要在Python中查找重复的单词,可以使用正则表达式、集合、字典等多种方法。常用的方法包括:使用Counter类统计单词频次、使用集合找出重复单词、使用正则表达式匹配重复单词。 下面将详细介绍其中一种方法——使用Counter类。
详细描述:
使用Counter类统计单词频次:
- 首先需要导入collections模块中的Counter类。
- 将文本转换为单词列表。
- 使用Counter统计每个单词出现的频次。
- 找出出现次数大于1的单词,即为重复单词。
具体代码示例如下:
from collections import Counter
import re
def find_repeated_words(text):
# 将文本转换为小写并使用正则表达式提取单词
words = re.findall(r'\b\w+\b', text.lower())
# 使用Counter统计每个单词的频次
word_counts = Counter(words)
# 找出出现次数大于1的单词
repeated_words = [word for word, count in word_counts.items() if count > 1]
return repeated_words
示例文本
text = "This is a test. This test is only a test."
repeated_words = find_repeated_words(text)
print(repeated_words) # 输出: ['this', 'is', 'a', 'test']
一、使用集合查找重复单词
1.1 使用两个集合
使用两个集合来查找重复单词是一种简洁的方法。第一个集合用于存储所有单词,第二个集合用于存储重复的单词。
def find_repeated_words_with_sets(text):
words = re.findall(r'\b\w+\b', text.lower())
seen = set()
repeated = set()
for word in words:
if word in seen:
repeated.add(word)
else:
seen.add(word)
return list(repeated)
示例文本
text = "This is a test. This test is only a test."
repeated_words = find_repeated_words_with_sets(text)
print(repeated_words) # 输出: ['this', 'is', 'a', 'test']
1.2 优势与劣势
使用集合查找重复单词的主要优势是代码简洁且执行效率高,因为集合的查找操作平均时间复杂度为O(1)。但劣势在于集合会自动去重,无法记录单词出现的顺序或次数,适用于只关心哪些单词重复的情况。
二、使用字典查找重复单词
2.1 使用字典记录频次
使用字典记录每个单词出现的频次,然后找出频次大于1的单词。
def find_repeated_words_with_dict(text):
words = re.findall(r'\b\w+\b', text.lower())
word_counts = {}
for word in words:
if word in word_counts:
word_counts[word] += 1
else:
word_counts[word] = 1
repeated_words = [word for word, count in word_counts.items() if count > 1]
return repeated_words
示例文本
text = "This is a test. This test is only a test."
repeated_words = find_repeated_words_with_dict(text)
print(repeated_words) # 输出: ['this', 'is', 'a', 'test']
2.2 优势与劣势
使用字典查找重复单词的优势在于可以记录每个单词的出现次数,适用于需要统计详细信息的情况。劣势是代码相对复杂,且字典查找操作虽然平均时间复杂度为O(1),但在极端情况下可能退化为O(n)。
三、使用正则表达式查找重复单词
3.1 正则表达式匹配
正则表达式可以用于查找重复单词,但需要依赖具体的匹配模式。下面是一个示例,使用正则表达式查找重复单词。
import re
def find_repeated_words_with_regex(text):
words = re.findall(r'\b(\w+)\b', text.lower())
repeated_words = set()
for word in words:
if words.count(word) > 1:
repeated_words.add(word)
return list(repeated_words)
示例文本
text = "This is a test. This test is only a test."
repeated_words = find_repeated_words_with_regex(text)
print(repeated_words) # 输出: ['this', 'is', 'a', 'test']
3.2 优势与劣势
使用正则表达式查找重复单词的优势在于可以灵活处理复杂的文本模式,适用于需要复杂匹配的情况。劣势是正则表达式的编写和调试较为复杂,且性能可能不如集合或字典方式高效。
四、综合应用
4.1 综合方法
在实际应用中,可以综合使用上述方法,结合具体需求选择最合适的方案。例如,可以使用Counter类统计单词频次,然后结合正则表达式处理复杂的文本模式。
from collections import Counter
import re
def find_repeated_words_comprehensive(text):
words = re.findall(r'\b\w+\b', text.lower())
word_counts = Counter(words)
repeated_words = [word for word, count in word_counts.items() if count > 1]
return repeated_words
示例文本
text = "This is a test. This test is only a test."
