在Python中加入文字的方法有很多,如使用字符串、字符串拼接、格式化字符串等。常见的方法包括使用字符串变量、字符串格式化、f-string格式化、以及结合字符串操作函数和模块。 通过这些方法,可以方便地在Python程序中加入、拼接和处理文本。下面将详细介绍其中的一种方法:字符串格式化。
字符串格式化方法可以通过使用%
操作符、str.format()
方法或f-string(格式化字符串)来实现。在Python 3.6及以上版本中,f-string提供了一种更简洁的方式来格式化字符串。下面是一个示例:
name = "Alice"
age = 30
message = f"Hello, my name is {name} and I am {age} years old."
print(message)
示例中,使用了f-string格式化字符串,将变量name
和age
的值插入到字符串中,从而生成了一条包含这些变量值的消息。
一、字符串操作基础
Python是一种非常适合处理文本的编程语言,字符串是Python最基础的数据类型之一。字符串可以通过单引号(')、双引号(")或三引号('''或""")来定义。以下是一些基本的字符串操作:
1.1、创建字符串
字符串可以通过直接赋值给变量来创建:
string1 = 'Hello, World!'
string2 = "Python is awesome!"
string3 = '''This is a
multi-line string.'''
string4 = """This is another
multi-line string."""
1.2、字符串拼接
字符串可以通过加号(+)进行拼接:
str1 = "Hello"
str2 = "World"
result = str1 + ", " + str2 + "!"
print(result) # 输出: Hello, World!
1.3、字符串重复
可以使用乘号(*)来重复字符串:
str1 = "Hello"
result = str1 * 3
print(result) # 输出: HelloHelloHello
二、字符串格式化
字符串格式化是将变量的值插入到字符串中的一种方法。Python提供了多种字符串格式化的方法,下面详细介绍几种常用的方法。
2.1、使用百分号(%)操作符
这是Python早期的字符串格式化方法,通过使用%
操作符来插入变量的值:
name = "Alice"
age = 30
message = "Hello, my name is %s and I am %d years old." % (name, age)
print(message) # 输出: Hello, my name is Alice and I am 30 years old.
2.2、使用str.format()方法
str.format()
方法是Python 3中引入的一种格式化字符串的方法:
name = "Alice"
age = 30
message = "Hello, my name is {} and I am {} years old.".format(name, age)
print(message) # 输出: Hello, my name is Alice and I am 30 years old.
也可以通过指定位置或关键字来格式化字符串:
name = "Alice"
age = 30
message = "Hello, my name is {0} and I am {1} years old.".format(name, age)
print(message) # 输出: Hello, my name is Alice and I am 30 years old.
message = "Hello, my name is {name} and I am {age} years old.".format(name=name, age=age)
print(message) # 输出: Hello, my name is Alice and I am 30 years old.
2.3、使用f-string(格式化字符串)
f-string是Python 3.6及以上版本中引入的一种更简洁的字符串格式化方法,通过在字符串前加上字母f
或F
,并在大括号内插入变量名或表达式:
name = "Alice"
age = 30
message = f"Hello, my name is {name} and I am {age} years old."
print(message) # 输出: Hello, my name is Alice and I am 30 years old.
f-string还支持表达式和函数调用:
a = 5
b = 10
message = f"The sum of {a} and {b} is {a + b}."
print(message) # 输出: The sum of 5 and 10 is 15.
三、字符串操作函数和模块
Python提供了丰富的字符串操作函数和模块,可以方便地对字符串进行各种操作。
3.1、常用字符串方法
以下是一些常用的字符串方法:
str.upper()
:将字符串转换为大写字母。str.lower()
:将字符串转换为小写字母。str.capitalize()
:将字符串的第一个字母转换为大写。str.strip()
:去除字符串两端的空格或指定字符。str.replace(old, new)
:将字符串中的指定子字符串替换为新的子字符串。str.split(sep)
:将字符串按照指定分隔符分割为列表。str.join(iterable)
:将可迭代对象中的元素连接成一个字符串。
示例:
text = " Hello, World! "
print(text.upper()) # 输出: " HELLO, WORLD! "
print(text.lower()) # 输出: " hello, world! "
print(text.capitalize()) # 输出: " hello, world! "
print(text.strip()) # 输出: "Hello, World!"
