Python进行多次选择的方法包括:if-elif-else语句、字典映射、函数映射、选择结构嵌套。 使用if-elif-else语句是最常见的方法,通过多个条件判断来实现多次选择。字典映射则利用字典的键值对来管理选择项,更加高效和清晰。函数映射则通过将函数作为字典的值,进一步简化代码结构。选择结构嵌套则是在复杂场景下的组合使用。
一、IF-ELIF-ELSE语句
基本用法
if-elif-else语句是Python中最常见的多次选择结构,通过多个条件判断来实现不同的选择路径。基本用法如下:
choice = input("Enter your choice: ")
if choice == '1':
print("You chose option 1")
elif choice == '2':
print("You chose option 2")
elif choice == '3':
print("You chose option 3")
else:
print("Invalid choice")
在这个例子中,根据用户输入的不同选项,程序会执行相应的代码块。如果输入的选项不符合任何一个条件,则执行else部分。
复杂条件判断
if-elif-else语句不仅可以用于简单的选择,还可以处理复杂的条件判断。例如:
score = int(input("Enter your score: "))
if score >= 90:
grade = 'A'
elif score >= 80:
grade = 'B'
elif score >= 70:
grade = 'C'
elif score >= 60:
grade = 'D'
else:
grade = 'F'
print(f"Your grade is {grade}")
这种方式适用于需要根据多个条件进行分类的场景,如成绩等级评定、不同情况下的错误处理等。
二、字典映射
基本用法
字典映射是一种更为简洁和高效的多次选择方法,特别适用于条件较多且条件值已知的情况。以下是一个基本示例:
def option1():
print("You chose option 1")
def option2():
print("You chose option 2")
def option3():
print("You chose option 3")
options = {
'1': option1,
'2': option2,
'3': option3
}
choice = input("Enter your choice: ")
if choice in options:
options[choice]()
else:
print("Invalid choice")
在这个例子中,我们使用字典将选择项与对应的函数关联起来,然后根据用户输入调用相应的函数。这种方法可以使代码更加清晰和易于维护。
动态映射
字典映射还可以用于动态生成选择项。例如:
def add(a, b):
return a + b
def subtract(a, b):
return a - b
def multiply(a, b):
return a * b
def divide(a, b):
return a / b
operations = {
'1': add,
'2': subtract,
'3': multiply,
'4': divide
}
a = float(input("Enter first number: "))
b = float(input("Enter second number: "))
choice = input("Enter your choice (1:Add, 2:Subtract, 3:Multiply, 4:Divide): ")
if choice in operations:
result = operations[choice](a, b)
print(f"The result is: {result}")
else:
print("Invalid choice")
在这个例子中,字典映射不仅关联了选择项,还动态调用了相应的函数进行计算。
三、函数映射
基本用法
函数映射是一种将选择项与函数直接关联的方法,适用于需要频繁调用不同函数的场景。以下是一个基本示例:
def greet():
print("Hello!")
def farewell():
print("Goodbye!")
def unknown():
print("Unknown option")
def choose_option(option):
options = {
'greet': greet,
'farewell': farewell
}
return options.get(option, unknown)
choice = input("Enter your choice (greet/farewell): ")
choose_option(choice)()
在这个例子中,我们定义了一个函数choose_option,它根据用户输入返回相应的函数。如果输入不在选项之内,则返回unknown函数。
复杂场景应用
函数映射还可以用于更复杂的场景,例如状态机的实现:
class StateMachine:
def __init__(self):
self.state = 'start'
def start(self):
print("Starting...")
self.state = 'process'
def process(self):
print("Processing...")
self.state = 'end'
def end(self):
print("Ending...")
