在Python中将内容写入文件,可以使用内置的open
函数来打开文件,并使用write
或writelines
方法将内容写入文件。要写入文件,可以选择使用文本模式或二进制模式。常用的方法包括:使用with
语句确保文件正确关闭、选择正确的文件模式、处理文件异常。例如,使用with open
语句确保文件在操作完成后自动关闭,避免资源泄露的问题。
一、使用open
函数和with
语句写入文件
在Python中,使用open
函数可以打开文件进行读写操作。open
函数有两个主要参数:文件名和模式。常用的模式包括:
'w'
:写入模式。如果文件不存在,会创建一个新文件;如果文件存在,会覆盖原有内容。'a'
:追加模式。如果文件不存在,会创建一个新文件;如果文件存在,新的内容会追加到文件末尾。'r+'
:读写模式。文件必须存在。'b'
:二进制模式。与其他模式结合使用,例如'wb'
表示以二进制写入模式打开文件。
# 使用 'w' 模式写入文件
with open('example.txt', 'w') as file:
file.write('Hello, World!\n')
file.write('This is a new line.\n')
使用 'a' 模式追加内容
with open('example.txt', 'a') as file:
file.write('Appending a new line.\n')
二、使用write
和writelines
方法
write
方法:将字符串写入文件。不自动添加换行符。writelines
方法:接收一个字符串列表,将每个字符串依次写入文件。不自动添加换行符。
# 使用 write 方法写入文件
with open('example.txt', 'w') as file:
file.write('Line 1\n')
file.write('Line 2\n')
使用 writelines 方法写入文件
lines = ['Line 3\n', 'Line 4\n']
with open('example.txt', 'a') as file:
file.writelines(lines)
三、处理文件异常
在进行文件操作时,可能会遇到文件不存在、权限不足等异常情况。可以使用try
…except
语句进行异常处理,确保程序不会因为文件操作异常而崩溃。
try:
with open('example.txt', 'w') as file:
file.write('This is a test.\n')
except IOError as e:
print(f"An I/O error occurred: {e.strerror}")
四、写入二进制文件
除了文本文件外,Python还可以处理二进制文件,例如图片、视频等。使用二进制模式打开文件,并使用write
方法将二进制数据写入文件。
# 写入二进制文件
data = bytes([0x00, 0x01, 0x02, 0x03])
with open('example.bin', 'wb') as file:
file.write(data)
五、文件路径处理
在写入文件时,可能需要处理文件路径。可以使用Python的os
模块或pathlib
模块进行路径处理,确保文件路径正确。
import os
使用 os 模块处理文件路径
file_path = os.path.join('directory', 'example.txt')
with open(file_path, 'w') as file:
file.write('Using os module.\n')
from pathlib import Path
使用 pathlib 模块处理文件路径
file_path = Path('directory') / 'example.txt'
with file_path.open('w') as file:
file.write('Using pathlib module.\n')
六、文件编码
在写入文件时,可以指定文件编码。常见编码包括utf-8
、ascii
等。指定编码可以避免字符编码问题,确保文件内容正确写入和读取。
# 写入文件时指定编码
with open('example.txt', 'w', encoding='utf-8') as file:
file.write('你好,世界!\n')
七、示例:日志记录
日志记录是文件写入的一个常见应用。可以编写一个简单的日志记录器,将日志信息写入文件。
import datetime
def log_message(message, log_file='log.txt'):
timestamp = datetime.datetime.now().strftime('%Y-%m-%d %H:%M:%S')
log_entry = f"{timestamp} - {message}\n"
with open(log_file, 'a') as file:
file.write(log_entry)
log_message("This is a log message.")
log_message("Another log entry.")