print(text.replace("World", "Python")) # 输出: " Hello, Python! "
print(text.split(",")) # 输出: [' Hello', ' World! ']
words = ["Hello", "World"]
print(" ".join(words)) # 输出: "Hello World"
3.2、字符串模块
Python的string
模块提供了一些有用的常量和函数来处理字符串:
import string
print(string.ascii_letters) # 输出: 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'
print(string.digits) # 输出: '0123456789'
print(string.punctuation) # 输出: '!"#$%&\'()*+,-./:;<=>?@[\\]^_`{|}~'
四、处理多行字符串和文本文件
在处理多行字符串和文本文件时,Python提供了许多方便的方法来读取、写入和操作文本内容。
4.1、处理多行字符串
多行字符串可以通过三引号('''或""")来定义:
multi_line_string = """This is a
multi-line string."""
print(multi_line_string)
4.2、读取和写入文本文件
Python的内建函数open()
可以用来读取和写入文本文件:
# 写入文本文件
with open("example.txt", "w") as file:
file.write("Hello, World!\n")
file.write("Python is awesome!\n")
读取文本文件
with open("example.txt", "r") as file:
content = file.read()
print(content)
也可以逐行读取文件内容:
with open("example.txt", "r") as file:
for line in file:
print(line.strip())
五、正则表达式处理字符串
正则表达式(Regular Expression, 简称regex)是一种强大的字符串匹配和操作工具。Python的re
模块提供了对正则表达式的支持,可以方便地进行复杂的字符串匹配和替换操作。
5.1、基本用法
以下是一些常用的正则表达式操作:
re.search(pattern, string)
:在字符串中搜索符合模式的子字符串,返回第一个匹配的对象。re.match(pattern, string)
:从字符串的起始位置开始匹配模式,返回匹配的对象。re.findall(pattern, string)
:在字符串中找到所有符合模式的子字符串,返回一个列表。re.sub(pattern, repl, string)
:将字符串中所有符合模式的子字符串替换为指定的字符串。
示例:
import re
text = "The quick brown fox jumps over the lazy dog."
搜索模式
match = re.search(r"\bfox\b", text)
if match:
print("Found:", match.group()) # 输出: Found: fox
匹配模式
match = re.match(r"The", text)
if match:
print("Matched:", match.group()) # 输出: Matched: The
查找所有匹配
matches = re.findall(r"\b\w{3}\b", text)
print("Matches:", matches) # 输出: Matches: ['The', 'fox', 'the', 'dog']
替换模式
result = re.sub(r"\blazy\b", "active", text)
print("Result:", result) # 输出: The quick brown fox jumps over the active dog.
六、字符串编码和解码
在处理不同编码的字符串时,Python提供了内置的编码和解码方法,可以方便地在不同编码之间转换。
6.1、字符串编码
可以使用str.encode()
方法将字符串编码为字节对象:
text = "Hello, World!"
encoded_text = text.encode("utf-8")
print(encoded_text) # 输出: b'Hello, World!'
6.2、字符串解码
可以使用bytes.decode()
方法将字节对象解码为字符串:
encoded_text = b'Hello, World!'
decoded_text = encoded_text.decode("utf-8")
print(decoded_text) # 输出: Hello, World!
七、处理字符串模板
Python的string
模块提供了Template
类,可以方便地使用字符串模板进行文本的动态生成。
7.1、基本用法
以下是Template
类的基本用法:
from string import Template
template = Template("Hello, $name! Welcome to $place.")
result = template.substitute(name="Alice", place="Wonderland")
print(result) # 输出: Hello, Alice! Welcome to Wonderland.
7.2、使用安全替换
Template.safe_substitute()
方法可以在缺少某些占位符时,避免抛出异常:
template = Template("Hello, $name! Welcome to $place.")
result = template.safe_substitute(name="Alice")
print(result) # 输出: Hello, Alice! Welcome to $place.
