要使用Python获取XMind主题数据,可以通过读取XMind文件、解析XMind文件结构、提取主题数据来实现。以下是详细的步骤和方法:
一、读取XMind文件
首先,需要读取XMind文件。XMind文件实际上是一个压缩包,包含了多个文件和文件夹。可以使用Python的zipfile模块来读取XMind文件。
import zipfile
def read_xmind_file(xmind_file_path):
with zipfile.ZipFile(xmind_file_path, 'r') as z:
z.extractall('xmind_content')
二、解析XMind文件结构
XMind文件主要包含content.xml
文件,该文件包含了XMind思维导图的主要内容。可以使用Python的xml.etree.ElementTree模块来解析XML文件。
import xml.etree.ElementTree as ET
def parse_xmind_content(content_file_path):
tree = ET.parse(content_file_path)
return tree.getroot()
三、提取主题数据
XMind文件的主题数据包含在<topic>
标签中,可以通过递归方式遍历XML树来提取主题数据。
def extract_topics(element):
topics = []
for topic in element.findall('.//topic'):
topic_data = {
'title': topic.find('title').text,
'id': topic.get('id'),
'children': extract_topics(topic)
}
topics.append(topic_data)
return topics
四、结合所有步骤获取XMind主题数据
最后,将上述步骤结合起来,编写一个完整的函数来获取XMind主题数据。
import zipfile
import xml.etree.ElementTree as ET
def read_xmind_file(xmind_file_path):
with zipfile.ZipFile(xmind_file_path, 'r') as z:
z.extractall('xmind_content')
def parse_xmind_content(content_file_path):
tree = ET.parse(content_file_path)
return tree.getroot()
def extract_topics(element):
topics = []
for topic in element.findall('.//topic'):
topic_data = {
'title': topic.find('title').text,
'id': topic.get('id'),
'children': extract_topics(topic)
}
topics.append(topic_data)
return topics
def get_xmind_topics(xmind_file_path):
read_xmind_file(xmind_file_path)
root = parse_xmind_content('xmind_content/content.xml')
return extract_topics(root)
示例使用
xmind_file_path = 'example.xmind'
topics = get_xmind_topics(xmind_file_path)
print(topics)
通过上述方法,可以从XMind文件中提取出主题数据,并将其存储在一个嵌套的字典列表中。这种方法不仅可以提取主题的标题,还可以提取主题的ID和子主题信息。
深入解析XMind文件结构
XMind文件的结构较为复杂,除了content.xml
文件外,还包含其他一些文件,如meta.xml
、styles.xml
等。这些文件可以提供更多的信息,如XMind文件的元数据和样式信息。
1、解析meta.xml文件
meta.xml
文件包含了XMind文件的一些元数据,如创建时间、修改时间、作者等信息。可以使用xml.etree.ElementTree模块来解析该文件。
def parse_meta_file(meta_file_path):
tree = ET.parse(meta_file_path)
root = tree.getroot()
meta_data = {
'creator': root.find('creator').text,
'created_time': root.find('created-time').text,
'modified_time': root.find('modified-time').text
}
return meta_data
2、解析styles.xml文件
styles.xml
文件包含了XMind文件的样式信息,如主题的颜色、字体等。可以使用xml.etree.ElementTree模块来解析该文件。
def parse_styles_file(styles_file_path):
tree = ET.parse(styles_file_path)
root = tree.getroot()
styles = []
for style in root.findall('style'):
style_data = {
'id': style.get('id'),
'type': style.get('type'),
'properties': {prop.tag: prop.text for prop in style.find('properties')}
}
styles.append(style_data)
return styles
3、整合所有解析步骤
最终,可以将所有的解析步骤整合到一个函数中,以便于获取XMind文件的所有信息。
import zipfile