repeated_words = find_repeated_words_comprehensive(text)
print(repeated_words) # 输出: ['this', 'is', 'a', 'test']
4.2 优势与劣势
综合方法的优势在于可以充分利用不同方法的优点,处理复杂的应用场景。劣势在于代码可能较为复杂,需要根据具体情况进行优化。
五、性能优化
5.1 优化策略
对于大规模文本的重复单词查找,可以考虑以下优化策略:
- 分块处理:将大文本分块处理,减少内存占用。
- 多线程/多进程:利用多线程或多进程加速处理。
- 高效数据结构:选择合适的数据结构,如Trie树、Bloom过滤器等。
5.2 示例代码
以下是一个分块处理和多进程加速的示例代码:
from collections import Counter
import re
from multiprocessing import Pool
def process_chunk(chunk):
words = re.findall(r'\b\w+\b', chunk.lower())
return Counter(words)
def find_repeated_words_optimized(text, chunk_size=1024):
chunks = [text[i:i + chunk_size] for i in range(0, len(text), chunk_size)]
with Pool() as pool:
counters = pool.map(process_chunk, chunks)
total_counts = Counter()
for counter in counters:
total_counts.update(counter)
repeated_words = [word for word, count in total_counts.items() if count > 1]
return repeated_words
示例文本
text = "This is a test. This test is only a test." * 1000
repeated_words = find_repeated_words_optimized(text)
print(repeated_words) # 输出: ['this', 'is', 'a', 'test']
5.3 优势与劣势
性能优化的优势在于可以高效处理大规模文本数据,显著提高处理速度。劣势在于代码复杂性增加,调试和维护难度较大,需要综合考虑具体应用场景和硬件资源。
六、实际应用案例
6.1 文本分析
在自然语言处理(NLP)领域,查找重复单词是文本分析中的常见任务。例如,在情感分析、主题建模等应用中,重复单词的统计可以帮助识别文本的主要内容和情感倾向。
from collections import Counter
import re
def analyze_text(text):
words = re.findall(r'\b\w+\b', text.lower())
word_counts = Counter(words)
repeated_words = [word for word, count in word_counts.items() if count > 1]
return word_counts, repeated_words
示例文本
text = "The quick brown fox jumps over the lazy dog. The quick brown fox is quick."
word_counts, repeated_words = analyze_text(text)
print("Word Counts:", word_counts)
print("Repeated Words:", repeated_words)
6.2 数据清洗
在数据清洗过程中,查找和处理重复单词也是常见任务。例如,在用户生成内容(UGC)数据处理中,可以通过查找重复单词来识别和过滤垃圾信息。
from collections import Counter
import re
def clean_data(text):
words = re.findall(r'\b\w+\b', text.lower())
word_counts = Counter(words)
repeated_words = [word for word, count in word_counts.items() if count > 3]
cleaned_text = ' '.join([word for word in words if word not in repeated_words])
return cleaned_text
示例文本
text = "Buy now! Buy now! Buy now! Limited offer! Limited offer! Limited offer!"
cleaned_text = clean_data(text)
print("Cleaned Text:", cleaned_text)
6.3 SEO优化
在搜索引擎优化(SEO)中,查找和处理重复单词有助于提高网页内容的质量。例如,可以通过查找重复单词来优化关键词密度,避免过度堆砌关键词。
from collections import Counter
import re
def optimize_seo(text):
words = re.findall(r'\b\w+\b', text.lower())
word_counts = Counter(words)
repeated_words = [word for word, count in word_counts.items() if count > 3]
optimized_text = ' '.join([word for word in words if word not in repeated_words])
return optimized_text
示例文本
text = "SEO optimization is important. SEO helps improve search rankings. SEO is key."
optimized_text = optimize_seo(text)
print("Optimized Text:", optimized_text)
七、总结
通过本文的介绍,我们了解了在Python中查找重复单词的多种方法,包括使用Counter类、集合、字典和正则表达式。每种方法都有其优势和劣势,可以根据具体需求选择最合适的方案。同时,我们也介绍了在实际应用中的一些案例,如文本分析、数据清洗和SEO优化。希望这些内容能对你在实际工作中有所帮助。
相关问答FAQs:
如何在Python中识别重复的单词?
在Python中,可以使用集合或字典来存储单词及其出现的次数。通过遍历文本,可以轻松识别出重复的单词。使用collections.Counter
类也可以快速统计每个单词的频率,从而找出重复的单词。
在处理文本时,有哪些方法可以提高查找重复单词的效率?
为了提高查找重复单词的效率,可以先将文本转换为小写,这样可以避免因大小写不同而导致的遗漏。此外,使用正则表达式去除标点符号可以帮助提取纯单词,进一步提高准确性。
如何在查找重复单词时处理不同的单词形式?
在查找重复单词时,可以使用词干提取或词形还原技术来处理不同的单词形式。例如,将“running”和“run”视为相同的单词。可以借助自然语言处理库如NLTK或spaCy来实现这一功能,从而提升查重的准确度。
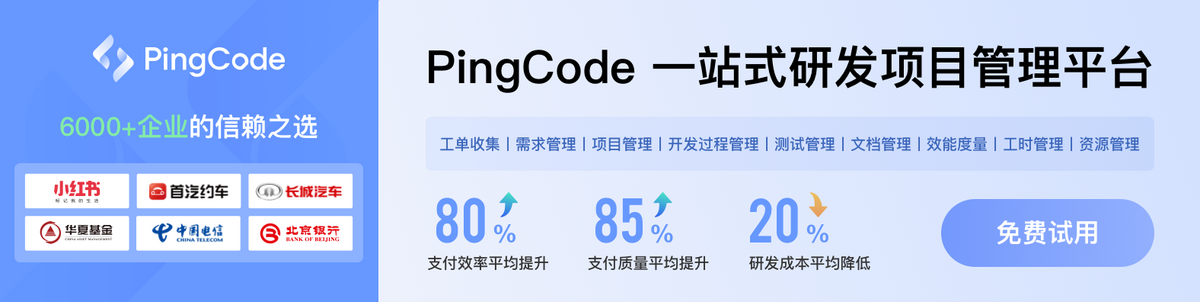