利用Python爬虫下载图片的步骤包括:选择合适的爬虫框架、发送HTTP请求、解析网页内容、提取图片URL、下载并保存图片。选择合适的爬虫框架是整个过程的基础,常用的框架有BeautifulSoup、Scrapy、Selenium等。以下是详细描述选择合适爬虫框架的理由:
选择合适的爬虫框架是非常重要的,因为不同的框架具有不同的特点和适用场景。例如,BeautifulSoup适用于快速解析HTML和XML文档,语法简单易用,适合初学者;Scrapy是一个功能强大的爬虫框架,适用于需要处理复杂爬取任务和大规模数据抓取的场景;Selenium则适用于需要模拟用户操作的动态网页爬取。选择合适的框架不仅能提高工作效率,还能避免不必要的麻烦。
一、选择合适的爬虫框架
1、BeautifulSoup
BeautifulSoup是一个Python库,用于从HTML和XML文件中提取数据。它提供了Pythonic的方式来处理HTML文档的解析和数据提取。以下是使用BeautifulSoup的步骤:
-
安装BeautifulSoup和requests库:
pip install beautifulsoup4
pip install requests
-
使用BeautifulSoup解析网页:
import requests
from bs4 import BeautifulSoup
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
提取图片URL
images = soup.find_all('img')
for img in images:
img_url = img.get('src')
print(img_url)
2、Scrapy
Scrapy是一个用于爬取网站并提取结构化数据的应用框架。它非常适合处理复杂的爬取任务。以下是使用Scrapy的步骤:
-
安装Scrapy:
pip install scrapy
-
创建Scrapy项目并编写爬虫:
scrapy startproject myproject
cd myproject
scrapy genspider example example.com
-
在spider文件中编写爬虫代码:
import scrapy
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['http://example.com']
def parse(self, response):
for img in response.css('img'):
img_url = img.attrib['src']
yield {'image_url': img_url}
3、Selenium
Selenium是一个自动化测试工具,用于模拟用户操作浏览器。它适用于需要处理动态内容的网页。以下是使用Selenium的步骤:
-
安装Selenium和浏览器驱动(如ChromeDriver):
pip install selenium
-
使用Selenium模拟浏览器操作:
from selenium import webdriver
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
driver.get('http://example.com')
images = driver.find_elements_by_tag_name('img')
for img in images:
img_url = img.get_attribute('src')
print(img_url)
driver.quit()
二、发送HTTP请求
在选择好爬虫框架后,下一步是发送HTTP请求,获取网页内容。常用的库有requests和urllib。requests库使用简单,功能强大,是发送HTTP请求的首选。
1、使用requests库
import requests
url = 'http://example.com'
response = requests.get(url)
if response.status_code == 200:
print('Request successful')
html_content = response.content
else:
print('Request failed')
2、使用urllib库
import urllib.request
url = 'http://example.com'
response = urllib.request.urlopen(url)
html_content = response.read()
print(html_content)
三、解析网页内容
解析网页内容是爬取数据的关键步骤。常用的库有BeautifulSoup和lxml。BeautifulSoup适用于简单的HTML文档解析,而lxml适用于处理复杂的XML和HTML文档。
1、使用BeautifulSoup解析HTML
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
print(soup.prettify())
2、使用lxml解析HTML
from lxml import etree
parser = etree.HTMLParser()
tree = etree.fromstring(html_content, parser)
print(etree.tostring(tree, pretty_print=True).decode('utf-8'))
四、提取图片URL
在解析网页内容后,需要提取图片的URL。可以使用BeautifulSoup或lxml库来提取图片URL。
1、使用BeautifulSoup提取图片URL
images = soup.find_all('img')
for img in images:
img_url = img.get('src')
print(img_url)
2、使用lxml提取图片URL
images = tree.xpath('//img')
for img in images:
img_url = img.get('src')
print(img_url)
五、下载并保存图片
最后一步是下载并保存图片。可以使用requests库或urllib库来下载图片,并将其保存到本地。
1、使用requests库下载图片
import os
def download_image(img_url, save_path):
response = requests.get(img_url)
if response.status_code == 200:
with open(save_path, 'wb') as f:
f.write(response.content)
print(f'Successfully downloaded {img_url}')
else:
print(f'Failed to download {img_url}')
img_url = 'http://example.com/image.jpg'
save_path = os.path.join('images', 'image.jpg')
download_image(img_url, save_path)
2、使用urllib库下载图片
import urllib.request
def download_image(img_url, save_path):
