要用Python编写网络爬虫,首先需要了解一些核心工具和步骤。使用requests库发送HTTP请求、利用BeautifulSoup解析HTML内容、处理反爬虫机制,其中利用requests库发送HTTP请求是最基础且重要的一步。
使用requests库发送HTTP请求
requests库是Python中最常用的HTTP库之一。通过它,我们可以轻松地向目标网站发送HTTP请求,并获取响应内容。以下是一个简单的示例,展示了如何使用requests库发送GET请求并获取网页内容:
import requests
url = 'http://example.com'
response = requests.get(url)
if response.status_code == 200:
print(response.text)
else:
print('Failed to retrieve the webpage')
在这个示例中,我们首先导入了requests库,然后定义了目标URL。通过requests.get(url)
发送GET请求,并将响应保存到response
变量中。如果请求成功(即状态码为200),我们将打印网页内容。
一、了解HTTP请求和响应
在编写网络爬虫之前,了解HTTP请求和响应是至关重要的。HTTP请求通常包括GET、POST、PUT、DELETE等方法。GET请求用于获取资源,而POST请求用于提交数据。每个请求都会返回一个HTTP响应,其中包含状态码、响应头和响应体。
GET请求
GET请求用于从服务器获取数据。以下是一个使用requests库发送GET请求的示例:
import requests
url = 'http://example.com'
response = requests.get(url)
print('Status Code:', response.status_code)
print('Response Headers:', response.headers)
print('Response Body:', response.text)
POST请求
POST请求用于向服务器提交数据。以下是一个使用requests库发送POST请求的示例:
import requests
url = 'http://example.com/login'
data = {'username': 'user', 'password': 'pass'}
response = requests.post(url, data=data)
print('Status Code:', response.status_code)
print('Response Headers:', response.headers)
print('Response Body:', response.text)
二、解析HTML内容
获取网页内容后,下一步是解析HTML内容。BeautifulSoup是一个流行的Python库,它提供了简单的API来解析和遍历HTML文档。
安装BeautifulSoup
使用pip安装BeautifulSoup:
pip install beautifulsoup4
pip install lxml
使用BeautifulSoup解析HTML
以下是一个使用BeautifulSoup解析HTML并提取所有链接的示例:
import requests
from bs4 import BeautifulSoup
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'lxml')
links = soup.find_all('a')
for link in links:
print(link.get('href'))
在这个示例中,我们首先发送GET请求并获取网页内容。然后,我们使用BeautifulSoup解析HTML文档,并提取所有的链接。
三、处理反爬虫机制
许多网站都有反爬虫机制,以防止大量自动化请求。为了绕过这些机制,我们需要使用一些技巧,如模拟浏览器行为、添加请求头、使用代理等。
模拟浏览器行为
通过添加User-Agent请求头,可以模拟浏览器行为。以下是一个示例:
import requests
url = 'http://example.com'
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
print(response.text)
使用代理
使用代理可以隐藏真实IP地址,从而避免被网站封禁。以下是一个示例:
import requests
url = 'http://example.com'
proxies = {'http': 'http://10.10.1.10:3128', 'https': 'http://10.10.1.10:1080'}
response = requests.get(url, proxies=proxies)
print(response.text)
四、存储数据
爬取的数据通常需要存储到本地或数据库中。我们可以使用Python的内置文件操作函数、SQLite、MySQL等来存储数据。
存储到本地文件
以下是一个将数据存储到本地文件的示例:
import requests
from bs4 import BeautifulSoup
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'lxml')
links = soup.find_all('a')
with open('links.txt', 'w') as file:
for link in links:
file.write(link.get('href') + '\n')
存储到SQLite数据库
以下是一个将数据存储到SQLite数据库的示例:
import requests
from bs4 import BeautifulSoup
import sqlite3
创建SQLite数据库和表
conn = sqlite3.connect('data.db')
c = conn.cursor()
c.execute('''CREATE TABLE IF NOT EXISTS links (url TEXT)''')
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'lxml')
links = soup.find_all('a')
for link in links:
c.execute('INSERT INTO links (url) VALUES (?)', (link.get('href'),))
conn.commit()
conn.close()
五、处理多页面爬取
在实际应用中,往往需要爬取多个页面的数据。这时可以使用循环或递归来遍历所有页面。
使用循环遍历页面
以下是一个使用循环遍历页面并爬取数据的示例:
import requests
from bs4 import BeautifulSoup
base_url = 'http://example.com/page/'
for i in range(1, 11):
url = base_url + str(i)
response = requests.get(url)
soup = BeautifulSoup(response.text, 'lxml')
links = soup.find_all('a')
for link in links:
print(link.get('href'))
使用递归遍历页面
以下是一个使用递归遍历页面并爬取数据的示例:
import requests
from bs4 import BeautifulSoup
def crawl_page(url, page):
full_url = url + str(page)
response = requests.get(full_url)
soup = BeautifulSoup(response.text, 'lxml')
links = soup.find_all('a')
for link in links:
print(link.get('href'))
# 假设存在"Next"按钮链接到下一页
next_page = soup.find('a', text='Next')
if next_page:
crawl_page(url, page + 1)
base_url = 'http://example.com/page/'
crawl_page(base_url, 1)
六、处理动态内容
有些网站使用JavaScript动态加载内容。对于这种情况,可以使用Selenium等工具来模拟浏览器行为,执行JavaScript并获取完整的网页内容。
安装Selenium
使用pip安装Selenium:
pip install selenium
还需要下载对应的浏览器驱动,如ChromeDriver。
使用Selenium获取动态内容
以下是一个使用Selenium获取动态内容的示例:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
设置Chrome选项
options = webdriver.ChromeOptions()
options.add_argument('--headless') # 无头模式
启动Chrome浏览器
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()), options=options)
url = 'http://example.com'
driver.get(url)
等待页面加载完成
driver.implicitly_wait(10)
获取动态内容
content = driver.page_source
print(content)
关闭浏览器
driver.quit()
七、提高爬取效率
在大规模爬取时,效率是一个重要的考虑因素。我们可以使用多线程或异步编程来提高爬取效率。
使用多线程
以下是一个使用多线程爬取数据的示例:
import requests
from bs4 import BeautifulSoup
from concurrent.futures import ThreadPoolExecutor
def fetch_url(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'lxml')
links = soup.find_all('a')
for link in links:
print(link.get('href'))
base_url = 'http://example.com/page/'
urls = [base_url + str(i) for i in range(1, 11)]
with ThreadPoolExecutor(max_workers=5) as executor:
executor.map(fetch_url, urls)
使用异步编程
以下是一个使用aiohttp和asyncio进行异步爬取的示例:
import aiohttp
import asyncio
from bs4 import BeautifulSoup
async def fetch_url(session, url):
async with session.get(url) as response:
text = await response.text()
soup = BeautifulSoup(text, 'lxml')
