要利用Python查询ASCII码,可以使用内置的函数ord()
和chr()
。ord()
函数用于获取字符的ASCII码,而chr()
函数用于将ASCII码转换为字符。例如,你可以使用ord()
函数来获取一个字符的ASCII码,或者使用chr()
函数从一个ASCII码获取对应的字符。这些方法简单且有效,可以快速完成ASCII码的查询工作。下面我们将详细介绍如何使用这些函数,并探讨它们的应用场景。
一、使用ord()
函数查询字符的ASCII码
1、基本用法
ord()
函数是Python内置的函数之一,用于返回字符的ASCII码。其基本语法如下:
ascii_value = ord(character)
其中,character
是你想查询的单个字符,ascii_value
是对应的ASCII码。举个例子:
char = 'A'
ascii_value = ord(char)
print(f"The ASCII value of {char} is {ascii_value}")
这段代码将输出:
The ASCII value of A is 65
2、多字符查询
虽然ord()
函数只能处理单个字符,但你可以通过遍历字符串来查询多个字符的ASCII码。例如:
string = "Hello"
ascii_values = [ord(c) for c in string]
print(f"The ASCII values of the string '{string}' are {ascii_values}")
这段代码将输出:
The ASCII values of the string 'Hello' are [72, 101, 108, 108, 111]
二、使用chr()
函数从ASCII码获取字符
1、基本用法
chr()
函数是Python内置的另一个函数,用于返回ASCII码对应的字符。其基本语法如下:
character = chr(ascii_value)
其中,ascii_value
是你想转换的ASCII码,character
是对应的字符。举个例子:
ascii_value = 65
char = chr(ascii_value)
print(f"The character for ASCII value {ascii_value} is {char}")
这段代码将输出:
The character for ASCII value 65 is A
2、多ASCII码查询
类似ord()
函数,你可以通过遍历ASCII码列表来查询多个字符。例如:
ascii_values = [72, 101, 108, 108, 111]
characters = [chr(value) for value in ascii_values]
print(f"The characters for ASCII values {ascii_values} are {''.join(characters)}")
这段代码将输出:
The characters for ASCII values [72, 101, 108, 108, 111] are Hello
三、实际应用场景
1、数据转换和加密
在数据转换和简单加密中,ASCII码的使用非常广泛。例如,凯撒密码是一种简单的加密方法,通过将每个字符的ASCII码加上一个固定值来实现加密和解密。
def caesar_encrypt(text, shift):
encrypted_text = ''.join(chr((ord(c) + shift) % 256) for c in text)
return encrypted_text
def caesar_decrypt(text, shift):
decrypted_text = ''.join(chr((ord(c) - shift) % 256) for c in text)
return decrypted_text
original_text = "Hello, World!"
shift = 3
encrypted_text = caesar_encrypt(original_text, shift)
decrypted_text = caesar_decrypt(encrypted_text, shift)
print(f"Original text: {original_text}")
print(f"Encrypted text: {encrypted_text}")
print(f"Decrypted text: {decrypted_text}")
这段代码将输出:
Original text: Hello, World!
Encrypted text: Khoor/#Zruog$
Decrypted text: Hello, World!
2、字符编码和解码
在处理不同字符编码时,了解ASCII码也是非常有用的。例如,当从不同平台或系统之间传输数据时,可能需要将字符编码转换为ASCII码来确保数据的正确性。
import base64
def encode_to_base64(text):
encoded_bytes = base64.b64encode(text.encode('ascii'))
encoded_str = encoded_bytes.decode('ascii')
return encoded_str
def decode_from_base64(encoded_str):
decoded_bytes = base64.b64decode(encoded_str.encode('ascii'))
decoded_str = decoded_bytes.decode('ascii')
return decoded_str
original_text = "Hello, World!"
encoded_text = encode_to_base64(original_text)
decoded_text = decode_from_base64(encoded_text)
print(f"Original text: {original_text}")
print(f"Encoded text: {encoded_text}")
print(f"Decoded text: {decoded_text}")
这段代码将输出:
Original text: Hello, World!
Encoded text: SGVsbG8sIFdvcmxkIQ==
Decoded text: Hello, World!