八、文件写入的高级技巧
1. 使用内存缓冲区
在处理大量数据时,可以使用内存缓冲区来提高写入性能。Python的io
模块提供了BytesIO
和StringIO
类,可以将数据写入内存缓冲区,然后再一次性写入文件。
import io
使用 StringIO 写入内存缓冲区
buffer = io.StringIO()
buffer.write('Line 1\n')
buffer.write('Line 2\n')
将缓冲区内容写入文件
with open('example.txt', 'w') as file:
file.write(buffer.getvalue())
2. 多线程写入
在某些情况下,可以使用多线程提高文件写入的效率。Python的threading
模块可以实现多线程写入。
import threading
def write_data(file_path, data):
with open(file_path, 'a') as file:
file.write(data)
threads = []
for i in range(5):
data = f"Thread {i} data\n"
thread = threading.Thread(target=write_data, args=('example.txt', data))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
3. 异步写入
Python的asyncio
模块可以实现异步文件写入,提高写入性能。
import asyncio
async def write_data(file_path, data):
async with aiofiles.open(file_path, 'a') as file:
await file.write(data)
async def main():
tasks = []
for i in range(5):
data = f"Async task {i} data\n"
tasks.append(write_data('example.txt', data))
await asyncio.gather(*tasks)
asyncio.run(main())
九、文件写入的最佳实践
1. 确保文件关闭
使用with
语句可以确保文件在操作完成后自动关闭,避免资源泄露。
with open('example.txt', 'w') as file:
file.write('Ensure file closed.\n')
2. 处理文件异常
在进行文件操作时,使用try
…except
语句处理可能出现的异常,确保程序稳定运行。
try:
with open('example.txt', 'w') as file:
file.write('Handle exceptions.\n')
except IOError as e:
print(f"An I/O error occurred: {e.strerror}")
3. 使用合适的文件模式
根据具体需求选择合适的文件模式,例如写入模式、追加模式、二进制模式等。
with open('example.txt', 'a') as file:
file.write('Choose appropriate mode.\n')
4. 指定文件编码
在写入文件时,指定文件编码可以避免字符编码问题,确保文件内容正确写入和读取。
with open('example.txt', 'w', encoding='utf-8') as file:
file.write('Specify file encoding.\n')
十、总结
在Python中,将内容写入文件是一个常见且重要的操作。通过使用open
函数和with
语句,可以方便地打开和关闭文件。使用write
和writelines
方法可以将字符串或字符串列表写入文件。处理文件异常、指定文件编码、使用内存缓冲区、多线程和异步写入都是提高文件写入效率和可靠性的有效方法。通过遵循文件写入的最佳实践,可以确保文件操作的稳定性和正确性。
相关问答FAQs:
如何在Python中打开一个文件进行写入?
在Python中,可以使用内置的open()
函数打开一个文件,并指定文件模式为'w'
(写入模式)或'a'
(追加模式)。例如,with open('文件名.txt', 'w') as file:
可以创建或覆盖一个名为“文件名.txt”的文件,并在其中写入内容。使用with
语句可以确保文件在操作完成后自动关闭。
使用Python写入文件时需要注意哪些事项?
在使用Python写入文件时,有几个关键事项需要注意。首先,确保文件路径正确,避免因路径错误导致的文件未找到错误。其次,选择合适的文件模式,写入模式会覆盖文件内容,而追加模式则会在文件末尾添加内容。此外,确保在写入操作后及时关闭文件,以避免数据丢失或文件损坏。
如何在写入文件时处理编码问题?
在Python中,处理文件编码时,可以在open()
函数中添加encoding
参数。例如,使用open('文件名.txt', 'w', encoding='utf-8')
来确保文件以UTF-8编码进行写入。选择合适的编码格式可以避免读取文件时出现乱码问题,尤其是在处理非ASCII字符时。
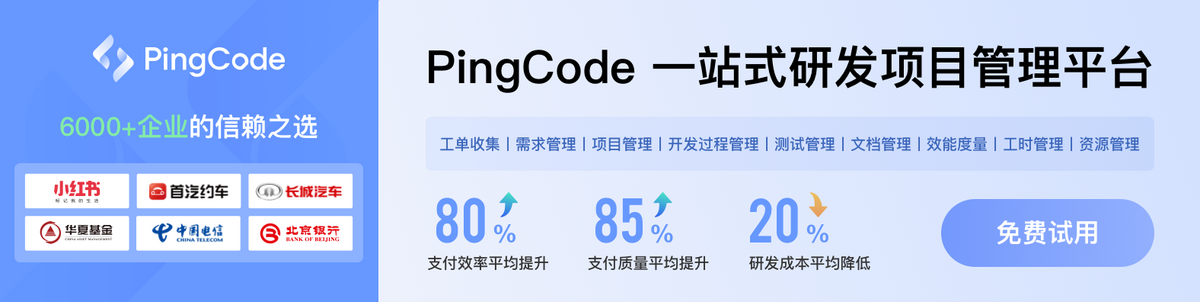