links = soup.find_all('a')
for link in links:
print(link.get('href'))
async def main():
base_url = 'http://example.com/page/'
urls = [base_url + str(i) for i in range(1, 11)]
async with aiohttp.ClientSession() as session:
tasks = [fetch_url(session, url) for url in urls]
await asyncio.gather(*tasks)
asyncio.run(main())
八、爬虫策略与道德规范
在编写和运行网络爬虫时,需要遵守一些策略和道德规范,如尊重网站的robots.txt文件、控制爬取频率、避免对服务器造成过大压力等。
遵守robots.txt
robots.txt是网站管理员用来告诉爬虫哪些页面可以爬取、哪些不可以爬取的文件。我们可以使用robots.txt来确定爬取策略。
import requests
from urllib.robotparser import RobotFileParser
url = 'http://example.com'
robots_url = url + '/robots.txt'
response = requests.get(robots_url)
robots_parser = RobotFileParser()
robots_parser.parse(response.text.split('\n'))
if robots_parser.can_fetch('*', url):
print('Allowed to fetch the URL')
else:
print('Not allowed to fetch the URL')
控制爬取频率
为了避免对服务器造成过大压力,我们需要控制爬取频率。可以使用time.sleep()函数来设置请求间隔。
import time
import requests
url = 'http://example.com/page/'
for i in range(1, 11):
response = requests.get(url + str(i))
print(response.status_code)
time.sleep(1) # 间隔1秒
九、处理异常
在爬取过程中,可能会遇到各种异常,如请求超时、连接错误等。需要对这些异常进行处理,以保证爬虫的稳定性。
捕获请求异常
以下是一个捕获请求异常的示例:
import requests
url = 'http://example.com'
try:
response = requests.get(url)
response.raise_for_status()
except requests.exceptions.RequestException as e:
print(f'Request failed: {e}')
设置重试机制
可以使用retrying库来设置重试机制,以应对临时性错误。
from retrying import retry
import requests
@retry(stop_max_attempt_number=3, wait_fixed=2000)
def fetch_url(url):
response = requests.get(url)
response.raise_for_status()
return response.text
url = 'http://example.com'
try:
content = fetch_url(url)
print(content)
except requests.exceptions.RequestException as e:
print(f'Failed to fetch the URL after retries: {e}')
十、总结
通过本文,我们介绍了如何使用Python编写网络爬虫,从发送HTTP请求、解析HTML内容到处理反爬虫机制、存储数据等各个方面进行了详细说明。使用requests库发送HTTP请求、利用BeautifulSoup解析HTML内容、处理反爬虫机制是编写网络爬虫的核心步骤。希望这些内容能够帮助你更好地理解和实现网络爬虫。
相关问答FAQs:
使用Python编写网络爬虫需要哪些基本知识?
要成功编写网络爬虫,首先需要掌握Python编程语言的基础知识,包括数据结构、控制流和函数等。了解HTTP协议的基本原理也是必不可少的,因其是网络爬虫与网页交互的基础。此外,熟悉使用常见的库如Requests(用于发送网络请求)和Beautiful Soup(用于解析HTML和XML文档)将大大提高你的爬虫效率。学习正则表达式可以帮助你更好地处理和提取数据。
如何处理网络爬虫中的反爬机制?
许多网站为了保护其数据,会实施反爬机制。应对这些机制的策略包括使用随机的User-Agent头部来伪装请求、设置合理的请求间隔以避免频繁访问、使用代理IP池来隐藏真实IP等。还可以使用动态请求库如Selenium来模拟浏览器行为,从而绕过某些简单的反爬措施。此外,保持对目标网站的使用条款的尊重,避免过于频繁的请求,以免被封禁。
Python网络爬虫的常见应用场景有哪些?
Python网络爬虫广泛应用于数据采集和分析。比如,可以用于抓取电商网站上的商品信息和价格波动,以便进行市场分析。也可以用来收集社交媒体上的用户评论和互动数据,帮助企业进行品牌监测和舆情分析。此外,爬虫还可以用于新闻网站的内容聚合,或是获取学术论文和技术文档,以便于进行信息挖掘和研究。
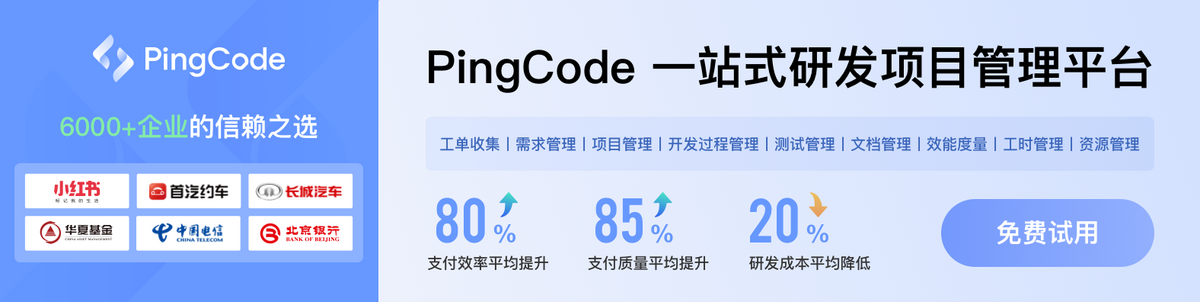