urllib.request.urlretrieve(img_url, save_path)
print(f'Successfully downloaded {img_url}')
img_url = 'http://example.com/image.jpg'
save_path = os.path.join('images', 'image.jpg')
download_image(img_url, save_path)
六、处理反爬虫机制
在进行网页爬取时,常常会遇到反爬虫机制,如验证码、IP封禁等。可以使用以下几种方法来应对反爬虫机制:
1、设置请求头
通过设置User-Agent等请求头,可以伪装成正常用户访问网页,避免被识别为爬虫。
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get(url, headers=headers)
2、使用代理IP
通过使用代理IP,可以避免被目标网站封禁IP。可以使用免费的代理IP,也可以购买付费的代理IP服务。
proxies = {
'http': 'http://123.456.789.012:8080',
'https': 'http://123.456.789.012:8080'
}
response = requests.get(url, headers=headers, proxies=proxies)
3、模拟用户操作
通过使用Selenium等工具,模拟用户操作浏览器,可以绕过一些反爬虫机制,如验证码、动态加载等。
from selenium import webdriver
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
driver.get('http://example.com')
模拟用户操作
driver.find_element_by_id('input').send_keys('example')
driver.find_element_by_id('submit').click()
html_content = driver.page_source
driver.quit()
七、存储爬取的数据
在爬取数据后,需要将数据存储到本地或数据库中。常用的存储方式有文件存储和数据库存储。
1、文件存储
可以将爬取的数据存储到本地文件中,如文本文件、CSV文件等。
with open('data.txt', 'w') as f:
f.write(html_content)
2、数据库存储
可以将爬取的数据存储到数据库中,如MySQL、MongoDB等。
import mysql.connector
conn = mysql.connector.connect(
host='localhost',
user='user',
password='password',
database='database'
)
cursor = conn.cursor()
sql = "INSERT INTO images (url) VALUES (%s)"
val = (img_url,)
cursor.execute(sql, val)
conn.commit()
cursor.close()
conn.close()
八、处理大规模数据爬取
在进行大规模数据爬取时,需要考虑性能优化和数据处理效率。可以使用以下几种方法来优化爬虫性能:
1、使用多线程
通过使用多线程,可以同时发送多个请求,提高爬取速度。
import threading
def download_image_thread(img_urls):
for img_url in img_urls:
download_image(img_url, os.path.join('images', img_url.split('/')[-1]))
threads = []
for i in range(10):
thread = threading.Thread(target=download_image_thread, args=(img_urls[i::10],))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
2、使用异步爬虫
通过使用异步爬虫,如aiohttp,可以进一步提高爬取效率。
import aiohttp
import asyncio
async def download_image_async(img_url, session):
async with session.get(img_url) as response:
if response.status == 200:
img_data = await response.read()
with open(os.path.join('images', img_url.split('/')[-1]), 'wb') as f:
f.write(img_data)
print(f'Successfully downloaded {img_url}')
else:
print(f'Failed to download {img_url}')
async def main(img_urls):
async with aiohttp.ClientSession() as session:
tasks = [download_image_async(img_url, session) for img_url in img_urls]
await asyncio.gather(*tasks)
img_urls = ['http://example.com/image1.jpg', 'http://example.com/image2.jpg']
asyncio.run(main(img_urls))
通过选择合适的爬虫框架、发送HTTP请求、解析网页内容、提取图片URL、下载并保存图片,以及处理反爬虫机制和大规模数据爬取,可以高效地利用Python爬虫下载图片。这些步骤不仅适用于图片下载,还可以应用于其他类型的数据爬取。希望本文能为你提供有价值的参考,帮助你在实际工作中更好地利用Python进行数据爬取。
相关问答FAQs:
如何选择合适的Python库进行图片下载?
在Python中,有几个库可以帮助你轻松下载图片,如requests、BeautifulSoup和Scrapy。requests库非常适合处理HTTP请求,能够快速下载单张图片;BeautifulSoup则常用于解析HTML文档,适合从网页中提取图片链接;Scrapy则是一个强大的爬虫框架,适合进行大规模的数据抓取和处理。根据项目的需求选择合适的库,可以提高开发效率。
使用Python爬虫下载图片时需要注意哪些法律问题?
在进行图片下载时,务必遵循相关的法律法规和网站的使用条款。很多网站对其内容有版权保护,未经允许下载和使用这些图片可能会导致法律问题。建议在爬取之前查看网站的robots.txt文件,了解允许抓取的内容,以及是否需要进行授权。
如何处理下载图片时的错误和异常?
在使用Python爬虫下载图片时,可能会遇到网络连接问题、URL错误或文件保存错误等情况。通过使用try-except语句来捕获这些异常,可以确保程序的稳定性。例如,当请求失败时,可以设置重试机制,或在下载图片时检查文件是否已存在,以避免覆盖现有文件。这些措施有助于提高爬虫的鲁棒性和用户体验。
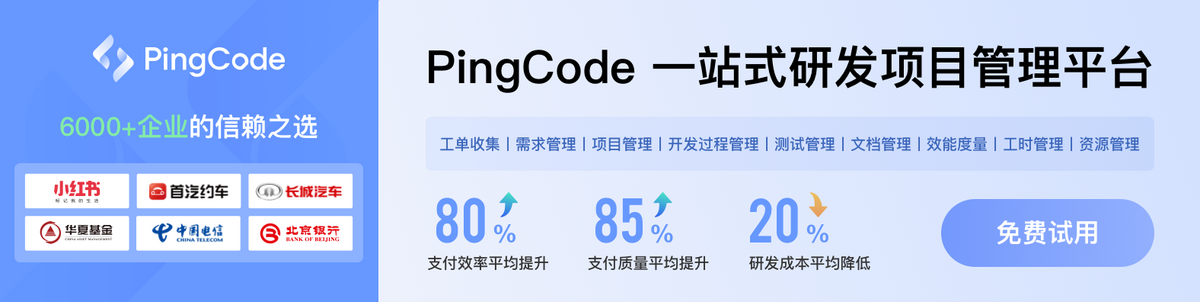