import xml.etree.ElementTree as ET
def read_xmind_file(xmind_file_path):
with zipfile.ZipFile(xmind_file_path, 'r') as z:
z.extractall('xmind_content')
def parse_xmind_content(content_file_path):
tree = ET.parse(content_file_path)
return tree.getroot()
def extract_topics(element):
topics = []
for topic in element.findall('.//topic'):
topic_data = {
'title': topic.find('title').text,
'id': topic.get('id'),
'children': extract_topics(topic)
}
topics.append(topic_data)
return topics
def parse_meta_file(meta_file_path):
tree = ET.parse(meta_file_path)
root = tree.getroot()
meta_data = {
'creator': root.find('creator').text,
'created_time': root.find('created-time').text,
'modified_time': root.find('modified-time').text
}
return meta_data
def parse_styles_file(styles_file_path):
tree = ET.parse(styles_file_path)
root = tree.getroot()
styles = []
for style in root.findall('style'):
style_data = {
'id': style.get('id'),
'type': style.get('type'),
'properties': {prop.tag: prop.text for prop in style.find('properties')}
}
styles.append(style_data)
return styles
def get_xmind_data(xmind_file_path):
read_xmind_file(xmind_file_path)
content_root = parse_xmind_content('xmind_content/content.xml')
meta_data = parse_meta_file('xmind_content/meta.xml')
styles = parse_styles_file('xmind_content/styles.xml')
topics = extract_topics(content_root)
return {
'meta_data': meta_data,
'styles': styles,
'topics': topics
}
示例使用
xmind_file_path = 'example.xmind'
xmind_data = get_xmind_data(xmind_file_path)
print(xmind_data)
处理复杂的XMind主题结构
在实际应用中,XMind文件的主题结构可能非常复杂,包含多个层级的子主题和关联信息。为了更好地处理这些复杂结构,可以进行一些优化和扩展。
1、处理主题的备注和标签
除了主题的标题和ID外,主题还可能包含备注和标签信息。可以通过解析<notes>
和<labels>
标签来获取这些信息。
def extract_topics(element):
topics = []
for topic in element.findall('.//topic'):
notes = topic.find('notes')
labels = topic.find('labels')
topic_data = {
'title': topic.find('title').text,
'id': topic.get('id'),
'notes': notes.text if notes is not None else None,
'labels': labels.text.split(',') if labels is not None else [],
'children': extract_topics(topic)
}
topics.append(topic_data)
return topics
2、处理关联关系
XMind文件中还可能包含主题之间的关联关系,可以通过解析<relationships>
标签来获取这些信息。
def extract_relationships(element):
relationships = []
for relationship in element.findall('.//relationship'):
relationship_data = {
'id': relationship.get('id'),
'end1_id': relationship.get('end1-id'),
'end2_id': relationship.get('end2-id')
}
relationships.append(relationship_data)
return relationships
3、整合复杂结构的解析步骤
最终,可以将处理复杂结构的解析步骤整合到一个函数中,以便于获取XMind文件的所有信息。
import zipfile
import xml.etree.ElementTree as ET
def read_xmind_file(xmind_file_path):
with zipfile.ZipFile(xmind_file_path, 'r') as z:
z.extractall('xmind_content')