self.state = 'done'
def run(self):
while self.state != 'done':
state_method = getattr(self, self.state)
state_method()
machine = StateMachine()
machine.run()
在这个例子中,我们使用函数映射实现了一个简单的状态机,根据当前状态动态调用相应的方法。
四、选择结构嵌套
基本用法
选择结构嵌套是指在一个选择结构中嵌套另一个选择结构,适用于需要多层次判断的复杂场景。以下是一个基本示例:
choice1 = input("Enter your first choice (A/B): ")
if choice1 == 'A':
choice2 = input("Enter your second choice (1/2): ")
if choice2 == '1':
print("You chose A1")
elif choice2 == '2':
print("You chose A2")
else:
print("Invalid second choice")
elif choice1 == 'B':
choice2 = input("Enter your second choice (1/2): ")
if choice2 == '1':
print("You chose B1")
elif choice2 == '2':
print("You chose B2")
else:
print("Invalid second choice")
else:
print("Invalid first choice")
在这个例子中,我们根据用户的第一个选择进行第二次选择,从而实现更复杂的多次选择结构。
深层嵌套
选择结构嵌套还可以用于更深层次的判断,例如多级菜单:
def main_menu():
print("Main Menu:")
print("1. Option 1")
print("2. Option 2")
choice = input("Enter your choice: ")
if choice == '1':
sub_menu_1()
elif choice == '2':
sub_menu_2()
else:
print("Invalid choice")
main_menu()
def sub_menu_1():
print("Sub Menu 1:")
print("1. Sub Option 1")
print("2. Sub Option 2")
choice = input("Enter your choice: ")
if choice == '1':
print("You chose Sub Option 1")
elif choice == '2':
print("You chose Sub Option 2")
else:
print("Invalid choice")
sub_menu_1()
def sub_menu_2():
print("Sub Menu 2:")
print("1. Sub Option 1")
print("2. Sub Option 2")
choice = input("Enter your choice: ")
if choice == '1':
print("You chose Sub Option 1")
elif choice == '2':
print("You chose Sub Option 2")
else:
print("Invalid choice")
sub_menu_2()
main_menu()
在这个例子中,我们实现了一个多级菜单系统,根据用户的选择逐层进入不同的子菜单。
五、综合应用
结合多种方法
在实际项目中,我们可以结合多种多次选择方法,以实现更加灵活和高效的选择结构。例如:
def add(a, b):
return a + b
def subtract(a, b):
return a - b
def multiply(a, b):
return a * b
def divide(a, b):
if b != 0:
return a / b
else:
return "Division by zero is not allowed"
operations = {
'1': add,
'2': subtract,
'3': multiply,
'4': divide
}
def main_menu():
print("Main Menu:")
print("1. Add")
print("2. Subtract")
print("3. Multiply")
print("4. Divide")
print("5. Exit")
choice = input("Enter your choice: ")
if choice in operations:
a = float(input("Enter first number: "))
b = float(input("Enter second number: "))
result = operations[choice](a, b)
print(f"The result is: {result}")
elif choice == '5':
print("Exiting...")
return
else:
print("Invalid choice")
main_menu()
main_menu()
在这个例子中,我们结合了函数映射和选择结构嵌套,实现了一个功能丰富的计算器程序。
实现复杂业务逻辑
在一些复杂的业务场景中,我们可能需要结合多种选择方法来实现业务逻辑。例如,在一个电商系统中,根据用户的角色和操作类型进行不同的处理:
def admin_add_product():
print("Admin: Adding a product")
def admin_remove_product():
print("Admin: Removing a product")
def user_view_product():
print("User: Viewing a product")
def user_purchase_product():
print("User: Purchasing a product")
def invalid_operation():
print("Invalid operation")
operations = {
'admin': {
'add': admin_add_product,
'remove': admin_remove_product
},
'user': {
'view': user_view_product,
'purchase': user_purchase_product
}
}
def main_menu():
role = input("Enter your role (admin/user): ")
operation = input("Enter your operation (add/remove/view/purchase): ")
if role in operations and operation in operations[role]:
operations[role][operation]()
else:
invalid_operation()
main_menu()
main_menu()
在这个例子中,我们根据用户的角色和操作类型动态调用相应的函数,实现了一个简单的权限管理和操作处理系统。
六、实战案例
案例一:学生成绩管理系统
我们可以使用多次选择方法来实现一个学生成绩管理系统,支持添加学生、查看成绩、更新成绩和删除学生等功能。
students = {}
def add_student():
name = input("Enter student's name: ")
score = float(input("Enter student's score: "))
students[name] = score
print(f"Student {name} added with score {score}")
def view_scores():
if not students:
print("No students found")
else:
for name, score in students.items():
print(f"{name}: {score}")
def update_score():
name = input("Enter student's name to update: ")
if name in students:
score = float(input("Enter new score: "))
students[name] = score
print(f"Student {name}'s score updated to {score}")
else:
print(f"Student {name} not found")
def delete_student():
name = input("Enter student's name to delete: ")
if name in students:
del students[name]
print(f"Student {name} deleted")
else:
print(f"Student {name} not found")
def main_menu():
print("Student Management System:")
print("1. Add Student")
print("2. View Scores")
print("3. Update Score")
print("4. Delete Student")
print("5. Exit")
choice = input("Enter your choice: ")
if choice == '1':
add_student()
elif choice == '2':
view_scores()
elif choice == '3':
update_score()
elif choice == '4':
delete_student()
elif choice == '5':
print("Exiting...")