八、处理复杂文本操作
在处理复杂文本操作时,可以结合前面介绍的各种方法和工具,编写高效的字符串处理代码。
8.1、处理CSV文件
CSV(Comma-Separated Values)是一种常见的文本文件格式,Python的csv
模块提供了对CSV文件的读写支持。
import csv
写入CSV文件
with open("example.csv", "w", newline="") as csvfile:
writer = csv.writer(csvfile)
writer.writerow(["Name", "Age", "City"])
writer.writerow(["Alice", 30, "New York"])
writer.writerow(["Bob", 25, "Los Angeles"])
读取CSV文件
with open("example.csv", "r") as csvfile:
reader = csv.reader(csvfile)
for row in reader:
print(row)
8.2、处理JSON数据
JSON(JavaScript Object Notation)是一种常见的数据交换格式,Python的json
模块提供了对JSON数据的解析和生成支持。
import json
data = {
"name": "Alice",
"age": 30,
"city": "New York"
}
将Python对象转换为JSON字符串
json_string = json.dumps(data)
print(json_string) # 输出: {"name": "Alice", "age": 30, "city": "New York"}
将JSON字符串转换为Python对象
parsed_data = json.loads(json_string)
print(parsed_data) # 输出: {'name': 'Alice', 'age': 30, 'city': 'New York'}
九、总结
在Python中加入文字的方法和技巧多种多样,从基本的字符串操作到高级的文本处理工具,都提供了丰富的功能和灵活性。通过熟练掌握这些方法和工具,可以有效地处理各种文本操作需求。无论是简单的字符串拼接,还是复杂的文本解析和生成,Python都能提供强大的支持。希望本文的介绍能够帮助你更好地理解和应用Python中的文字处理技术。
相关问答FAQs:
如何在Python中添加文本到文件中?
在Python中,可以使用内置的open()
函数和文件模式来添加文本。例如,使用'a'
模式打开文件,可以将新文本追加到文件末尾。示例代码如下:
with open('example.txt', 'a') as file:
file.write('这是要添加的新文本。\n')
这种方法确保了原有内容不被覆盖,而是将新内容添加到现有内容的后面。
在Python中如何处理用户输入的文本?
可以使用input()
函数来接收用户的输入,并将其存储在变量中。接着,可以将这个变量的内容进行处理或输出。例如:
user_input = input("请输入一些文本:")
print(f"您输入的文本是:{user_input}")
这种方法使得程序能够与用户进行交互,接受并处理用户提供的文本信息。
Python中如何创建带有文本的图形界面?
可以使用tkinter
库来创建图形用户界面,并在窗口中加入文本。以下是一个简单的示例:
import tkinter as tk
root = tk.Tk()
label = tk.Label(root, text='欢迎使用Python图形界面!')
label.pack()
root.mainloop()
通过这种方式,可以轻松创建具有文本说明的窗口应用程序,提升用户体验。
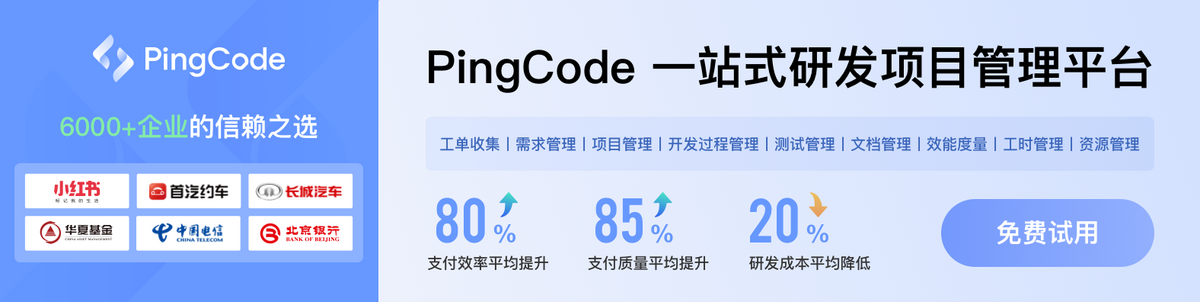