'
params = {
'access_token': access_token,
'count': count
}
response = requests.get(url, params=params)
return response.json()
示例
public_timeline = get_public_timeline(access_token)
for status in public_timeline['statuses']:
print(status['text'])
三、模拟登录并爬取微博
有时,微博API的限制可能无法满足我们所有的需求,这时候我们可以考虑模拟登录并爬取微博。需要注意的是,这种方式可能会违反微博的使用协议,使用时需谨慎。
1、使用Selenium模拟登录
Selenium是一个用于自动化浏览器操作的工具,可以用于模拟登录微博:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
import time
def login_weibo(username, password):
driver = webdriver.Chrome()
driver.get('https://weibo.com/login.php')
time.sleep(5)
username_field = driver.find_element(By.NAME, 'username')
password_field = driver.find_element(By.NAME, 'password')
username_field.send_keys(username)
password_field.send_keys(password)
password_field.send_keys(Keys.RETURN)
time.sleep(5)
return driver
示例
driver = login_weibo('YOUR_USERNAME', 'YOUR_PASSWORD')
2、抓取微博内容
登录成功后,我们可以使用Selenium来抓取微博内容:
def get_weibo_content(driver, url):
driver.get(url)
time.sleep(5)
weibo_contents = driver.find_elements(By.CSS_SELECTOR, '.WB_text')
for content in weibo_contents:
print(content.text)
示例
get_weibo_content(driver, 'https://weibo.com/u/YOUR_USER_ID')
四、使用第三方库
还有一些第三方库可以帮助我们简化抓取微博的过程,比如weibo
库。这个库封装了微博API,可以更方便地进行数据抓取。
1、安装weibo库
pip install weibo
2、使用weibo库抓取微博数据
from weibo import Client
client = Client(api_key='YOUR_APP_KEY', api_secret='YOUR_APP_SECRET', redirect_uri='YOUR_CALLBACK_URL')
client.set_code('CODE_FROM_CALLBACK_URL')
获取用户信息
user_info = client.get('users/show', uid='USER_ID')
print(user_info)
获取用户微博
user_timeline = client.get('statuses/user_timeline', uid='USER_ID', count=10)
for status in user_timeline['statuses']:
print(status['text'])
获取热门微博
public_timeline = client.get('statuses/public_timeline', count=10)
for status in public_timeline['statuses']:
print(status['text'])
五、数据存储与处理
抓取到的数据可以存储到本地文件或数据库中,以便后续处理和分析。
1、存储到本地文件
import json
def save_to_file(data, filename):
with open(filename, 'w', encoding='utf-8') as f:
json.dump(data, f, ensure_ascii=False, indent=4)
示例
save_to_file(user_timeline, 'user_timeline.json')
2、存储到数据库
可以使用SQLite或其他数据库来存储抓取到的数据:
import sqlite3
def save_to_db(data, db_name='weibo.db'):
conn = sqlite3.connect(db_name)
c = conn.cursor()
c.execute('''CREATE TABLE IF NOT EXISTS weibo
(id INTEGER PRIMARY KEY, text TEXT)''')
for status in data['statuses']:
c.execute("INSERT INTO weibo (id, text) VALUES (?, ?)", (status['id'], status['text']))
conn.commit()
conn.close()
示例
save_to_db(user_timeline)
六、数据分析与可视化
抓取到的数据可以进行各种分析和可视化操作,以获取有价值的信息。
1、词频分析
可以使用jieba
库进行中文分词,并使用collections
库进行词频统计:
import jieba
from collections import Counter
def analyze_word_frequency(texts):
words = []
for text in texts:
words.extend(jieba.cut(text))
word_count = Counter(words)
return word_count
示例
texts = [status['text'] for status in user_timeline['statuses']]
word_count = analyze_word_frequency(texts)
print(word_count.most_common(10))
2、情感分析
可以使用snownlp
库进行情感分析:
from snownlp import SnowNLP
def analyze_sentiment(texts):
sentiments = []
for text in texts:
s = SnowNLP(text)
sentiments.append(s.sentiments)
return sentiments
示例
sentiments = analyze_sentiment(texts)
print(sentiments)
3、可视化
可以使用matplotlib
或pandas
进行数据可视化:
import matplotlib.pyplot as plt
def plot_word_frequency(word_count):
words, counts = zip(*word_count.most_common(10))
plt.bar(words, counts)
plt.show()
示例
plot_word_frequency(word_count)
七、总结
通过上述步骤,我们可以使用Python抓取微博数据,并进行数据存储、分析和可视化。无论是使用微博API、模拟登录还是第三方库,数据的合法性和隐私保护都需要我们特别注意。在实际应用中,抓取到的数据可以帮助我们进行各种有价值的分析和研究。
相关问答FAQs:
如何选择合适的库来抓取微博数据?
在使用Python抓取微博数据时,可以选择多个库,如Requests、BeautifulSoup和Scrapy等。Requests库可以帮助你发送HTTP请求,获取网页内容,而BeautifulSoup则可以解析HTML文档,提取所需的信息。Scrapy是一个功能强大的框架,适合处理大规模数据抓取。根据你的需求和抓取复杂度,选择最适合的库。
抓取微博时需要注意哪些法律法规?
在抓取微博数据时,遵循相关法律法规非常重要。确保遵循数据保护法和隐私政策,避免抓取用户的私人信息。此外,了解微博的使用条款,确保不违反平台的限制,如频繁请求可能导致账号被封禁。
如何处理抓取过程中遇到的反爬虫机制?
微博等社交平台通常会实施反爬虫机制。为了应对这些挑战,可以采取多种策略,如设置请求头以模拟浏览器行为、使用代理IP来隐藏真实地址、以及控制请求频率以避免被识别为爬虫。此外,使用随机延迟也有助于降低被封禁的风险。
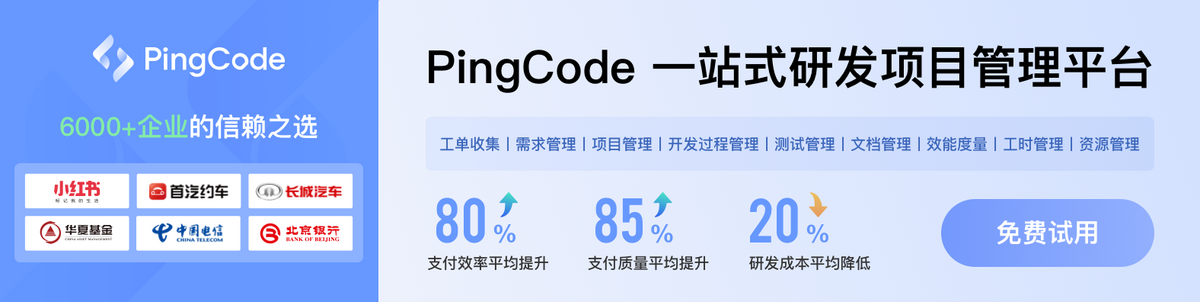