return
else:
print("Invalid choice")
main_menu()
main_menu()
在这个例子中,我们使用if-elif-else语句和函数映射,实现了一个功能完整的学生成绩管理系统。
案例二:任务管理系统
我们还可以使用多次选择方法来实现一个任务管理系统,支持添加任务、查看任务、更新任务和删除任务等功能。
tasks = {}
def add_task():
task_id = input("Enter task ID: ")
task_desc = input("Enter task description: ")
tasks[task_id] = task_desc
print(f"Task {task_id} added with description '{task_desc}'")
def view_tasks():
if not tasks:
print("No tasks found")
else:
for task_id, task_desc in tasks.items():
print(f"{task_id}: {task_desc}")
def update_task():
task_id = input("Enter task ID to update: ")
if task_id in tasks:
task_desc = input("Enter new task description: ")
tasks[task_id] = task_desc
print(f"Task {task_id} updated to '{task_desc}'")
else:
print(f"Task {task_id} not found")
def delete_task():
task_id = input("Enter task ID to delete: ")
if task_id in tasks:
del tasks[task_id]
print(f"Task {task_id} deleted")
else:
print(f"Task {task_id} not found")
def main_menu():
print("Task Management System:")
print("1. Add Task")
print("2. View Tasks")
print("3. Update Task")
print("4. Delete Task")
print("5. Exit")
choice = input("Enter your choice: ")
if choice == '1':
add_task()
elif choice == '2':
view_tasks()
elif choice == '3':
update_task()
elif choice == '4':
delete_task()
elif choice == '5':
print("Exiting...")
return
else:
print("Invalid choice")
main_menu()
main_menu()
在这个例子中,我们同样使用if-elif-else语句和函数映射,实现了一个功能完整的任务管理系统。
七、总结
多次选择结构是编程中非常常见的一种逻辑结构,Python提供了多种实现方法,包括if-elif-else语句、字典映射、函数映射和选择结构嵌套等。根据实际需求选择合适的方法,可以使代码更加简洁、清晰和高效。在复杂的业务场景中,可以结合多种方法灵活应用,以实现复杂的业务逻辑。通过实战案例,可以更好地理解和掌握这些方法的使用技巧。
相关问答FAQs:
如何在Python中实现多次选择的功能?
在Python中,可以使用input()
函数结合循环和条件语句来实现多次选择。通过创建一个菜单,用户可以根据提示输入选项,程序根据用户的输入执行相应的操作。使用while
循环可以让用户反复选择,直到他们决定退出。
Python中有哪些库可以帮助实现多次选择的功能?
Python中有多个库可以帮助实现多次选择。例如,cmd
库可以用于构建命令行应用,允许用户输入多次选择。click
库也提供了更友好的命令行界面,支持多次选择和参数处理。使用这些库可以让你的程序更易于使用。
如何处理用户输入错误的情况?
在处理多次选择时,用户可能会输入无效选项。可以使用try-except
语句来捕获异常,或通过条件语句检查输入的有效性。提供明确的错误提示和重新输入的机会,有助于提升用户体验。例如,可以在用户输入不符合预期时,提示用户重新选择有效选项。
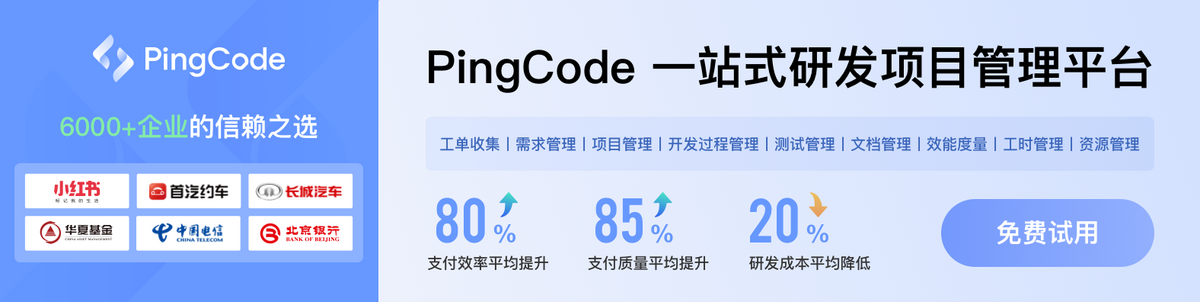