Python可以通过使用网络爬虫、API接口、数据处理库等工具来实现AD采集功能。常见的方法包括:使用requests库进行网页请求、BeautifulSoup库进行网页解析、Scrapy框架进行爬虫开发、使用第三方API获取广告数据、以及使用pandas库进行数据处理。 其中,Scrapy框架是一个非常强大且灵活的网络爬虫工具,适合大规模数据采集任务。
下面将详细介绍如何使用Python实现AD采集功能,包括如何进行网页爬取、数据处理以及如何处理反爬虫机制等。
一、使用Requests库进行网页请求
Requests库是一个简单易用的HTTP库,可以用来发送HTTP请求,从而获取网页内容。
1. 安装Requests库
首先,你需要安装Requests库,可以通过pip命令来安装:
pip install requests
2. 发送HTTP请求
使用Requests库发送HTTP请求,获取网页内容:
import requests
url = 'https://example.com'
response = requests.get(url)
if response.status_code == 200:
content = response.text
print(content)
else:
print(f"Failed to retrieve content, status code: {response.status_code}")
3. 处理网页内容
获取到网页内容后,可以使用正则表达式或者其他解析工具来提取广告数据。
import re
假设广告数据在网页内容中的某个特定位置,可以使用正则表达式提取
ads_pattern = re.compile(r'<div class="ad">(.*?)</div>', re.DOTALL)
ads = ads_pattern.findall(content)
for ad in ads:
print(ad)
二、使用BeautifulSoup库解析网页
BeautifulSoup是一个用于解析HTML和XML的库,可以方便地提取网页中的数据。
1. 安装BeautifulSoup和lxml解析器
pip install beautifulsoup4 lxml
2. 解析网页内容
from bs4 import BeautifulSoup
soup = BeautifulSoup(content, 'lxml')
ads = soup.find_all('div', class_='ad')
for ad in ads:
print(ad.text)
三、使用Scrapy框架进行大规模爬虫开发
Scrapy是一个强大的爬虫框架,适合进行大规模的数据采集任务。
1. 安装Scrapy
pip install scrapy
2. 创建Scrapy项目
scrapy startproject ad_scraper
3. 定义爬虫
在ad_scraper/spiders
目录下创建一个新的爬虫:
import scrapy
class AdSpider(scrapy.Spider):
name = 'ad_spider'
start_urls = ['https://example.com']
def parse(self, response):
ads = response.css('div.ad')
for ad in ads:
yield {
'title': ad.css('h2::text').get(),
'description': ad.css('p::text').get()
}
4. 运行爬虫
scrapy crawl ad_spider
四、使用API接口获取广告数据
有些广告平台提供API接口,可以直接获取广告数据。
1. 获取API Key
首先,你需要在广告平台上申请一个API Key。
2. 发送API请求
import requests
api_url = 'https://api.adplatform.com/get_ads'
api_key = 'your_api_key'
response = requests.get(api_url, headers={'Authorization': f'Bearer {api_key}'})
if response.status_code == 200:
ads = response.json()
for ad in ads:
print(ad)
else:
print(f"Failed to retrieve ads, status code: {response.status_code}")