def parse_xmind_content(content_file_path):
tree = ET.parse(content_file_path)
return tree.getroot()
def extract_topics(element):
topics = []
for topic in element.findall('.//topic'):
notes = topic.find('notes')
labels = topic.find('labels')
topic_data = {
'title': topic.find('title').text,
'id': topic.get('id'),
'notes': notes.text if notes is not None else None,
'labels': labels.text.split(',') if labels is not None else [],
'children': extract_topics(topic)
}
topics.append(topic_data)
return topics
def extract_relationships(element):
relationships = []
for relationship in element.findall('.//relationship'):
relationship_data = {
'id': relationship.get('id'),
'end1_id': relationship.get('end1-id'),
'end2_id': relationship.get('end2-id')
}
relationships.append(relationship_data)
return relationships
def parse_meta_file(meta_file_path):
tree = ET.parse(meta_file_path)
root = tree.getroot()
meta_data = {
'creator': root.find('creator').text,
'created_time': root.find('created-time').text,
'modified_time': root.find('modified-time').text
}
return meta_data
def parse_styles_file(styles_file_path):
tree = ET.parse(styles_file_path)
root = tree.getroot()
styles = []
for style in root.findall('style'):
style_data = {
'id': style.get('id'),
'type': style.get('type'),
'properties': {prop.tag: prop.text for prop in style.find('properties')}
}
styles.append(style_data)
return styles
def get_xmind_data(xmind_file_path):
read_xmind_file(xmind_file_path)
content_root = parse_xmind_content('xmind_content/content.xml')
meta_data = parse_meta_file('xmind_content/meta.xml')
styles = parse_styles_file('xmind_content/styles.xml')
topics = extract_topics(content_root)
relationships = extract_relationships(content_root)
return {
'meta_data': meta_data,
'styles': styles,
'topics': topics,
'relationships': relationships
}
示例使用
xmind_file_path = 'example.xmind'
xmind_data = get_xmind_data(xmind_file_path)
print(xmind_data)
总结
通过上述方法,可以使用Python全面解析XMind文件并获取其主题数据。这包括读取XMind文件、解析XMind文件结构、提取主题数据、处理复杂的主题结构、解析关联关系,以及获取元数据和样式信息。这些方法和步骤提供了一个完整的解决方案,适用于各种复杂度的XMind文件解析需求。
相关问答FAQs:
如何使用Python读取Xmind文件中的主题数据?
要读取Xmind文件中的主题数据,可以使用xmindparser
库,它允许你轻松解析Xmind文件并提取主题信息。首先,确保你已安装该库,可以通过pip install xmindparser
来安装。接下来,使用代码加载Xmind文件并获取主题内容,示例如下:
from xmindparser import xmind_to_dict
xmind_data = xmind_to_dict('your_file.xmind')
themes = xmind_data[0]['topic']['topics'] # 提取主题数据
Xmind文件的格式是什么?我需要了解哪些内容才能处理它?
Xmind文件通常是以压缩格式保存的,里面包含多个文件和文件夹,主要包括主题、备注、标签等信息。了解Xmind的结构和不同组件之间的关系,将有助于你更有效地提取和使用主题数据。
在Python中处理Xmind主题数据时,有哪些常见的挑战?
处理Xmind主题数据时,常见的挑战包括文件格式不兼容、数据提取不完整和主题层级关系的复杂性。为了应对这些问题,确保使用最新版本的解析库,并仔细阅读文档,理解数据结构。可以使用调试工具来检查提取的数据是否符合预期。
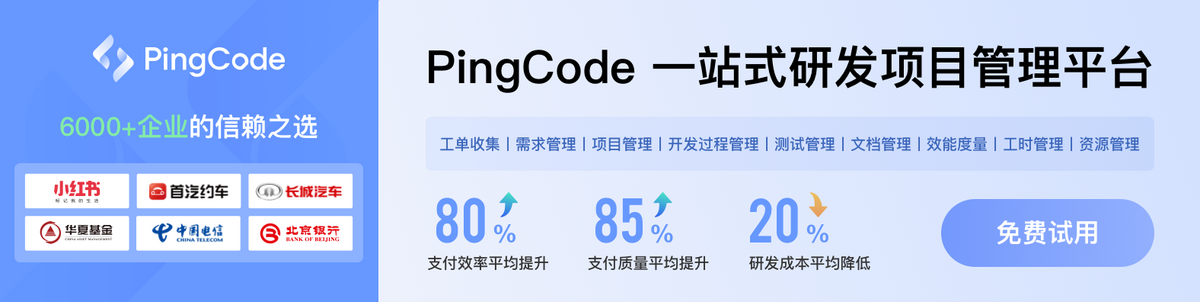