Python保存文件到C盘的方法包括使用内置的open()
函数、使用os
模块来创建目录、使用shutil
模块进行文件复制等。 其中,最常用的方法是使用open()
函数来创建并写入文件。接下来详细介绍如何使用open()
函数保存文件到C盘。
with open(r"C:\path\to\your\directory\filename.txt", "w") as file:
file.write("Your content goes here")
在上述代码中,r
前缀表示原始字符串(防止路径中的反斜杠被转义),"w"
参数表示以写模式打开文件。如果文件不存在,将自动创建它;如果文件已存在,将覆盖其内容。
一、使用open()函数保存文件
1、基础用法
使用open()
函数是最直接和常见的方法。以下是一个简单的例子:
content = "This is the content to be saved in the file."
file_path = r"C:\my_directory\my_file.txt"
with open(file_path, "w") as file:
file.write(content)
在这个例子中,我们指定了文件路径,并使用open()
函数打开文件。如果路径中的目录不存在,需要先创建目录。
2、创建不存在的目录
在保存文件之前,通常需要确保目录存在。可以使用os
模块来创建目录:
import os
directory = r"C:\my_directory"
if not os.path.exists(directory):
os.makedirs(directory)
file_path = os.path.join(directory, "my_file.txt")
with open(file_path, "w") as file:
file.write(content)
3、追加模式
如果你希望将内容追加到现有文件中而不是覆盖,可以使用追加模式"a"
:
with open(file_path, "a") as file:
file.write("\nAdditional content to be appended.")
二、使用os模块进行文件操作
1、os.path模块
os.path
模块提供了一些有用的函数来处理文件路径:
import os
file_path = r"C:\my_directory\my_file.txt"
获取文件名
file_name = os.path.basename(file_path)
获取目录名
directory = os.path.dirname(file_path)
判断文件是否存在
file_exists = os.path.exists(file_path)
2、创建和删除目录
可以使用os
模块创建和删除目录:
# 创建目录
os.makedirs(r"C:\my_directory\sub_directory", exist_ok=True)
删除空目录
os.rmdir(r"C:\my_directory\sub_directory")
三、使用shutil模块复制和移动文件
shutil
模块提供了高级的文件操作功能,例如复制和移动文件:
1、复制文件
使用shutil.copyfile()
可以复制文件内容:
import shutil
source_path = r"C:\source_directory\source_file.txt"
destination_path = r"C:\destination_directory\destination_file.txt"
shutil.copyfile(source_path, destination_path)
2、移动文件
使用shutil.move()
可以移动文件:
shutil.move(source_path, destination_path)
四、处理大文件
1、逐行读取和写入
对于大文件,逐行处理可以减少内存使用:
source_path = r"C:\source_directory\source_file.txt"
destination_path = r"C:\destination_directory\destination_file.txt"
with open(source_path, "r") as source_file, open(destination_path, "w") as destination_file:
for line in source_file:
destination_file.write(line)
2、分块读取和写入
分块处理可以更高效地处理大文件:
chunk_size = 1024 * 1024 # 1MB
with open(source_path, "rb") as source_file, open(destination_path, "wb") as destination_file:
while chunk := source_file.read(chunk_size):
destination_file.write(chunk)
五、使用pandas保存数据
如果处理的是数据框,可以使用pandas
库保存数据到文件,例如CSV或Excel:
import pandas as pd
data = {
"Column1": [1, 2, 3],
"Column2": ["A", "B", "C"]
}
df = pd.DataFrame(data)
df.to_csv(r"C:\my_directory\my_data.csv", index=False)
同样地,可以将数据保存为Excel文件:
df.to_excel(r"C:\my_directory\my_data.xlsx", index=False)
六、使用pickle模块保存对象
在Python中,可以使用pickle
模块保存和加载对象:
1、保存对象
import pickle
data = {"key": "value"}
file_path = r"C:\my_directory\my_data.pkl"
with open(file_path, "wb") as file:
pickle.dump(data, file)
2、加载对象
with open(file_path, "rb") as file:
loaded_data = pickle.load(file)
七、处理文件异常
在文件操作中,处理异常是非常重要的。可以使用try-except
块来捕获并处理可能的异常:
try:
with open(file_path, "w") as file:
file.write(content)
except IOError as e:
print(f"An IOError occurred: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
八、使用第三方库
有时,使用第三方库可以简化文件处理任务。例如,pathlib
库提供了面向对象的文件路径操作:
from pathlib import Path
file_path = Path(r"C:\my_directory\my_file.txt")
file_path.parent.mkdir(parents=True, exist_ok=True)
with file_path.open("w") as file:
file.write(content)
九、多线程和多进程文件操作
在某些情况下,使用多线程和多进程可以提高文件操作的效率:
1、多线程
import threading
def write_to_file(file_path, content):
with open(file_path, "w") as file:
file.write(content)
threads = []
for i in range(5):
thread = threading.Thread(target=write_to_file, args=(f"C:\\my_directory\\file_{i}.txt", f"Content for file {i}"))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
2、多进程
import multiprocessing
def write_to_file(file_path, content):
with open(file_path, "w") as file:
file.write(content)
processes = []
for i in range(5):
process = multiprocessing.Process(target=write_to_file, args=(f"C:\\my_directory\\file_{i}.txt", f"Content for file {i}"))
processes.append(process)
process.start()
for process in processes:
process.join()
十、文件加密和解密
1、使用第三方库进行加密
可以使用cryptography
库对文件进行加密和解密:
from cryptography.fernet import Fernet
生成密钥
key = Fernet.generate_key()
cipher_suite = Fernet(key)
加密文件
with open(file_path, "rb") as file:
file_data = file.read()
encrypted_data = cipher_suite.encrypt(file_data)
with open(file_path, "wb") as file:
file.write(encrypted_data)
解密文件
with open(file_path, "rb") as file:
encrypted_data = file.read()
decrypted_data = cipher_suite.decrypt(encrypted_data)
with open(file_path, "wb") as file:
file.write(decrypted_data)
十一、文件压缩和解压缩
1、使用zipfile模块
可以使用zipfile
模块压缩和解压缩文件:
import zipfile
压缩文件
with zipfile.ZipFile(r"C:\my_directory\my_archive.zip", "w") as zipf:
zipf.write(file_path, arcname="my_file.txt")
解压缩文件
with zipfile.ZipFile(r"C:\my_directory\my_archive.zip", "r") as zipf:
zipf.extractall(r"C:\my_directory\extracted_files")
十二、总结
在Python中,保存文件到C盘的方法有很多,包括使用open()
函数、os
模块、shutil
模块以及第三方库如pandas
、pickle
等。不同的方法适用于不同的场景,可以根据具体需求选择合适的方法进行文件操作。同时,处理文件操作中的异常、使用多线程和多进程提高效率、对文件进行加密和压缩等也是非常重要的技巧。通过掌握这些方法和技巧,可以更加高效和安全地进行文件操作。
相关问答FAQs:
如何在Python中指定文件保存路径?
在Python中,可以通过指定完整的文件路径来保存文件到C盘。例如,使用open()
函数时,可以传入C盘的路径,如C:\\Users\\YourUsername\\Documents\\example.txt
。确保路径中使用双反斜杠\\
或者原始字符串r'C:\Users\YourUsername\Documents\example.txt'
来避免转义字符的问题。
Python保存文件时遇到权限问题怎么办?
如果在尝试将文件保存到C盘时遇到权限问题,可以考虑以管理员身份运行你的Python IDE或脚本,或者选择一个用户有写入权限的目录,如用户的文档文件夹。如果需要在系统文件夹中保存文件,请确保你有足够的权限。
如何在Python中保存不同格式的文件到C盘?
Python支持多种文件格式的保存,例如文本文件、CSV文件和Excel文件。可以使用内置的open()
函数保存文本文件,使用pandas
库的to_csv()
方法保存CSV文件,以及使用pandas
的to_excel()
方法保存Excel文件。确保在保存时提供正确的文件路径和格式后缀。
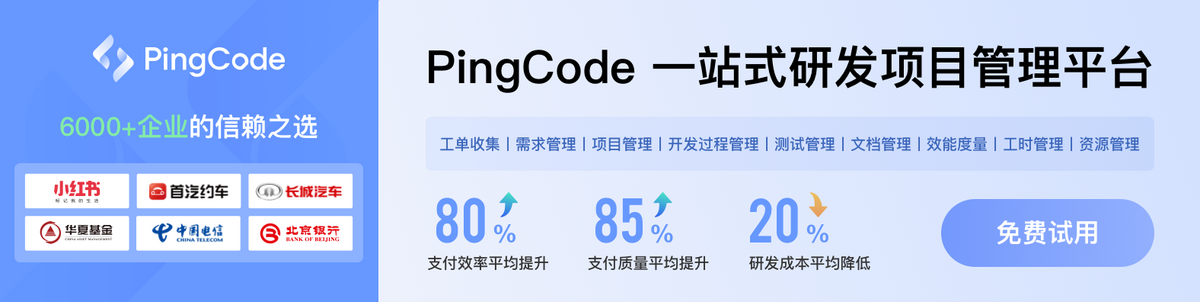