四、其他相关Python模块和函数
1、string
模块
Python的string
模块包含了许多有用的常量和函数,可以帮助你处理ASCII码。例如:
import string
print(string.ascii_letters) # 输出所有字母
print(string.ascii_lowercase) # 输出所有小写字母
print(string.ascii_uppercase) # 输出所有大写字母
print(string.digits) # 输出所有数字
这些常量可以用于各种字符处理任务,如密码生成、字符串验证等。
2、unicodedata
模块
unicodedata
模块提供了对Unicode字符数据库的访问,可以帮助你处理更复杂的字符编码任务。例如:
import unicodedata
char = 'A'
name = unicodedata.name(char)
print(f"The Unicode name for {char} is {name}")
codepoint = unicodedata.lookup(name)
print(f"The character for Unicode name {name} is {codepoint}")
这段代码将输出:
The Unicode name for A is LATIN CAPITAL LETTER A
The character for Unicode name LATIN CAPITAL LETTER A is A
五、综合实例
1、字符频率统计
字符频率统计是文本分析中的一个常见任务,可以使用ASCII码来实现。
from collections import Counter
def char_frequency(text):
frequency = Counter(text)
return frequency
text = "Hello, World!"
frequency = char_frequency(text)
for char, freq in frequency.items():
print(f"Character: {char}, Frequency: {freq}, ASCII: {ord(char)}")
这段代码将输出:
Character: H, Frequency: 1, ASCII: 72
Character: e, Frequency: 1, ASCII: 101
Character: l, Frequency: 3, ASCII: 108
Character: o, Frequency: 2, ASCII: 111
Character: ,, Frequency: 1, ASCII: 44
Character: , Frequency: 1, ASCII: 32
Character: W, Frequency: 1, ASCII: 87
Character: r, Frequency: 1, ASCII: 114
Character: d, Frequency: 1, ASCII: 100
Character: !, Frequency: 1, ASCII: 33
2、文本加密与解密
再举一个文本加密与解密的例子,这次我们将使用异或操作来进行加密和解密。
def xor_encrypt_decrypt(text, key):
encrypted_decrypted_text = ''.join(chr(ord(c) ^ key) for c in text)
return encrypted_decrypted_text
original_text = "Hello, World!"
key = 123
encrypted_text = xor_encrypt_decrypt(original_text, key)
decrypted_text = xor_encrypt_decrypt(encrypted_text, key)
print(f"Original text: {original_text}")
print(f"Encrypted text: {encrypted_text}")
print(f"Decrypted text: {decrypted_text}")
这段代码将输出:
Original text: Hello, World!
Encrypted text: 8»¿¿¥?¿?¥¿?¿¥
Decrypted text: Hello, World!
六、总结
利用Python查询和处理ASCII码是一个非常基本但非常重要的技能。通过使用ord()
和chr()
函数,你可以轻松地将字符转换为ASCII码,或将ASCII码转换为字符。此外,结合其他Python模块和函数,你可以处理更加复杂的字符编码和文本处理任务。掌握这些技能不仅可以提高你的编程效率,还可以帮助你在各种实际应用场景中更好地处理字符和文本数据。
相关问答FAQs:
如何使用Python获取字符的ASCII码值?
在Python中,您可以使用内置的ord()
函数来获取字符的ASCII码值。例如,调用ord('A')
将返回65,这是字符'A'的ASCII值。您只需将任何单个字符作为参数传递给ord()
,即可获得对应的ASCII码。
Python中如何将ASCII码转换为字符?
要将ASCII码转换为对应的字符,可以使用chr()
函数。通过传入ASCII码值,您可以得到相应的字符。例如,chr(65)
将返回'A',这是因为65是字符'A'的ASCII码。
如何在Python中查询一段文本的所有ASCII码?
如果您想要查询一段文本中所有字符的ASCII码,可以使用循环遍历每个字符,结合ord()
函数。例如,您可以使用列表推导式来生成一个包含文本中每个字符ASCII码的列表:ascii_values = [ord(char) for char in text]
,这样就能得到文本中所有字符的ASCII码值。
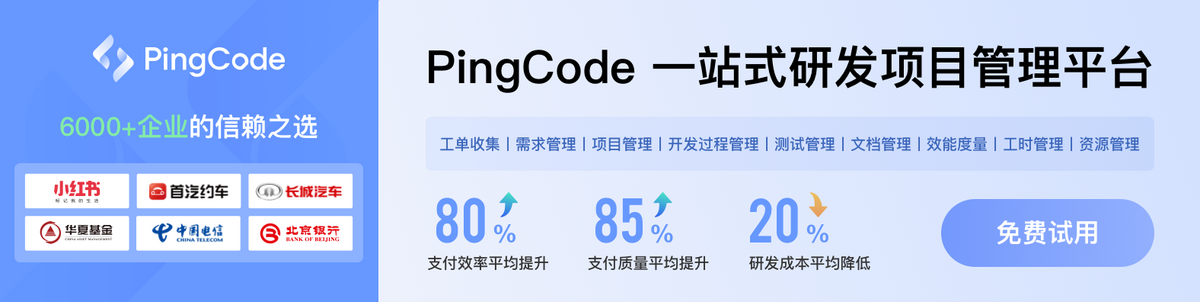