五、使用Pandas库进行数据处理
Pandas是一个强大的数据处理库,可以用来处理和分析广告数据。
1. 安装Pandas
pip install pandas
2. 数据处理
import pandas as pd
假设广告数据已经存储在一个列表中
ads = [
{'title': 'Ad 1', 'description': 'Description 1'},
{'title': 'Ad 2', 'description': 'Description 2'},
# 更多广告数据...
]
df = pd.DataFrame(ads)
print(df)
保存数据到CSV文件
df.to_csv('ads.csv', index=False)
六、处理反爬虫机制
在进行网页数据采集时,可能会遇到反爬虫机制。以下是几种常见的处理方法:
1. 使用请求头模拟浏览器
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/85.0.4183.121 Safari/537.36'
}
response = requests.get(url, headers=headers)
2. 使用代理IP
可以通过使用代理IP来隐藏真实IP地址,避免被封禁。
proxies = {
'http': 'http://10.10.1.10:3128',
'https': 'http://10.10.1.10:1080',
}
response = requests.get(url, proxies=proxies)
3. 设置请求间隔
通过设置请求间隔,可以避免频繁请求导致被封禁。
import time
for url in urls:
response = requests.get(url, headers=headers)
time.sleep(1) # 请求间隔1秒
七、实际案例:采集广告数据并存储到数据库
下面是一个完整的案例,展示如何采集广告数据并存储到数据库中。
1. 安装必要的库
pip install requests beautifulsoup4 lxml pymysql
2. 创建数据库和表
CREATE DATABASE ad_data;
USE ad_data;
CREATE TABLE ads (
id INT AUTO_INCREMENT PRIMARY KEY,
title VARCHAR(255),
description TEXT
);
3. 编写Python代码
import requests
from bs4 import BeautifulSoup
import pymysql
数据库连接
db = pymysql.connect(host='localhost', user='root', password='password', database='ad_data')
cursor = db.cursor()
发送HTTP请求
url = 'https://example.com'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/85.0.4183.121 Safari/537.36'
}
response = requests.get(url, headers=headers)
if response.status_code == 200:
content = response.text
soup = BeautifulSoup(content, 'lxml')
ads = soup.find_all('div', class_='ad')
for ad in ads:
title = ad.find('h2').get_text()
description = ad.find('p').get_text()
# 插入数据到数据库
sql = "INSERT INTO ads (title, description) VALUES (%s, %s)"
cursor.execute(sql, (title, description))
db.commit()
else:
print(f"Failed to retrieve content, status code: {response.status_code}")
关闭数据库连接
cursor.close()
db.close()
八、总结
通过以上介绍,可以看到Python有多种方式实现广告数据采集功能。使用Requests库进行网页请求、使用BeautifulSoup库解析网页、使用Scrapy框架进行大规模爬虫开发、使用API接口获取广告数据、以及使用Pandas库进行数据处理,都是常见且有效的方法。不同的方法适用于不同的场景,开发者可以根据具体需求选择合适的工具和方法。此外,处理反爬虫机制也是数据采集过程中需要考虑的重要问题,通过合理设置请求头、使用代理IP、设置请求间隔等方法,可以有效应对反爬虫机制。
相关问答FAQs:
如何使用Python进行广告数据采集?
使用Python进行广告数据采集通常涉及网络爬虫技术。可以利用库如Scrapy或Beautiful Soup来抓取网页内容,同时结合Selenium进行动态内容加载的处理。确保遵循网站的robots.txt文件,以避免违反网站的使用条款。
哪些Python库适合广告数据采集?
常用的Python库包括Scrapy、Beautiful Soup和Requests。Scrapy是一个强大的框架,可以处理复杂的爬虫任务,Beautiful Soup则适合解析HTML和XML文档,Requests库方便进行HTTP请求。结合这些工具可以高效实现广告数据的采集。
在进行广告数据采集时,如何处理反爬虫机制?
许多网站会采用反爬虫机制来阻止数据采集。可以通过设置请求头、使用代理IP、模拟用户行为等方式来规避这些限制。此外,适当调整请求频率和间隔也能有效降低被封禁的风险。保持对网站政策的关注,确保采集行为合法合规。
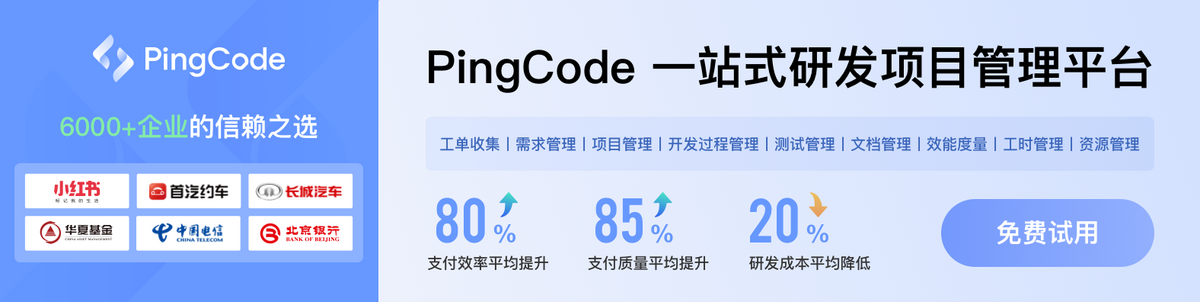